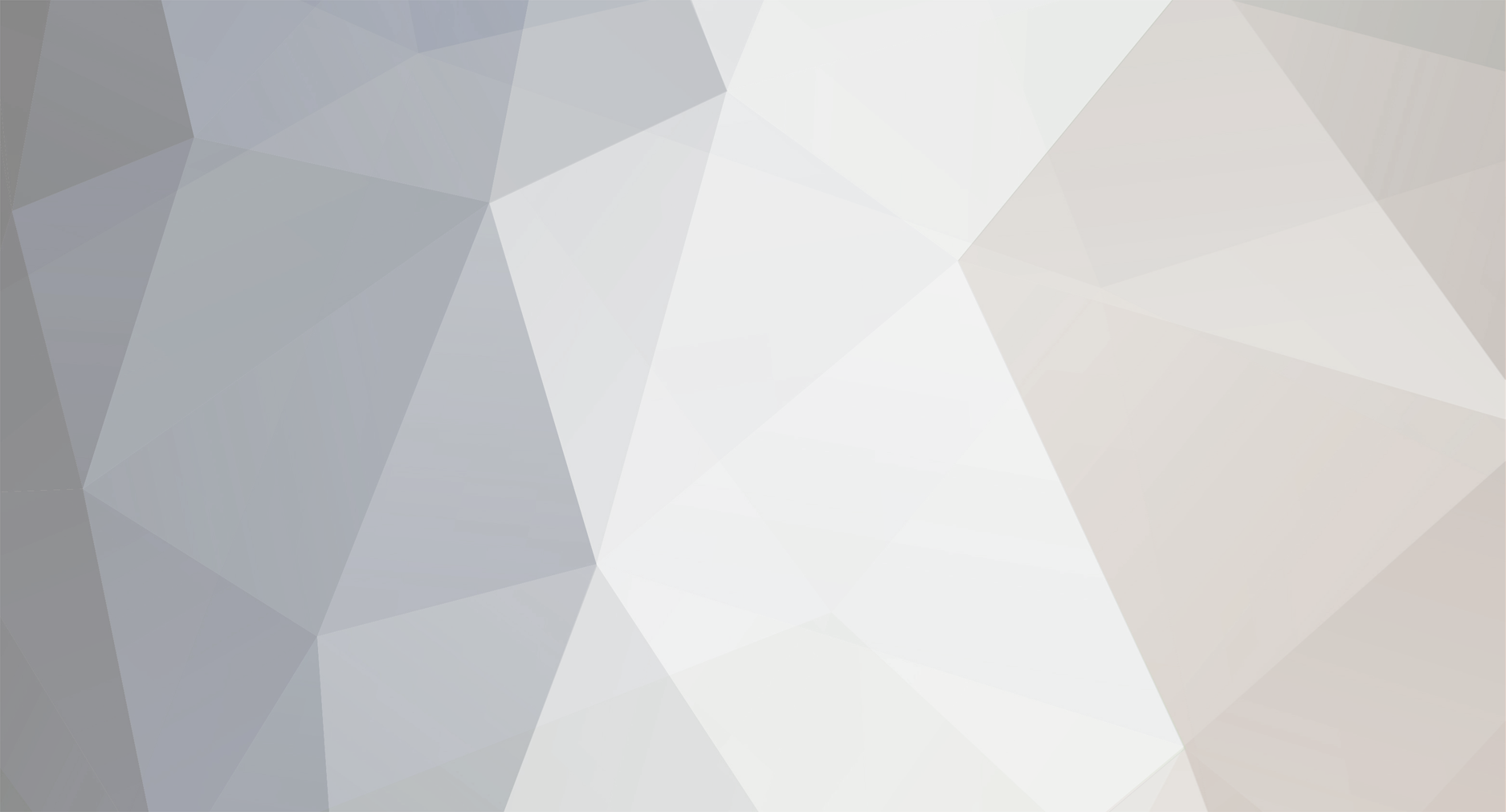
tmallen
-
Posts
112 -
Joined
-
Last visited
Never
Posts posted by tmallen
-
-
Thanks! I'm more interested in the proper application of OOP techniques for either generating HTML, in this case for a form, or better yet, for filling a view. There really aren't any "Build your own MVC framework using OOP" books floating around at my bookstores...My PHP knowledge is pretty good, but I'm hitting a wall right now as I try to implement OO techniques. Maybe I should write an imperative/procedural series of functions and try to convert it to an Object??
-
This type of code would be inside index.php
-----------------------------------------
include('header.php')
if(isset($_GET['page'])){
switch($_GET['page']){
case 1 : include('page1.php');
break;
case 2 : include('page2.php');
break;
case 3 : include('page3.php');
break;
default: include('main.php');
}
else{
include('main.php');
}
}
include(footer.php);
I think that this is a better method, correct me if I'm wrong:
<?php if (isset($_GET['id'])) { $page = 'page' . $_GET['id'] . '.php'; if (!include_once $page) { include_once 'main.php'; } }
Not sure about include_once's return value, and I haven't tested it locally. Give it a try though. And this is just for arguments sake; there are much better ways to do this I'm sure.
-
I'm trying to really learn OOP for PHP so I can reuse more code locally. The problem is, knowing all of the terminology hasn't done a thing for me as far as producing real results. I want to write a class that easily generates and processes a standard mail form. It could be more generic even and have a mail() function.
So, from scratch:
<?php class Form { public function display() { } }
Where do I start as far as outputting an HTML form based on, say, a hash? Please point out anything and everything wrong here, because I don't know very much about using OOP practically.
- Really f'ing confused...
-
Well, if you build your navigation dynamically, you can just test the value against page_id...a simple example:
<?php $pages = array( 'home' => 'Home', 'about' => 'About Us', 'contact' => 'Contact Us', ); $page_id = $_GET['page_id']; foreach($pages as $id=>$title) print "<a href=\"index.php?page_id={$id}\"".($id==$page_id?" class=\"current\"":"").">{$title}</a>"; ?>
I think I like this one best. It's like what I was hacking on last night, but cleaner. I hadn't thought of using the key as both the URL and ID; I was defining the two separately.
-
I was up late last night hacking on a new (to me) way to do selected links. I got pretty far, but I'd like to hear how you guys do it. Is there a better method than the horribly tedious (and often recommended):
<a class="<?php if($page_id == "home") echo "current "; ?>"
My solution used a GET variable which mod_rewrite transformed into a friendly URL to identify the current page. Then, the navigation was generated from an associative array for which the key was the link ID, and the value for this key was another array with the human link title and another item for the url. Links are generated from this array (which could be fetched from the DB) and the current page ID is checked against each nav item's key.
I can post the code later this evening once I'm out of the office.
-
Hate to go on something blindly. I've read over the docs for ob_start(), and it's not entirely clear to me what this function will do when called at the start of the page, and why I should use it.
-
My server's been throwing errors when I send a late header after using the mail() function to send myself user feedback from a site contact form. I know that all headers have to be sent right away, so what's the way to redirect the user after the form's been sent successfully? Right now, I have the form and its script in the same file, so form action="contact.php" which is the form's page.
<?php if (empty($errors)) { // $to, $subject, $message, and $headers are all assigned values // Send the email mail($to, $subject, $message, $headers); // This line seems to be generating header() errors. // Check header() functionality to correct header("Location: ./thanks.php"); } else { echo "\n<p>There were errors sending your message:</p>\n<ul>\n"; foreach ($errors as $message) { echo "<li>$message</li>\n"; } echo "</ul>\n"; } ?>
How do I send users to thanks.php after mail() works?
-
Here's my working solution. It's far from perfect, but gets the job done well. Any advice about the regex, especially if I can somehow remove the need for my two substr() calls, will be much appreciated. Thanks!
for ($i=0; $i < 10; $i++) { echo "<a href=\"". $scores['channel']['item'][$i]['link'] ."\" title=\"". $scores['channel']['item'][$i]['description'] ."\">"; echo $scores['channel']['item'][$i]['title']; echo "</a><br />"; preg_match("/^[A-Z][A-Za-z]*/", $scores['channel']['item'][$i]['title'], $match); preg_match("/[0-9][0-9]*/", $scores['channel']['item'][$i]['title'], $match2); preg_match("/,.[A-Z][A-Za-z]*/", $scores['channel']['item'][$i]['title'], $match3); preg_match("/[0-9]*[0-9].\(F\)$/", $scores['channel']['item'][$i]['title'], $match4); $results = array(); $results[$i]['TeamA'] = $match[0]; $results[$i]['ScoreA'] = $match2[0]; $results[$i]['TeamB'] = substr($match3[0], 2); $results[$i]['ScoreB'] = substr($match4[0], 0, -3); echo " <table border='1'> <tr> <td>".$results[$i]['TeamA']."</td> <td>".$results[$i]['ScoreA']."</td> </tr> <tr> <td>".$results[$i]['TeamB']."</td> <td>".$results[$i]['ScoreB']."</td> </tr> </table> "; }
The table format is just so I can easily see how the data's being separated.
-
Here's the string I can parse in with an RSS feed:
// Returns true "Hornets 118, Knicks 110 (F)" == $scores['channel']['item'][0]['title'];
So I'm working with scores in the format: "TeamA ScoreA, TeamB ScoreB (F)". I'd like to be able to break up the above string into these four parts, and have it work for various game results: "TeamA", "ScoreA", "TeamB", "ScoreB".
I understand that I can do this with Regex, and I even have a good idea of what my rules should be to grab these parts. I just can't translate them into Regex. "TeamA" begins with a Capital letter and ends with a lower-case letter preceding a space and a number. "ScoreA" is any number of digits, ending with a comma. I can strip the comma later, or exclude it with the search, whichever is easier. "TeamB" begins with the first capital letter after that comma and ends with a lower-case letter before a space, before a parentheses.
What regex patterns, using preg_match($pattern, $input, $output), I'm assuming, will do this?
-
If I want to redirect all .jpg images to a single .jpg file, how would I do it with .htaccess and mod_rewrite? Right now I have a rule that works except when the files are with the same ending. Ideally, the first part would exclude the exact filename of the destination file. Here's what I have now:
<IfModule mod_rewrite.c> RewriteEngine On RewriteRule (.)jpg ImageA.jpg </IfModule>
This causes an internal server error, of course, because it repeats forever. How can I exclude ImageA from this rule?
EDIT: This works now, and I think it's because I stopped defining the paths on each side. In any case, how can I exclude ImageA with certainty?
-
I've been using HostGator for about sixteen months now for my personal sites, company site, and a handful of client websites. Today, there was a big problem with downtime on the server, and I can't afford to have this happen in the future. Plus, I can easily afford more than $10/month, which I pay for my current account which has performed well to this point. What's a good, reasonably priced *AMP host? SSH access and such are also nice to have, if possible, since MySQL through phpMyAdmin can be a pain.
I've considered MediaTemple ( http://www.mediatemple.net/webhosting/gs/ ), but have also heard stories about their reliability. Any takes?
And any recommendations will be appreciated.
- Just another PHP freelancer
P.S. (edit) Having access to PostgreSQL would be a nice bonus, and other scripting languages as well (mainly Ruby, Python, and maybe Perl).
-
I chmodded all relevant files to 775. The cache script does make the output files 775. My point was to be able to generate these product pages using the few PHP files, and not needing to use a caching method.
-
I'm using a dynamic method to generate pages in a Yahoo store. In short, Yahoo has some funny-looking HTML comments that its servers understand how to parse into relevant product information. I was wondering why my pages weren't rendering properly, and I was able to solve my problems by using a cache script: Yahoo will only render these comments properly if the file is set to 775, so using chmod() to the result file was enough to make the generated pages work normally.
This all feels very roundabout: Is there a way to make my files rendered dynamically (not the cache ones, the older method I explained) be recognied as 775 by the server? I'd really like to avoid having to cache all of these pages.
Thanks!
-
Never mind, found a nice solution here. It's unfortunately JS-based, but seems to work well: http://code.google.com/p/syntaxhighlighter/
-
How can I pluck out all instances of, for example <div class="foo">...</div> with all enclosing code selected? I'm customizing phpBB to do specific code highlighting, in this case, for CSS. I'll assign each instance to a single array, foreach that (to process the code), and echo the "highlighted" HTML with a number of <spans>.
Would this be any easier if I just had phpBB insert unique HTML comments for this BBCode instance, grabbing the contents with Regex? <!-- End CSS --> is easier to pick out than any of a number of </div>, but if PHP has a sense of XHTML open/close rules and nesting, I should be able to take the simpler approach.
-
I worked in an ASP.NET environment for about 14 months, and one feature I always liked was the reliance on a simple "web.config" file that handled many global app settings. One obvious use I can think of for this would be to have the web app's root set by this sort of XML attribute, and to have a separate config file on my server and localhost. That way, I could easily preview all of my changes locally while having the web app work fine on my server.
Any tips? Thanks!
-
Hold on, left out the most important part:
<?php $file_list = glob("*"); echo "<ul>\n"; foreach ($file_list as $file) { echo " <li><a href=\"$file\">$file</a></li>\n"; } echo "</ul>\n"; ?>
-
Which is better? I think that this is certainly more readable:
<?php echo "<ul>\n"; foreach ($file_list as $file) { echo " <li><a href=\"$file\">$file</a></li>\n"; } echo "</ul>\n"; ?>
-
I've noticed that glob() doesn't return my hidden files. However, the following method does. Is there an option to return hidden files with glob()?
if ($handle = opendir('./')) { while (false !== ($file = readdir($handle))) { echo "<li><a href=\"$file\">$file</a></li>"; } }
-
OK, cool, that works. I browsed the docs for glob(), but I can't figure out how to grab the name of the current directory. How can I track this down?
-
I'd like a script that will list all files in the present working directory (pwd) as links. How can this be done? I don't know much about using PHP to read local files. Is there something like the "ls" command to do this with PHP?
-
I'd prefer to do this with PHP so that handling is done server-side, before the page begins loading. In any case, it shouldn't be an issue for the moment.
-
Haha, you have to be kidding me about needing a dedicated server for 10GB. I regularly share 30GB of data between family and friends (music, and so on), and my host is $10 per month, HostGator. I also host my own project sites (4) and the sites of eight clients.
-
Not tables here, links for about seven services. On my business site.
OOP for Forms?
in PHP Coding Help
Posted
OK, I'm slowly getting this. Can anyone take a look here and point out what I'm doing right/wrong? Note that I know I haven't taken care of every input type.