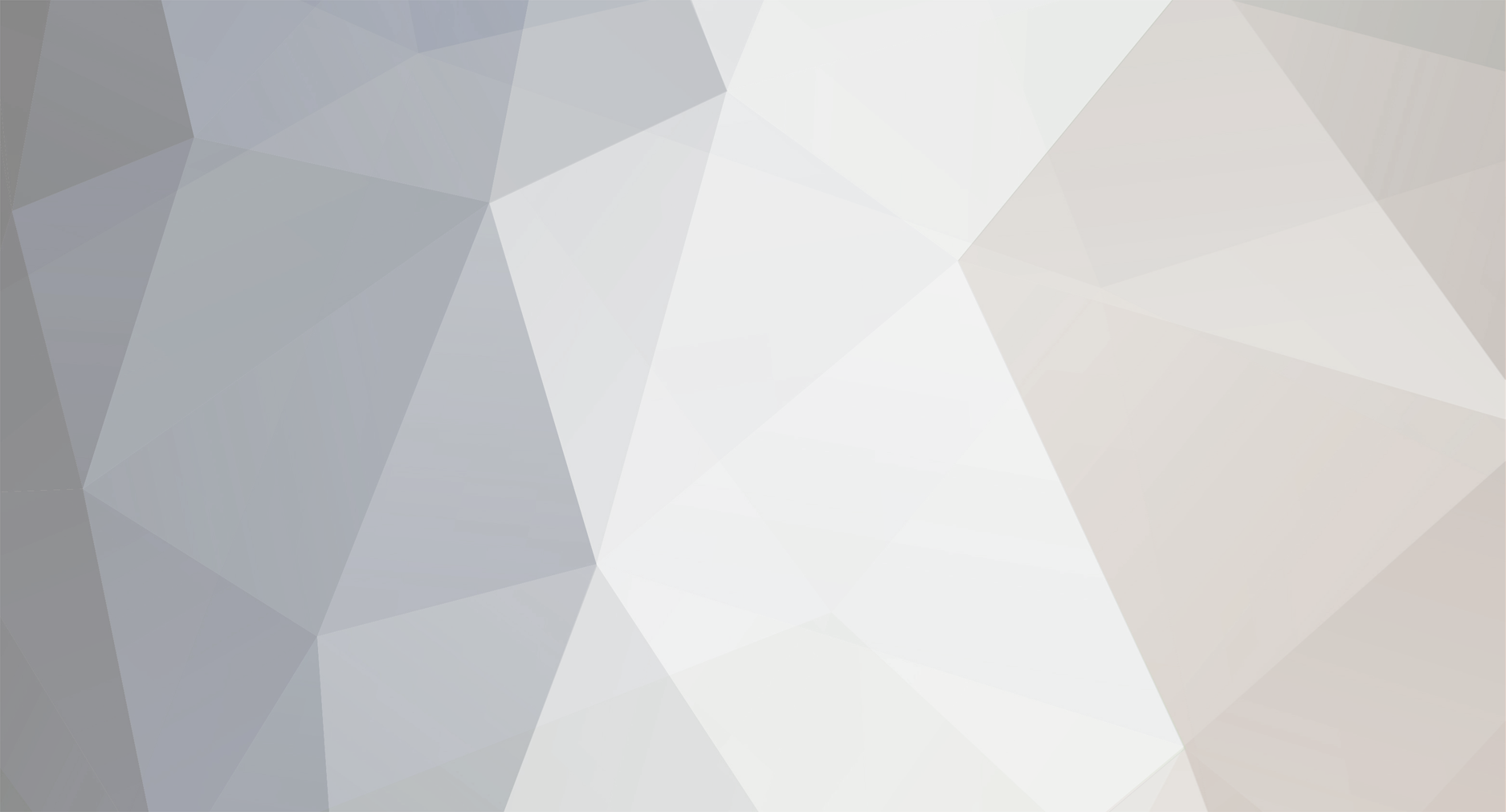
dreamwest
-
Posts
1,223 -
Joined
-
Last visited
Never
Posts posted by dreamwest
-
-
Heres another way:
say you have a normal link to download files:
http://site.com/get.php?id=876
function password_gen($min=6,$max=6,$id){ /*has a default min value of 6 and maximum value of 6*/ $pwd=""; // to store generated password for($i=0;$i<rand($min,$max);$i++){ $num=rand(48,122); if(($num >= 97 && $num <= 122)) $pwd.=chr($num); else if(($num >= 65 && $num <= 90)) $pwd.=chr($num); else if(($num >=48 && $num <= 57)) $pwd.=chr($num); else $i--; } return "encrypt->".strtoupper($pwd).mt_rand()."l".$id."l".strtoupper($pwd).time(); } $file_id = password_gen(6,6,$row['id']);
As you can see the link will be something like this:
http://site.com/get.php?=encrypt->3EA4VTRM1875445687l876lA4VTRM1252451005
You can see in the center l876l so in get.php simply explode the "l" to get the id which is 876, because the link is always changing its almost imposible for ppl to run grabbers on your site
Now someone could write a script to simply explode the "l" anyways, so pull the "salt" from a database, update the salt whenver you like
-
They are directories:
/_inc/js/$1 [L]
Fake - left hand side
Real - right hand side
If the directory is real put htaccess in this directory
/_inc/js/.htaccess
-
RewriteRule ^ willmott index.php?option=com_content&view=article&id=130:paul-a-vanessa-willmott&catid=36:full-time-evangelists&Itemid=56&authid=91 [L]
-
<script> function toggleSections(section) { document.getElementById(section).style.display = "block"; document.remember_page.my_value.value = section; document.remember_page.submit(); return false; } function onload_remember_page() { // always store the current page on page load document.remember_page.my_value.value = "details"; document.remember_page.submit(); } </script> <a href="#details" onclick="toggleSections('details_57');return false; " >show</a> <div id="details_57" style="display: none" >hidden stuff here</div>
-
By the way which is faster, regex or php dom??
-
You can grab the URLs with regex:
<?php $str = "html here<a class='link' href='http://www.site.com/?q=6J4W2G4fASI'>html here html here<a class='link' href='http://www.site.com/?q=637yfuhqelhe'>html here html here<a class='link' href='http://www.site.com/?q=hilghye8oyhi'>html here"; preg_match_all('~<a\b[^>]+href\s?=\s?[\'"](.*?)[\'"]~is', $str, $matches); echo '<pre>' . print_r($matches[1], true) . '</pre>'; ?>
Note that $matches[1] will be an array of the URLs.
Sweet thanks!
I knew preg_match_all would do it but couldnt get my head around the regex
-
-
Im trying to break apart a group of hyperlinks so im just left with the actual url:
html here<a class='link' href='http://www.site.com/?q=6J4W2G4fASI'>html here
html here<a class='link' href='http://www.site.com/?q=637yfuhqelhe'>html here
html here<a class='link' href='http://www.site.com/?q=hilghye8oyhi'>html here
etc...
so the result would be :
http://www.site.com/?q=6J4W2G4fASI
http://www.site.com/?q=637yfuhqelhe
http://www.site.com/?q=hilghye8oyhi
-
Im trying to seperate a group of hyperlinks, but cant seem to do it
<a target="_self" href="http://site.com/index.php?q=search">search</a>
<a target="_self" href="http://site.com/index.php?q=search2">search2</a>
<a target="_self" href="http://site.com/index.php?q=search3">search3</a>
Basically i want to strip everything away except what between the hyperlink tags so it looks like this:
search
search2
search3
-
take a look at the file .htaccess
find line :
browse.php/id=(.*) browse.php?id=$1
Theres nothing wrong with it , it all does the same thing
-
<?php $textarea=$_POST['textarea1']; if($textarea == ""){ echo "wrong"; }else{ echo "true"; } ?>
-
-
You need to select distinct * when selecting multiple fields, the order by name
-
select table > select edit pencil on login id field > extra dropdown , select auto_increment
-
Ok went to youtube with javascript off, tried to watch a vid and:
"Hello, you either have JavaScript turned off or an old version of Adobe's Flash Player. Get the latest Flash player."
Also comments and ratings dont work, related images dont display thumbs, thumbchange doesn work in video section the list goes on.
Verdict: If they dont care neither do i
-
Just of curiosity, does your site function with JS turned off?
Never thought about it....its their fault for not having it on anyway.
WHAT IF they dont anyway, whats the alternative??
Google indexing doesn't parse JS either. So basically go to your site with JS disabled, see how well it works, then think of Google indexing it like that....
Yeah tried it just now most of the site is ok, but features like rating, quicklist and onclick dropdowns etc dont do anything.
BUT when i came back here with javascript off the "insert quote" link didnt work so every site is most likely designed with javascript on by default.
I also checked my logs and less...thats LESS than 1% have javascript turned off.
Im going to add a message in the header to tell ppl they need it on to use the fuctionality of the site
-
http://en.wikipedia.org/wiki/Dedicated_hosting_service - All hail Wikipedia ! - oh i forgot to add wiki as one of my best apps from my other post http://www.phpfreaks.com/forums/index.php/topic,266164.0.html
-
Just of curiosity, does your site function with JS turned off?
Never thought about it....its their fault for not having it on anyway.
WHAT IF they dont anyway, whats the alternative??
-
Yeah it is a buffer with a loop, heres the finished script:
Check to make sure proper amount of deliminators "|" in a file
$handle = @fopen("1.txt", "r"); if ($handle) { $count = 1; while (!feof($handle)) { $buffer = trim(fgets($handle, 10096)); $check = explode('|', $buffer); $check = trim($check[1]); if ($check == "" || $check == " " ){ //delete line $err .= "ERROR on line {$count} - {$buffer}<br>"; }else{ //write the rest to a new file $current .= $buffer."\n"; } ++$count; } fclose($handle); } $current = rtrim($current); file_put_contents("2.txt", $current);
-
<IfModule mod_security.c> SecFilterEngine Off SecFilterScanPOST Off </IfModule> # explicitly disable caching for scripts and other dynamic files <FilesMatch "\.(pl|php|cgi|spl|scgi|fcgi)$"> Header unset Cache-Control </FilesMatch> # stop ppl from browsing indexes Options -Indexes # disable the server signature ServerSignature Off # prevent folder listing IndexIgnore *
-
You try to avoid Javascript, but use AJAX for everything else? You do realize that AJAX is a Javascript technique, right?
Well my ajax request has about 7 lines of javascript and is 1kb in size, and for what it can do thats impressive - pretty much run the entire sites functionality. Also the fact it can query pages without initially including them is great..I LOV IT!
You want to see javascript overdone get coppermine.....now only 10MB in size .....for what picture galleries and a slideshow
-
I went from having 10+ second loading times to < 1 second +- 0.5 seconds - thats what gets me excited
after all that your on about speed lol.
Im obsessed with speed! - Crazy obsessed
-
I try to avoid javascript too. I have LESS than 5kb of javascript combined on my entire site (one of the reasons it loads so fast)
I use ajax http request for everything else, its non intrusive and fun to play with and add lots of value to your apps
-
Once your done you could use rtrim to remove the last newline before writing to the file.
Just what i was looking for. Thanks!
Secure Database Connection
in PHP Coding Help
Posted
True, anything thats included can be outside browser viewing areas, you never need to view it directly anyways