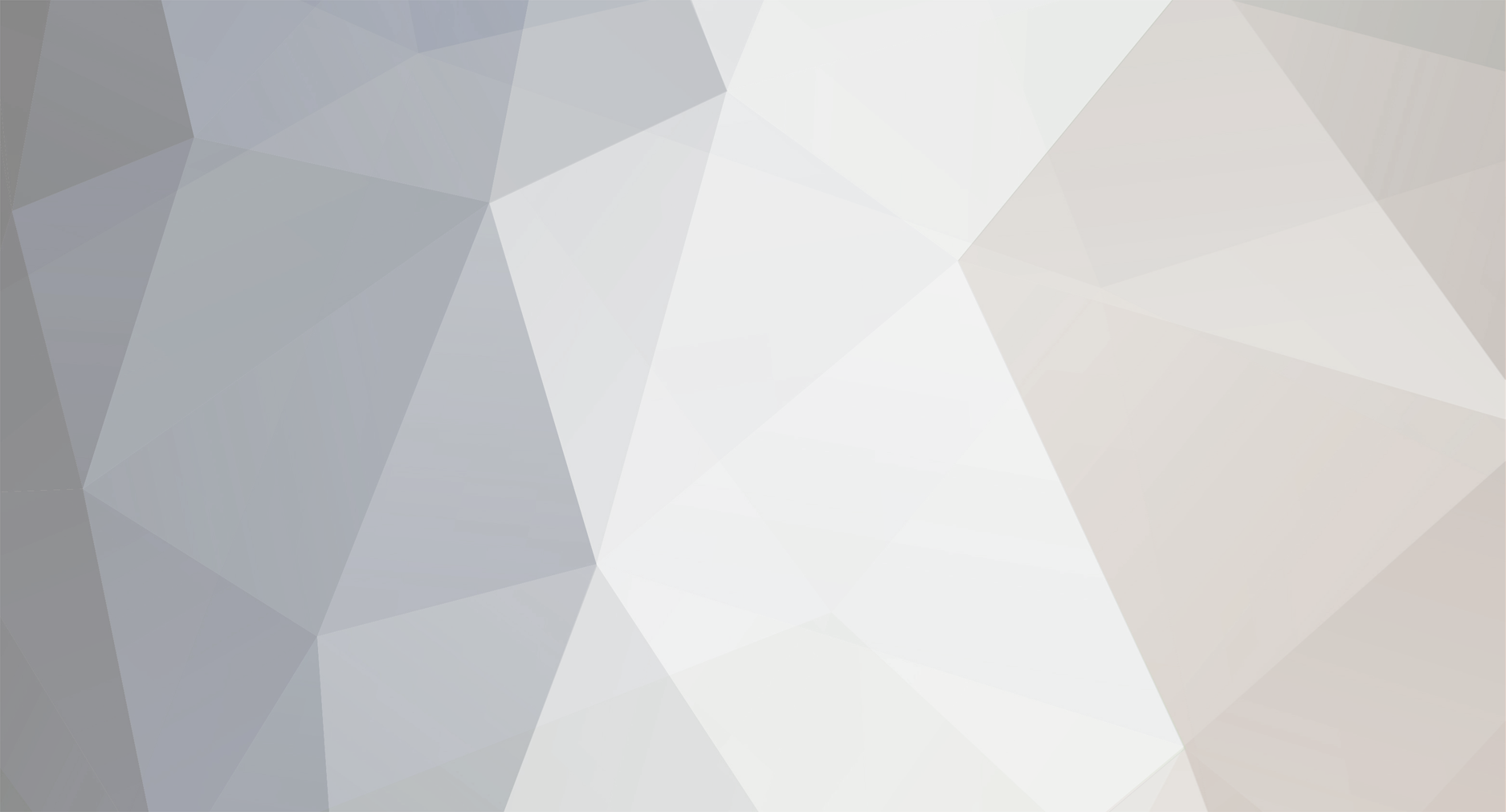
Wolphie
-
Posts
682 -
Joined
-
Last visited
Never
Posts posted by Wolphie
-
-
What is the code for the password function? If I were you, I would just use:
<?php $password = 'mycoolPA55w0rd'; $con = mysql_connect(...); mysql_select_db(...); $query = sprintf("SELECT * FROM `login` WHERE `uname` = '%s' AND `pass` = '%s' LIMIT 1", sha1($password)); // Need code for password()!! $result = mysql_query($query) or trigger_error(mysql_error()); ?>
-
Looks more like a CSS/Mark-up issue. Although if you want some advice I would be using correct mark-up i.e. using head tags for headers, paragraphs for text etc..
-
To center things in IE, you just add the text-align: center; property to the element. Although remember to change it back to text-align: left; when writing text unless you want it to appear in the middle also.
In firefox it's margin: 0 auto;
body {margin:0 auto;text-align:center;width: 900px;} // Remember the body needs to be a fixed width.
-
I think this is what you're looking for: http://validator.w3.org/
-
www.php.net
-
You can create a timestamp field in the database, and when a new record is inserted, it will insert the current time that corresponding record was added. All done automatically. It's in the form of a unix timestamp though, I.e. 01-01-2008 12:45
-
Just use
$val = (float) str_replace(".", "," $val);
-
You would need to submit the form to itself, or use JavaScript and Ajax.
Simple normal example:
<?php if(isset($_POST['submit'])) { if(empty($_POST['name'])) { print 'Please enter a name!'; } } ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="POST"> <input type="text" name="name" /> <input type="submit" name="submit" /> </form>
-
<?php function check_phone_number($cellphone) { if(trim($cellphone) == 10) { return true; } else { return false; } } ?>
This will make sure that the phone number has to be 10 characters. The trim function removes any white spaces...
Sorry i can not do this for you using regular expressions but im sure someone else could if you ask
This wouldn't check to see if the phnone number is 10 characters, it would check to see if it was equal to 10.
if(strlen(trim($cellphone)) == 10) { return true; }
-
Basically, regular expressions are used to detect certain characters/tags in text and gets the value in between them. Depending on which tag is used, the HTML is appropriately formatted. This also helps prevent XSS, and Injections too, by not allowing the user to directly enter HTML tags.
-
Please use the Code BB tags in future. But the answer to your question is to submit the form to itself using $_SERVER['PHP_SELF']
For example:
<?php if(isset($_POST['submit'])) { if(!empty($_POST['name']) { echo $_POST['name']; } else { echo 'Please enter your name!'; } } ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="POST"> <p>Name: <input type="text" name="name" /></p> <p><input type="submit" name="submit" value="Submit" /></p> </form>
-
It should be put outside of the loop i.e.
while(condition) { // Do something... } mysql_free_result($mysql_resource);
If that was me I would remove it, but if you're worried about large memory allocations then by all means keep it. In my experience it hasn't really done anything significant for me.
As I said, this may not be the only problem.
-
You can also add hover properties to elements other than anchors.
-
My favorite editors for each OS..
Windows: phpDesigner 2008, in my opinion this is by far the best IDE, it also has framework support.
Linux: gEdit, the native Gnome text editor, you can change the preferences and use plug-in's to change it into more of an IDE.
Mac: Text-Mate, Text-Mate is my favorite IDE of all time, it supports almost all languages, has dozens of macro's, etc..
-
You should be declaring mysql_free_result() after initiating the loop. At least that's what php.net recommends, personally I don't use mysql_free_result().
However, that may not solve the problem.
-
You haven't really given valid comparisons. Each language can be used for a multitude of things, for example PHP is primarily a web development language, although it's also a scripting language and can be used outside of the web. Dot Net for example covers many aspects, C Sharp, ASP.NET etc..
I will always stick to PHP for web development, although I still find myself inching closer and closer to Python. I'm fluent with C/C++ and those are my primary desktop development languages.
I will *always* avoid Java where ever possible.
I've never really took interest in Ruby, so I've never really used it, and I'm doubtful I ever will.
I use Perl quite often in *nix applications as well as Python.
It's a toughy..
-
Remember to hit "Topic Solved"!
-
Remember to hit "Topic Solved"!
-
Add some error handling, it may indicate whats wrong.
$result = pg_query($query) or trigger_error(pg_error()); // I don't know what the PostgreSQL error function is, I don't use it sorry.
-
You're re-declaring $search, thus replacing the value of it. If somebody has used the GET method, that value will be stored and then replaced by a POST method which will contain no value at all.
Try this:
<?php //Connect to mysql server include 'connect.php'; //Function to sanitize values received from the form. Prevents SQL injection function clean($str) { $str = @trim($str); if(get_magic_quotes_gpc()) { $str = stripslashes($str); } return mysql_real_escape_string($str); } if(isset($_GET['ID'])) { $search = clean($_GET['ID']); } else if(isset($_POST['search'])) { $search = clean($_POST['search']); } $qry = "SELECT * FROM battle_users WHERE cname='$search'"; $result = mysql_query($qry); if($result) { $result_array = mysql_fetch_assoc($result); if($result_array > 0) { include "side.php"; print "</td>"; print "<td valign='top' width=70%>"; print "<table class='maintable'><tr class='headline'><td><center>View Profile</center></td></tr>"; print "<tr class='mainrow'><td>"; print "<br> Character's Profile <br> <br><br>"; ?> <table width="300" border="0" align="center" cellpadding="2" cellspacing="0"> <tr> <center><h2><?php print $result_array["cname"]; ?></h2> </center> <tr> <th> Character's Name :-</th> <td><?php print $result_array["cname"]; ?></td> </tr> <tr> <th> Rank :-</th> <td><?php if($result_array["rank"] == 0){$result_array["rank"] = "Unranked";} print $result_array["rank"]; ?></td> </tr>
Bear in mind you also need to sanitize GET data too!
-
Yes it is, $_SERVER['SCRIPT_NAME']
-
Theres nothing wrong with it, it could prove useful for other people. In my personal experience, the best ever Windows IDE I've found/used is phpDesigner 2008.
-
Simple things tend to be the trickiest, at least in my experience. You're welcome, remember to mark the topic solved.
-
Did you change the necessary values? Such as table name, column name, etc..?
Please post the relevant code you're using.
Is Holding Table Names In A Constants File A Good Idea?
in PHP Coding Help
Posted
I personally don't see any problem with storing them in CONSTANTS, however you really don't want this file being viewable/accessible by users. If I were you, i'd just store them in a database table for convenience.