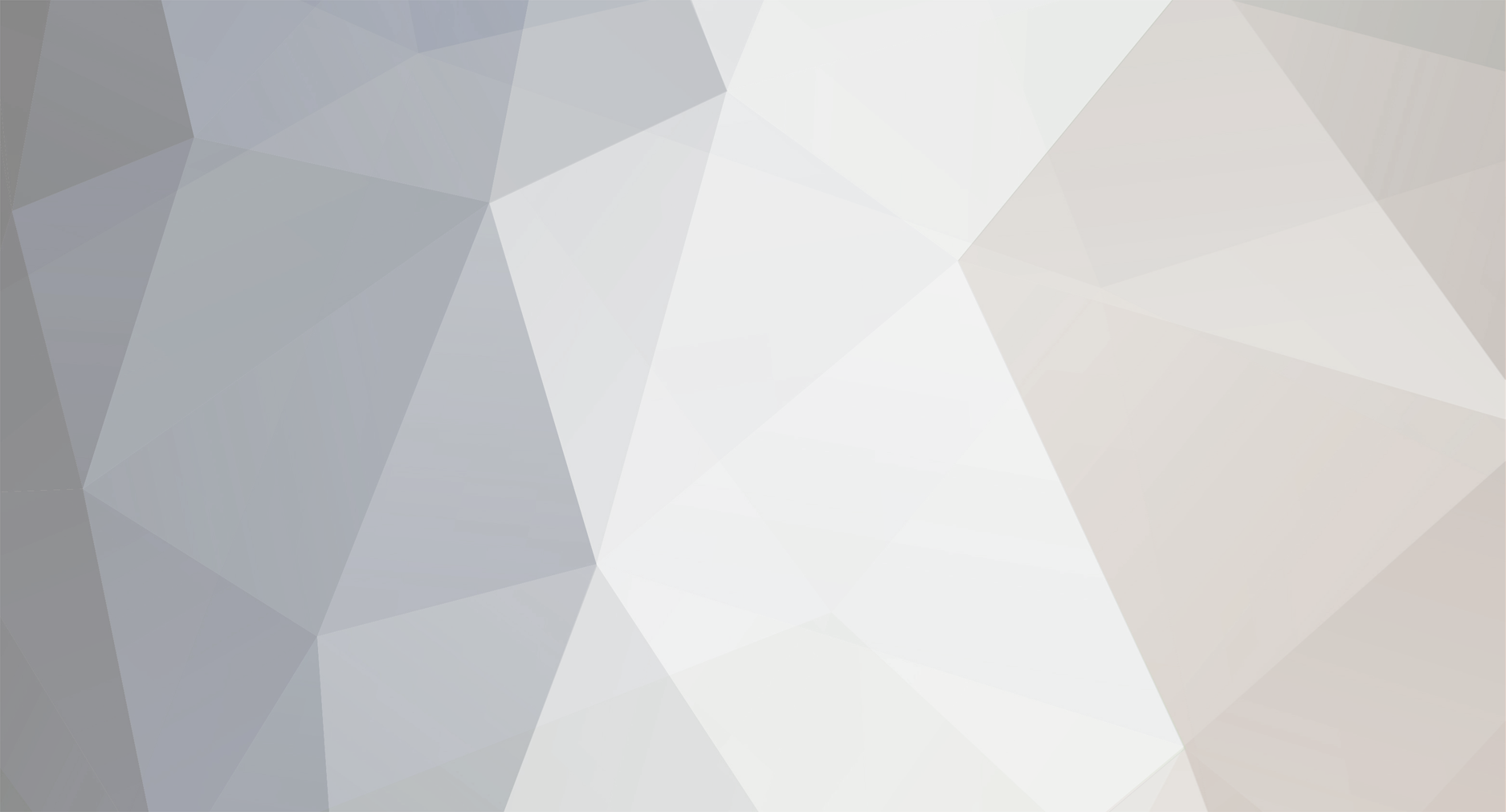
jonsjava
-
Posts
1,579 -
Joined
-
Last visited
Posts posted by jonsjava
-
-
The first parameter has to either be a string of name of the class you're testing or either an instance of the class. So in your example either 'test' or $test. The second parameter has to be a string, 'test2'.
if (method_exists($test, 'test2')){ echo "found method"; }
or
if (method_exists('test', 'test2')){ echo "found method"; }
Forgot to mention that. Thanks, Alex.
-
dirty way. Ok, I'll show the proper method:
<?php class test{ public function test2(){ echo "hello"; } } $test = new test(); if (method_exists(test,test2)){ echo "found method"; } ?>
-
sounds like schoolwork. No cheating.
-
here's a safer way to do what you are wanting to do:
<?php function clearup($input){ if (is_array($input)){ foreach ($input as $key=>$val){ $out[$key] = mysql_real_escape_string($val); } } else{ $out = mysql_real_escape_string($input); } return $out; } $post = clearup($_POST); $get = clearup($_GET); $ip = $_SERVER['REMOTE_ADDR']; $sql="INSERT INTO `articlecomments`(`articleID`, `posterNickname`, `email`, `comment`, `ip`) VALUES ('{$get['id']}','{$post['name']}','{$post['email']}','{$post['comment']}', '$ip')"; mysql_query($sql); ?>
-
where are you storing the IP address? Does your database table have a column for IP address?
-
as far as dream weaver -- don't know. Haven't used it in years. Vim is my friend
about the time: PHP gets the time from the server.
-
bad code in my last example. That's what I get for using untested code.
<?php $image_dir = "images/countdown"; function countdown($month,$day,$year) { // subtract desired date from current date and give an answer in terms of days $remain = ceil((mktime(0,0,0,$month,$day,$year) - time()) / 86400); // show the number of days left if ($remain > 0) { return $remain; } // if the event has arrived, say so! else { return "arrived"; } } $days = countdown(7,11,2010); $image = $image_dir."/$days.gif"; //assuming the image set is gif, and you have them named 1.gif, 2.gif, 3.gif,... and one for arrived.gif echo "<img src='$image'>"; ?>
-
lol. I copy pasted the cases, and forgot to renumber.
cases should be 1,2,3,4,...
-
here's a sample of what you can do. Simple, quick, dirty, but it should work. If you need help understanding anything, let us know.
<?php $image_dir = "images/countdown"; $day = 31; // Day of the countdown $month = 12; // Month of the countdown $year = 2010; // Year of the countdown $hour = 23; // Hour of the day (east coast time) $event = "New Year's Eve, 2010"; //event $calculation = ((mktime ($hour,0,0,$month,$day,$year) - time(void))/3600); $days = (int)($hours/24); $image = $image_dir."/$days.gif"; //assuming the image set is gif, and you have them named 1.gif, 2.gif, 3.gif, etc. echo "<img src='$image'>"; ?>
-
injections are easy. They can take your form, change the type of the fields, and enter whatever they like.
-
could cause problems when the subject isn't in the array. My method has a catch-all.
-
Without going into code, I will tell you my experience with writing SOAP calls.
When I first started writing them, I had issues, because their SOAP calls didn't always conform to the standards. I found a tool (soapui) to help me build the basic calls, and then reverse-engineered the calls for PHP. What I would recommend you do is find a good SOAP client, get the hang of it, get used to calls, and then build from there. You can do calls from SOAPui without much work at all. it will even build the call templates for you.
-
Here's how I usually do it:
I make a drop-down with possible subjects. each has an integer value. On the back side, I do something like this:
$subject = userData($_POST['subject']); switch ($subject){ case 1: $subj = "hello"; $sendmailto = "someuser@yoursite.com"; break; case 2: $subj = "need assistance"; $sendmailto = "someuser2@yoursite.com"; break; case 1: $subj = "I'm bored"; $sendmailto = "someuser3@yoursite.com"; break; case 1: $subj = "Cheeseburger in paradise"; $sendmailto = "someuser3@yoursite.com"; break; default: $should_i_send = false; }
Now, how this works: it gets the integer for the subject. If the integer is in the list of cases, it sets the subject to a text value, and changes the sendmailto to a given user. If the subject isn't in the list of allowed integers, it drops them.
-
noticed that I didn't see you executing the queries:
<?php echo "<h1>Register</h1>"; $submit = $_POST['submit']; $username = ucfirst(strip_tags($_POST['username'])); $password = strip_tags($_POST['password']); $confpassword = strip_tags($_POST['confpassword']); $email = strip_tags($_POST['email']); $fname = ucfirst(strip_tags($_POST['fname'])); //$ref = strip_tags($_POST['ref']); $joindate = date("Y-m-d"); //I should only give the 50 credits once the user has surfed OR activated email? $credits = 50.000; $ip = $_SERVER['REMOTE_ADDR']; $level = 1; if ($submit) { include('inc/connect.php'); $namecheck = mysql_query("SELECT username FROM users WHERE username='$username'"); $usernamecount = mysql_num_rows($namecheck); $emailcheck = mysql_query("SELECT email FROM users WHERE email='$email'"); $emailcount = mysql_num_rows($emailcheck); if ($usernamecount!=0) { die("Username already taken!"); } if ($emailcount!=0) { die("E-mail already being used"); } function validEmail($email) { $isValid = true; $atIndex = strrpos($email, "@"); if (is_bool($atIndex) && !$atIndex) { $isValid = false; } else { $domain = substr($email, $atIndex+1); $local = substr($email, 0, $atIndex); $localLen = strlen($local); $domainLen = strlen($domain); if ($localLen < 1 || $localLen > 64) { // local part length exceeded $isValid = false; } else if ($domainLen < 1 || $domainLen > 255) { // domain part length exceeded $isValid = false; } else if ($local[0] == '.' || $local[$localLen-1] == '.') { // local part starts or ends with '.' $isValid = false; } else if (preg_match('/\\.\\./', $local)) { // local part has two consecutive dots $isValid = false; } else if (!preg_match('/^[A-Za-z0-9\\-\\.]+$/', $domain)) { // character not valid in domain part $isValid = false; } else if (preg_match('/\\.\\./', $domain)) { // domain part has two consecutive dots $isValid = false; } else if (!preg_match('/^(\\\\.|[A-Za-z0-9!#%&`_=\\/$\'*+?^{}|~.-])+$/', str_replace("\\\\","",$local))) { // character not valid in local part unless // local part is quoted if (!preg_match('/^"(\\\\"|[^"])+"$/', str_replace("\\\\","",$local))) { $isValid = false; } } if ($isValid && !(checkdnsrr($domain,"MX") || checkdnsrr($domain,"A"))) { // domain not found in DNS $isValid = false; } } return $isValid; } // Check for filled out form if ($username&&$password&&$confpassword&&$email&&$fname) { //Encrypt password if ($password==$confpassword) { if (strlen($username)>25) { echo "Max limit for Username is 25 characters"; } if (strlen($password)>32||strlen($password)<6) { echo "Password must be between 6 and 32 characters"; } else { //Register the user $password = md5($password); $confpassword = md5($confpassword); echo "Success!"; mysql_query("INSERT INTO users VALUES ('','$username','$email','$fname','','','','$joindate','$password','$ip')"); mysql_query("INSERT INTO userstats VALUES ('','$username','$level','','$credits','','','','')"); header("location:index.php"); } if (strlen($email)>25) { echo "Max limit for E-mail is 64 characters"; } if (strlen($fname)>25) { echo "Max limit for First Name is 25 characters"; } /*if (strlen($lname)>25) { echo "Max limit for Last Name is 25 characters"; }*/ } else echo "Your passwords do not match!"; } else echo "Please fill in <strong>all</strong> fields!"; } ?> <html> <head> <link rel="stylesheet" type="text/css" href="style.css" /> <script type="text/javascript" language="javascript"> function inputLimiter(e,allow) { var AllowableCharacters = ''; if (allow == 'FirstNameChar'){AllowableCharacters='ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz';} if (allow == 'UsernameChar'){AllowableCharacters='ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz1234567890';} var k; k=document.all?parseInt(e.keyCode): parseInt(e.which); if (k!=13 && k!=8 && k!=0){ if ((e.ctrlKey==false) && (e.altKey==false)) { return (AllowableCharacters.indexOf(String.fromCharCode(k))!=-1); } else { return true; } } else { return true; } } </script> </head> <body> <form action="register.php" method="POST"> <div id="register">Username: <input type="text" id="UsernameChar" onkeypress="return inputLimiter(event,'UsernameChar')" name="username" maxlength="25" value="<?php echo $username ?>"><br /> Password: <input type="password" maxlength="32" name="password"><br /> Confirm Password: <input type="password" maxlength="32" name="confpassword"><br /> First Name: <input type="text" id="FirstNameChar" onkeypress="return inputLimiter(event,'FirstNameChar')" name="fname" maxlength="25" value="<?php echo $fname ?>"><br /> E-mail: <input type="text" name="email" maxlength="64" value="<?php echo $email ?>"><br /> <input type="submit" name="submit" value="Register"></div> </form> </body> </html>
-
try something like this:
set a session variable, that once set flags the system to not re-check the IP:
<?php session_start(); if (!isset($_SESSION['ip_checked']) || $_SESSION['ip_checked'] != true){ //run the check }
Now, on the script that does the check with fsockopen()
<?php session_start(); if (!isset($_SESSION['ip_check']) || $_SESSION['ip_check'] != true){ // check them $_SESSION['ip_check'] = true; $_SESSION['location'] = $LOCATION_FOUND_FROM_WEB_SERVICE; }
Complete pseudocode, but it should point you in the right direction.
-
A breakdown of your script, as read:
run a query, and return everything in the table.
While parsing through the results, assign variable $id to equal column "transid".
Insert into the table named `table_2` at columns name, city the values "john" and "london", respectively.
Delete all occurneces from `table_1` where the `id`=$id, even though it's true name is `transid`.
Ok, I think you see the issue here:
<?php $var1= mysql_query("SELECT `transid` FROM table1"); while ($var2= @mysql_fetch_assoc($var1)) { $id = $var2['transid']; mysql_query("INSERT INTO table 2(name, city) VALUES ('John', 'London')") or die (error25); mysql_query("DELETE FROM table1 WHERE `transid` = '$id' ") or die(mysql_error()); } ?>
-
I don't write to excel spreadsheets, per se, but I do write to CSV files, which excel...excels at reading.
<?php //sql goes here $newcount = 0; $res = mysql_query($sql); while ($row = mysql_fetch_assoc($res)){ if ($newcount == 0){ $out = implode("\",\"",array_keys($row))."\n"; $newcount++; } $out .= implode("\",\"",$row)."\n"; } header("Content-type: application/octet-stream"); header("Content-Disposition: attachment; filename=\"my-data.csv\""); echo $out; ?>
-
I should have told him to implode. need to have more caffeine.
-
try executing the SQL query:
while ($row = mysql_fetch_array($result)) { $f1=$row['firstname']; $e1=$row['emailname']; $sA= $_POST['sumA']; $sB= $_POST['sumB']; $sC= $_POST['sumC']; $sD= $_POST['sumD']; $sE= $_POST['sumE']; $sF= $_POST['sumF']; $sG= $_POST['sumG']; $sH= $_POST['sumH']; $sumTOTAL = $sA + $sB + $sC + $sD + $sE + $sF + $sG + $sH; ?> <?PHP mysql_select_db($db_name); $sql="INSERT INTO score (f1, scoreA, scoreB, scoreC, scoreD, scoreE, scoreF, scoreG, scoreH) VALUES ('$f1','$sA','$sB','$sC','$sD','$sE','$sF','$sG','$sH')"; mysql_qmery($sql); ?>
-
Here's the actual code I use when I use this:
foreach($_POST as $key => $value) { if ($value != "DELETE"){ $removal_sql = "DELETE FROM `{$_SESSION['username']}_table_{$list_name}` WHERE id={$value} LIMIT 1;"; mysql_query($removal_sql); }
So, as you can see, what I do is different, but you could take the ID, and query agains them:
<?php foreach($sanitized_post as $key=>$value){ $countme = 0; if (is_numeric($key)){ if ($countme == 0){ $q_values = "'$key'"; } else{ $q_values .= ",'$key'"; } } } $sql = "SELECT * FROM `tables` WHERE `id` IN ($q_values);";
-
When you set checkboxes, you use the primary key (id number, for example) as the integer to increment the keys. This way, you can say something like this
foreach ($checkbox as $key=>$val){ $sql = "DELETE FROM `table` WHERE `id`=$key;"; mysql_query($sql); }
-
I had a headache with this one myself. My dirty work-around: I gave each checkbox an integer as a name, so I could say:
<?php foreach($sanitized_post as $key=>$value){ if (is_numeric($key)){ $checkbox[$key] = $value; } }
-
I'm not certain how your database is configured, but wouldn't it be easier to do all the checking on the database?
SELECT COUNT(*) AS `total` FROM `forum_thread` WHERE `thread_boardid`='$boardid' AND `read` LIKE '%$logged_id%'
Here's the modified version:
<?php while($board = mysql_fetch_array($r2)){ $r3 = mysql_query("SELECT * FROM `forum_thread` WHERE `thread_boardid` = '".$board['board_id']."' AND `thread_deleted` = '0' ORDER BY `thread_id` DESC") or die(mysql_error()); $thread_count = mysql_num_rows($r3); $title_get3 = stripslashes($board['board_name']); $title3 = str_replace($title_current,$title_replace,$title_get3); echo " <tr> <td style='text-align:center;'> "; if($logged['userlevel']=='9'){ $boardid = $board['board_id']; $logged_id = $logged['id']; $get_new2 = mysql_query("SELECT COUNT(*) AS `total` FROM `forum_thread` WHERE `thread_boardid`='$boardid' AND `read` LIKE '%$logged_id'") or die(mysql_error()); $m = 1; $row = mysql_fetch_assoc($get_new2); $total = $row['total']; } echo $total; if($total != '0' && $logged['userlevel'] == '200'){ echo " <a href='".$url."forums".$a_act."markread".$a_view.$board['board_id'].$end."'>NEW</a> "; } else { echo " "; } ?>
Remember: this is a concept code, so you will need to change it to fit your needs, but if I understand what you are needing, that should be the way you want to go:
1) find all threads that are unread for that user
2) if there is anything unread, show them a link to mark them read.
-
I did some cleanup on your script, to make it smaller, and should be quicker.
<?php require_once('auth.php'); require_once('functions.php'); require_once('costs.php'); //Hehehe $login = "Ardivaba"; $result = mysql_query("SELECT * FROM squads WHERE user='$login'")or die(mysql_error()); $player = mysql_fetch_array( $result ); include("header.php"); $error = 0; if($_GET["action"] == "create_squad"){ $sqad_slots[] = (int)$_POST["create_squad_unit_specforce"]; $sqad_slots[] = (int)$_POST["create_squad_unit_sniper"]; $sqad_slots[] = (int)$_POST["create_squad_unit_assault"]; $sqad_slots[] = (int)$_POST["create_squad_unit_support"]; $sqad_slots[] = (int)$_POST["create_squad_unit_engineer"]; $sqad_slots[] = (int)$_POST["create_squad_unit_medic"]; $sqad_slots[] = (int)$_POST["create_squad_unit_antitank"]; $create_squad_units = array_sum($sqad_slots); if($create_squad_units > 5){ $teade = "You can't have that many units in squad!"; $error = 1; } if($squad_slots[0] > $player['unit_specforce']){ $teade = "You dont have that many Special Force units!"; $error = 1; } if($squad_slots[1] > $player['unit_sniper']){ $teade = "You dont have that many Sniper units!"; $error = 1; } if($squad_slots[2] > $player['unit_assault']){ $teade = "You dont have that many Assault units!"; $error = 1; } if($squad_slots[3] > $player['unit_support']){ $teade = "You dont have that many Support units!"; $error = 1; } if($squad_slots[4] > $player['unit_engineer']){ $teade = "You dont have that many Engineer units!"; $error = 1; } if($squad_slots[5] > $player['unit_medic']){ $teade = "You dont have that many Medic units!"; $error = 1; } if($squad_slots[6] > $player['unit_antitank']){ $teade = "You dont have that many Anti-Tank Force units!"; $error = 1; } if($error != 1){ $units = array(); foreach ($sqad_slots as $key=>$value){ switch ($key){ case 0: $name = "Special Force"; break; case 1: $name = "Sniper"; break; case 2: $name = "Assault"; break; case 3: $name = "Support"; break; case 4: $name = "Engineer"; break; case 5: $name = "Medic"; break; case 6: $name = "Anti-Tank"; break; default: $name = "Invalid"; } echo "Unit $name: $value<br />"; } } } ?> <?php include("side.php"); ?> <div id="main"> <p class="user-links"><a href="#">Register now</a> - <a href="#">Forgot password</a></p> <p class="time">Money: <span><?php echo $player['money']; ?></span></p> <div id="content"> <h2 class="cat"><a name="help_text" href="#help_text"><?php if(isset($teade)) { echo $teade; } else { echo "Here you can assemble squads"; } ?></a></h2> <div class="post"> <h3 class="post-title">Create squad</h3> <p class="post-time">You have: <span><?php echo $squad['squads']; ?> </span>squads</p> <div class="entry"> <p>Creating squads are vital part of this game. In order to explore the battlefield, you must have at least one squad assembled. To assemble an squad, you must choose 5 units, and then create squad. When you are making squad, try to make it as effective as possiple. Make sure you have medic in squad, so less soldiers will die in fight, they get wounded instead.</p> <form action="squads.php?action=create_squad" method="post">How many special forces units you wish to put in squad? <input type="text" name="create_squad_unit_specforce" /> <b>You have:</b> <?php echo $player['unit_specforce']; ?> <br /> <br /> How many sniper units you wish to put in squad? <input type="text" name="create_squad_unit_sniper" /><b>You have:</b> <?php echo $player['unit_sniper']; ?> <br /> <br /> How many assault units you wish to put in squad? <input type="text" name="create_squad_unit_assault" /> <b>You have:</b> <?php echo $player['unit_assault']; ?> <br /> <br /> How many support units you wish to put in squad? <input type="text" name="create_squad_unit_support" /> <b>You have:</b> <?php echo $player['unit_support']; ?> <br /> <br /> How many engineer units you wish to put in squad? <input type="text" name="create_squad_unit_engineer" /> <b>You have:</b> <?php echo $player['unit_engineer']; ?> <br /> <br /> How many medic units you wish to put in squad? <input type="text" name="create_squad_unit_medic" /> <b>You have:</b> <?php echo $player['unit_medic']; ?> <br /> <br /> How many anti-tank units you wish to put in squad? <input type="text" name="create_squad_unit_antitank" /> <b>You have:</b> <?php echo $player['unit_antitank']; ?> <br /> <br /> <input type="submit" name="action_create_squad" value="Create squad" /> <br /> <br /> </form> </div> </div> </div> <?php include("footer.php"); ?>
You will notice I explicitly set the $_POST values to integers, as well as pushing the POST to an array. Arrays are easier to work with when you are doing a foreach loop.
Tracking IP address
in PHP Coding Help
Posted
1) You weren't storing the IP address
2) You weren't sanitizing your inputs.