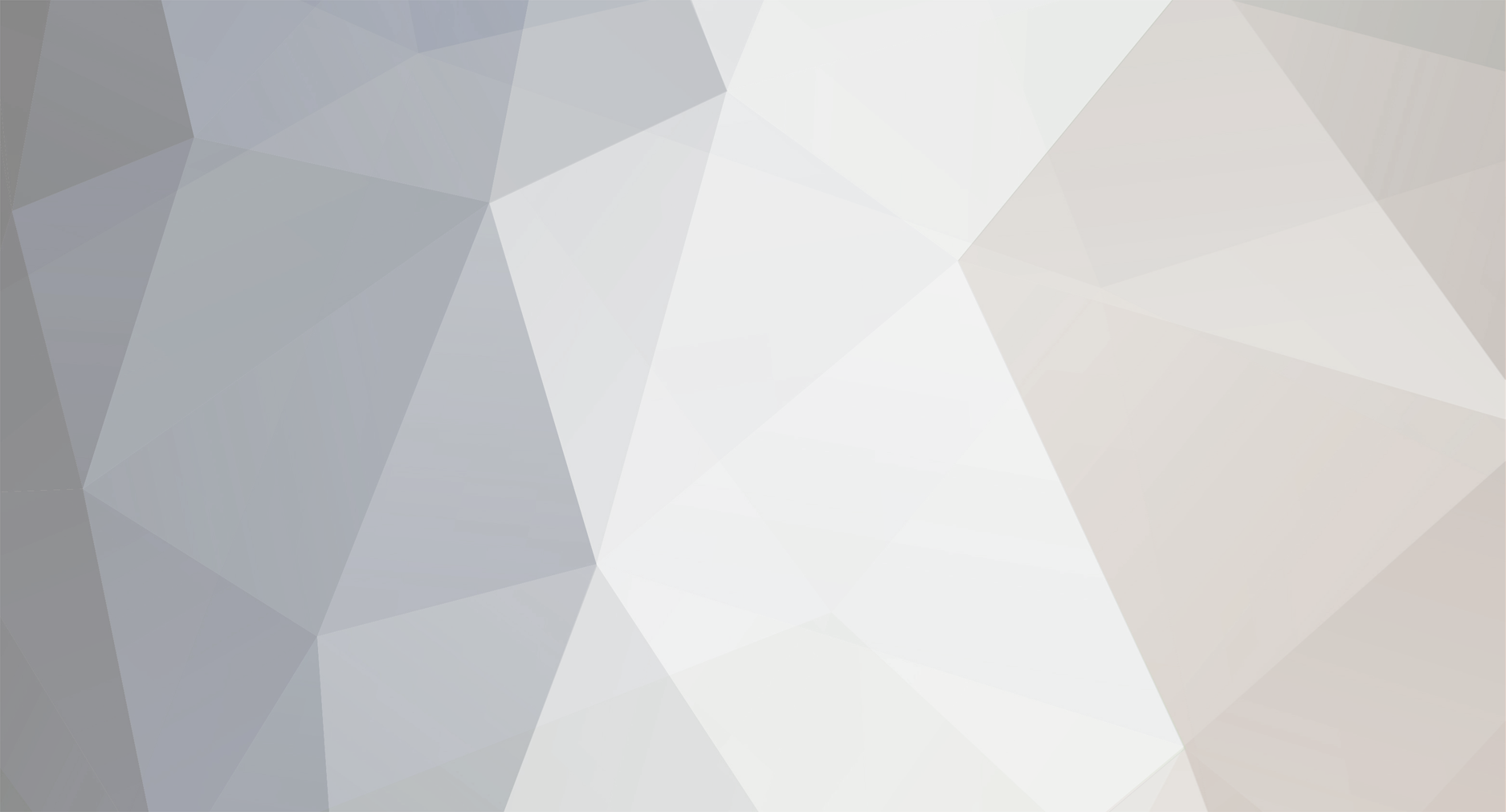
aximbigfan
Members-
Posts
329 -
Joined
-
Last visited
Never
Everything posted by aximbigfan
-
Is there any way to fix it? Recursive/loop stuff is not my strong point.. Thanks, Chris
-
I have this code (Thanks Aidan Lister!) function copyr($source, $dest) { // Simple copy for a file if (is_file($source)) { return copy($source, $dest); } // Make destination directory if (!is_dir($dest)) { mkdir($dest); } // If the source is a symlink if (is_link($source)) { $link_dest = readlink($source); return symlink($link_dest, $dest); } // Loop through the folder $dir = dir($source); while (false !== $entry = $dir->read()) { // Skip pointers if ($entry == '.' || $entry == '..') { continue; } // Deep copy directories if ($dest !== "$source/$entry") { copyr("$source/$entry", "$dest/$entry"); } } // Clean up $dir->close(); return true; } Say I have this dir /Test1/Test2/Test3/ Say I try to copyr("/Test1/Test2/", "/Test1"); The end result is that nothing happens. No errors. Literally, nothing. Anyone see any reason for this to be happening? It does NOT happen when I copyr("/Test1/Test2", "/otherdir/"); Thanks, Chris
-
Huh... Well... Drat... That was... Simple... Thanks! Chris
-
Hi, I have the following code. function recurse_copy($source, $target) { if (is_dir($source)) $dir_handle = opendir($source); $dirname = substr($source, strrpos($source, '/')+1); mkdir($target.'/'.$dirname,0777); while($file=readdir($dir_handle)) { if ($file!='.' && $file!='..') { if (!is_dir($source.'/'.$file)) copy($source.'/'.$file, $target.'/'.$dirname.'/'.$file); else { $target1 = $target.'/'.$dirname; $this->recurse_copy($source.'/'.$file, $target1); } } } closedir($dir_handle); return true; } The function works somewhat, BUT... It isn't copying the source dir to the new dir, it is copying the CONTENTS of the source dir, to the target dir. if I were to do this: recurse_copy("/opt/", "/opt2"); Lets assume that in /opt/ is /1/2/3/4 Now, after the above code is executed, the structure of /opt2/ will look like this: /opt2/1/2/3/4 What I would like it to look like is: /opt2/opt/1/2/3/4 Is there any way to solve this? I have a mental block when it comes to working with loops like this... Thanks! Chris
-
Hi, I have here the source code to a PHP socket server that is "multithreaded". The issue is that it will only accept up to $max_clients connections. Can anyone see any way to modify this so that it will accept more than $max_clients connections? <?php while (true) { $read[0] = $sock; for ($i = 0; $i < $max_clients; $i++) { if ($client[$i]['sock'] != null) $read[$i + 1] = $client[$i]['sock'] ; } $ready = socket_select($read,null,null,null); if (in_array($sock, $read)) { for ($i = 0; $i < $max_clients; $i++) { if ($client[$i]['sock'] == null) { $client[$i]['sock'] = socket_accept($sock); break; } elseif ($i == $max_clients - 1) echo "Client overflow error"; } if (--$ready <= 0) continue; } // If a client is trying to write - handle it now for ($i = 0; $i < $max_clients; $i++) // for each client { if (in_array($client[$i]['sock'] , $read)) { $input = socket_read($client[$i]['sock'] , 1024); //Stuff here } } else { // Close the socket socket_close($client[$i]['sock']); unset($client[$i]); } } } socket_close($sock); ?> Loops aren't my thing.. Thanks, Chris
-
I have been struggling with filesystem stuff for a bit now. I am trying to make a function to copy one dir or file, to another dir. like this source target recurse_copy("/test1/test2", "/testa/testb"); My current code is this, but it is making the complete dir structure leading up to the dir I want to copy, in the target. private function recurse_copy($source, $target) { $s2 = substr($source, 0, strrpos($source, '/')); if (is_dir($source)) $dir_handle = opendir($source); mkdir($target . '/' . $s2, 0777, true); while ($file = readdir($dir_handle)) { if ($file!="." && $file!="..") { if (!is_dir($source . '/' . $file)) copy($source . '/' . $file, $target . '/' . $s2 . '/' . $file); else $this->recurse_copy($source . '/' . $file, $target); } } closedir($dir_handle); return true; } Thanks, Chris
-
I am trying to make a function to move directories and files. When the function is called, I'm getting this error My code is simply rename("/test/testdir", "/test2/test3"); Thanks, Chris
-
[SOLVED] Remove everything after the last "/"?
aximbigfan replied to aximbigfan's topic in PHP Coding Help
Darn, it's not working. Here's my code. The idea is that I have a path like this, aaaa/bbb/ccc I want to rename ccc to something else. So I wanted to use the code I requested ot get the path before ccc, so I could append the new name for ccc on to it, and rename it there. SNIP.... { $old_new = substr($dir, 0, strpos($dir, '/')); $path_old = $this->opt_regd . $dir; $path_new = $this->opt_regd . $old_new . $new; } if (!rename($path_old, $path_new)) /SNIP... EDIT: I'm an idiot. I missed that extra "r". I'll blame it on my laptop keyboard. Clearly I typed it, but the keyboard dropped it. Thanks! Thanks, Chris -
[SOLVED] Remove everything after the last "/"?
aximbigfan replied to aximbigfan's topic in PHP Coding Help
Perfect, thanks! And yeah, it is for dirs. It is for renaming the top level dir. Chris -
Hi, I need to find a way to remove everything after the last "/" in a string. For example, this: /this/is/a/string Needs to become this /this/is/a Thanks! Chris
-
Yep. PHP can't play music. It is a serverside scripting lang. With some obscure plugin, it may be able to output sound on CLI applications, but otherwise, no. Chris
-
Hi DarkWater, I think what he means is if you are running PHP, is there a predefined variable for the local computer's MAC or asset number. I can say that the asset number is a definite "no", without a special plugin. For the MAC address, I'm not exactly sure. I would hazard to say no, but honistly PHP is constantly surprising me with all the obscure stuff it has built in. For example, I had no iea PHP had low-level socket functions. Chris
-
(Interesting characteristic about machine)(integer) For example MOBILE2 (My tablet) S1 (My X86 server) DUAL1 (My dual core desktop) Normally the integer is in order from when the machine was purchased/built. DUAL one was my first dual core desktop, S1 was my first X86 server, and MOBILE2 was my second mobile system. MOBILE1 was re purposed, and was no longer mobile. For my embedded devices, it's pretty much whatever comes to mind MV21 (My HP Mediavault MV2120, running Debian Etch) HPMV (My original HP MediaVault MV2010, running my own custom firmware) LSLUG (My Linksys NSLU2 running Unslung) Chris
-
What is the highest performance hashing method that comes with PHP? I am currently using MD5, but was wondering if there is something that might be slightly faster. Thanks, Chris
-
Huh... Well there goes about 15minutes implementing a system which I thought was smart. Thanks for your help everyone! Chris
-
Actually, I was trying to solve a problem before it started. So I could do a file_put_contents("test/test123/test.txt","This-is-a-test"); on Windows and it would work? Thanks, Chris
-
I need some way of figuring out if I'm in a Linux or Windows environment. I looked at predefined stuff, but they don't give me a solid answer. This is needed because I need to know whether to use "/"s or "\"s in paths. Thanks, Chris
-
Obviously you haven't explored the limits of php or your definition of a game is pretty weak because there are a ton of great games written in php out there. How? Your not going to do vectors and other things of the like in PHP. Are you talking about using external plugins or something, like GTK only not? EDIT: I see what you mean, I thought he wanted to make a game completely out of PHP, where the game's core was made from PHP code. Chris
-
First off, the closest to a game your going to get with PHP is going to be a text thing. PHP can't do games like Halo or any web based games. I think you really want to learn flash. PHP is only going to be good if you need a socket server to store scores and the like. If you do need a socket server, PM me. Chris
-
Thanks! I see a way I may be able to do what I want with explode(). Chris
-
No, I need to write the values, not read them. Basically, the function is going into the file, finding the directive with the pattern, than replacing the directive's setting with a new one, by replacing the entire line. Thanks, Chris
-
Thanks! Is there a spot where I can put a varitable, and it will find that directive in the haystack? Say the "input var" is "option_address". The haystack will look like this: opt_port = "123" opt_address = "MV2" opt_auth = "test" I'll use preg_replace to use that pattern and try to find "opt_address"'s directive, then remove that whole line, and put a new one in it's place, with a new setting. I have everything else figured out, I just need a pattern I can plug a var into, then have it find the directive. Thanks alot for your help! Chris
-
Hi, I am not very good at regExp. I need a pattern that will find a line like this $somevar1 = "randomstring" The first var name may have any chars in it (except '=' and ' ') then there will be some spaces, and '=' then a second string in between ""s . This will be used in a function that finds a setting in an INI file, and replaces it with a new setting. For example, preg_replace("pattern with variable name in it", "the new string", "the string"); Thanks! Chris
-
HappyLand - Kids game built for Lutheran Media
aximbigfan replied to matthewjumpsoffbuildings's topic in Website Critique
Try listening to Teech with Pink Floyd's "In the flesh" playing At any rate, about the boat thing, what about if once the boat reached the island, and the game had not loaded completely yet, if another animation were to play. It's kind of hard to explain this... Chris -
HappyLand - Kids game built for Lutheran Media
aximbigfan replied to matthewjumpsoffbuildings's topic in Website Critique
I did a quick test, while listening to a Pink Floyd track... That was one messed up experience... ...Anyway seems to work pretty well, with the "big button" style in which it is in. Chris