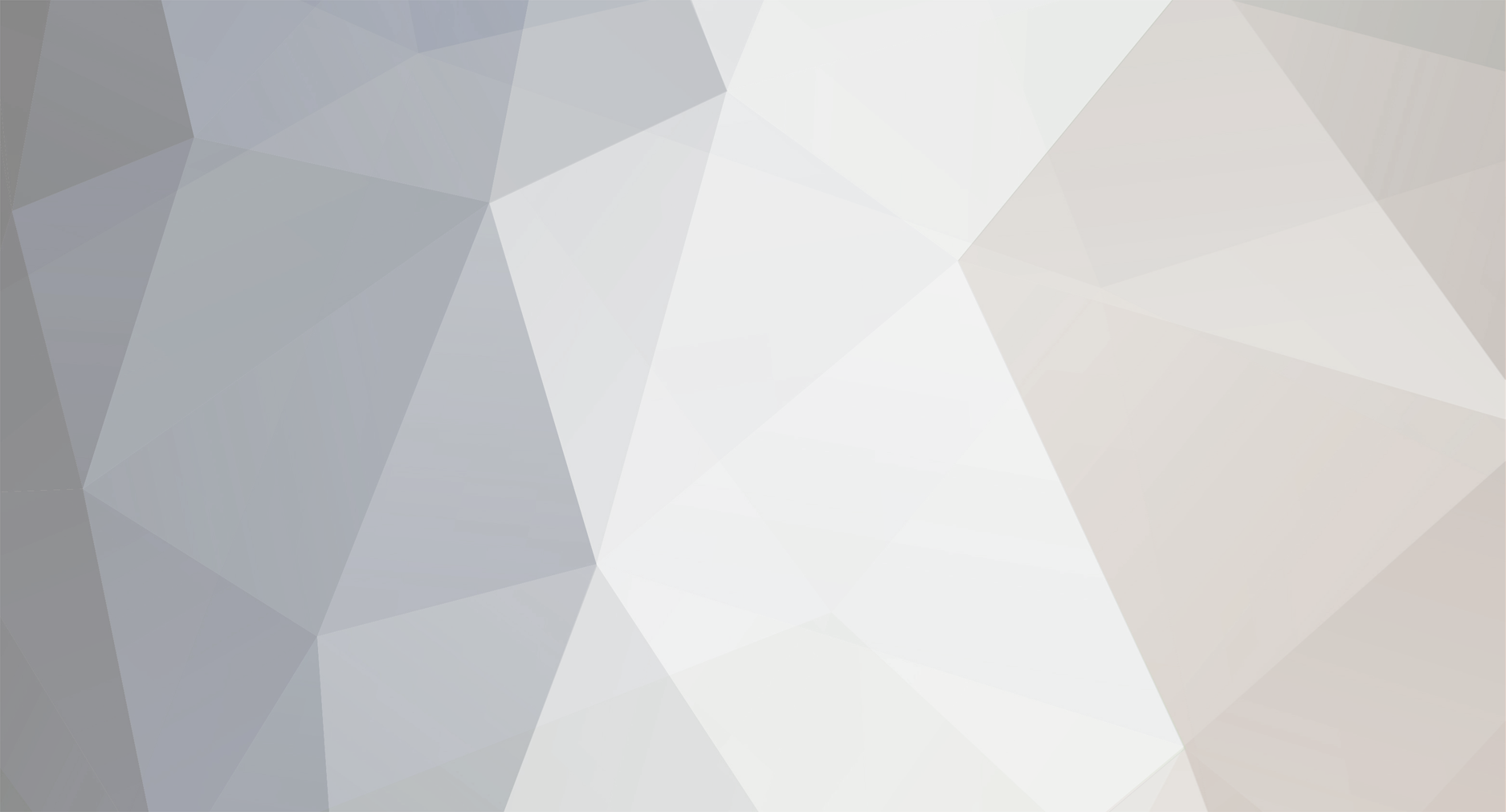
hansford
Members-
Posts
562 -
Joined
-
Last visited
-
Days Won
4
Everything posted by hansford
-
PHP and MYSQL join tables and select users id in both tables.
hansford replied to blmg2009's topic in PHP Coding Help
It seems that you are limiting your selection by using the distinct keyword SELECT DISTINCT(td.team_id) -
Here is how object references can be saved during serialization. <?php error_reporting(E_ALL); ini_set('display_errors',1); define('br','<br />'); class RegistryClass { private $data; private $regList = array(); public function set($name,$value) { $this->regList[$name] = $value; } public function getData() { return $this->regList; } } class RegList { private $name; public function __construct($name) { $this->name = $name; } public function getName() { return $this->name; } } $reg = new RegistryClass(); $a = new RegList("fred"); $reg->set('a',$a); $b = new RegList("jane"); $reg->set('b',$b); $c = serialize($reg); // doesn't work as references to other objects are lost //we can save the Registry object and the referenced objects in an array $c = array(); $c[0] = $reg; foreach($reg->getData() as $key => $value) { $c[1][$key] = $value; } // now serialize the array $d = serialize($c); // everything is saved // un-serialize the array $e = unserialize($d); // retrieve our Registry object $reg = $e[0]; // now add the instances back into the Registry object foreach($e[1] as $key => $value) { $reg->set($key,$value); } // test it out $instance = $reg->getData(); foreach($instance as $key => $value) { echo $key . ': ' . $value->getName() . br; }
- 5 replies
-
- 1
-
-
- serializable
- singleton
-
(and 2 more)
Tagged with:
-
That regex is not going to work. Lay out in detail what you expect this class to do.
-
Make changes as needed, but this will give you what you indicated. <!doctype html> <html> <head> <style type="text/css"> .white-text { display:block; color: #ffffff; } .black-text { display:block; color: #000000; } .white { width:50px; height:50px; background-color: #ffffff; } .black { width:50px; height:50px; background-color: #173534; } .green { width:50px; height:50px; background-color: #247422; } .pee-green { width:50px; height:50px; background-color: #A3BC34; } .gray { width:50px; height:50px; background-color: #AABBCC; } .dark-blue { width:50px; height:50px; background-color: #1351AC; } .lt-green { width:50px; height:50px; background-color: #547142; } .red { width:50px; height:50px; background-color: #AD2431; } .teal { width:50px; height:50px; background-color: #113344; } .purple { width:50px; height:50px; background-color: #431241; } </style> </head> <body> <table border=1> <?php $colors = array(array('white','FFFFFF'),array('black','173534'), array('green','247422'),array('pee-green','A3BC34'),array('gray','AABBCC'), array('dark-blue','1351AC'),array('lt-green','547142'),array('red','AD2431'), array('teal','113344'),array('purple','431241')); for($i = 0; $i < 10; $i++) { echo "<tr>"; for($j = 0; $j < 10; $j++) { $rand = rand(0,9); echo "<td class='" . $colors[$rand][0] . "'> <span class='black-text'>{$colors[$rand][1]}</span> <span class='white-text'>{$colors[$rand][1]}</span> </td>"; } echo "</tr>"; } ?> </table> </body> </html>
-
Here is one way of accomplishing the task. You don't need the two arrays as you could just have an array of 2 values and randomly select a 0 or 1 for each square. <!doctype html> <html> <head> <style type="text/css"> .white { width:50px; background-color: #ffffff; } .black { width:50px; height:50px; background-color: #000000; } </style> </head> <body> <table border=1> <?php $white = array_fill(0,10,'white'); $black = array_fill(0,10,'black'); $colors = array_merge($white,$black); shuffle($colors); //$colors = array('white','black'); for($i = 0; $i < 10; $i++) { echo "<tr>"; for($j = 0; $j < 10; $j++) { echo "<td class='" . $colors[rand(0,19)] . "'></td>"; } echo "</tr>"; } ?> </table> </body>
-
You need to break this problem down into simple steps. Get rid of that try/catch block. Work on a single file outside of your class/function and attempt to make a connection and query data from your database. Once you get that working, then move the parts over to your class and methods. <?php error_reporting(E_ALL); ini_set('display_errors',1); $tablename = 'table_name'; $myvalue = 'what_to_search_for'; $conn = new PDO("mysql:host=localhost;dbname=$dbname",$user,$pass); $conn->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION); $myquery = "SELECT id, company_name, company_reg_number, vat_number, website FROM $tablename where company_name like ? ORDER BY company_name ASC"; $myvalue = "%". $myvalue ."%"; $mystmt = $this->conn->prepare( $myquery ); $mystmt->bindParam(1, $myvalue, PDO::PARAM_STR); $mystmt->execute(); $myresult = $mystmt->fetch(PDO::FETCH_ASSOC); print_r($myresult);
-
Apologies. I've added comments to the code. function readLike( $searchval ){ // altered the SELECT statement to embed $this->table_name directly into the double quoted string // It is not necessary to concatenate variables and strings when using double quotes. $myquery = "SELECT id, company_name, company_reg_number, vat_number, website FROM $this->table_name where company_name like ? ORDER BY company_name ASC"; $searchval = "%".$searchval."%"; // removed single quotes from string as they are being included in the search term $mystmt = $this->conn->prepare( $myquery ); $mystmt->bindParam(1, $searchval, PDO::PARAM_STR); $mystmt->execute(); $myresult = $mystmt->fetch(PDO::FETCH_ASSOC); return $myresult; }
-
It's all outlined in the Manual. Read this: http://php.net/manual/en/faq.passwords.php
-
function readLike( $searchval ){ $myquery = "SELECT id, company_name, company_reg_number, vat_number, website FROM $this->table_name where company_name like ? ORDER BY company_name ASC"; $searchval = "%".$searchval."%"; $mystmt = $this->conn->prepare( $myquery ); $mystmt->bindParam(1, $searchval, PDO::PARAM_STR); $mystmt->execute(); $myresult = $mystmt->fetch(PDO::FETCH_ASSOC); return $myresult; }
-
There are a many problems with this page. Md5 should not be used for password hashing. Here is a quick fix for what you are attempting however. $user = $_POST['username']; $pswd = $_POST['password']; $repeat_pswd = $_POST['repeatpassword']; if ($user == '' || $pass == '' || $repeat_pswd == '') { ?><script>alert("Sorry username or password,repeatpassword is empty!!");</script> <?php } elseif(($pswd != '' && $repeat_pswd != '') && ($pswd != $repeat_pswd)) { ?><script>alert("Password fields do not match");</script> <?php } ?>
-
How to display random results within selection
hansford replied to mcmuney's topic in PHP Coding Help
You can try this, untested. There may be more robust ways of doing this. See if others chime in. "SELECT mem_id,type_id,date_added FROM(SELECT value FROM sc_coins_asset WHERE value<>'0' ORDER BY RAND() DESC LIMIT 0,15) u ORDER BY date_added" -
It doesn't appear the class is being loaded and an object generated. Is the SteamUser class file named according to the frameworks' guidelines. Is it located in the main libraries folder or the application/libraries folder. If it is located in a sub-directory folder you'll need to add that path to the class loader. $this->load->library('subdirectory/SteamUser', array('userId' => $member['steamId']));
-
how i can getting xpath address of a string in html code?
hansford replied to mikhak's topic in PHP Coding Help
$html ="<div><div> <div><div> <div> <span>YES</span> <div> <p>b text</p> </div> </div> <div> <p>NO</p> <div> <p>a text</p> </div> </div> </div> <div> <div> <p>YES</p> <div> <p>b text</p> </div> </div> </div></div> </div></div>"; $dom = new DOMDocument(); $dom->loadHTML($html); $xpath = new DOMXPath($dom); foreach($xpath->query('//*[.="YES"]') as $node) { echo $node->getNodePath() . '<br />'; } -
Put all of that code between a single pair of php tags. Place the opening tag at the beginning of the file.
-
Can you post what the city.xml file looks like to see if there are any traversing issues.
-
No need for the multiple submits. When the user selects a date, trigger an ajax event to retrieve the data for available times to display.
-
This should be noted: the following code is meant as an example for how to query a database using trusted variable data. If the variables you are embedding in the sql string are from forms or other sources, then this data needs to be sanitized first and the code modified to use prepared statements. The mysqli way: $link = new mysqli('localhost', 'xxx_admin', 'xxxxxx','xxx_database1'); if($link->connect_errno) { echo 'Database connection failed: ' . $link->connect_error; exit(); } if( ! $link->set_charset('utf8')) { echo 'Error loading character set: ' . $link->error; } $sql = "SELECT $rowname FROM $tablename WHERE $finder='$findervalue' LIMIT 1"; if( ! $result = $link->query($sql)) { echo 'No results returned from query'; exit(); } The PDO way: try { $link = new PDO('mysql:host=localhost;dbname=xxx_database1','xxx_admin','xxxxxx', array(PDO::MYSQL_ATTR_INIT_COMMAND => 'SET NAMES utf8')); $con->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION); } catch(PDOExecption $e) { echo $e->getMessage(); exit(); } $sql = "SELECT $rowname FROM $tablename WHERE $finder='$findervalue' LIMIT 1"; $result = $link->query($sql); $row = $result->fetch(PDO::FETCH_ASSOC);
-
As Barand stated, the structures of the table need to be identical in order to use the insert and select combination. If there is a chance that you will bring these deleted rows back to life in the future then I believe Ch0cu3r suggestion of just adding another column which marks the row as "deleted" sounds more reasonable.
-
$datapoints = array(7,3,11,8,2,10,9,6,7,; $avg_1 = array_splice($datapoints, 0,3); $avg_2 = array_splice($datapoints, 0,3); $avg_3 = array_splice($datapoints, 0, count($datapoints)); $average = array($avg_1,$avg_2,$avg_3); $final_array = array(); foreach($average as $arg) { $total = 0; $total_units = count($arg); $min = 1000000; $max = 0; $average = 0; foreach($arg as $unit) { if($unit < $min) { $min = $unit; } if($unit > $max) { $max = $unit; } $total += $unit; } $average = round($total / $total_units); $final_array[] = array('Pool Size'=>$total,'Max'=>$max,'Min'=>$min,'Average'=>$average); } print_r($final_array);
-
I messed up the previous error handling...should be: $db = new mysqli('localhost',$username,$password,$dbname); if($db->connect_errno) { echo $db->connect_errno . ': ' . $db->connect_error; exit(); } $sql = "SELECT product_name,product_code,price,details FROM table_name"; if($result = $db->query($sql)) { while($row = $result->fetch_assoc()) { echo "<span class='class_name'>{$row['product_code']}</span>"; echo "<span class='class_name'>{$row['product_name']}</span>"; echo "<span class='class_name'>{$row['price']}</span>"; echo "<span class='class_name'>{$row['details']}</span>"; } } else { echo 'No results returned from database'; }
-
This will get you started. You'll need to make adjustments depending if you just want to return a specific row or all rows. And format the database output. <?php error_reporting(E_ALL); ini_set('display_errors',1); define('br','<br />'); $db = new mysqli('localhost',$username,$password,$dbname); if( !$db) { echo $db->errno . ': ' . $db->error . br; } $sql = "SELECT product_name,product_code,price,details FROM table_name"; if($result = $db->query($sql)) { while($row = $result->fetch_assoc()) { echo "<span class='class_name'>{$row['product_code']}</span>"; echo "<span class='class_name'>{$row['product_name']}</span>"; echo "<span class='class_name'>{$row['price']}</span>"; echo "<span class='class_name'>{$row['details']}</span>"; } }
-
I'm probably missing something, but why do you need 2 queries to get the data you require. $sql1="SELECT DISTINCT filename,gallery FROM photos WHERE user='{$_SESSION['uname']}' ORDER BY RAND() LIMIT 1";
-
You have created a connection to the database. Now, you have to run a query for anything to happen. What is it you are trying to accomplish ?
-
include('config.inc.php'); // would need error handling if production code // look up mysqli prepared statements $db = new mysqli(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME); $query = "INSERT INTO table_name(field_1,field_2,field_3) VALUES(?,?,?)"; $stmt = $db->prepare($query); $stmt->bind_param('iii',$_POST['field_1'],$_POST['field_2'],$_POST['field_3']); $stmt->execute();
-
You are looping through the array using an array expression. You can loop through the array a number of different ways, but foreach is convenient for many jobs. $fruits = array('apples', 'bananas', 'oranges'); foreach ($fruits as $fruit) { // $fruit initially points to the first element in the array // after each loop is complete the array pointer is advanced to point to the next element in the array } This -> is the object operator. It is used to access properties and methods of an object instance. class Foo { private $name; public function __construct( $value ) { $this->name = $value; } public function getName() { return $this->name; } } $foo = new Foo('MyFoo'); // create an object of class Foo and assign it to the variable $foo echo $foo->getName(); // call a method of our object