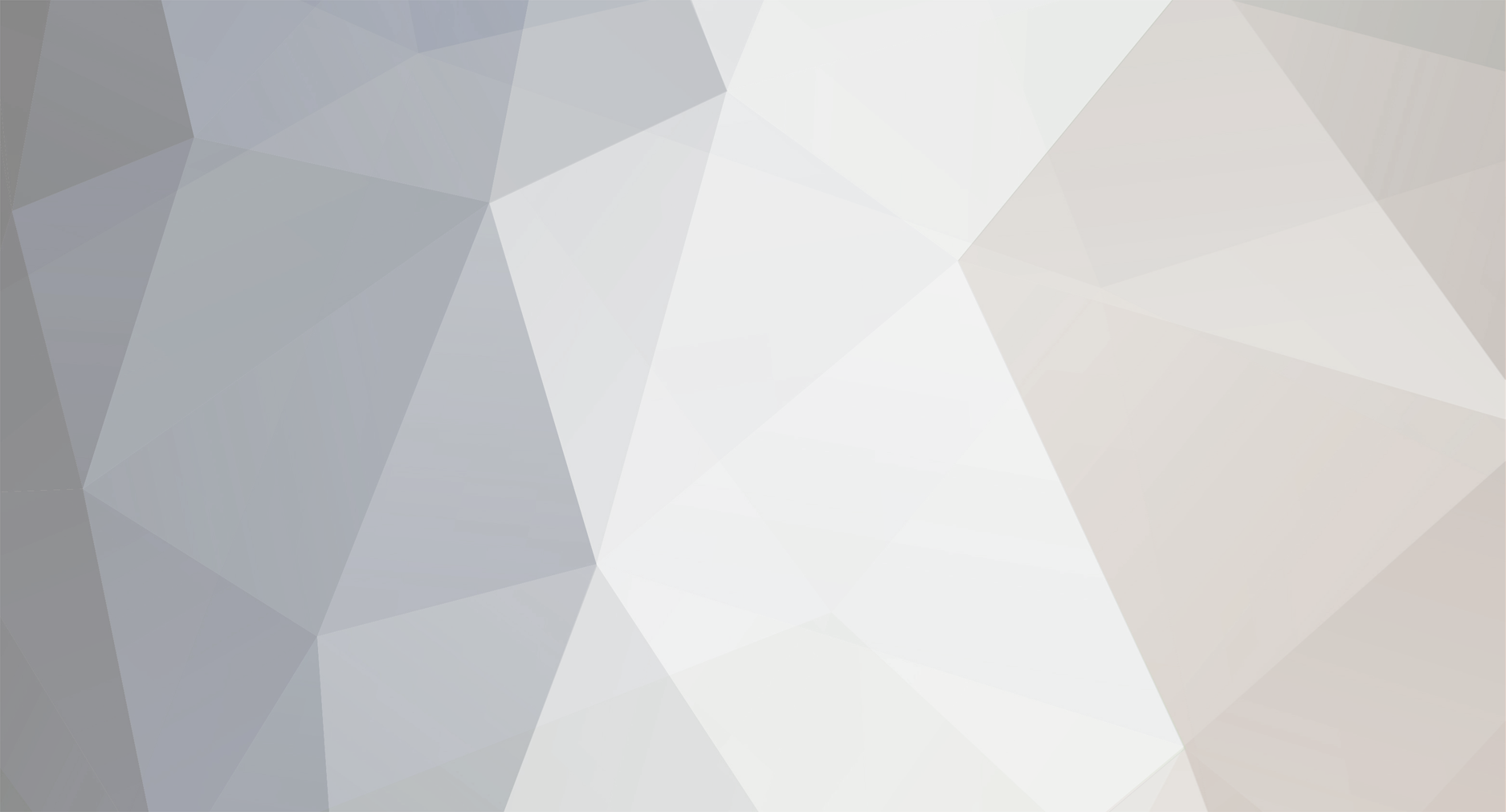
Grayda
Members-
Posts
59 -
Joined
-
Last visited
Everything posted by Grayda
-
Sounds like your script can't find the file which is causing getimageresize. It fails and imagecreatetruecolor can't work either. It goes along the chain throwing up error after error until the end. You might want to make sure that: 1) Your script has permission to access /home/p24tnugh/public_html/modelprofiles/Ann/large3.jpg 2) The file actually exists in that folder. Remember that most Linux hosts are VERY picky about case, so Ann is NOT the same as ann which is NOT the same as ANN which is not the same as AnN etc. Double check that you have spelling and capitalisation correct and just double check that your folder has permission to be written to it. Hope that helps!
-
Your best bet is to contact your host and let them know you'll be doing a lot of heavy processing, then try and break up your script so it'll work on it's task in chunks. If, for example you're collecting words from long text documents, you could find the length of the document, open only 1/4, 1/8, 1/100, 1/1000000 of the file (depending on it's length) and read that small bit and process it. Then you store the offset so when your script runs again, it'll start from the 2/4, 2/8, 2/100 or whatever you split your task into. Could there also be another place you can source your data from, already compiled for you? Either way, you're going to have to re-write your script or face having your hosting terminated for using up a shared host's resources (P.S, another thought would be to have your own Virtual Private Server -- VPS. I don't know if they have limits on time but that might be what you're after. More pricey though..)
-
when to delete old forum topics and their replies?
Grayda replied to dadamssg's topic in Application Design
MySQL is a sort of "on-demand" service that lives on a hard-drive. You do a SQL query on your database and MySQL only loads what it needs in to the memory, opens part of the file, extracts the information then closes the file and cleans up it's memory. That's why a MySQL (or indeed, almost any good SQL) server can have a billion records in it and still be as fast as lightning. Loading a billion records in to memory would kill your server really quickly Hope that makes some more sense? I've simplified the process greatly, but that's how it works in essence.. -
Downloading a file and reading contents... (USPS API)
Grayda replied to shauntain's topic in Application Design
First off, can I clarify some things? 1) What does $stream return? Is it blank? A PHP error? Something else? (just do echo var_dump($stream); and paste the results here) 2) Do you have allow_url_fopen set to true in your php.ini? Setting that option to true lets PHP fopen files from URLs (See http://au2.php.net/manual/en/filesystem.configuration.php#ini.allow-url-fopen for information on this) From the sounds of things, your browser is going to ShippingAPITest.dll and the script is returning some XML data with a HTTP header to force the XML data to be downloaded. Perhaps because the script (ShippingAPITest.dll) hasn't really done anything else with the data other than echo it out, your browser attempts to download the file using the filename ShippingAPITest.dll. I imagine this is normal, as you would only access that URL via your code meaning you don't download the file, only the contents of your file So in short, I'd look at setting allow_url_fopen and hardening your code so nobody can abuse that and also posting back the contents of $stream so we can tell if PHP is stopping this or if the other end is doing something wrong. -
I've managed to solve my problem. Turns out I needed to call $db->SetFetchMode(ADODB_FETCH_ASSOC) before connecting. I thought GetAssoc would do that for me, but it kept the FetchMode as ADODB_FETCH_DEFAULT. Hope this helps someone else out there
-
Disable Links Script Works, Just If Theres An iFrame.
Grayda replied to cursed's topic in Third Party Scripts
If the Javascript were to be run, that could cause a whole lot of security problems and possible sharing of private information And just as a quick note, if you're planning to use this on a big website, I'd think twice because people could just right click the link, click "Copy Location" and paste it in to their address bar. Just thought I'd add that in -
I'm rather confused here. I'm using ADOdb (the database abstraction library, [link url=http://adodb.sf.net]ADOdb on SF.net[/link]) to grab some data out of a table as an associative array. My code looks like this: <?php require("./adodb5/adodb.inc.php"); $db = &ADONewConnection("mysql"); $db->PConnect("localhost", "someone", "password", "myapp"); $data = $db->GetAssoc("SELECT * FROM security", false, true); # Second parameter is binding, third forces the array to be two dimensional, even if the data isn't echo var_dump($data); ?> And my table has five columns: id, name, access, group and description. ID is a unique ID, name is the name of the security object (eg. news:manageNews), access is what the object can do (eg. news:writeArticle, news:deleteArticle), group is the security group that object belongs to (eg. group:news) and description is obviously, a description (eg. Group that manages news) What I expect to see, is: array(1) { [0]=> array(5) { ["id"]=> string(1) "1" ["name"]=> string(13) "Administrator" ["access"]=> string(12) "news:canPost" ["groups"]=> string(19) "group:administrator" ["description"]=> string(20) "Administrator Object" } } But what I get back, is this: array(1) { [1]=> array(9) { ["id"]=> string(1) "1" [0]=> string(13) "Administrator" ["name"]=> string(13) "Administrator" [1]=> string(12) "news:canPost" ["access"]=> string(12) "news:canPost" [2]=> string(19) "group:administrator" ["groups"]=> string(19) "group:administrator" [3]=> string(20) "Administrator Object" ["description"]=> string(20) "Administrator Object" } [/code] Notice how there's four array items in there with the keys "0", "1", "2" and "3"? This isn't standard ADOdb (or MySQL) behaviour, as the documentation for GetAssoc shows data being returned with no numerical indexes and pasting a "GET * FROM security" into phpMyAdmin AND into the MySQL command line returns the data without the numerical indexes Is there a flag I'm missing here? Something I'm not doing with my database? Cheers!
-
Just keep in mind that encryption is a good measure against people opening up your database and extracting the passwords from the table, but doesn't help much against brute-force attacks via your site And @random1, I strongly urge you to check out recaptcha.net so you can add a CAPTCHA to your site. I was able to break this generation function in 30 minutes using a simple script. The script generates 100,000 passwords, stores them in a list, then generates another 100,000 and compares the two. If there is even a single match in the lists, the script aborts and lets you know there is a match. If I had pre-generated a list of millions of passwords, this could have been done quicker. Also, if you're going to encrypt your information before you store it, pick and use a strong salt (ie. a phrase used alongside the encryption to make it even more secure) so if people get your encrypted text, it'll be tricky to work out the password AND the salt.. Sorry if I'm pulling this into a security discussion, but it's important to know all you can before going ahead with something that involves sensitive information
-
Well, by really random, I meant, more random than English letters Here's the code I have, taken from my latest project. Does an alright job of generating passwords, but this is only one step in a secure password scheme: <?php /** * Password security. These optiona are to be used for toggling or enforcing password strength. To use effectively, you need to bitmasks. */ define("passEnforceMinimum", 1); # Should user::registerUser enforce a minimum password length? define("passRequireAlpha", 2); # Do we need to have letters in the password? define("passRequireNumbers", 4); # Do we need to have number(s) in the password? define("passRequireSymbol", ; # Do we require non alphanumeric characters in the password? define("passRequirements", passRequireAlpha | passRequireNumbers | passRequireSymbols); # Our passwords require: letters, numbers and symbols /** * generatePassword creates a random password for you * * This function takes no parameters, instead it uses the password requirements (passRequirements) to determine what the password needs. * If you have passRequireAlpha set, your password will contain letters. If you have passRequireAlpha and passRequireNumbers, your password * will contain letters and numbers, and so forth. * Minimum password length is determined by passMinimumLength (which by default, is * * @return string String containing your password */ function generatePassword() { if(passRequireAlpha & passRequirements) { # If we require letters in our password for($i = 0; $i <= passMinimumLength - 1; $i++) { # Loop passMinimumLength times to ensure a nice random mix $pass[] = chr(rand(65, 90)); # Generate random uppercase letters one at a time and put them into an array for shuffling $pass[] = chr(rand(97, 122)); # Generate random lowercase letters } } if(passRequireNumbers & passRequirements) { # Same as above, but only for numbers for($i = 0; $i <= passMinimumLength - 1; $i++) { $pass[] = chr(rand(48, 57)); # 48-57 on the ASCII table is 0-9 } } if(passRequireSymbol & passRequirements) { # Symbols! for($i = 0; $i <= passMinimumLength - 1; $i++) { $pass[] = chr(rand(33, 47)); $pass[] = chr(rand(58, 64)); $pass[] = chr(rand(91, 95)); $pass[] = chr(rand(123, 126)); } } shuffle($pass); # Finally, shuffle our array to mix it up $pass = implode("", $pass); # Glue the array together with no delimiter $pass = substr($pass, 0, passMinimumLength); # Then trim off all but passMinimumLength letters return $pass; # We're done! } ?> It's still in it's infancy and could be a LOT faster, but it works well enough. I can generate a few thousand passwords in a few seconds. Just paste it in and call $myPass = generatePassword(); to get a nice, pseudo-random password If this is what you're after, mind you!
-
Looks like this product was built using the Zend Framework (Zend Loader is a part of that, if I recall correctly) so you'll need to include the Zend Framework with your site. You can get the framework from http://framework.zend.com I've never actually used or looked at the framework, so forgive me if some of my answers are wrong, but from the sound of things, you can just extract the framework into a folder called Zend in the same directory as your script and it should start behaving a little better. Hope this helps!
-
So you're after a password generator that will output a password that LOOKS like an English word but isn't? I'd be wary of doing that, given that if you follow basic English rules, they will be easy to crack. The best method is to create a really random password that (ideally) includes letters, numbers and symbols, and is of a decent length. I've got a function prepared that will do just that and is easy to configure depending on what your password requirements are. But if you are really determined to have English-looking words, I have links to several large dictionaries that you can pluck words from and mash them up, but again, I wouldn't recommend it, given that most password cracking software could get your password in a short amount of time So, let us know exactly what you're after so we can give you a better answer
-
PHP site... mass users, single folder or individual folders?
Grayda replied to joel24's topic in Application Design
I would tend to go for the first option. Here's why: Pros: Updating two folders is easier than updating two million folders Save a ton of diskspace that can be used by your database that is build for size and speed Easy to keep track of which version a client has and easy to add "custom" versions Cons: One database. If a malicious user breaks free, ALL of your data is in their hands. Same with files And for option two: Pros: Small individual database size (which doesn't matter much considering MySQL is built to handle large databases) Security. A person breaks out, they can get to their own files, but that's it. (See Cons for database considerations) Greater customization. Each person has their own files and therefore own logo, login pages etc. (Can be a moot point if you store logo information in the database) And finally the cons: Still susceptible to database security issues (If a user breaks out, they can still access all the data if they are housed on the same machine and / or database) A million and one files to update should something go wrong If you have a million users, you'll have a million tables. If you use more than one table per configuration, it quickly adds up (My latest site has 10 tables and counting. 10x1,000,000 = 10,000,000 tables. Imagine finding a table in that, and imagine the loading times if you use something like phpMyAdmin) There are many more cons, but you get the idea. Option one is the way to go, if it's written correctly. Avoid big database calls, sanitize EVERYTHING that your users give you, and do LOTS of testing. Hopefully this'll bulletproof your application from having a "ha ha, hacked by <handle>" message appear on all your clients' sites. Enjoy! -
Just on the topic of "splash" pages, they are generally a bad idea. People know they've come to your website and don't need to be reminded again. They chew up precious time when people are picking at your site. They come to get some information or have a quick read, and don't want to sit around for 10-20 seconds waiting for something to load. If it's not informational, they don't want it (generally XD) Unless your site warns that content may not be suitable for underage people, or requires some kind of terms and conditions to be met or offers people a choice between flash and HTML (which again begs the question of relevance) then it's best not to have a splash page at all. Overall your site is coming along. I agree with PugJr in getting rid of the counter. It seems like bragging a little, even if that's not your intention. If it's there to let people know how popular your site / services are, then you're marketing it all wrong because measure of a good business / site is based on word of mouth and clients, not some easily adjustable numbers at the bottom of the page The flash containing images on the main page is alright, but the spinning is distracting. Perhaps some subtle fading in and out would look better. You might even be able to do this in Javascript using Mootools or something. Well worth looking in to because most browsers have Javascript and not everyone has Flash. Plus if written well, the images would degrade nicely in browsers that don't have Javascript either. The horizontal line between your header and content is rather distracting too. A simple <hr noshade='noshade' /> would work just as well and not break or look strange if you have an odd resolution or widescreen / tall monitor. I'd also remove the two basketball player silhouettes from the header. They're not a part of your logo and don't really contribute anything. Other than that, you're off to a great start and with a few more refinements, will be a great site. And sorry if I sounded too harsh, they say criticism is the best way to improve
-
Completely New Design, please say what you think.
Grayda replied to npsari's topic in Website Critique
I agree with arg_alpha about the scrolling text. It's very distracting. Plus it goes way too fast and doesn't stop when you mouse over, meaning it's almost blind luck which page you'll end up on. Perhaps you should look at making the scroller work vertically. In other words, scroll one link up at a time, pause for 5-10 seconds, then scroll again. This is less distracting and while it is still a little cheesy, it's far better than a fast flowing stream of information I'm also still seeing squashed thumbnails (I don't know if you've fixed that or not, just mentioning). Perhaps you need to enforce some kind of size requirements for images or not make the profile box so big. Asides from that, it's a nice looking site. The colours are simple and the blue liquid-y effect adds some playfulness. Keep it up -
[SOLVED] Ready made dictionary for password checking
Grayda replied to Grayda's topic in Application Design
Thanks for posting this. It has more common words than the other big list from SCOWL so I'll include both and developers can decide what one to use depending on their site. And I don't think it's creepy. Mirroring his entire site purely for your own.. er.. enjoyment, then that would be creepy haha -
[SOLVED] Ready made dictionary for password checking
Grayda replied to Grayda's topic in Application Design
I know how you feel. My hosting bill got sent to the wrong address and as a result I almost lost all of my and my clients' websites. Payday was a week away too, so I was under a lot of stress For the record, I've downloaded SCOWL from this site and am currently writing up a function so different dictionary sizes can be "dropped" in. The word list is free for redistribution, sale etc. as long as the copyright notice is displayed somewhere within the website or in the source Now, to get testing! Thanks for your help Daniel and good luck getting your stuff back.. -
What are these files actually used for? Are they for a gallery? Are they a part of the layout? Are they images in the blog post? In either way, you could probably store all of these files in the one file and systematically get them. For example (and this is based off the assumption that these 50 files are for a gallery): <?php $file[0]["src"] = "File1.jpg"; $file[0]["blurb"] = "Description for File one"; $file[1]["src"] = "File2.jpg"; $file[1]["blurb"] = "Description for File two"; // .... $file[49]["src"] = "File50.jpg"; $file[49]["blurb"] = "Description for File fifty"; for($i = 0; $i <= $numOfFiles; $i++) { echo "<img src='" . $file[$i]["src"] . "'>" . $file[$i]["blurb"] . "<br />"; } ?> But that's a horrible way of doing it. My suggestion would be create a table on your database called 'tblGallery' or something, store the image locations in there then use a simple PHP function to get the src and blurb. That way, you can programatically maintain the list meaning you can have fifty, one hundred or even a million image locations in your database But until you can tell us more about your application, this is the best I can offer
-
[SOLVED] Ready made dictionary for password checking
Grayda replied to Grayda's topic in Application Design
Thanks for the link Daniel. However, it's broken: "Not Found. The requested URL /dict-en.txt.bz2 was not found on this server." I'll take a look at using ASpell in the meantime. cheers! -
I was toying around with some of the $_SERVER[] variables in PHP earlier and wondered, is it possible to spoof $_SERVER["REMOTE_USER"]? Can Joe Hacker fool PHP / IIS / Whatever into thinking that they're Bob Admin, or would this require a way of emulating Windows security? Is this superglobal property unique to IIS or is it possible via another server based security method? No purpose, just a curious question..
-
There are three ways to achieve what you're after: Number one (anywhere in your script, preferably at the bottom so the page has time to load): <script language='javascript'>setTimeout('window.location="gohere.html"', 5000);</script> Pros: Quick and easy Doesn't break W3C standards Works well with back buttons Cons: Doesn't work in Javascript is turned off Number two (put this between your header tags): <meta http-equiv="refresh" content="5;url=gohere.html" /> Pros: Doesn't require Javascript Cons: Can prevent people from using the back button if redirected too quickly Discouraged by the W3C. Instead they encourage you to use 30x status code Number three (place BEFORE your PHP script outputs anything, since we're messing with headers): <?php Header( "HTTP/1.1 303 See Other" ); Header( "Location: gohere.html" ); ?> Pros: All browsers can understand this Encouraged by the W3C Cons: Should only be used for updating URLs. (eg. redirect from newsite.example.com to example.com when newsite goes live) Requires you use output buffering unless no content comes before the header change In essence, pick your poison. All three work well depending on your situation
-
Something to look at, is RESTful applications. Basically, this is simple HTTP verbs (GET, PUT, DELETE etc.) used with PHP so people can send information to http://www.example.com/api/calendar/ and have it inserted into the calendar or access http://www.example.com/api/calendar/2009/23/05 to access information about the 23rd of May 2009 Here's a sample of code. It's a lot more involved than this, but it's a start. <?php // Are we working with a PUT request? if($_SERVER['REQUEST_METHOD'] == 'PUT') { // Yes? Then parse the string and put the results into $results. php://input is a special "file" that contains the PUT information. You can use this with DELETE etc. parse_str(file_get_contents("php://input"), $results); // Use your own "login" function here to make sure people are logged in BEFORE sending calendar information $isLoggedIn = userLogin($results["username"], $results["password"]); if(!$isLoggedIn) { // If we failed to log in, return a 401 status code so the browser knows we aren't authorized to do this action header('HTTP/1.1 401 Unauthorized'); // Then exit, because we don't want to process anything else exit(); } // Add the calendar information $success = addCalendarInformation($results["calendarTitle"], $results["calendarDate"], $results["calendarDescription"]); // If all went well, if($success) { // Let them know with a "Created" HTTP status code header("HTTP/1.1 201 Created"); } else { // They forgot to put some information in, because $success is false header("HTTP/1.1 412 Precondition Failed"); } } ?> I hope this is a start. Check out these links for more information and code snippets: http://www.recessframework.org/page/towards-restful-php-5-basic-tips http://www.lornajane.net/posts/2008/Accessing-Incoming-PUT-Data-from-PHP http://www.gen-x-design.com/archives/create-a-rest-api-with-php/ (WARNING: Lots of code in classes, great for copy-pasta into your code ) Enjoy
-
As a part of my new framework I'm writing, I'd like to have a dictionary of common words for checking passwords against. If the password equals or is close to this common word, reject the password. I've got the code written up, I'm just after a ready made dictionary that I can freely use and redistribute. As an array would be preferable, but whatever format would work. If worse comes to worst, I can make one myself, but if I can get my hands on something, that'd be fantastic. Anyone have any suggestions?
-
Thanks for the information! I tried a sample implementation of your code and found that it was good for a fixed number of security levels and pages, but my application needed a little more dynamic-ness to handle custom pages and custom security levels. So I spent the last 3 hours poking around and came up with this which seems to work rather well so far: <?php // .. Some class code and variables here .. // Function checkPermission // $user = the username to check // $uAccess = the section the user is trying to access function checkPermission($user, $uAccess) { // This function fills up three variables: $this->access, $this->groups and $this->edit. // The data is pulled from the database and is split up using explode() $this->getPermissions($user); // First we loop through the groups we've got from the database for($i = 0; $i <= count($this->groups); $i++) { // I'm using ADOdb and some custom functions here. // This gets all the information related to the group in $this->groups[$i] (eg. AdminGroup or BasePages) $data = $this->database->getSQL("SELECT * FROM security WHERE Name = '" . $this->groups[$i] . "'"); // Now, we explode the information. First the groups related to $this->groups[$i] then access information then edit information $group = explode(",", @$data[0]["Group"]); $access = explode(",", @$data[0]["Access"]); $edit = explode(",", @$data[0]["Edit"]); // Now comes the magic. Looping! for($b = 0; $b <= count($group); $b++) { // If the groups aren't empty then: if(!empty($group[0])) { // Merge the results of $group and $this->groups $groupTemp = array_merge($this->groups, $group); // Then return only the unique groups. // When the loop goes back around, it will re-calculate count($this->groups) and keep going until we stop adding stuff to the array $this->groups = array_unique($groupTemp); } else { // But if the groups are empty, then we've come to the end of the line, so we // merge all the information we've got so far into our class variables obtained above $accessTemp = array_merge($this->access, $access); $this->access = array_unique($accessTemp); $editTemp = array_merge($this->edit, $edit); $this->edit = array_unique($accessTemp); } } // Same goes for the access for($b = 0; $b <= count($access); $b++) { if(!empty($access[0])) { $accessTemp = array_merge($this->access, $access); $this->access = array_unique($accessTemp); } } // And for edit for($b = 0; $b <= count($edit); $b++) { if(!empty($edit[0])) { $editTemp = array_merge($this->edit, $edit); $this->edit = array_unique($editTemp); } } } // Finally, once we have all the access, group and edit information we need, we check to see if $uAccess is in the arrays and if it is, then we return true, meaning they have access. PHEW! if(in_array($uAccess, $this->access) or in_array($uAccess, $this->edit) or in_array($uAccess, $this->groups)) { return true; } else { return false; } } ?> I tried it on some large group sets. (eg. guest -> blah1 -> blah2 ->blah3 ->blah4 -> blah5 -> main) and when I monitored the SQL calls through ADOdb, I got a series of calls to the right groups and at the end, it returned the data I was after. However, there might be some code optimizations I could make, and I should really test it with TONS of security objects. But for anyone out there looking for a way to do this kind of thing, this might be for you?
-
I'm building in some new security modules for my web application and need some help regarding a simple waterfall or path-building solution In my application, security is handled through a simple table like this: Username Access Group Edit Grayda somepage AdminGroup someotherpage guest [/td] BasePages BasePages main,header,footer AdminGroup main,header,footer [td]main,header,footer In this example, Grayda can access somepage and edit someotherpage and is also in the group "AdminGroup". Guest is a part of the group "BasePages". If you follow the table down, you will see the AdminGroup can access "main", "header" and "footer" and can also edit "main", "header" and "footer". "BasePages" is the same as "AdminGroup" but without the edit permissions. What I'm trying to build, is a function that will let me build a path from point A to B. For example if Grayda wants to access Main, and the path to Main is: Grayda -> AdminGroup -> BaseGroup -> CoreGroup -> Main then calling checkPermission("Grayda", "Main"); will return true because Grayda is in AdminGroup which points to BaseGroup etc. I've got simple one-level groups going on (eg. Grayda can access Main if it's only one level deep) but anything deeper eludes my knowledge. Can anyone give me some assistance or some ideas on where to start with this?
-
It's been out to the public for a while (as a beta), but I'm still doing security tests with it. Before I move my main website to this engine, can someone give it a thorough testing? It's a dead-easy to use CMS. You install it, log in and your page reloads with some "Edit <page>" links next to each section of content. Click that link, type your new content in to the WYSIWYG editor and click Save. The page reloads and your website is updated. Also uses phpGACL for security. This is my first major PHP Project released (despite using PHP for 3-4 years now) and I want to make sure it's good. You can try out the live version at: http://demo.spage.solidinc.org and download the latest build (beta 2) from http://spage.solidinc.org/index.php?page=downloads&latest=1. Beta 2 comes with an installer so it should be rather easy to install. I'd like you to fiddle with everything, see if unauthenticated users can get anywhere they're not supposed to, log in as moderators and try and escalate privileges, stuff like that. Obviously no stuff that will break the demo site (the demo site resets on the hour anyway). And if you have time, some comments on what you think of it's ease of use and stuff, but PM or email me with those because it's off-topic