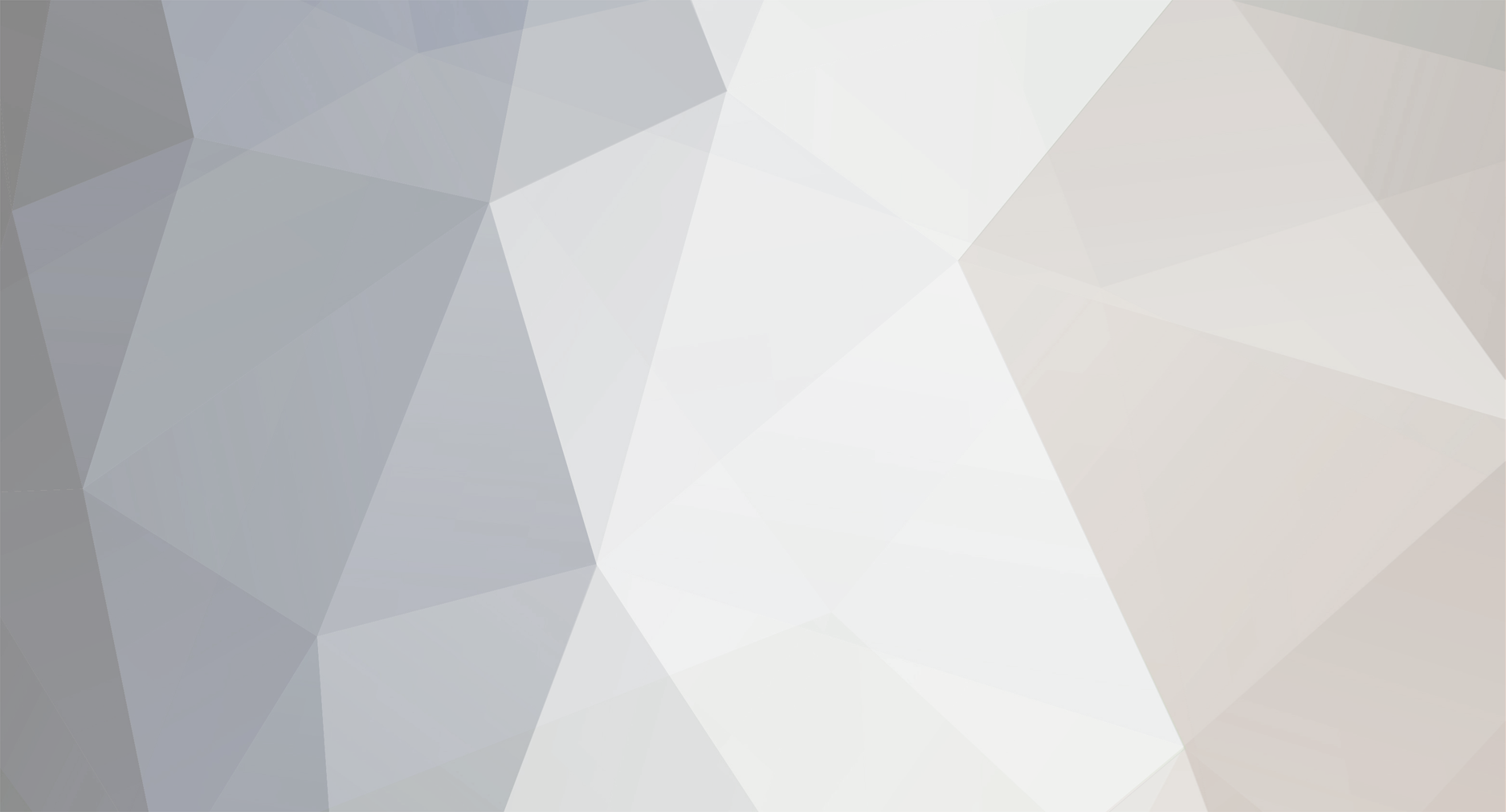
tmallen
-
Posts
112 -
Joined
-
Last visited
Never
Posts posted by tmallen
-
-
No, that's a very bad approach. What if you want all links to appear this way? What you need to do is link a stylesheet:
<link href="path/to/screen.css" media="screen" type="text/css" rel="stylesheet" />
And in screen.css, add:
a { text-decoration: none; }
Also, quote your attributes as in my first example, and get rid of the font tag. Instead, ask yourself, "why does this need to be black?" Let's say that it needs to be black because you hate the site and think it's smelly. In that case, change your link to:
<a href="whateverurl" class="smelly">link text</a> <!-- People know how to open new windows. Only do it for them if you must -->
Then, you'd add this to your screen.css file:
a.smelly { color: black; }
And you could make any smelly links appear in that color. Or, if you want all links to be black, move that "color: black;" line to the other rule ("a {") right below the text-decoration rule.
-
Sorry, internationalization is not my strength...you may be interested in using utf8_encode and/or utf8_decode, which converts between UTF8 chars and ISO-whatever number.
-
I'll bet your answer is here: http://en.wikipedia.org/wiki/IP_address
-
By the way, if you're trying to make a product grid or something similar, you're better off just using floats and adjusting the containing element's width so that only 2, 3, etc. fit per row. That's also the preferred approach because the number per row has very little to do with the nature of the content itself. For that reason, you want to keep this out of your code and use CSS instead. If you still want to enforce a number of elements per row, only do one check ($i % $numberPerRow == 0) and add a class to that item. From your CSS, use "clear: left" and that item will always reset the row.
-
<?php for($i = 1; $i <= $total; $i++) { // I'm not sure if switch accepts expressions. You may need to assign the switch variable beforehand switch($i % 3) { case 1: break; // = 1/3 case 2: break; // = 2/3 case 0: break; // = 3/3 default: break; } }
To do that, you'll need to use this for loop instead. Just fill the cases in with the necessary HTML
-
Oops, didn't actually run the code. Barring that, it should work fine. Also edited the original because $i should start at one, not zero.
-
Do you know if some sort "expires" header involved? Feedburner may even be limiting your IP's refreshes, who knows. Nothing relevant here: http://us.php.net/manual/en/function.simplexml-load-file.php , but you may want to browse that part of the Manual.
-
Easy. Assume an array of 10 elements:
<?php // $data = 10-item array $total = count($data) $html = "<table>"; for($i = 1; $i++; $i <= $total) { // Calculate $total ahead of time, otherwise it checks each loop // Also, note that we start at 1 instead of 0 to make things easier, // otherwise the first item is even (0) if($i % 2 = 1) { // If odd $newHtml = "<tr><td>{$data[$i]}</td>"; } else { // it's even $newHtml = "<td>{$data[$i]}</td></tr>"; } $html .= $newHtml; } echo $html;
-
You should use htmlentitites() to convert these characters. Or, you could make sure that your scripts handle all input and output in UTF8, which would be ideal.
-
How can I add a a row for every error? I know I did this before, but lost the code. The reason that this is necessary: I want errors to build into a table which is positioned absolutely (bottom: 1em; left: 1em). I can only have one copy of the table, otherwise errors overlap each other.
What I have now doesn't work, and I'm not entirely sure why (I know it's crappy code, but error handling is a black box indeed, plus this is for a prototype CSS framework, so I'm not being picky):
<?php $errors = "<table class='phpError'>" . "<tr><th>Level</th><th>Error</th><th>File</th><th>Line</th></tr>"; $errorCount = 0; function golondrinaErrors($number, $string, $file, $line) { global $errors; global $errorCount; $errorCount++; $errors .= "<tr><td>" . $number . "</td>" . "<td>" . $string . "</td>" . "<td>" . $file . "</td>" . "<td>" . $line . "</td>" . "</tr>"; return true; } if($errorCount > 0) { $errors .= "</table>"; echo $errors; } set_error_handler('golondrinaErrors');
-
Oops, missed the first sticky. I'd delete this post if I could.
-
I'm writing my new company site from scratch (the current one just uses a header and footer include and injects $title plus a couple other things, not much in the way of a dynamic setup), and I really wish I could find some good resources on PHP site/application design. The closest I have to this is "PHP in Action" (which is great for other reasons). And, I know, I can look at how other software is set up.
-
Yes. Nano, Pico, ed, etc.
-
Would you guys be interested in me porting my SyntaxHighlighter plugin for Vanilla to SMF? It works very well, see for yourself.
-
Thanks, that fixed it. Feel so unnecessary...
-
Please, please wrap your code in BBCode "code" tags so that it prints as code and syntax highlights. I can't fight through this.
-
With this sort of code:
<?php //... $this->sortState = $_GET[$name . 'Sort'] ? $_GET[$name . 'Sort'] : self::DEFAULTSORT; //... ?>
E_ALL reporting throws this notice:
Notice: Undefined index: YearsSort in ScriptName.php on line 16
depending on which $name . 'Sort' isn't in the URL. But that ternary checks if it exists, right? So why do I get that notice? Obviously, the script works fine, and I shouldn't stress over this sort of notice, but I'd like to know what the preferred way is to fix it. An (!empty($_GET[...])) check?
-
Sounds simple enough. If a user is changing their info, obviously you don't throw an error when the new email is the same as the old one, only if it's the same as another user's. A new user would perform the same check, except there'd be no need to see if their new email is the same as a former address, as they're a new user.
-
Any advice?
-
Also, this is for escaping/transforming output, not filtering input.
-
Edit: I've replaced the manual < and > substitution lines with the simpler:
<?php $hlString = htmlspecialchars($String, ENT_NOQUOTES); ?>
It seems to be working fine.
A big hurdle for me is that I want to further build this parser. I'd like it to, at the very least, convert URLs to links and maybe even add some basic HTML/markdown/BBCode support.
-
This extension loads some stylesheets and scripts, then extends the StringFormatter class to provide my own forum post parser. The parser does only four things:
1,2. Replace < with < and > with >
3. Replace
[code/] with <pre title="code"/>, andwith <pre title="code" class="$1"/> 4. Replace all newlines not occurring in a "pre" block with an HTML line break Judging by how complex the Regex needed to be for step four, I know I'm doing something very wrong. Here's the class: [code]<?php class SyntaxHighlighterFormatter extends StringFormatter { function Parse($String, $Object, $FormatPurpose) { if ($FormatPurpose == FORMAT_STRING_FOR_DISPLAY) { $hlString = $String; // Steps 1 and 2 $hlString = str_replace('<', '<', $hlString); $hlString = str_replace('>', '>', $hlString); // Step 3 $hlString = preg_replace("/\[code( lang=\"([A-Za-z]+)\")?\]/", "<pre title=\"code\" class=\"$2\">", $hlString); // I had to remove part of the close "code" tag because the PHPFreaks forum was // butchering the code otherwise. $hlString = str_replace('/code]', '</pre>', $hlString); // Step 4 $hlString = preg_replace("#(?:\r\n|[\r\n])(?=(?:[^<]|<(?!/pre))*(?:<pre|\Z))#", "<br />", $hlString); return $hlString; } // This extension only needs to deal with 'FORMAT_STRING_FOR_DISPLAY' else { return $String; } } } ?>
Any advice? Specifically, I'm interested in the best way to parse the Post string, as I'm sure that my current method is impractical. Note that it does execute steps 1-4 perfectly every time, so nothing's functionally broken.
-
1. There's no reason you can't hold onto all of that data client-side using JavaScript
2. If that's too fragile, make each tab switch "forward" trigger a DB save using a simple AJAX request, and if you want to drop this data between tabs, use another request to fetch the form data from the server when navigating to a previously visited tab.
Both ways are easy to implement.
-
Updated again, now with legend and radio type included. Any advice is appreciated. Should I be breaking this up into more functions/classes at this point?
<?php class Form { public $elements; function __construct($elements, $method, $action, $legend) { $this->elements = $elements; $this->method = $method; $this->action = $action; $this->legend = $legend; } public function build() { $html = '<form action="' . $this->action . '" method="' . $this->method . '">'; $html .= '<fieldset>'; $html .= "<legend>$this->legend</legend>"; foreach($this->elements as $name => $properties) { switch($properties['type']) { case 'text': case 'textarea': $html .= "<label for='$name'>{$properties['title']}</label>"; break; } switch($properties['type']) { case 'text': $html .= "<input type='text' name='$name' id='$name' />"; break; case 'textarea': $html .= "<textarea name='$name'></textarea>"; break; case 'radio': $html .= '<fieldset>'; foreach($properties['options'] as $id => $title) { $html .= "<input type='radio' id='$id' value='$id' name='$name' />"; $html .= "<label for='$id'>$title</label>"; } $html .= '</fieldset>'; break; } } $html .= '</fieldset>'; $html .= '</form>'; return $html; } } $formFields = array( 'name' => array( 'title' => 'Name', 'type' => 'text' ), 'comments' => array( 'title' => 'Comments', 'type' => 'textarea' ), 'preferred_com' => array( 'type' => 'radio', 'options' => array( 'email' => 'Email', 'phone' => 'Phone', 'fax' => 'Facsimile' ) ), 'submit' => array( 'title' => 'Submit Form', 'type'=> 'submit' ), ); $myForm = new Form($formFields, 'post', 'form.php', 'Signup'); echo $myForm->build();
Underlined Hyperlink
in HTML Help
Posted
Allow me to approach this from a practical point of view: Nobody makes something some way for a completely arbitrary reason. There is always a purpose when it comes to websites. If you're just coloring links for fun, your users will be confused. But if there's some semantic reason, you want to build that in a maintainable manner.