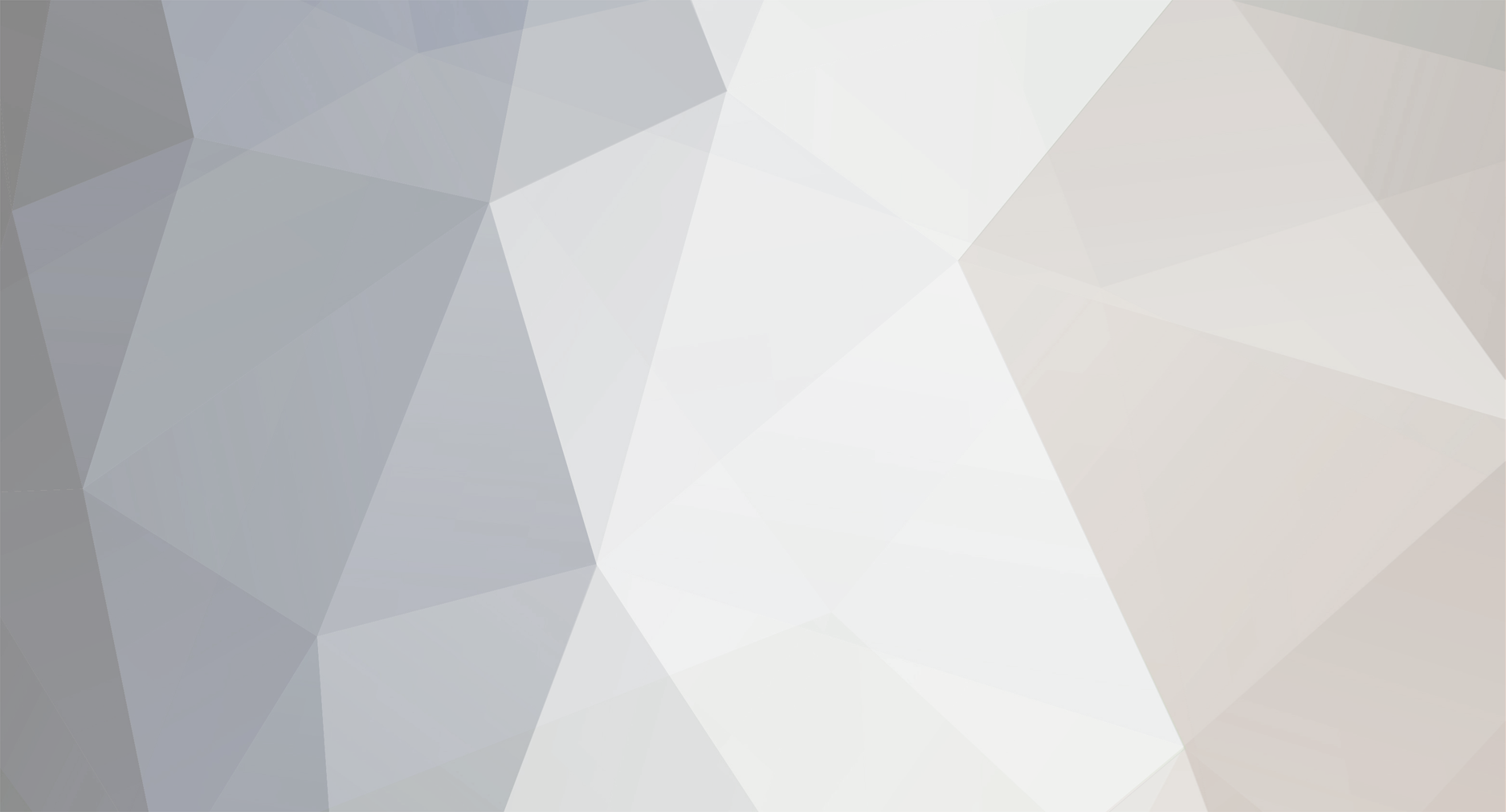
codebyren
Members-
Posts
156 -
Joined
-
Last visited
Everything posted by codebyren
-
Implications of CURLOPT_FOLLOWLOCATION for Amazon S3
codebyren replied to Jonob's topic in PHP Coding Help
The CURLOPT_FOLLOWLOCATION option being set to true just tells cURL to follow redirects set by the server at the address being accessed. It's been a while since I've worked with Amazon S3 (I can barely remember it) but I'd think it's extremely unlikely that they would change the URL to which you post your data, and therefore possibly redirect you elsewhere. Also, if they were to change the URL, I don't believe the post data would carry to the new page anyway - but I may be wrong or there may be ways to configure cURL to keep the post intact. This should be easy enough to test on your own server just by uploading one file that prints the $_POST array and another that redirects to it. Then just post to the redirecting page via cURL and see what your result is. -
You're heading in the right direction but are traversing the data incorrectly. <?php $json = file_get_contents('weather.json'); $json = json_decode($json); // You can learn a lot about how PHP interpreted the data by un-commenting these lines: // print_r($json); // exit; // Now it's just a case of following the nested structure to the "forecastday" array. // (which is an array of objects as requinix mentioned) foreach ($json->forecast->simpleforecast->forecastday as $day) { echo "{$day->date->weekday}: {$day->conditions} <br>"; echo "{$day->low->fahrenheit} - {$day->high->fahrenheit} °F <br>"; echo '<br>'; } exit; ?> Hope that helps clear it up.
-
Without seeing what you've tried (if anything), it's difficult to know where you're at. For example, are you at least managing to capture the post data in your proposed curl.php file before sending it to the other php file? There's a decent tutorial here that covers posting to another URL using cURL. Have a look at the aptly named "POSTing to a URL" section. That should at least help you out with what I'd say is the more difficult part of your problem.
-
Why not just go with what people are used to? Look at twitter, this forum and, well, any site similar to the one that you are working on as an example. These get by with restricting usernames to something like 15 alphanumeric characters and an underscore or two. And since several hundred million people have been ok with that, I think you should run with it.
-
Problem linking to a file when using javascript in php
codebyren replied to facarroll's topic in PHP Coding Help
Where your javascript code is being printed out, you are outside of PHP tags - so "urlencode" means nothing and the periods to concatenate your string also mean nothing. You need to "re-enter PHP" so functions etc. are recognised: var my_javascript_url = 'check/check.php?quizTitle=<?php echo urlencode($quizTitle); ?>'; -
Just as a side note, you could look at htmlpurifier for removing html tags etc. I believe it's very thorough. Then, what's wrong with PHP's strtolower function for converting characters to lower case? Finally, do you have a sample document? I don't see why a single preg_replace() with a whitelist of characters shouldn't work and am curious to try.
-
If you are set on using xml_parser_create etc. then please ignore this post because I'm basically just suggesting an alternative that (to me) seems a bit easier. Otherwise, you could look into using simplexml or even DOMDocument. They might be be a little tricky to start with, particularly because of the namespaces in your sample XML document (and the object oriented approach), but once you catch on it's easy usually enough to keep your head above the water. Consider simplexml: <?php $xml = file_get_contents('xml/tim.xml'); $xml = simplexml_load_string($xml); // Note that we call children($namespace, true) often. // This is to indicate which namespace (e.g. 'farm' in <farm:name>) we're interested in. $farms = $xml->children('export', true) ->account_item->children('cust', true) ->account->children('cust', true) ->farms->children('farm', true); echo 'There are ', count($farms), ' farms. <br>'; foreach ($farms as $farm) { $name = $farm->children('farm', true)->name; echo 'Current farm in loop: ', $name, '<br>'; $fields = $farm->children('farm', true)->businessfields->children('businessfield', true)->field; if ($fields) { foreach ($fields as $field) { $label = $field->children('businessfield', true)->label; echo "Found a field with label $label in farm $name<br>"; } } } exit; ?> The nested objects basically follow the flow of the XML document. Without namespaces, the above might be as simple as something like: <?php $farms = $xml->account_item->account->farms; // And then loop through each farm and do whatever you want with it. ?>
-
It would be a lot easier to go with the standard of having the same code that generates the form, process the form. But since you've been guided down this unfortunate path (at least in my view) , you could look into sessions. Sessions can be used to store data between/across page requests so you can: 1. Store a copy of the $_POST array in the session so you can repopulate the form with the user's previously entered data 2. Store the validation error messages in the session The session data will then still be available after the user has been redirected back to the login form and you can handle it as appropriate (and remove it from the session when you're done)
-
Well a 10+ second loading time for a page is unreasonable - this was just an example to illustrate the throttling that seems to be in place. Caching is basically just storing the result of an expensive (complex/slow) operation so that you can have direct access to the result at a later stage without having to redo the slow/expensive operation. In your XML example, if the data at those URLs doesn't change all that often or your application doesn't require the absolute latest data, you could fetch the data once, and save a copy of it somewhere else (e.g in a database, a file or memcache etc.) along with an expiry of a few minutes/hours. Then when the data is needed, your application can check your cached copy of the data before resorting to fetching fresh data via the URLs you provided (slow) and updating your cache.
-
It looks like there's some kind of throttle in place to limit how frequently you can request the URLs. When I try in rapid succession, at least one of my requests always gets met with a 403 (Forbidden). The following seems to work ok so you may need to cache responses if you're planning on working with them a lot: <?php $xml1 = 'http://www.psnapi.com.ar/ps3/api/psn.asmx/getGames?sPSNID=Lilgezus'; $xml2 = 'http://www.psnapi.com.ar/ps3/api/psn.asmx/getPSNID?sPSNID=Lilgezus'; $xml1 = simplexml_load_string(file_get_contents($xml1)); sleep(10); # wait 10 seconds before loading the next URL $xml2 = simplexml_load_string(file_get_contents($xml2)); echo "The Game Title from the XML in URL #1 is: {$xml1->Game->Title} <br>"; echo "The ID pulled from the XML at URL #2 is: {$xml2->ID}"; exit; ?>
-
The, 'tmp_name' is the absolute path to the temporary file that your server processes the uploaded file as. You can move the uploaded file to wherever you want it to be using this path once you are happy that it passes your requirements (e.g. file type, size etc.) You could look at the W3 schools site for a decent-enough breakdown of the $_FILES array: http://www.w3schools.com/php/php_file_upload.asp If I recall correctly, a lot of the info in the array is provided by the user's browser during upload though so you can't really trust it (e.g. reported file type or size). For this reason, you'll want to use PHP functions to actually access the file and determine it's attributes as part of your validation. Finally, the $_FILES['thumbnail']['error'] value could be one of several values detailed here: http://www.php.net/manual/en/features.file-upload.errors.php
-
The 'userfile' key in the array is determined by what you named your file field in the HTML form. In the following case: <input type="file" name="thumbnail"> .. it would be $_FILES['thumbnail']['tmp_name'] It works this way since you might have multiple file fields in your form and would need to be able to differentiate between them.
-
Well, for a start you seem to be missing the FROM `table_name` JOIN `another_table_name` ON ... part of your statement. Try simplify the statement - then once you know you're on the right track and can see results from both tables, add the BETWEEN etc.
-
Echoing from within a function (particularly one that generates a rather random value each time) prevents you from using that result elsewhere. Imagine you wanted to generate an address for a user and also stash it in a database. You would call the function and it would be displayed to the user - but how would you capture that value for the database since if you were to call the function again, a different address would be generated. So sometimes it's better to: <?php $address = my_function(); # now we have a copy of the result. save_value_to_database($address); # save the address to the database echo $address; # show the address to the user ?> As for the second part of your question, I have to go so will try answer it later if no one else has.
-
In addition to what Pikachu2000 said, you could also look for a tutorial on "variable scope". This should cover how even though you assigned your final value to $address within your function, it won't be available as $address outside of the function. Variable Scope
-
( ! ) Parse error: syntax error, unexpected $end in (line 77)
codebyren replied to Lorenzdakid's topic in PHP Coding Help
You are missing a closing curly brace for your printNumber110 function. The switch statement inside this function closes fine but not the function itself. -
To associate a qty field to a product ID, you can name the qty fields as an array with the product ID as the key. Something like: <?php echo "<tr><td>" . $row['product_code'] . "</td><td>" . $row['product_name'] . "</td><td><input type='text' maxlength = '3' value='0' name='qty[". $row['product_id'] . "]'></td></tr>"; ?> Then once the form has been submitted (assuming data was submitted using the post method), you can loop through the qty fields that were submitted and determine which have values greater than 0: <?php $products = isset($_POST['qty']) AND is_array($_POST['qty']) ? $_POST['qty'] : array(); if ($products) { foreach ($products as $product_id => $qty) { $qty = (int) $qty; $product_id = (int) $product_id; if ($qty > 0) echo "User wants $qty of product with ID $product_id <br>"; } } ?> So basically, you need to be looking for tutorials on forms and processing forms with PHP - these will be quite easy to find via Google.
-
It's possible your HTML contains a repeated broken tag somewhere and so it just looks like only some results are showing. View the generated page's source to make sure all the results aren't actually in the output. For example, this line of yours has a broken <hr /> tag at the end: $search_output ="<hr /> 0 results for <strong>$searchquery</strong><hr /> $sql_command <hr />****ELSE**POST = $_POST[searchquery] Count=$count, Query= $query<hr /" ; Otherwise, try inspecting the query results before printing any HTML to the page using something like: <?php $results = array(); while($row = mysql_fetch_array($query, MYSQL_NUM)) { $results[] = $row; } print_r($results); exit; ?> Are they all there?
-
It sounds like your variables are not what you think they are or should be. Try echo the variable containing the date from the database and also your constructed date to make sure they are in the format you expect and contain the date/time you expect. As long as these values are in the correct format, the code I provided should work the difference in minutes out just fine. Just keep in mind that if the difference between the times is less than 1 minute, it will say 0 minutes, not something like 0.5 minutes. If you still have problems, post your updated code here and I'll take a closer look.
-
Try take this out from inside your while statement (since the while statement means your are already looping through the results): $row = mysql_fetch_array($query); Then, as a side note, you should look into SQL injection and XSS (cross site scripting) and how to overcome these vulnerabilities in your code. There are quite a few of these, including the use of $_SERVER['PHP_SELF'] to set your form's action URL (which introduces a means for malicious users to inject code into your page).
-
Is it possible to do Window Programming in PHP?
codebyren replied to G.kanojiya's topic in PHP Coding Help
If you mean desktop applications then yes, although I don't think it is very common. You could look into Winbinder or PHPGTK and go from there. -
When you use the DateTime class and its diff method: <?php $date1 = new DateTime('2012-01-30 02:43:13'); $date2 = new DateTime('2012-01-31 05:00:00'); $interval = $date1->diff($date2); echo $interval->format("%I minutes"); # prints 16 minutes (which is only part of the difference) ?> This is working out the time/date difference in terms of years, months, days etc. so if you're only using minutes (like above), and the time difference is more than 60 minutes you're ignoring a large portion of the time. E.g. if the time difference were 70 minutes, the above would show only 10 minutes since it stashes the rest of the time as 1 hour - e.g. 1 hour and 10 minutes. If you want the exact number of minutes between two dates from the database, you could do something like: <?php $time1 = strtotime('2012-01-30 02:43:13'); # gets a timestamp (in seconds) $time2 = strtotime('2012-01-31 05:00:00'); $diff = $time2 - $time1; printf("The difference between time1 and time2 is %d minutes", ($diff/60)); # 60 seconds in a minute exit; ?> I hope that helps solve your problem - I got a little lost reading the code you provided.
-
I believe you can stack cases like so: <?php $x = 5; switch ($x) { case 5: case 12: echo "x is 5 or 12"; break; default: echo "x is neither 5 nor 12"; break; } exit; ?> You could also stash the counties in different arrays and then set $to based on a series of if statements using the in_array function: http://nz.php.net/manual/en/function.in-array.php
-
Ok, I took an array approach here. I'll leave working out when all the winnings have been depleted up to you. For example, you can't have 5 users win the second tier and 3 users win the third tier - because that would total 210% of the winnings (i.e. you're bankrupt). <?php $numbers_chosen = "8|18|3,18|8|3,8|18|20,8|30|3"; $winning_numbers = "8|18|3"; // Let's get those winning numbers into an array $jackpot = explode('|', $winning_numbers); $tiers_won = array(); $tickets = explode(',', $numbers_chosen); foreach ($tickets as $ticket) { $balls = explode('|', $ticket); if ($balls == $jackpot) { // All three balls match the jackpot (in the right order too) $tiers_won[] = 100; } elseif ( ! array_diff($balls, $jackpot)) { // All the balls are the same but not necessarily in the correct order. $tiers_won[] = 30; } elseif ($balls[0] == $jackpot[0] AND $balls[1] == $jackpot[1]) { // The first two balls match those of the jackpot $tiers_won[] = 20; } elseif (count(array_diff($balls, $jackpot)) == 1) { // Only one ball is a mismatch (not considering the order) $tiers_won[] = 5; } } if ($tiers_won) { foreach ($tiers_won as $tier) { echo "User won $tier% of the winnings with one of their tickets <br>"; } } else echo "No winning ticket for this run in ANY tiers. <br>"; exit; ?>
-
You need to decide on whether you are telling the user they got 2/3 numbers correct or 2/3 numbers correct AND in the correct position. This makes quite a difference in terms of difficulty. Then keep in mind that the first and third balls might be correct, or the first and second, or the second and third so that's a lot of possibilities to account for. This is where using arrays instead of string comparisons might be easier. I can easily think of how to do either scenario with arrays but maybe one of the other forum prowlers can suggest some magic with strings if you're going down that road. But first decide how much of a match you want the check for 2/3 correct balls to be (just numbers or numbers and position)?.