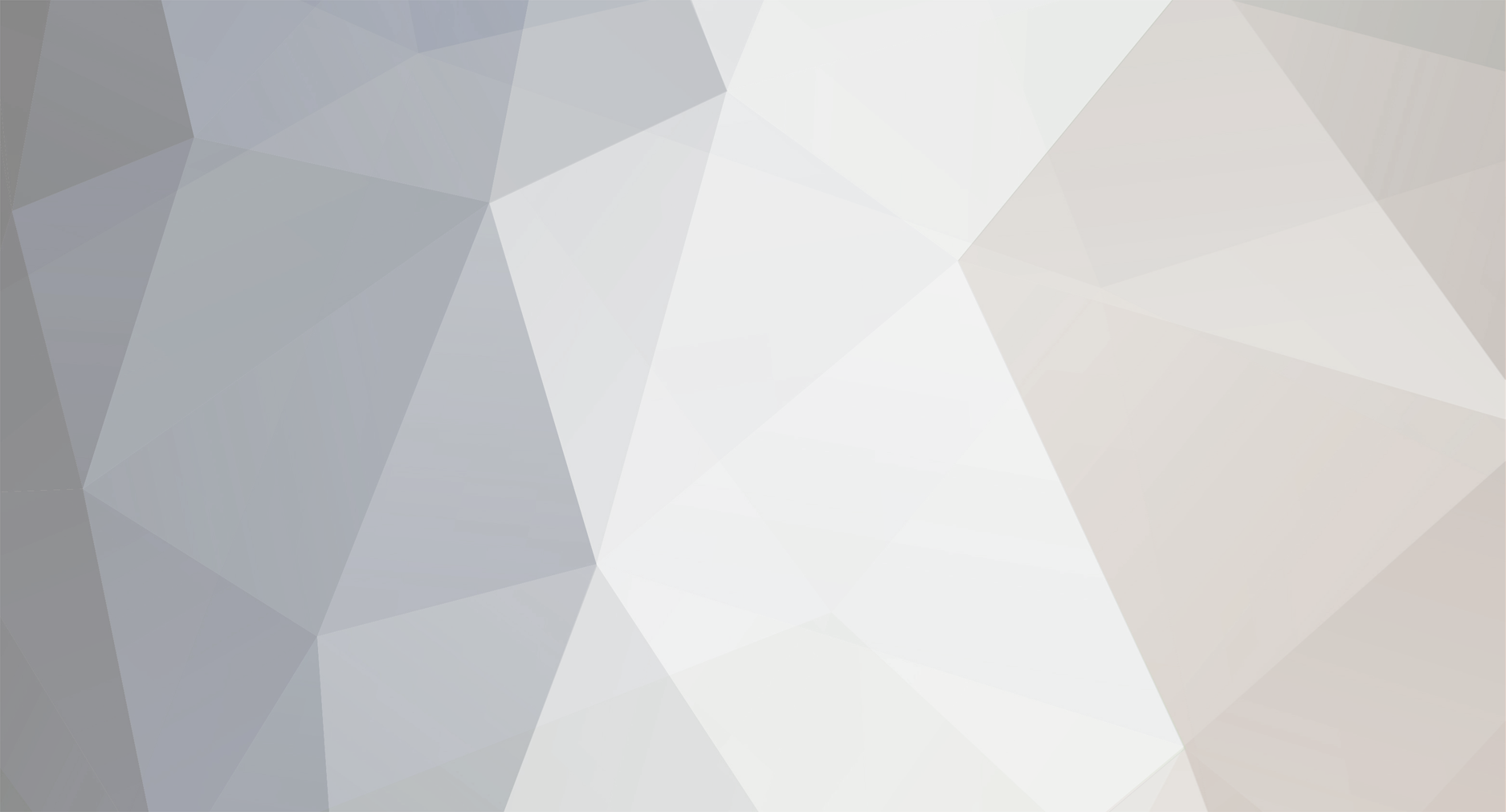
jonsjava
-
Posts
1,579 -
Joined
-
Last visited
Posts posted by jonsjava
-
-
That would be an AJAX request.
-
foreach isn't the best method for what you are doing:
<?php
$search->setsearchname($_POST['queryString']);
$results = $search->results ();
for ($i=0;$i<11;$i++){
echo "<li> <a href=\"suggest.php?mid=".$res->imdbid()."\">".$res->title()." (".$res->year()."</a></li>";
}
-
I'm blind some times. Sorry.
-
$descrip = nl2br($_SESSION['descriptemp']); $pageShowGuide = new Page(); $pageShowGuide->incpath = "../../"; $pageShowGuide->sidebar = ""; $pageShowGuide->title = $_SESSION['titletemp']; $pageShowGuide->content = " <div id=\"wide_main\"> <div id=\"guide_summ_".$_SESSION['colortemp']."\"> <div id=\"guide_pic\"> <img src=\"images/game/".$type."/".$img."\" /> </div> ".$descrip." </div> <h1>".$_SESSION['titletemp']."</h1> </div>"; $pageShowGuide->showPage();
you don't need preg_replace with nl2br
-
Here's a simple example for simplexml_load_file:
<?php $xml = simplexml_load_file("http://www.w3schools.com/XML/note.xml"); var_dump($xml); ?>
-
$data = nl2br($_POST['data']);
That doesn't work?
-
So I can understand fully, you want to redirect to a different page while keeping the GET variables? If so, That should be pretty simple.
-
a simple method would be to add this to the top of your first page:
header("Cache-Control: private, max-age=10800, pre-check=10800"); header("Pragma: private"); header("Expires: " . date(DATE_RFC822,strtotime("+1 hour"))); // Change this to how long you are willing to have them go back
-
mod_rewrite doesn't work for creating sub domains, to my knowledge. sub domains require A or CNAME records, or they don't work.
He was almost right. You need a wildcard A record (*.yourdomain.com), then you need to add it to your vhost, then here's an example .htaccess file to get it done:
RewriteEngine on RewriteCond %{HTTP_HOST} ^[^.]+\.example\.com$ RewriteRule !^index\.php$ index.php [L]
-
an easier method would be to add the CentOS testing repo:
cd /etc/yum.repos.d vim CentOS-Testing.repo
copy and paste this into CentOS-Testing.repo:
[c5-testing] name=CentOS-5 Testing baseurl=http://dev.centos.org/centos/$releasever/testing/$basearch/ enabled=1 gpgcheck=0 gpgkey=http://dev.centos.org/centos/RPM-GPG-KEY-CentOS-testing # CentOS-Testing: # !!!! CAUTION !!!! # This repository is a proving grounds for packages on their way to CentOSPlus and CentOS Extras. # They may or may not replace core CentOS packages, and are not guaranteed to function properly. # These packages build and install, but are waiting for feedback from testers as to # functionality and stability. Packages in this repository will come and go during the # development period, so it should not be left enabled or used on production systems without due # consideration.
The testing repo has PHP 5.2.10
-
Try this
EDIT*
We prefer to help people who have code. Try the 3rd party or freelancing section for more help.
-
<shameless plug>
I built an open-source system to send e-mail for you. It's not automated (yet), but it has all the RFC stuff already built in (opt-in/opt-out), multi-user support, and you can modify the code so you can have it grab your users, or I can write a script to import them into my scripts database. Remember, It's open source. Free as in beer and freedom:
http://sourceforge.net/projects/mailgroup/
</shameless plug>
-
I prefer to use fopen, read the data, and make sure there isn't any errors from there.
/* Define the file, so we can use the name to check size of the file in the future. Much faster when dealing with a large number of files */ $file = "/home/myuser/info.txt"; /* Open the file for reading */ $fh = fopen($file, "r"); /* Read the file, in its entirety */ $data = fread($fh, filesize($file)); /* If reading the file failed, or if it is empty, throw an error. If not, move on */ if (!$data || empty($data)){ echo "[-] Error : couldn't read $file"; exit(); } /* do whatever you are planning on doing from here */ /* Close the file */ fclose($fh);
-
This sounds like a javascript issue, and IE is known to have a less than ideal javascript engine. I've been playing with the dev release of IE9, and I gotta say: that problem may be fixed when it comes out.
-
you could do this:
$file = "$current_dir/$filename"; $file = addslashes($file); $md5 = md5_file($file);
This way, you don't need to exec the script to get the md5.
-
What I'm getting from it (without having all the code to work with):
If there's are error messages, and they are in array form, display each one in a unordered list, then unset all errors, so we don't display them again
-
....and made a goof up as well...
-
Oh, and I decided to clean the code up a bit.
<? $Name = "Initiate Touch of Fire"; $query = "SELECT * FROM `Runesred` WHERE `Name`='$Name';" or die('Error Selecting\n<br />'.mysql_error()); $result = mysql_query($query, $connection)or die("Error Results: \n<br />".mysql_error()); while ($res = mysql_fetch_assoc($result)){ $Name2 = $res['Name']; $Image = $res['Image']; $Class = $res['Class']; $Level = $res['Level']; $Damage = $res['Damage']; $Effect = $res['Effect']; $DroppedBy = $res['DropedBy']; $Description = $res['Description']; $Mana = $res['Mana']; echo "<tr><td>$Name2</td><td></td></tr><tr><td>$Image</td><td> </td></tr><tr><td>$Class</td><td> </td></tr><tr><td>$Level</td><td> </td></tr><tr><td> </td><td>$Damage</td></tr><tr><td> </td><td>$Effect</td></tr><tr><td> </td><td>$DroppedBy</td></tr><tr> <td> </td><td>$Description</td></tr><tr><td> </td><td>$Mana</td></tr></table>"; } ?>
-
forgot to put your query in quotes.
-
I didn't modify your code aside from clean it up. Before, your code was hard to read. This should be easier. It's also how most people code (roughly. One of the perks of PHP is that you can "freestyle" your code, and it will still work). [code=php:0] <?php echo '<table class="products-grid" id="products-grid-table">'; $_columnCount = $this->getColumnCount(); $prodsPerRow = 3; $i=0; foreach ($_productCollection as $_product){ $product_image_url = $_product->getProductUrl(); $product_image_title = $this->htmlEscape($_product->getName()); $product_image_tag = $this->htmlEscape($this->getImageLabel($_product, 'small_image')); $product_image = $this->helper('catalog/image')->init($_product, 'small_image')->resize(150, 150); $product_image_alt = $this->htmlEscape($this->getImageLabel($_product, 'small_image')); if($i % $prodsPerRow == 0){ echo "<tr>"; } echo<<<EOF <td> <h3 class="product-name"><a href="$product_image_url" title="$product_image_title">$product_image_title</a></h3> <div class="product-image-box"><a class="product-image" href="$product_image_url" title="$product_image_tag"> <img src="$product_image" width="150" height="150" alt="$product_image_tag" title="$product_image_tag" /></a></div> EOF; if($_product->getRatingSummary()){ echo $this->getReviewsSummaryHtml($_product, 'short'); } echo $this->getPriceHtml($_product, true); if($_product->isSaleable()){ echo '<button type="button" class="button" onclick="setLocation(\''.$this->getAddToCartUrl($_product).'\')"><span><span><span>'.$this->__('Add to Cart').'</span></span></span></button>'; } else{ echo '<p class="availability"><span class="out-of-stock">'.$this->__('Out of stock').' </span> </p>'; } echo '<ul class="add-to-links">'; if ($this->helper('wishlist')->isAllow()){ echo '<li><a class="wishlist-link" href="'.$this->helper('wishlist')->getAddUrl($_product).'">'.$this->__('Add to Wishlist'); } if($_compareUrl=$this->getAddToCompareUrl($_product)){ echo '<li><span class="separator">|</span> <a href="'.$_compareUrl.'">'.$this->__('Add to Compare').'</a></li>'; } echo "</ul> </td>"; if($i+1 % $prodsPerRow == 0 && $i != 0){ echo "</tr> <tr> <td colspan=\"2\" class=\"devider-product-2\"></td> </tr>"; } $i++; }
all errors are still there, but I figured I could show you how to write the code that everybody can easily read.
-
I don't have the javax.servlet code, and I'm not a huge fan of compiling from jar if I don't have to. Basically, from what I've googled, javax.servlet is just a package that makes running http requests easier. Your script appears to be the java equivelent of an AJAX call, minus the call.
Basically, what it says:
#Javascript is the closest thing I can think of function doPost(requestURL, requestString){ var response = new XMLHttpRequest(); if (response.responseText == ""){ alert("empty result!"); } else{ alert(response.responseText); } }
This is hastily written, and missing a bunch, but that's what the servlet is for. It makes it so you don't have to write the complete call yourself.
-
I'm no java expert, but this script just writes HTML, and upon success, it closes the script, right?
Just wanting to make certain what it does. About to run it through Eclipse now, to see if I have the servlet.http, etc.
-
Seeing how PHP works, you will need to use AJAX for this. PHP processes the source file, and outputs the data that is requested, then it's done. It does not pause for client-side inputs. Might want to go to the AJAX section to learn how to make those kinds of calls.
-
you *could* use gmail as your mailer for your php scripts (read how here)
making a web page force a payment before forwarding to the information page
in PHP Coding Help
Posted
You will need to set up 2 parts: A front end that they enter the data, and view the results, if and only if the payment is made, as well as a back-end that will verify the payment, and if it processes it, have it store that in a database so you know to show them the data. AJAX would be best for this. If you had code, I would help further.