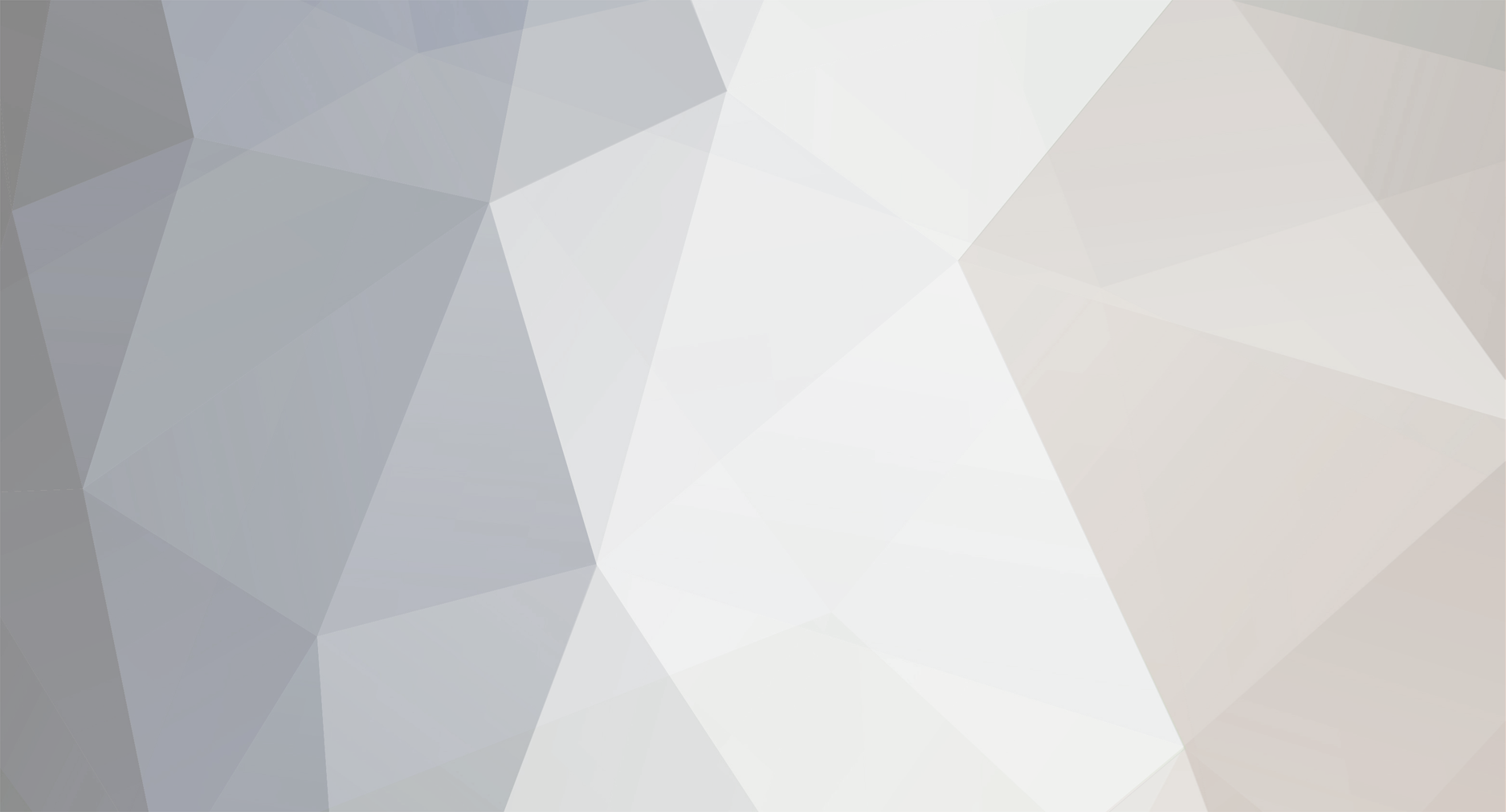
tmallen
-
Posts
112 -
Joined
-
Last visited
Never
Posts posted by tmallen
-
-
Man, you're way above my head here. Could you please explain in more detail?
-
I have a <dl> list of my services for my business website. The way a <d> works is that every <dt> is coupled with a <dd>, although there's no way to know this from the code (you just put you list in the order <dt></dt><dd></dd><dt></dt><dd></dd> so that is makes sense visually. Anyway, here's what I'd like.
My services list has my most important services (Web Design, etc.) at the top. I'd like the visitor to have the option of sorting these alphabetically. How would a script be written that graps the <dt> tags and matches them with their <dd> tags, sorts these pairs based on the first letter of the <dt> tag, and then prints them in alphabetical order?
-
I tried sessions on the first line, and it still didn't work.
-
That can't happen. If I do that, the entire authentication system breaks down:
<?php include '../includes/header.php'; $admin = TRUE; ?>
The $admin flag is too late then, and they've already gained access.
EDIT: Your advice unfortunately didn't work. I moved session_start() to the top of the pages I've been testing, and the login still fails in IE6.
-
I have a fairly simple login script that won't work in Internet Explorer 6. Errors aren't returned for a false login, and correct logins don't work either:
From login.php
<form action="../scripts/login.php" method="post"> <fieldset> <ol> <li><label>Username: <em>*</em></label><input type="text" name="username" /></li> <li><label>Password: <em>*</em></label><input type="password" name="password" /></li> <?php if ($_SESSION['error']) { echo "<p class='clear alert'><strong>".$_SESSION['error']."</strong></p>"; session_destroy(); } ?> <p><input type="submit" value="Login" /></p> </ol> </fieldset> </form>
The script this refers to is the other login.php:
<?php $username = $_REQUEST['username']; $password = $_REQUEST['password']; session_start(); $_SESSION['uid'] = $username; $_SESSION['pass'] = $password; header("Location: "); // this redirects to the user's home URL on my site, and works fine. omitted for security ?>
And the header.php section that checks for an authenticated session:
if ($admin) { $users = array( // Array of usernames matched to passwords. Omitted for security reasons ); if ($_SESSION['pass'] == $users[$_SESSION['uid']]) { $passed = TRUE; } if ($passed != TRUE) { $_SESSION['error'] = 'Login incorrect'; header("Location: http://"); // returns user to login page. omitted for security } if (!$_SESSION['uid'] || !$_SESSION['pass']) { header("Location: http://"); //returns user to login page. omitted for security } }
And any page that should require authentication has this in the top:
<?php $admin = TRUE; ?>
So why does this work in every browser except IE6? Seems odd.
-
I need to hide a part of my site from certain pages, all of which can be found in the projects/ directory. How can I test for this being in the current URL?
-
Wait, it does work, but the page is still loading. How can I kill the process?
-
It doesn't work though.
-
I want a simple user login page/script that does not run over a DB. The main reason for this is to ease deployment and because security is not a huge deal for the data being protected. Here's what I'm trying now. It isn't working.
The form at login.php:
<form action="../scripts/login.php" method="post"> <fieldset> <label>Username: <span class="alert">*</span></label><input type="text" name="username" /> <label>Password: <span class="alert">*</span></label><input type="password" name="password" /> <input type="submit" value="Login" class="submit clear" /> <div class="clear"></div> </fieldset> </form>
The script at ../scripts/login.php:
<?php $username = $_REQUEST['username']; $password = $_REQUEST['password']; session_start(); $_SESSION['uid'] = $username; $_SESSION['pass'] = $password; header("Location: The admin homepage"); ?>
Part of includes/header.php to check for access:
session_start(); if ($admin) { if ($_SESSION['uid']!='admin' || $_SESSION['pass']!='adminpw') { echo("Permission denied for $username at $password\n"); } }
And at the top of a sample admin page:
<?php $title = 'Downloads'; $admin = TRUE; include '../includes/header.php'; ?>
Right now, permission is not being denied in any way to false logins, and at the top of the page I get "Permission denied for at".
So basically, it sets two session variables (I have no prior session experience) based on form input, and checks these variables at every $admin page. I know I'm doing many, many things wrong. Please point them out, but be gentle :^)
-
No, it's just a friendly email. The only data being pulled from the form that gets into the body is the person's name.
$name = $_REQUEST['name']; $company = $_REQUEST['company']; $title = $_REQUEST['title']; $address = $_REQUEST['address']; $address2 = $_REQUEST['address2']; $city = $_REQUEST['city']; $state = $_REQUEST['state']; $phone = $_REQUEST['phone']; $email = $_REQUEST['email']; // Define the recipient, subject, and body $to = "$name <$email>"; $subject = 'Client Company Mailing List Registration'; $message = " <html> <head> <title>$subject</title> </head> <body> <div style='font: 11px verdana; line-height: 1.3; color: #333;'> <p>$name,</p> <p>Thank you for signing up with the Client's Company Name mailing list.</p> <p>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.</p> <p>Client's Company Name</p> </div> </body> </html> ";
-
I'm in a tough spot here. New registrants to my mailing list will be sent a non-interactive "confirmation" email, which will be based directly on what they enter in the Email field. How can I protect this from being exploited by spammers? Is there any risk of someone exploiting my hard-coded $body field which accepts other data using variables earlier defined with the $_REQUEST method? This is my primary concern.
-
Oye! Touche. Many thanks!
-
In a way this works but the "Additional Headers" line is no good. Here's what I see in the result email. The code follows.
Fake Name From: Sitename Cc: fakemail@email.com Bcc: fakemail2@email.com// Additional headers $headers .= 'Fake Name <myfakemail@email.com>' . "\r\n"; $headers .= 'From: Sitename' . "\r\n"; $headers .= 'Cc: fakemail@email.com' . "\r\n"; $headers .= 'Bcc: fakemail2@email.com' . "\r\n";
Note that I've censored the names and emails for privacy. The additional headers didn't successfully affect the From, To, Cc, or Bcc successfully.
The HTML mail does work very well though.
-
OK, thanks. I'll give it a try.
-
How can I make that send HTML email? I know how to use mail() for text emails.
-
I have two scripts, neither of which have been written, that will need to collect user information. They will either write to a flat file or to a MySQL DB, and I need a way for an administrator to grab an Excel file based on this data.
First, no more than 100 people are expected to sign up, so flat file is certainly viable. Do you recommend I use DB or flat file?
Second, based on your recommendation, how should I go about exporting the user data to an Excel file? There will be about ten form fields per registration to give you an idea.
-
I need to send HTML email using PHP, and the solution needs to be very portable (no need for server installations using PEAR, which as far as I know puts the mime_mail extension out of the question). How can I do this?
So, anyone know of portable, fairly easy to use HTML email solutions?
-
I have a form I've been using for a couple years to send email to me as feedback from my website. Now I'd like to use it to save a subscriber's information for a mailing list. Ideally, it will be moved into an XML file that I can easily output with XSLT. Anyway, here's the script. What would I need to modify to make this change? Not that the print line at the end is just there as a placeholder for a true redirect page.
<?php # Mailing List Script for {recipient} by {my name} 2007 $to = 'myemail@myemail.com'; $subject = 'Mailing List Signup'; $mailheaders = 'From: websiteURL'; // Grab the form and assign to variables $name = $_REQUEST['name']; $company = $_REQUEST['company']; $title = $_REQUEST['title']; $address = $_REQUEST['address']; $address2 = $_REQUEST['address2']; $city = $_REQUEST['city']; $state = $_REQUEST['state']; $phone = $_REQUEST['phone']; $email = $_REQUEST['email']; // Send the mail $body = "Name: " .$name."\n". "Company: " .$company."\n". "Title: " .$title."\n". "Address: " .$address."\n". "Address 2: " .$address2."\n". "City: " .$city."\n". "State: " .$state."\n". "Phone: " .$phone."\n". "Email: " .$email."\n"; mail ($to, $subject, $body, $mailheaders); print "sent successfully!"; ?>
-
One last thing, identical paths beginning with "/" are working for images, etc. but failing on plain include() statements. Why would this be?
-
First you set up mod_rewrite to direct all requests through index.php.
How do I do this? I don't have SSH access, is there a way to do this with PHP or something?
-
Where should I put constants.php?
-
OK, thanks. Let me wrap my brain around this one for a minute...
-
Problem is I can't edit $_SERVER['Doc...']. I would just precede my statements with the root indicator ('/') if that were the case. I'm declaring my own site root here, so that no matter where I deploy, I only have to change a single variable to adapt to the server and directory structure.
-
Oh, I understand my problem (sort of). Obviously $base couldn't be called because $base is defined in header.php! What's a better way of declaring this $base variable so that I can easily use it throughout the site? My thinking just feels...backward.
Also, $base calls within my PHP code don't work even when added properly with $base defined (include() calls, mainly), but <?php print $base; ?> works fine inline (calling an image, stylesheet, etc.).
PHP to sort <dt> and <dd> items?
in PHP Coding Help
Posted
Please?