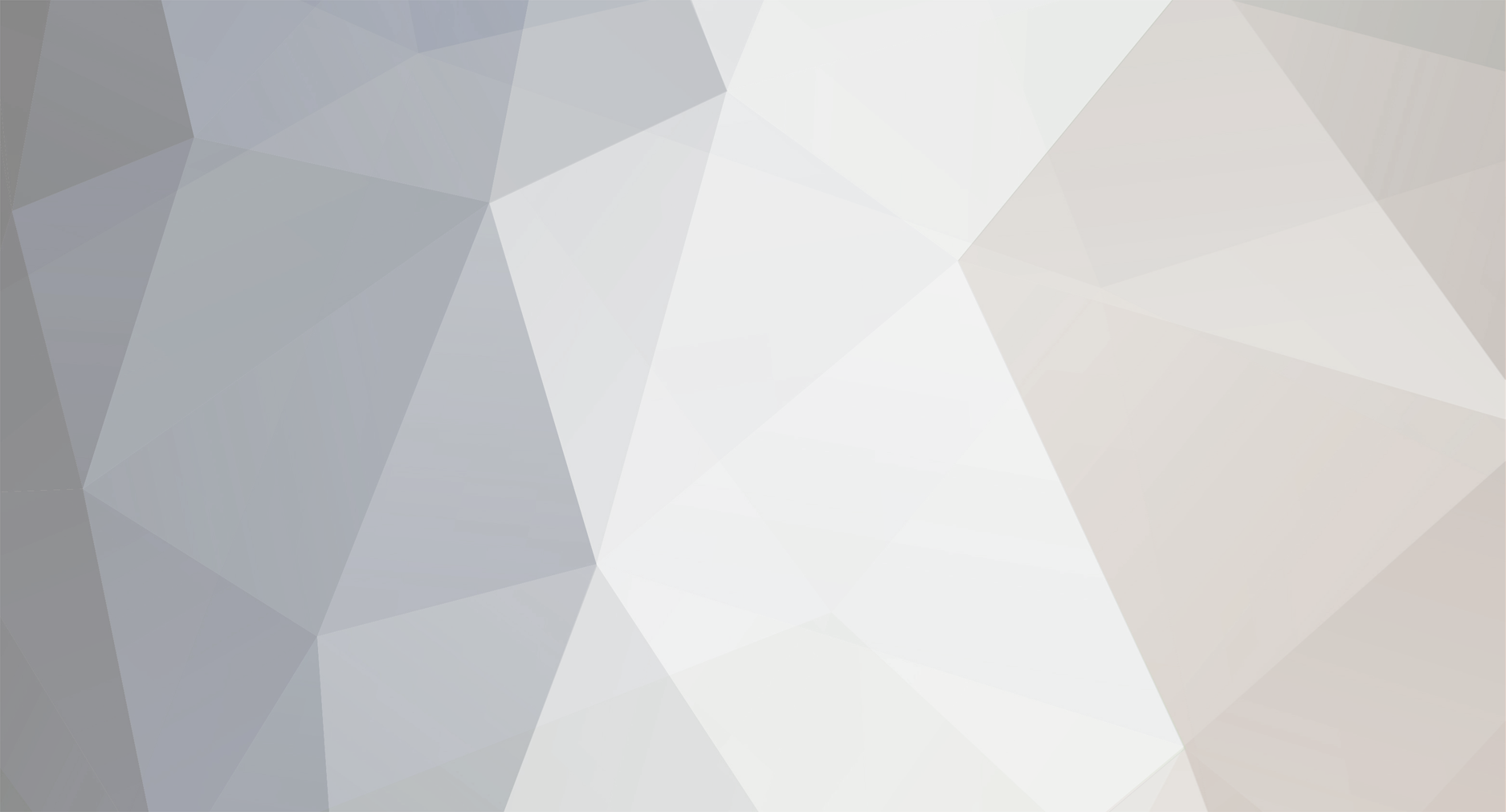
thebadbad
Members-
Posts
1,613 -
Joined
-
Last visited
Everything posted by thebadbad
-
http://random.org/ offers true random numbers (via atmospheric noise). Here's a direct link to their generator: http://www.random.org/integers/?num=1&min=1&max=100&col=1&base=10&format=plain&rnd=new (change parameters to your needs). But in most cases the PHP function mt_rand() would do the job fine I'm sure (although it's pseudorandom).
-
Mine would be even shorter (great joke potential right here) by using the ternary operator as well. And of course it can be a one line function: function sign() {$return = ''; foreach (func_get_args() as $arg) {$return .= ($arg != '') ? "<span>$arg</span>" : '';} return $return;} LOL
-
Why didn't you specify that in your original post? Would get your problem solved faster. But mikesta707's code should work. If you don't want to wrap blank vars in span tags, you can loop through each argument: <?php function sign() { $return = ''; foreach (func_get_args() as $arg) { if ($arg != '') { $return .= "<span>$arg</span>"; } } return $return; } ?>
-
As mikesta707 suggests, you can simply implode() the array returned by func_get_args() inside the function. No need for func_num_args() and the for loop. <?php function sign() { return implode('', func_get_args()); } ?>
-
Search text for links and make them to <a href>'s
thebadbad replied to seksislav's topic in Regex Help
No need to capture the match, as the full pattern match is stored in $0. And I would use \S instead of [^ ], to stop the matching at any whitespace character (most importantly a line break). And probably add the i modifier -
Something like echo date('H:i:s', strtotime('03:50 PM')); ?
-
If the database field simply contains the URL, it's just as simple as <?php //variable $url retrieved from database call echo "<a href=\"$url\">$url</a>"; ?> If you want 'inline' URLs to be converted, e.g. in a body text, you can use a fairly simple regular expression: <?php $text = 'Inline URLs like http://google.com/ found in this string will be converted to HTML links.'; echo preg_replace('~\bhttps?://\S+~i', '<a href="$0">$0</a>', $text); ?>
-
Script to catch email addresses in paragraph.
thebadbad replied to salman_ahad@yahoo.com's topic in Regex Help
Yeah, like salathe says, something simple like <?php preg_match_all('~\b[^@\s<>]+@[^@\s<>]+~', $_POST['para'], $matches); echo '<pre>' . print_r($matches[0], true) . '</pre>'; ?> should do the job fine, if you don't care about validating the emails. -
I don't see why your current code shouldn't work? <?php $word = 'I like you'; $pattern = '~\b' . preg_quote($word, '~') . '\b(?![^<]*?>)~i'; $str = 'I like you. I like your shoes.'; echo preg_match($pattern, $str) . ' match found!'; //1 match found! ?>
-
Look up preg_replace() in the manual, it takes three parameters at minimum.
-
A set of parentheses captures the match, as in stores it in the variable provided as the optional third parameter to preg_match() (often called $matches). And to your second question; yep, /pattern/D is right. If you're certain about the data (or if it doesn't matter) it's not necessary to use the D modifier, but I just tend to do it, when I want the dollar to match only at the very end of the string.
-
And just so you know, the dollar also matches immediately before the final character if it is a newline, unless you set the D modifier. So without setting it, a string like $str = "1_sign_in\n"; or $str = '1_sign_in '; //only on Linux/Unix would be matched by your pattern.
-
Or shortened: $str = preg_replace('~<((?:no)?script)\b[^>]*>.*?</\1>~is', '', $str);
-
And instead of using $r['word'] in the replacement string, you can use $0, which will be replaced by the pattern match. That way the casing of the word will always remain the same. $new_word = '<span class="flagged" onClick="alert(\'$0 needs replacing with a better one\');">$0</span>';
-
It kinda amazes me how you failed to post the contents of $r['word'] when you get the error. But aside from that, my guess is that it contains special regex characters. Either way, you should simply use preg_quote() to escape any characters with special meaning: $word = preg_quote($r['word'], '~');
-
[SOLVED] Automatically anchoring URLs that are *not* inside [code] tags
thebadbad replied to Goldeneye's topic in Regex Help
Example: <?php $strings = array( 'This is a [i]string[/i] with a link: http://foobar.com/ -- no twix for you.', '[raw]This is a [i]string[/i] with a link: http://foobar.com/ -- no twix for you[/raw]' ); //define BB code $replace = array( '~\[i\](.*?)\[/i\]~is' => '<em>$1</em>', '~\b(?:mailto:|(?:https?|ftp|nntp|news)://)\S+~i' => '<a href="$0">$0</a>' ); //define callback function for raw content function raw_convert($str, $reverse = false) { $raw = array( '[' => '&91;', ']' => '&93;', ':' => '&58;' ); if ($reverse) { return str_replace($raw, array_keys($raw), $str); } else { return str_replace(array_keys($raw), $raw, $str); } } foreach ($strings as $string) { //process raw tags $out = preg_replace_callback( '~\[raw\](.*?)\[/raw\]~is', create_function( '$matches', 'return \'[raw]\' . raw_convert($matches[1]) . \'[/raw]\';' ), $string ); //convert BB code $out = preg_replace(array_keys($replace), $replace, $out); //reconvert char substitutes in raw content $out = preg_replace_callback( '~\[raw\](.*?)\[/raw\]~s', create_function( '$matches', 'return raw_convert($matches[1], true);' ), $out ); echo "$out<br /><br />"; } ?> Only drawback I can think of, is that if any of the HTML entities from the raw_convert() function are present inside a pair of [raw] tags, they will be replaced by their character equivalent. But to overcome that you could simply use some other 'unique' tokens instead of their HTML entities. -
This should do it: <?php $html = '<b alt="swear_word">The word "swear_word" needs replacing with something less offensive.</b>'; $page = preg_replace('~\bswear_word\b(?![^<]*?>)~i', 'nice_word', $html); echo $page; //<b alt="swear_word">The word "nice_word" needs replacing with something less offensive.</b> ?>
-
Can you be more specific? What are you trying to do? I can't make much sense of it.
-
[SOLVED] convert part of a string using preg_replace
thebadbad replied to nuttycoder's topic in PHP Coding Help
Alex kinda beat me to it, though his code has an error (using double quotes for the second parameter in create_function()). You're best off using preg_replace_callback(), since using preg_replace() with the e modifier (as I guess you may have tried) gives us some escaping issues (article). Code: <?php $pr->postDesc = preg_replace_callback( '~\[code\](.*?)\[/code\]~is', create_function( '$matches', 'return \'<pre class="codeprint">\' . htmlentities($matches[1], ENT_QUOTES) . \'</pre>\';' ), $pr->postDesc ); ?> I'm assuming you're using square brackets for your code tags, since that's what you did in the pattern you said you've been using. If you want to syntax highlight the snippets, use highlight_string() instead of htmlentities(). -
That's because $admin isn't accessible inside your function (read up on variable scope). Simplest solution is to pass $admin to the function in question: function checkIt($id, $admin) //... and when you run the function further down the script; checkIt(1, $admin) Also, you really shouldn't use the short opening PHP tags.
-
That's because you're including that in your string. How it could be done, using the ternary operator: <?php $data = array( '<p><a href="logout.php">Logout</a></p> <p><a href="member.php?change=password">Change Password</a></p> <p><a href="member.php?change=email">Change Email</a></p> <p><a href="member.php?change=account">Close account</a></p>' . (($admin == 1) ? '<p><a href="member.php?change=admin">Admin Center</a></p>' : ''), '<p>Welcome to the members only page '.$_SESSION['s_name'].'!</p> <p>You are logged in as '.$_SESSION['s_username'].' from the IP address '.$_SERVER['REMOTE_ADDR'].'</p>' ); ?>
-
So what are you trying to match, and what should be replaced in the matched content?
-
I think htmlspecialchars() would do the job (converting & to &).
-
Works for me: <?php $message = 'Click the link below to fill out the request: https://words.morewords.domain.tld/word/addme?a=email@domain.tld&id=1n4Hlb4iO3Nl34g1'; if (preg_match('~\bhttps?://\S+~i', $message, $url)) { echo $url[0]; } ?>
-
It probably fails (in only grabbing the URL) because line breaks also are matched with your character class [^\" ]. There is heaps and heaps of complex URL regex patterns out there, but a really simple one could be ~\bhttps?://\S+~i It searches for a string starting with http:// or https:// (without a word character just before it) and then keeps on matching any character not a white space (space, tab, line break etc.).