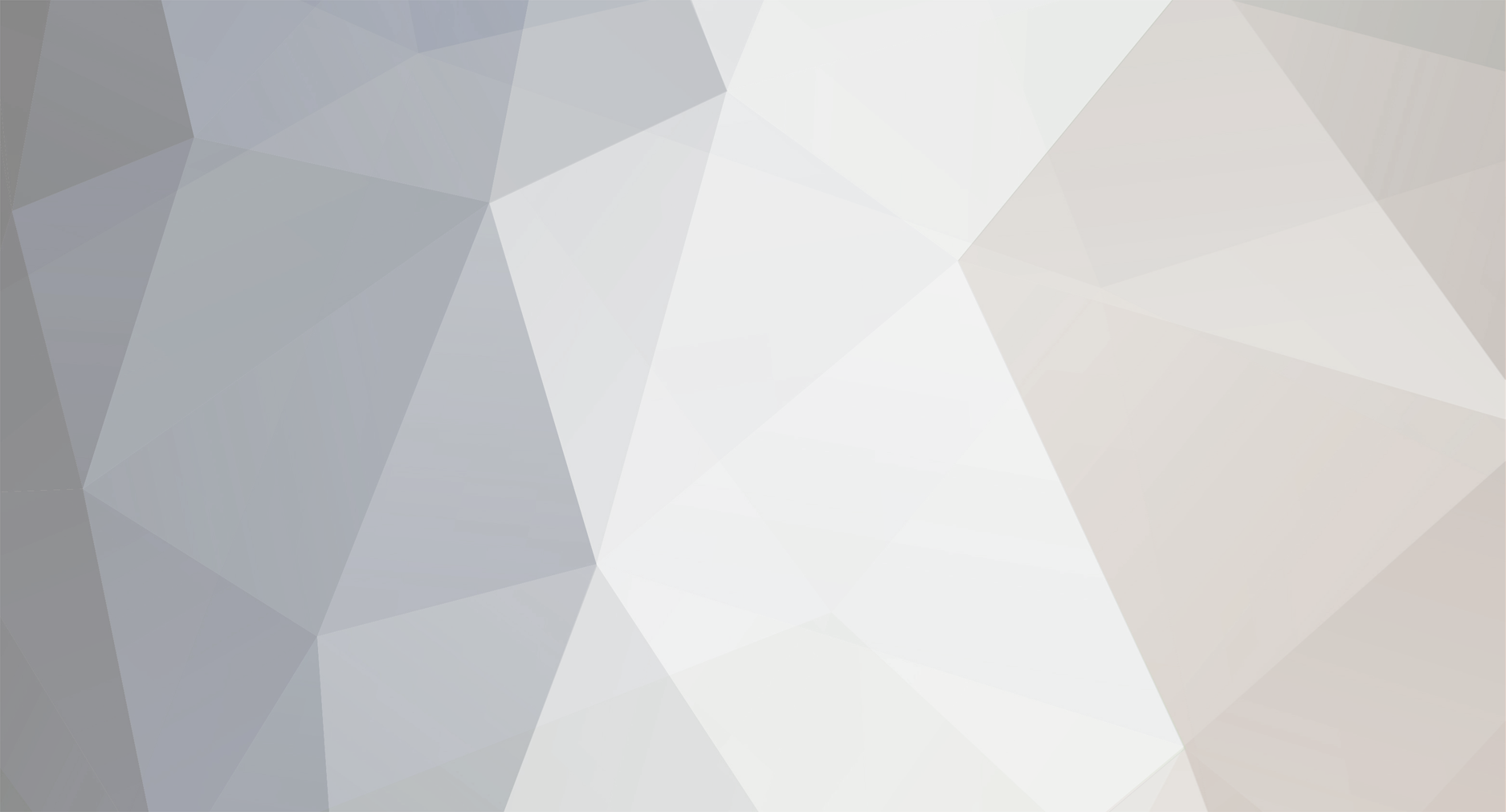
hansford
Members-
Posts
562 -
Joined
-
Last visited
-
Days Won
4
Everything posted by hansford
-
That is why if the code is going to be used over and over...they need to create the file with the headers and then just simply append each row of new data to the file. At some point they are going to have to think for themselves and figure out these trivial things.
-
Well, the code is based on their form so no need for multiple rows. Also, If they expect multiple form submissions then they need to change the "wb" flag to "a" or "ab" or else the file is going to be overwritten each time. They will also create the file along with the headers and simply open the file and append a new data row each time.
-
<html> <head> </head> <body> <form action="basicform.php" method="post"> First name:<br> <input type="text" name="firstname"> <br> Last name:<br> <input type="text" name="lastname"> <br><br> <input type="radio" name="sex" value="male" checked> Male <br> <input type="radio" name="sex" value="female"> Female <br><br> <input type="checkbox" name="box1" value="1"> #1 option <br> <input type="checkbox" name="box2" value="2"> #2 option <br><br> <input type="submit" value="Submit"> </form> </body> </html> basicform.php <?php error_reporting(E_ALL); ini_set('display_errors',1); // set path to file $path = __DIR__ . '\file.csv'; // open the file if it exists, if not create it if(($fp = fopen($path, 'wb')) === false) { exit('failed to open file'); } $posts = array(); if( ! empty($_POST['firstname'])) { $posts['firstname'] = $_POST['firstname']; } if( ! empty($_POST['lastname'])) { $posts['lastname'] = $_POST['lastname']; } if( ! empty($_POST['sex'])) { $posts['sex'] = $_POST['sex']; } if( ! empty($_POST['box1'])) { $posts['box1'] = $_POST['box1']; } if( ! empty($_POST['box2'])) { $posts['box2'] = $_POST['box2']; } // write the header row fputcsv($fp,array_keys($posts),','); // write a data row fputcsv($fp, array_values($posts)); fclose($fp);
-
Skinning a cat (Or how many ways to output "Hello World!")
hansford replied to benanamen's topic in Miscellaneous
You guys are really bored, but ah well. Here is my addition. print_r("hello world"); exec(print "hello world"); foreach(array('h','e','l','l','o',' ', 'w','o','r','l','d') as $letter) { echo $letter; } -
$fun = array( 'First Word' => 'Programming', 'Second Word' => 'in', 'Third Word' => 'PHP', 'Fourth Word' => 'is', 'Fifth Word' => 'fun!', '' => 'Programming in PHP is fun!' ); foreach($fun as $key => $val) { if($key == '') { echo $val; } else { echo "$key, $val"; } echo '<br />'; }
-
Typically a user logs in with their username and password and then a session variable is set which identifies the user. No need to place passwords in a session variable. There is only going to be one user with that username.
-
I've already addressed this in your last post. http://forums.phpfreaks.com/topic/298419-what-am-i-doing-wrong/
-
It doesn't even appear you need output buffering. I ran a mock-up of your code using the following and it works fine. //get_additional is a custom funcion $times = get_additional($tcgname,'Trades'); // this should be returning an integer value echo "<div class='tboxout' style='background: url($tcgimg);'>"; echo '<div class="tboxin">'; $imgurl = 'http://rheanna.magical-me.net/cosmos/cosmoscards/check.png'; for($i=0;$i<$times;$i++){ echo '<img src="'.$imgurl.'" />'; } echo '</div>'; echo '</div>';
-
Let's examine what the code is doing. //get_additional is a custom funcion ob_start(); // start output buffering echo get_additional($tcgname,'Trades'); echo nl2br("\n"); echo '<div class="tboxout" background='$tcgimg'>'; echo '<div class="tboxin">'; $buffered = ob_get_clean(); // get contents of output buffer echo $buffered; // echo contents $times = $buffered; // assign $times the contents of output buffer $imgurl = 'myimage.png'; // this never runs as $times in not a digit higher than 1 // $times was assigned the contents of the output buffer for($i=0;$i<$times;$i++){ echo '<img src="'.$imgurl.'" />'; }
-
Quick question regarding passing html form input values to jquery
hansford replied to mannyson's topic in Javascript Help
You need to change your code to actually use the date which was passed in. eventDate = Date.parse(settings.date) / 1e3; -
Quick question regarding passing html form input values to jquery
hansford replied to mannyson's topic in Javascript Help
Here is you code with with the addition of options. <script type="text/javascript"> (function(e) { $.fn.countdown = function(t,n,options) { var settings = $.extend({ date: 'October 2 2015', format: 'off', }, options); //you can access any option using: settings.date, settings.format etc //begin your code here function i() { eventDate = Date.parse(r.date) / 1e3; currentDate = Math.floor(e.now() / 1e3); console.log(currentDate); if (eventDate <= currentDate) { n.call(this); clearInterval(interval) } seconds = eventDate - currentDate; days = Math.floor(seconds / 86400); seconds -= days * 60 * 60 * 24; hours = Math.floor(seconds / 3600); seconds -= hours * 60 * 60; minutes = Math.floor(seconds / 60); seconds -= minutes * 60; days == 1 ? thisEl.find(".timeRefDays").text("day") : thisEl.find(".timeRefDays").text("days"); hours == 1 ? thisEl.find(".timeRefHours").text("hour") : thisEl.find(".timeRefHours").text("hours"); minutes == 1 ? thisEl.find(".timeRefMinutes").text("minute") : thisEl.find(".timeRefMinutes").text("minutes"); seconds == 1 ? thisEl.find(".timeRefSeconds").text("second") : thisEl.find(".timeRefSeconds").text("seconds"); if (r["format"] == "on") { days = String(days).length >= 2 ? days : "0" + days; hours = String(hours).length >= 2 ? hours : "0" + hours; minutes = String(minutes).length >= 2 ? minutes : "0" + minutes; seconds = String(seconds).length >= 2 ? seconds : "0" + seconds } if (!isNaN(eventDate)) { thisEl.find(".days").text(days); thisEl.find(".hours").text(hours); thisEl.find(".minutes").text(minutes); thisEl.find(".seconds").text(seconds) } else { alert("Invalid date. Example: 30 Tuesday 2013 15:50:00"); clearInterval(interval) } } var thisEl = e(this); var r = { date: null, format: null }; t && e.extend(r, t); i(); interval = setInterval(i, 1e3); } })( jQuery ); $(document).ready(function () { function e() { var e = new Date; e.setDate(e.getDate() + 60); dd = e.getDate(); mm = e.getMonth() + 1; y = e.getFullYear(); futureFormattedDate = mm + "/" + dd + "/" + y; return futureFormattedDate; } var newdate = $('.new-date').val(); $(".countdown").countdown({date: newdate, format: "on"}); }); </script> -
loading image during ajax process not working properly
hansford replied to EHTISHAM's topic in Javascript Help
I don't see the html associated with the jQuery which would be helpful. Anyways... 'bind' is not the preferred method for attaching events, it's 'on'. You attach the submit event on the form, not a button and there is a shortcut for doing that. $("#id-of-form").submit(function(e) { //stop the default form submission e.preventDefault(); //....rest of the code }); If your ajax returns fast then you might miss the loading image as it did not have enough time to load before being hid again. Don't hide the loading image and see if it displays. If it does, then you know it's working and the ajax speed is the issue. -
Quick question regarding passing html form input values to jquery
hansford replied to mannyson's topic in Javascript Help
Just add some option settings. You can set some defaults then override them by passing in the options when calling the function on the element. <script type="text/javascript"> (function ( $ ) { $.fn.countdown = function(options) { var settings = $.extend({ date: 'October 2 2015', format: 'off' }, options); } }( jQuery )); var newdate = "November 2, 2015"; $(".countdown").countdown({date: newdate, format: 'on'}); </script> -
Image upload/convert/resize and add to mysql problem
hansford replied to cobusbo's topic in PHP Coding Help
If the file is actually a png and the user renames it with a jpg/jpeg.....this should not be allowed on the part of the user. Storing the filename in the database should be secondary to storing the filename on the server. If the user is able to change the filename then there is obviously a flaw in the code. -
Calculate result based on separate total costs displayed
hansford replied to imkesaurus's topic in PHP Coding Help
If that is the case, then it would be impossible to determine C without the known costs of both A and B. If you know the costs of A or B when the user selects either one of those values then you, as Jacques1 stated, call one function which calculates C based on the return values of calculate_transfer_costs() and calculate_bond_costs() -
Should look something like the following. display_errors = On ; Default Value: On ; Development Value: On ; Production Value: Off Optionally, at the top of each php page you can set error handling with the following: error_reporting(E_ALL); ini_set('display_errors', 1);
-
You shouldn't be storing files in a database. Databases should store data and file systems should store files. Move the uploaded file into a directory and then place the location information to that file in the database. If you are going to be storing files in the database then you need to store it as type Blob and then you will have to write that data to a file with the correct extension for display purposes - which makes the whole operation pointless to store the file in the database in the first place.
-
Well, if it's not in use now, just place a default value for that variable. The default will get over ridden later on when you pass the variable in. function getID($staffid, $id=false)
-
I don't understand why you need the $id variable as you don't seem to use it. The only id variable I see you use is the $staffid $arg = getID($staffid); if(is_array($arg)) { $status = "<font color='green'>Punched</font>"; echo $arg[0]; //$id echo $arg[1]; //$first_name } else { $status = "<font color='red'>Invalid ID, try again</font>"; } Change the arguments in this function to this: function getID($staffid){
-
Pass the id variable to the function. You can also return multiple variables using an array. function getID($staffid, $id){ return array($id,$first_name);
-
Fatal error: Call to undefined function: filter_input()
hansford replied to keaauBoy's topic in PHP Coding Help
What requinix is referring to is one of these 2 functions as either one will return the php version you are using. echo phpversion(); echo phpinfo(); -
Here is all of what Ch0cu3r stated. // get array of results from the query $result = $Game->getAll(); // loop over array of results using foreach loop foreach($result as $row) { echo $row['name'] . '<br />'; } class Game { public $ID = ""; public $Name =""; public function getAll(){ $sql = "SELECT * FROM TBL_Game"; $query = $this->db->prepare($sql); $query->execute(); return $query->fetchAll(); } }
-
So, based on what Ch0cu3r stated, your function should look like this. public function getAll(){ $sql = "SELECT * FROM TBL_Game"; $query = $this->db->prepare($sql); $query->execute(); $result = $query->fetchAll(); return $result; }
-
Can use a function when defining a protected class variable
hansford replied to whitburn's topic in PHP Coding Help
Class properties can only be assigned a constant value and cannot rely on run-time evaluations. So, no returning values from functions.