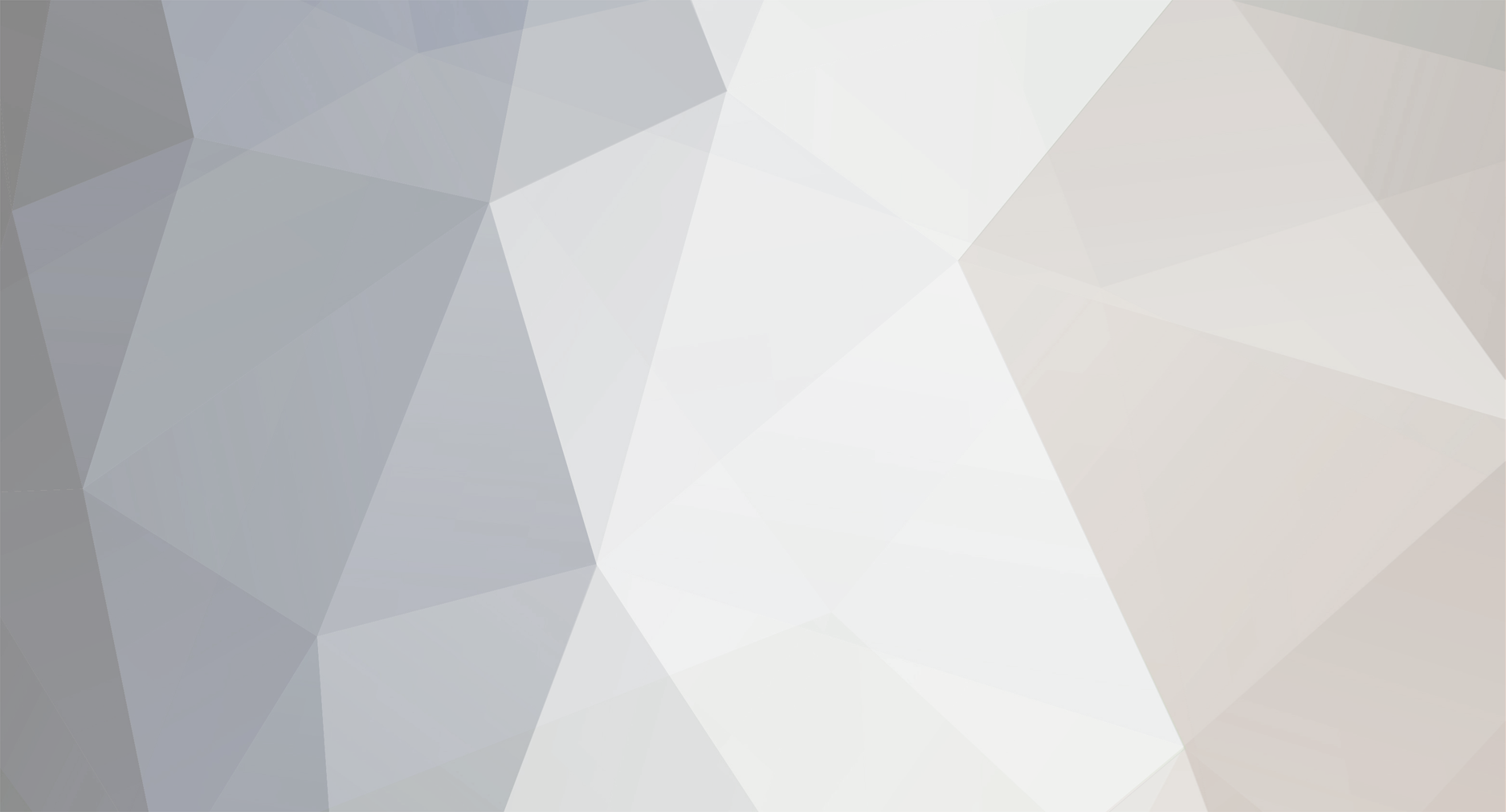
dreamwest
-
Posts
1,223 -
Joined
-
Last visited
Never
Posts posted by dreamwest
-
-
Im writing to a file but this put a blank line at the end, the reason why is because of r\n which is like<br> in html.
$current .= $buffer."r\n"; //has over 1000 lines file_put_contents($save_as, $current);
How can i get rid of this blank line at the end of the file??
-
this is my first go at file open and write. I need to delete a file on a specific line:
This file contains lines like
something|something
something|
something|something
something|something
So a error will script will output "ERROR on line 2 - something|"
$handle = @fopen("1.txt", "r"); if ($handle) { $count = 1; while (!feof($handle)) { $buffer = trim(fgets($handle, 4096)); //echo $buffer."<br>"; $check = explode('|', $buffer); if ($check[1] == "" || $check[1] == " " ){ echo "ERROR on line {$count} - {$buffer}<br>"; } $count = $count +1; } fclose($handle); }
Now all i need to do is delete that whole line from the file
-
Your right
-
<?php $page = "http://www.youtube.com/browse?s=bzb"; $page_contents = file_get_contents($page); $names = preg_match_all('/<span class="video-username"><a id="video-from-username-.*" class="hLink" href="\/user\/(.*)">.*<\/a><\/span>/', $page_contents, $matches); print_r($matches[1]); ?>
Thank you so much, this is exactly what I was looking for!
How would I list them without the array information? For example, make it say this:
user1, user2, user3
This is more flexible:
foreach ($matches[1] as $value) { echo $value; // add anything you want in front of or after }
-
<?php $page = "http://www.youtube.com/browse?s=bzb"; $page_contents = file_get_contents($page); $names = preg_match_all('/<span class="video-username"><a id="video-from-username-.*" class="hLink" href="\/user\/(.*)">.*<\/a><\/span>/', $page_contents, $matches); print_r($matches[1]); ?>
Output
Array ( [0] => ifbnews [1] => sigves [2] => lunamaria0708 [3] => PrismWeapon [4] => sugoidrama070109 [5] => ebonite45 [6] => AluminumFoils [7] => VenetianPrincess [8] => NGRNinfa [9] => cheezburger [10] => MootPoon817 [11] => SouljaBoy [12] => NationalGeographic [13] => jobros1love1dream2 [14] => cplfreeman [15] => 10LMessi7CRonaldo [16] => betamaxdc [17] => failblog [18] => DjGhostM [19] => jameslikecoulter [20] => XxNewXDisneyxX [21] => MondoMedia [22] => NokiaConversations )
Is this quicker than CURL or the same speed/resources??
-
$result = mysql_query( "select stuff"); while ( $record = mysql_fetch_assoc( $result ) ){ $counter = 0; echo $row['id']; if($counter == 2){ echo "Stopped!"; break; } $counter = $counter + 1; }
-
mysql_query( "UPDATE `table` SET download = download + 1 WHERE id = 'your id here' ");
-
$_SERVER['REQUEST_URI'] should give you that information without having to do rewrites, I think.
Your right. My way was getting a little buggy so i tried yours and it works.
Thanks!
-
Finally figured it out
# provide a universal error document RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteRule ^(.*)$ /404.php?url=$1 [L]
The just use $_GET in 404.php
-
Im interested in seeing what theyre after, itll give me some insite on how to better secure the site
Over the last 5 mins ive had 5 different ips trying to access restricted files, i dont want to ban them yet i want to spy on them and see what they do
-
$scheme = !empty($_SERVER['HTTPS']) && 'on' === $_SERVER['HTTPS'] ? 'https' : 'http'; echo $scheme, '://', $_SERVER['HTTP_HOST'], $_SERVER['SCRIPT_NAME'];
Almost what im looking for.. Im trying to track ppl trying to hack my site
I have this in htaccess: ErrorDocument 404 /404.php , so whenever someone tries to guess urls:
http://site.com/admin/something.php it will automatically go to http://site.com/404.php. But $_SERVER['HTTP_REFERER']; isnt working for this.
-
How can i get the current url without being referred from another page.
I can get a referred url : $_SERVER['REMOTE_ADDR']
But if i just typed in the url in the address bar : http://site.com/url.php, how can i echo this url out
-
Im trying to create a unique field, but when i test it and try to insert a duplicate row i get a error
error: Duplicate entry 'test' for key 2
Is there a way i can test the string "test" to see if its unique before inserting it??
-
$content_count = 1000; $page = $_GET['page']; $tpage = ceil( $content_count / 10 ); $startfrom = ( $page - 1 ) * 10; $prev = $page - 1; $next = $page + 1; $adjust = 9; if ( 1 < $page ) { $page_links .= "<a href='".$prev."'><< Prev</a><a href='1'>1</a>...."; } $k = $page - $adjust; for ( $page ; $k <= $page + $adjust; ++$k ) { if (( 0 < $k ) && ( $k <= $tpage ) ) { if(($page)==($k)){ $page_links.="<span> ".$k." </span>"; } else { $page_links .= "<a href='".$k."'>{$k}</a>"; } } } if ( $page < $tpage ) { $page_links .= ".....<a href='".$tpage."'>".$tpage."</a><a href='".$next."'>Next >></a>"; } echo $page_links;
Your stuffed with a total
Thanks, but it goes to 100, some searches only need to 2 pages, not all have 100 pages, the ones that dont have 100 pages just loop continuously until they get to 100.
Yeah what i meant to say was - Your stuffed without a total .
You need to be getting $content_count = ????????; which is the total amount of items from somewhere
-
$content_count = 1000; $page = $_GET['page']; $tpage = ceil( $content_count / 10 ); $startfrom = ( $page - 1 ) * 10; $prev = $page - 1; $next = $page + 1; $adjust = 9; if ( 1 < $page ) { $page_links .= "<a href='".$prev."'><< Prev</a><a href='1'>1</a>...."; } $k = $page - $adjust; for ( $page ; $k <= $page + $adjust; ++$k ) { if (( 0 < $k ) && ( $k <= $tpage ) ) { if(($page)==($k)){ $page_links.="<span> ".$k." </span>"; } else { $page_links .= "<a href='".$k."'>{$k}</a>"; } } } if ( $page < $tpage ) { $page_links .= ".....<a href='".$tpage."'>".$tpage."</a><a href='".$next."'>Next >></a>"; } echo $page_links;
Your stuffed with a total
-
If your table looks like this:
1 table for users: userID(auto increment) username password....
1 table for items id (auto increment) userID item desc image....
1 table for userItems id (auto increment) userID itemID
Then the query will be:
$user = 2; $results = mysql_query("SELECT * FROM users AS u, item as i WHERE u.userID = '{user]' and u.userID = i.userID ");
Think of userID as your anchor to other tables
-
Your going to end up with a thousand queries if the user has a thousand items
$results = mysql_query("SELECT * FROM users AS u, item as i WHERE u.ID = i.ID "); echo "xml=<?xml version=\"1.0\"?>"; echo "<items>"; while($line = mysql_fetch_assoc($results)){ echo "<val>". $line["ID"] . "</val>"; echo "<val>". $line["item"] . "</val>"; echo "<val>". $line["image"] . "</val>"; } echo "</items >";
Of couse thats assuming ID relates to the actual user and not auto increment
I would have it like this
1 table for users: userID(auto increment) username password....
1 table for items id (auto increment) userID item desc image....
1 table for userItems id (auto increment) userID itemID
-
No, it's actually turned into this monstrous piece of code (that is the cached version):
Yeah i know - you should see my compiled code, some pages are over 2000 lines. But regardless of this and the extra requests i bet you saw a decrease in frontend page loading time
I went from having 10+ second loading times to < 1 second +- 0.5 seconds - thats what gets me excited
Wow.... So apparently compiled Smarty code = fail. The first thing that jumps out at me is this:smarty_core_load_plugins(array('plugins' => array(array('modifier', 'escape', 'mysqltable.tpl', 5, false),array('modifier', 'date_format', 'mysqltable.tpl', 7, false),)), $this);
Since the file is included inside of a method, that means that there is the potential for the same plugins to be loaded over and over again.
Ill look into this...Im sure they wouldnt allow this bug
-
Have 1 table to store all users info
Theres no benefit to having multiple tables if theyre on the same machine - and its easier to have them in one spot
-
I notice you're still calling Avant a browser
If wikipedia says it is - it is http://en.wikipedia.org/wiki/Avant_Browser
C:\Users\daniel>ab -c 10 -n 1000 http://localhost/smarty_test/mysqltable_smarty.php This is ApacheBench, Version 2.3 <$Revision: 655654 $> Copyright 1996 Adam Twiss, Zeus Technology Ltd, http://www.zeustech.net/ Licensed to The Apache Software Foundation, http://www.apache.org/ Benchmarking localhost (be patient) Completed 100 requests Completed 200 requests Completed 300 requests Completed 400 requests Completed 500 requests Completed 600 requests Completed 700 requests Completed 800 requests Completed 900 requests Completed 1000 requests Finished 1000 requests Server Software: Apache/2.2.11 Server Hostname: localhost Server Port: 80 Document Path: /smarty_test/mysqltable_smarty.php Document Length: 9574 bytes Concurrency Level: 10 Time taken for tests: 7.575 seconds Complete requests: 1000 Failed requests: 0 Write errors: 0 Total transferred: 9740000 bytes HTML transferred: 9574000 bytes Requests per second: 132.01 [#/sec] (mean) Time per request: 75.754 [ms] (mean) Time per request: 7.575 [ms] (mean, across all concurrent requests) Transfer rate: 1255.60 [Kbytes/sec] received Connection Times (ms) min mean[+/-sd] median max Connect: 0 1 2.1 0 15 Processing: 17 74 26.4 71 209 Waiting: 15 73 26.5 69 207 Total: 19 75 26.3 72 209 Percentage of the requests served within a certain time (ms) 50% 72 66% 83 75% 90 80% 96 90% 112 95% 124 98% 139 99% 154 100% 209 (longest request)
More or less the same.
It doesnt make sense - ive tested normal code against smarty. My pages load within 1 second with smarty and has over 800 lines (formatted) of code. Smarty is slower without compile check because every time a page is viewed it checks to see if the file has been modified. So once youve finished designing the site use
$smarty->compile_check = false;
before displaying the templates and wella like magic
So instead of running this all the time:
<?php require './mysqltable_init.php'; echo '<h1>PHP Freaks Tutorials</h1>'; while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { echo '<div class="tutorial" id="tut-' . $row['content_id'] . '">' . '<h2>' . htmlentities($row['title']) . '</h2>' . '<small>' . date('F j, Y, H:i', strtotime($row['created_at'])) . '</small>' . '<div class="summary">' . htmlentities($row['summary']) . '</div>' . '<a href="' . htmlentities($row['permalink']) . '">Read more</a>' . '</div>'; }
It only compiling the php page :
<?php $db = new PDO('mysql:host=localhost;dbname=test', 'root', '******'); $stmt = $db->query('SELECT content_id, title, permalink, summary, created_at FROM content ORDER BY created_at DESC');
because the template is already compiled
Dont belive me? I dare you to try it in a real application, generate the template first by going to the php page then any further requests by anyone will be SUPA fast
-
Can you add this just under new Smarty and do the test again, im interested at seeing how fast this speeds it up:
$smarty = new Smarty;
$smarty->compile_check = false;
-
Lately i have been able to increase my sites performance with smarty by well over 500%.
I can build apps in a fraction of the time than traditional scripting and enjoy the performance, i can assign a simple query:
while ($row = mysql_fetch_assoc( $result )){ $show_content[] = $row; } $smarty->assign( 'show_content', $show_content );
and pull bits and pieces out separating content and code
{$show_content[i].field}
Its all so simple and flexible - I lov it!
I think this can go in my hall of fame for best apps
1. BING
2. Smarty
3. Avant browser
-
Yes. You don't need curly braces {} around simply if statements. Also, include is not a function and therefore doesn't require its arguments be enclosed within () braces.
It just looks better. Having
if($_COOKIE['snap']== "on" )
$snap = "on";
looks wrong. But having { is like having a border around the code to easily see the blocks
if($_COOKIE['snap']== "on" ){
$snap = "on";
}
-
$result = mysql_query("SELECT distinct * FROM table group by name"); while ($row = mysql_fetch_assoc($result)){ echo " <tr> <td>{$row['name']}</td> <td>{$row['city]}</td> <td>{$row['state']}</td> </tr>";
blank line at the end of the file??
in PHP Coding Help
Posted
!somehow! work out when the last line will be
I need the written file to be line by line so i cant remove it completley