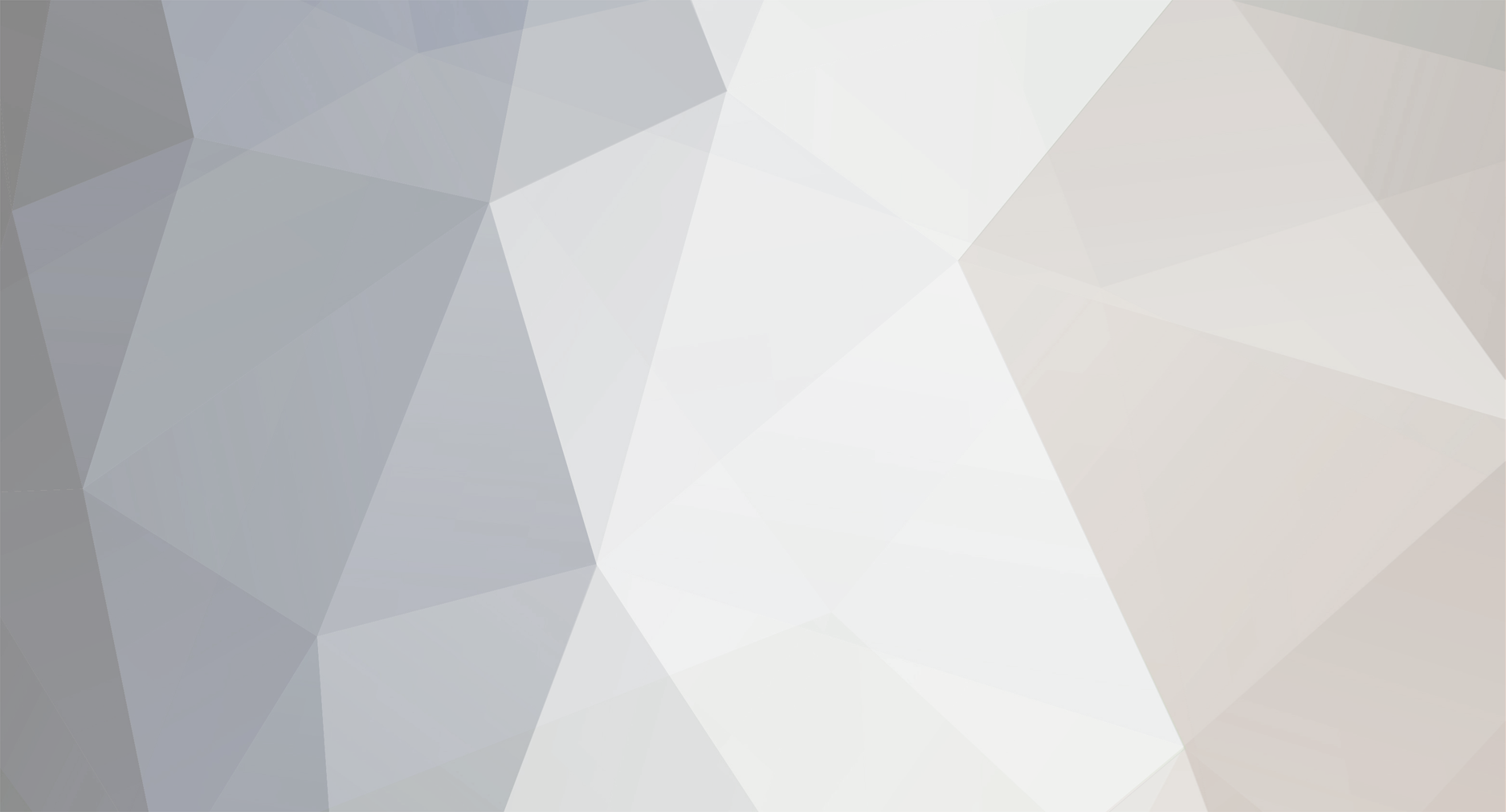
dreamwest
-
Posts
1,223 -
Joined
-
Last visited
Never
Posts posted by dreamwest
-
-
Simplest join possible
$result = mysql_query("SELECT * FROM term_taxonomy AS a, terms as b WHERE a.term_id=b.term_id");
-
Write to database instead of file.
Ive tried experiments on speed with ifelse and functions - ifelse wins so you cant do much there.
If your using this to display content use flush(); to output the outlay as fast as possible
-
You could have spaces in you keyword column _ppi
Try:
SELECT * FROM `keywords` WHERE `keyword` LIKE 'ppi'
-
Another way to do it to query the database and write the whole thing to a file
/*************** config ***************/ $mysql_host=''; $mysql_database='' database name $mysql_username=''; // user name $mysql_password=''; // password $storing_dir = ""; // directory path with an ending slash /******************* end config ************/ $file_name = $mysql_database."_".date('YmdHis').".sql"; $link = mysql_connect($mysql_host, $mysql_username, $mysql_password); if (!$link) { fwrite($fh, 'Could not connect: ' . mysql_error()); exit(); } $db_selected = mysql_select_db($mysql_database, $link); if (!$db_selected) { fwrite($fh, 'Can\'t use $mysql_database : ' . mysql_error()); exit(); } $myFile = $storing_dir . $file_name; $fh = fopen($myFile, 'w') or die("can't open file"); _mysqldump($mysql_database); fclose($fh); function _mysqldump($mysql_database) { global $fh; _mysqldump_schema_structure($mysql_database); $sql="show tables;"; $result= mysql_query($sql); if( $result) { while( $row= mysql_fetch_row($result)) { _mysqldump_table_structure($row[0]); _mysqldump_table_data($row[0]); } } else { $content = "/* no tables in $mysql_database */\n"; fwrite($fh, $content); } mysql_free_result($result); } function _mysqldump_schema_structure($schema) { global $fh; fwrite($fh, "/* database : `$schema` */\n"); $sql="show create schema `$schema`; "; $result=mysql_query($sql); if( $result) { if($row= mysql_fetch_assoc($result)) { fwrite($fh, $row['Create Database'].";\n\n"); } } fwrite($fh, "USE `$schema`; \n\n"); mysql_free_result($result); } function _mysqldump_table_structure($table) { global $fh; fwrite($fh, "/* Table structure for table `$table` */\n"); fwrite($fh, "DROP TABLE IF EXISTS `$table`;\n\n"); $sql="show create table `$table`; "; $result=mysql_query($sql); if( $result) { if($row= mysql_fetch_assoc($result)) { fwrite($fh, $row['Create Table'].";\n\n"); } } mysql_free_result($result); } function _mysqldump_table_data($table) { global $fh; $sql="select * from `$table`;"; $result=mysql_query($sql); if( $result) { $num_rows= mysql_num_rows($result); $num_fields= mysql_num_fields($result); if( $num_rows > 0) { fwrite($fh, "/* dumping data for table `$table` */\n"); $field_type=array(); $i=0; while( $i < $num_fields) { $meta= mysql_fetch_field($result, $i); array_push($field_type, $meta->type); $i++; } //print_r( $field_type); fwrite($fh, "insert into `$table` values\n"); $index=0; while( $row= mysql_fetch_row($result)) { fwrite($fh, "("); for( $i=0; $i < $num_fields; $i++) { if( is_null( $row[$i])) fwrite($fh, "null"); else { switch( $field_type[$i]) { case 'int': fwrite($fh, $row[$i]); break; case 'string': case 'blob' : default: fwrite($fh, "'".mysql_real_escape_string($row[$i])."'"); } } if( $i < $num_fields-1) fwrite($fh, ","); } fwrite($fh, ")"); if( $index < $num_rows-1) fwrite($fh, ","); else fwrite($fh, ";"); fwrite($fh, "\n"); $index++; } } } mysql_free_result($result); fwrite($fh, "\n"); }
-
You need to $_GET the page and use startfrom in your query
http://site.com/index.php?q=searching&page=3
This is an example
//get the total for the search here $total = //query here $tpage = ceil( $total / 30 ); $startfrom = ( $page - 1 ) * 30; $sql = "SELECT * FROM table where something ='query' LIMIT {$startfrom}, 30"; $result = mysql_query( $sql ); //now page stuff $content_count = mysql_num_rows( $result ); $start_num = $startfrom + 1; $end_num = $startfrom + $content_count; $prev = $page - 1; $next = $page + 1; $adjust = 9; if ( 1 < $page ) { $page_links .= "<a class=pagination_prev href='".$prev."'><< Prev</a><a class=pagination_next href='1'>1</a>...."; } $k = $page - $adjust; for ( $page ; $k <= $page + $adjust; ++$k ) { if (( 0 < $k ) && ( $k <= $tpage ) ) { if(($page)==($k)){ $page_links.="<span class=\"pagination_active\"> ".$k." </span>"; } else { $page_links .= "<a class=pagination href='".$k."'>{$k}</a>"; } } } if ( $page < $tpage ) { $page_links .= ".....<a class=pagination_next href='".$tpage."'>".$tpage."</a><a class=pagination_next href='".$next."'>Next >></a>"; }
-
Really!? What about this...
RewriteRule ^([^/\.]+)/(.*)/(.*) index.php?section=$2&action=$3&root=$1 [L,QSA]
I win
-
Your over complicating it. You just need the total and page reference
And dont forget ceil
Example:
$page = 1; $tpage = ceil( $total_pics / 30 ); $startfrom = ( $page - 1 ) * 30; $start_num = $startfrom + 1; $end_num = $startfrom + $total_pics; $prev = $page - 1; $next = $page + 1; $adjust = 9; if ( 1 < $page ) { $page_links_pics .= "<a class=pagination_prev href='/hdpics/".$prev."'><< Prev</a><a class=pagination_next href='/hdpics/1'>1</a>...."; } $k = $page - $adjust; for ( $page ; $k <= $page + $adjust; ++$k ) { if (( 0 < $k ) && ( $k <= $tpage ) ) { if(($page)==($k)){ $page_links_pics.="<span class=\"pagination_active\"> ".$k." </span>"; } else { $page_links_pics .= "<a class=pagination href='/hdpics/".$k."'>{$k}</a>"; } } } if ( $page < $tpage ) { $page_links_pics .= ".....<a class=pagination_next href='/hdpics/".$tpage."'>".$tpage."</a><a class=pagination_next href='/hdpics/".$next."'>Next >></a>"; }
-
RewriteRule ^index/login/members index.php?section=members&action=login [L,QSA]
-
$stuff = '<!-- open tag --> <a href="http://www.phpfreaks.com>PHP Freaks</a> <!-- / open tag -->'; $no_stuff = explode( "<a href="http://www.phpfreaks.com>PHP Freaks</a>", $stuff); echo $no_stuff[0] . $no_stuff[2];
-
All hail Bing!
-
function getWidth() { return imagesx($this->image); } function getHeight() { return imagesy($this->image); }
-
Apparently its the same thing:
href="http://www.mysite.com/?d=9FDS32S" target="_blank">http://www.mysite.com/?d=9FDS32S</a>
in this case yes ... in all cases ... maybe not.
that is why people are trying to give him a solution that works in ALL cases
just explode the href=" part to get the url
Now say after me
$message = 'Regex is the best ! All hail explode'; $value=explode("!", $message); $value = trim($value[1]); echo $value;
-
Apparently its the same thing:
href="http://www.mysite.com/?d=9FDS32S" target="_blank">http://www.mysite.com/?d=9FDS32S</a>
-
That way it's not very dynamic. What if you use like this <a href="something.php">DOWNLOAD</a> , then it fails.
<a href="something.php">DOWNLOAD</a> will become DOWNLOAD
See my second post
-
Thanks! Ive been trying to get that one for a while now
-
Of course it does....and this assumes this is a link
$message = '<a rel="nofollow" href="http://www.mysite.com/?d=9FDS32S" target="_blank">http://www.mysite.com/?d=9FDS32S</a>'; $value=explode("</a>", $message); $value=explode(">", $value[0]); $value = trim($value[1]); echo $value;
-
$message = '<a rel="nofollow" href="http://www.mysite.com/?d=9FDS32S" target="_blank">http://www.mysite.com/?d=9FDS32S</a>'; $value=explode('target="_blank">', $message); $value=explode("</a>", $value[1]); $value = trim($value[0]); echo $value;
-
Im transfering files using curl but get a timeout error:
Fatal error: Allowed memory size of 85983232 bytes exhausted (tried to allocate 50681183 bytes) in /home/user/public_html/vids.php on line 71
I have a timeout just before the curl but it doesnt seem to fix it:
set_time_limit(0);
-
Make css and images ...everything thats included, a full url http://site.com/style.css
-
I have a really long document, but only need a single word/s within two specific tags
words here.....
<link>only get this line</link>
lots of words here.....
How can i just get what is within the <link> tags??
-
im assuming this url is not real, but it has to exist http://www.health-issues.net/$1/ja. It has to be something like http://www.health-issues.net/ja/index.php?language=$1
What is the real address??
-
# permanently redirect from www domain to non-www domain RewriteEngine on Options +FollowSymLinks RewriteCond %{HTTP_HOST} ^www\.domain\.tld$ [NC] RewriteRule ^(.*)$ http://domain.tld/$1 [R=301,L]
OR
# redirect from old domain to new domain RewriteEngine On RewriteRule ^(.*)$ http://www.new-domain.com/$1 [R=301,L]
OR
# redirect an entire site via permanent redirect Redirect permanent / http://www.domain.com/
-
just remove the /ja from the fake url
-
site.com/news/ does not work
I deliberately left this out so you can test the dynamic rule
Now you just need the default ive changed it so it wont conflict with any other rule.
#default for all sections -> http://site.com/type=news or http://site.com/type=forum
RewriteRule ^type=(.*) index.php?type=$1 [L,QSA]
Fatal error: Call to a member function num_rows() on a non-object in /home/a8164
in PHP Coding Help
Posted
your simply calling a function that doesnt exist - num_rows() on a non-object