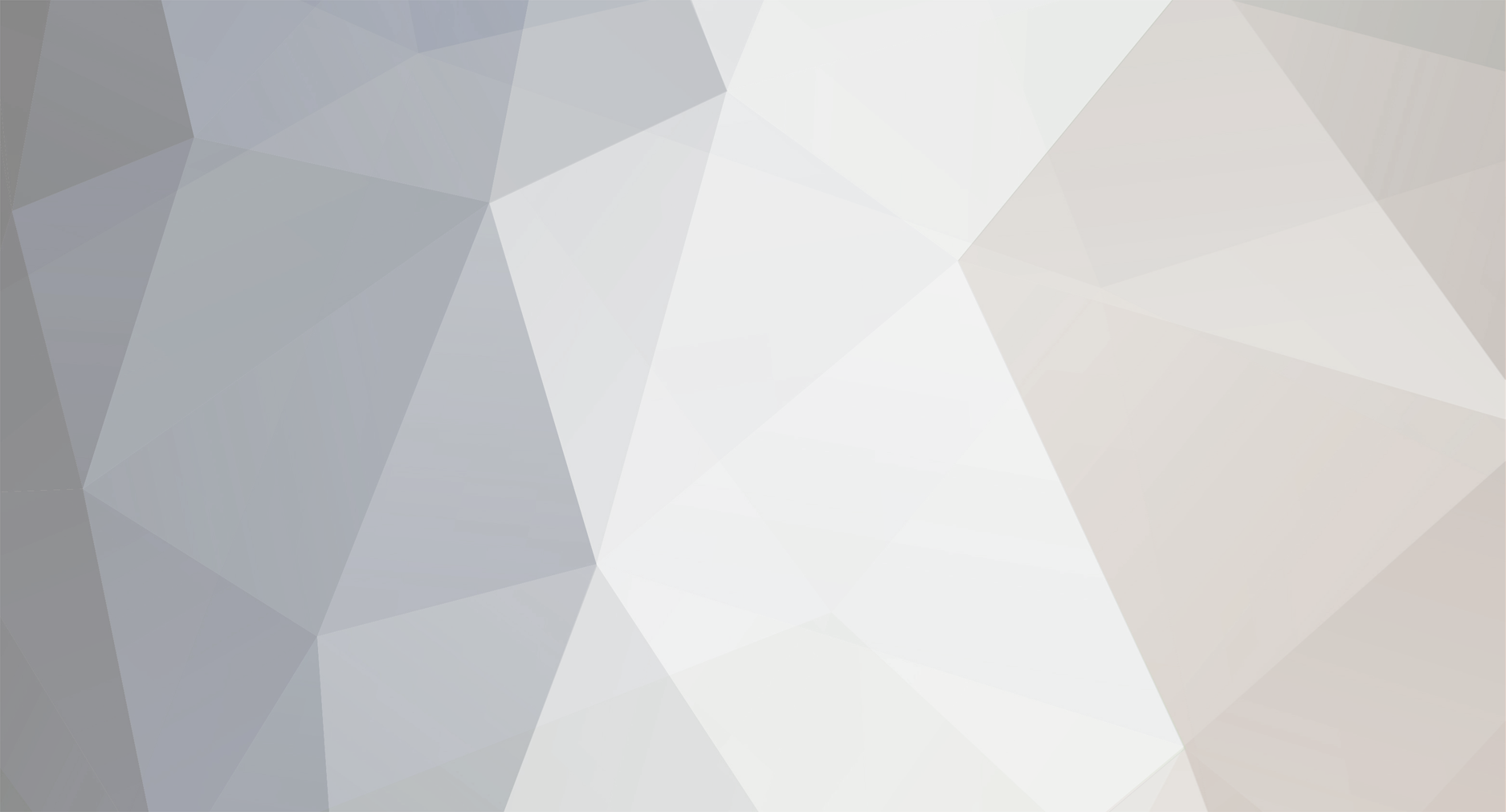
jonsjava
-
Posts
1,579 -
Joined
-
Last visited
Posts posted by jonsjava
-
-
Could you please tell me how my script is obfuscation? I said obfuscation because I wanted to make sure I covered all bases, but now, I realize that it's no way near obfuscation. Each character gets hashed, and it's undecipherable unless you know the salt and generation date to the second. You could supposedly get a rough estimate of what it says, if you put a 100 character encrypted file through a rainbow list for about 3 years or so, but by then, the message shouldn't matter.
-
TODO: write a usage. Sorry. I need to do that.
Here's a brief overview:
to encrypt, you do it as such:
$test_data = "aaaaaaaaaaaaa!@$#///"; $date = "1269639226"; $class = new trucrypt($test_data,"some_salt_goes_here",$date,true); echo $class->return;
to decrypt, you do pretty much the same thing, but only putting the encrypted array in to it:
$test_data = Array(0 => 'a3372b1b5b7f9a51f4f16f2bb0de08b4', 1 => "b928aad727461d2090d7ea4cd8840fcb", 2 => "8a5c80995f3a761e8d172bf8a33158a0", 3 => "35586bd8deb68dff35240fbebaa9e33f", 4 => "fbf5027b9dd8e09e881923dea0acaad6", 5 => "894f5885b146d9391e0d6c8409e7479c", 6 => "c8cc0fc5e05ee6a1c74177481fa55c41", 7 => "f394cdfe38e11127d1cfcb29fa32edce", 8 => "158621523d2133e11b8f41b32f210a20", 9 => "9cce4a1c5d55b5259f3111ca4f21cb05", 10 => "a373afa6210085ef202777ba9391b465", 11 => "8c607b517ce804fd1b7aeca956c8dbcc", 12 => "cb79d3111ce3b197c03330506c89cfd1", 13 => "6d09c1fe8523c19a70286440fb400391", 14 => "7773e559351770c4907ffe727b98f662", 15 => "5c72343aa62983df003acc2a734f721b", 16 => "210cfb2dfc9aee347468b1b8d09cb866", 17 => "1d74930ede0e2e46dbf71413526448de", 18 => "d8f61b3c7bd2fe584c73704d82bef568", 19 => "17b13c8b7354a56ef8955d3f2c3b1fe8"); $date = "1269639226"; $class = new trucrypt($test_data,"some_salt_goes_here",$date,false); echo $class->return;
See how I changed true to false. That determines if it is encrypting or decrypting. Sorry for not explaining the usage.
-
Glad to be of service.
First, create a batch file:
@echo off $PATH="C:\path_to_PHP_exec\"; :loop php your_script_to_execute.php @ping -5 127.0.0.1 > nul goto loop
Next, create the php file to execute the file:
<?php $link = mysql_connect("localhost","some_user","some_pass"); mysql_select_db("some_db",$link); $sql = "SELECT * FROM `commands` WHERE `executed` = 0;"; $res = mysql_query($sql); while ($row = mysql_fetch_assoc($res)){ $command = $row['command']; $id = $row['id']; shell_exec($command); mysql_query("UPDATE `commands` SET `executed`=1 WHERE `id`=$id LIMIT 1;"); } ?>
Now, you need a database that has a table called "commands" and it should have this schema:
id (int) length 5 auto increment primary key
command(varchar) length 255 not null
Now, when someone wants to set up their user, just store the command that will need to be run in the database, and it will be run in the next 5 minutes.
I kinda bashed this script together, because I can't post the one I use. IP and all. Company wouldn't be happy with me if I was to share trade secrets *yawn*
-
Give it a go. I can guarantee it can decrypt it just fine. It re-calculates the hash.
-
I was working on this encryption/obfuscation script, and posted it in the beta test section of the forums. That was 3 days ago, and haven't been approved. Still awaiting moderation. I hate multi-posting, but I could use insight as to what I can do to make it more obfuscated/encrypted.
<?php class trucrypt{ var $data; var $salt; var $date; var $encrypted; var $return; function __construct($data,$salt,$date,$encrypt=true){ $this->salt = $salt; $this->date = $date; if ($encrypt == true){ $this->data = $data; $this->crypt(); } else{ $this->encrypted = $data; $this->decrypt(); } } public function crypt(){ $data = $this->data; $salt = $this->salt; $date = $this->date; $out = array(); if (!is_numeric($date)){ $date = date("U",strtotime($date)); } $data_a = str_split($data); foreach ($data_a as $key=>$val){ $out[$key] = md5($val.$salt.$date.$key); } $this->return = $out; } public function decrypt(){ $encrypted = $this->encrypted; $salt = $this->salt; $date = $this->date; $out = ""; $charmap = array("\n",0,1,2,3,4,5,6,7,8,9,"A","B","C","D","E","F","G","H","I","J","K","L","M","N","O","P","Q","R","S","T","U","V","W","X","Y","Z","!","@","#","$","%","^","&","&","*","(",")","-","_","=","+",";",":","'","\"",",",".","/","\\","|","[","]","{","}","<",">","?","`","~"," ","a","b","c","d","e","f","g","h","i","j","k","l","m","n","o","p","q","r","s","t","u","v","w","x","y","z"); foreach ($encrypted as $key=>$val){ foreach ($charmap as $val2){ $crypt = md5($val2.$salt.$date.$key); if ($crypt == $val){ $out .= $val2; } } } $this->return = $out; } } $date = date("U"); $test = "This is a test I hope it works!"; $da = new trucrypt($test,"cheeseburger",$date,true); $encrypted = $da->return; print_r($encrypted); echo "\n<br /><br /><br />\n\n\n"; $da2 = new trucrypt($encrypted,"cheeseburger",$date,false); echo $da2->return; ?>
-
Here's a cleaned up version of your code.
<?php include ('config.php'); session_start(); $username = $_POST['username']; $password = $_POST['password']; if ($username && $password) { $username = mysql_real_escape_string($username); $password = mysql_real_escape_string($password); $query = mysql_query("SELECT * FROM `users` WHERE `username`='$username' LIMIT 1;"); $numrows = mysql_num_rows($query); if ($numrows!=0) { //code to login while ($row = mysql_fetch_assoc($query)) { $dbpassword = $row['password']; } //check to see if they match! if ($password==$dbpassword) { $_SESSION['username'] == $username; header("location:member.php"); exit(); } else{ echo "Wrong password!"; } } else{ echo $numrows; die ("User not found!"); } } else die ("Write your username and password!"); ?>
I changed
if ($numrows !=0) (
to
if ($numrows !=0) {
removed the semicolon at the end of the while line
fixed the indentation, bracketed the else statements, removed the check to see if $user=$dbuser, because the query would have come up empty if it didn't.
I also sanitized the user input so you aren't susceptible to injection attacks, cleaned up the query, so it only checks for one result, so it will run faster. I'm thinking I did more, but I lost track (boss came in, and I got side-tracked)
-
forgot to mention, I have a database that stores next commands to execute, and that's what the php script runs. This way, you set up a front-end, it posts data to the DB, the script kicks every so often, finds un-run scripts, and runs them.
-
I have a batch file that runs a PHP script with $PATH set so it can see the php-cgi. I then run that batch file in an infinate loop with 5 second sleeps and have it serviced as running as administrator. If you need help/pointers, let me know.
-
Thanks for the heads-up. I'll just post it in the help section for review for now.
-
but I cannot stand someone trying to make a fool out of me
thats why I lost it, I don't think anyone on here would want that.
Guess again. We don't care if you "lose it". We're volunteers here. We don't get paid a cent for helping. If you get an attitude, there are hundreds of other people who need our help, and will show a grain of respect. Have a nice day.
-
might be better to store dates in unix timetamp. Easier to calculate.
-
I posted a beta script to test, and it's been awaiting approval for a while now (3 days). I was wondering if anybody is checking for new posts to moderate, or if I should give up on that.
[attachment deleted by admin]
-
forgot to tell it how to order (ascending, descending)
$sql = mysql_query("SELECT * FROM `records` WHERE `read` < 1 ORDER BY `added` ASC;") or die("ERROR, Will Robinson! Here it is:\n".mysql_error());
-
try this:
<?php $n = 0; $fh = @fopen('count.txt', 'r+') or die('Error in opening file for read & write'); $n = fread($fh,filesize('count.txt')); if ($n < 3) { ftruncate($fh, 0); @fwrite($fh, ++$n) or die ('Unable to write to file!'); } else { print "Limit Reached\n"; } fclose($fh); ?>
Glad to see you work your way through it!
Oh, and you need to give the size of the file for it to open (length).
-
You weren't posting the data. You were having them click on a link to go to the post-process file. Try this:
<form id='order' name='order' method='post' action='members/order.php'> <input type='hidden' name='itemid' id='itemid' value='".$info['item_id']."'> <input type='hidden' name='status' id='status' value='Unconfirmed'> <input type='hidden' name='payment' id='payment' value='Not received'> <imput type='image' src='../img/buynow.jpg' value='Submit' alt='Submit'></a> </form>
-
I'm not sure what you are asking
Are you asking how you can convert the IP into a long, so it's easier to sort in the database?
$ip = $_SERVER['REMOTE_ADDR']; $long = ip2long[$ip];
-
try something like this:
$root = getenv("DOCUMENT_ROOT"); $inc = "/inc/some_file.php"; include($root.$inc);
just make sure that $inc is relative to $root
$root will show where your HTDOC root is (the root of your site)
-
Array ( [1] => 100 [11] => 90 [100] => 30 [200] => 20 [400] => 10 [1000] => 5 )
How do you calculate that 4 will be 94, and 1000 will be 5? Is there a way to calculate it? That's the problem. I don't see a pattern.
-
Don't worry. I don't mind the questions. The fact you are asking the right questions says a lot about your intelligence (a compliment, btw)
Ok, $_SERVER are the server environment variables. Think of it this way:
In Windows, you have environment variables. Don't believe me? well, check this out:
Go to Start-->Right click on My Computer-->Properties
If you are like me, and running windows 7, you would then click on "Advanced System Settings" on the left. Windows XP, you go to "Advanced", I think.
Click on "Environment Variables..."
All systems have enviousness variables. It allows the system to know how much RAM to allocate, the path to common applications, and more.
Well, most well-built applications have the same, so you can configure them yourself. Apache has quite a few. They tell the server what user to run the application/script as, where to store temp files, where to store session data, and more. By setting $_SERVER['PHP_AUTH_USER'], it is telling Apache to set the REMOTE_USER to the user who has authenticated with the given credentials via PHP. I'm mucking it up, but I'll gladly answer any other questions this causes you to pose.
-
Cookies and sessions are what your browser sends back to the server so it knows which session you are working from. All the actual data for the sessions and cookies are stored on the server, usually in the /tmp folder (if linux, and they have enough ram for that)
-
That would only work if you had a set formula to work off of. If each set will have a different formula, then writing a static function to calculate possible results would be near impossible. The best route would be to write a function that will take in 2 inputs: the array, and the formula to calculate the missing portions of the array.
-
might want to look at PHP_AUTH
You would do something like this:
<?php session_start(); if (isset($_SESSION['username']) && isset($_SESSION['password']) && isset($_SESSION['is_valid']) && $_SESSION['is_valid'] == true){ $_SERVER['PHP_AUTH_USER'] = $_SESSION['username']; $_SERVER['PHP_AUTH_PW'] = $_SESSION['password']; }
This isn't well thought out code, but it should get you on the right path.
-
you can use the same variable numerous times, but remember: every time you re-declare a variable, it loses it's old values. Set a unique variable to hold each result set.
-
if DNS is failing, it's not Apache. Most likely it's one of 2 things: cached DNS result, or bad/corrupt entry on your NS's
Lots of tables, But no longer access to Database?
in PHP Coding Help
Posted
Unfortunately, you will need to know the basics of how databases work, the best types to use for what kind of data, max length needed to handle the data, etc. Next, you would need to parse through the code and find all references to results from MySQL, as well as all sql queries. With that knowledge, and the skillset described, you should be able to reconstruct it.