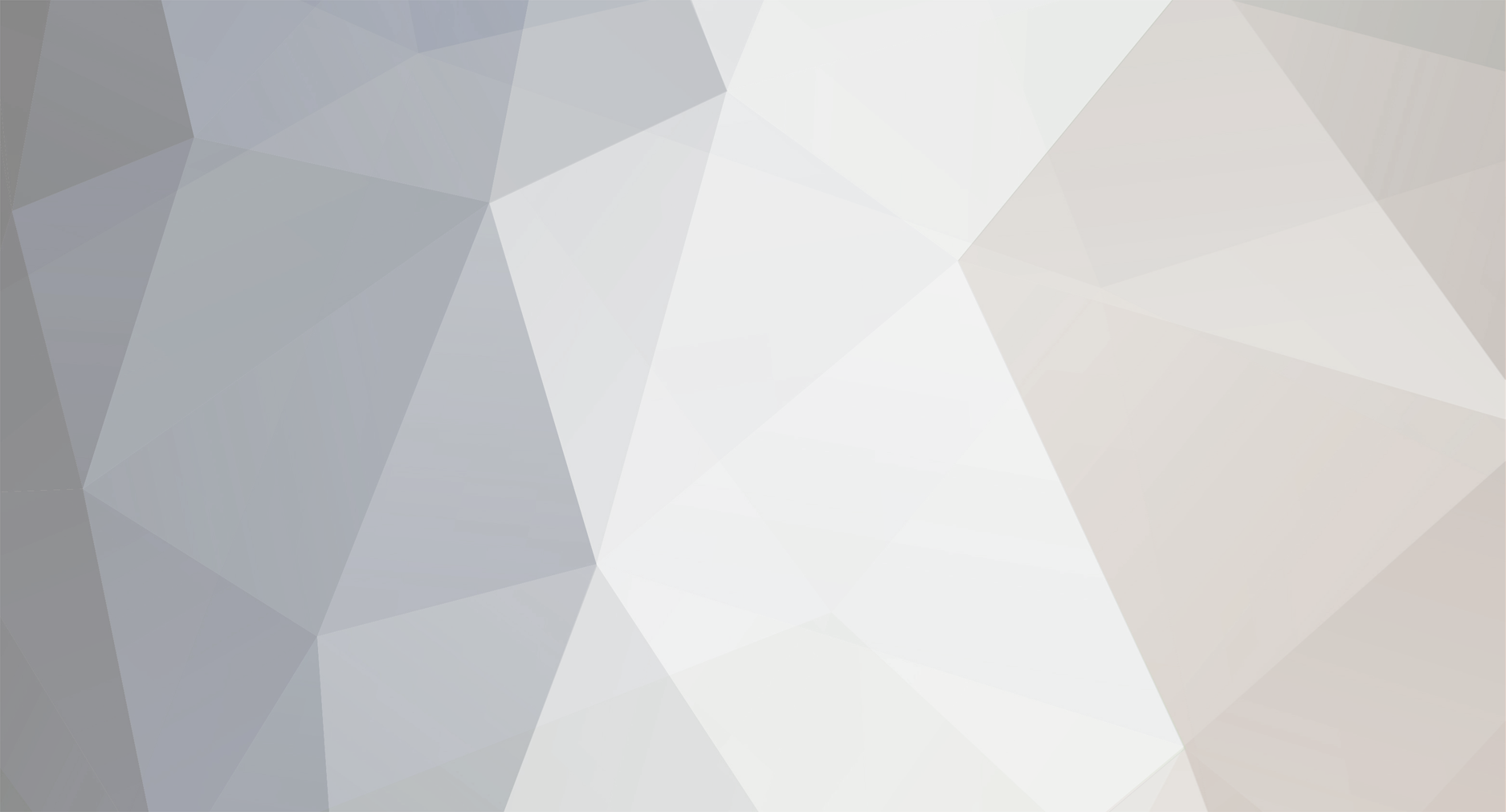
Pikachu2000
Staff Alumni-
Posts
11,377 -
Joined
-
Last visited
-
Days Won
11
Everything posted by Pikachu2000
-
The error is pretty self-explanatory. You can't send anything to the browser, not even whitespace, before sending headers. Something on line 25 is outputting to the browser, and setcookie() comes after line 25, correct?
-
You should really get in the habit of separating your query strings from the query execution. Combining them makes debugging more difficult, as you can see. Using mysql_error() during development is a good idea, on a live site, you'd want to echo a generic "Sorry, there was a problem, try later" error message, and log the mysql_error() rather than display it. See if this now returns any errors. // Update new value $query = "UPDATE vars SET `credits500`=$newvalue[0] WHERE id=2" // removed quotes, assuming integer data is expected $sql = mysql_query($query) or die('<br />Query string: ' . $query . '<br />Produced this error: ' . mysql_error());
-
I assume you want to change the file's contents if a valid string is entered in the <textarea></textarea>. This should work just fine. Read through the comments so you can see what exactly it does, and why. I only quickly tested it, but if anyone sees any problems, I'm sure they'll point them out . . . <?php $file = 'data1.txt'; // name of file to be used, if not in same directory as script, include path $min_input = 5; // Minimum string length to be considered as valid input. Any less will show error, and leave file unchanged. if( $_POST['submitted'] == 1 ) { // presence of hidden field value indicates form has been submitted. if(strlen(trim(strip_tags($_POST['data']))) < $min_input ) { // if prepared string will be less than $min_input chars, assume bad input. $errors[] = 'Entered text was too short, file not updated'; // string too short, store error in array } else { $data = trim(strip_tags($_POST['data'])); // remove leading/trailing whitespace, and any markup tags to help prevent XSS attacks if( !$fp = fopen($file, "w") ) { // open file for writing $errors[] = "File open failed for writing."; // if no open, store error as above trigger_error( "Opening of file '$file' for writing failed" ); // log, if file open errors } else { fwrite($fp, $data); // write the string fclose($fp); // close the file handle unset( $_POST['data'] ); // clear the sring from $_POST['data'] so form is no longer sticky. } } } // code below is executed always $handle = fopen("$file", "r"); // open file for reading if ($handle) { while (!feof($handle)) { $buffer = fgets($handle, 4096); // allow for larger file size $read .= $buffer; // add each chunk to $buffer } fclose($handle); // close the file echo "File '$file' currently contains:<br /><pre>\"" . $read . "\"</pre><br />"; // display the contents of $file } else { $errors[] = 'Unable to open file.'; // if file won't open, store and log error trigger_error("Opening of file: '$file' for reading failed"); } if( !empty($errors) ) { // if there were errors, loop through the array and display them. foreach( $errors as $v ) { echo "<font color=\"red\">$v</font><br />"; } } ?> <form name="form" method="post" action=""> <?php // Form submits to itself. No need for meta redirects. // ?> <textarea name="data" rows="4"><?php if( !empty($_POST['data'])) { echo $_POST['data']; } ?></textarea> <?php // If text entered doesn't validate, it is redisplayed // ?> <input type="submit" value="write" /> <input type="hidden" name="submitted" value="1" /> <?php // Added hidden field to pander to some versions of M$ Internet Exploder's non-compliance with standards // ?> </form>
-
But then a single space would be validated as good input. if( strlen(trim($_POST['var'])) > 0 ) { // Or greater than the minimum valid value for the data type . . . // then perhaps go a step farther and run a preg_match() or other check on it
-
Also, you may also to unset any transaction-related SESSION vars . . .
-
echo var_dump($data); and see what it shows for values. Also, shouldn't this be an elseif? else($submit == 1) {
-
That sounds like a great deal. Can I have the URL? Just kidding. A header() redirect on successful completion of the transaction should do the trick.
-
What are you having trouble with? What is/isn't it doing?
-
You're missing a closing curly brace } before the closing php tag.
-
Yes, rather than fix the cause of any errors, just suppress the messages.
-
Just noticed I didn't edit the code back to your database image array. Did you get that figured out as well?
-
Changed the structure to supply mysql_fetch_assoc() with a valid result . . . $q = "SELECT COUNT(*) AS count FROM attack WHERE attacker_id = $AttackerId AND defender_id = $AttackedId AND attacktime BETWEEN DATE_SUB(NOW(), INTERVAL 1 HOUR) AND NOW()"; $result = mysql_query($q) or die('<br />Query string: ' . $q . '<br />Produced error: ' . mysql_error() . '<br />'); $row = mysql_fetch_assoc($result) or die(mysql_error()); if($row['count'] == 3){ die("You have already attacked this person 3 times this hour."); }
-
Without the ability to echo the query, you can't really debug it. Separate the query from the query execution, and echo it along with mysql_error(). $q = "SELECT COUNT(*) AS count FROM attack WHERE attacker_id = $AttackerId AND defender_id = $AttackedID AND attacktime BETWEEN DATE_SUB(NOW(), INTERVAL 1 HOUR) AND NOW()" mysql_query($q) or die('<br />Query string: ' . $q . '<br />Produced error: ' . mysql_error() . '<br />');
-
mysql_insert_id() after the iNSERT query will return the auto-incremented index of the table (you do have one, right?) for the last inserted record. You can pass that as a $_GET var in the URL to form a link to the post.
-
You can use the modulus operator to see when to end and reopen the <tr> tags. This will keep the images 2 across regardless of the number of images the query limited to, and sill complete the last table row if it's an odd number, so the table isn't "broken". <?php $limit=4; //$latest=mysql_query("select photoname from `queued_photos` LIMIT $limit"); $i = 1; echo "<table width=\"150\" style=\"border: 1px solid black;\" cellpadding=\"0\" cellspacing=\"0\">\n"; while($i < { echo $i % 2 == 0 ? '' : "<tr>\n"; echo "<td><img src=\"../queued/{$thumbs['photoname']}_thumb.jpg\"></td>\n"; echo $i % 2 != 0 ? '' : "</tr>\n"; $i++; } echo $i % 2 != 0 ? '' : "<td> </td>\n</tr>\n"; echo '</table>'; ?>
-
Perhaps you should replace the comments at the end of the script with some echos so you can see what's going on.
-
Best way to copy mysql DB structure from template upon new sign up?
Pikachu2000 replied to msaz87's topic in PHP Coding Help
Store the creation query string in the admin DB, with a variable for the table name. Then just run the query to create the table with the IF NOT EXISTS parameter to make sure the table name isn't duplicated, or by using some other unique value as the table name. -
I believe it's all in php. It's fun, and it has tons of users. I find it to be a great escape when I'm stressed out, which seems to be always.
-
Have you seen www.kingdomofloathing.com ? Might give you some pretty good ideas.
-
First, you'll want to get in the habit of separating the query string from the query execution. It makes debugging easier. Then add the or die() for testing. Change die() to trigger_error() for live use, and check for a result $query = "SELECT whatever FROM table WHERE field = 3" $result = mysql_query($query) or die( 'Query string: ' . $query . '<br />Cratered with error: ' . mysql_error() ); if($result) { //do something, echo output, whatever. } else { //error, so present generic error message } Also, any time you use a header() redirect, you need to issue an exit() to stop the script from executing further } else { header("location: index.php"); exit(); }
-
if (isset($_POST['submit'])) { is unclosed. I'd imagine it should be closed right before the ?> php close tag.
-
That error almost always indicates a missing curly brace.
-
foreach only puts first check box in db
Pikachu2000 replied to turpentyne's topic in PHP Coding Help
Well, that's a start. At least we can narrow things down. Paste the errors here. -
foreach only puts first check box in db
Pikachu2000 replied to turpentyne's topic in PHP Coding Help
To have the code in the code or php boxes, just post it within . . . or . . . bbcode tags. I've just tested this, and it should work fine. There is no plant_id field in the form, there is item_id. See my comment in the code regarding that, also, to submit a form to itself, you can simply leave it as <form action="". . . There's no need to specify the script name. <html> <title>title</title> <?php if (isset($_POST['submitted'])) { $errors = array(); $item_id = ($_POST['item_id']); // This was $_POST['plant_id'], which didn't exist in the form. $column2 = ($_POST['column2_id']); if (empty($errors)) { require ('databaseconnect.php'); foreach ($column2 as $val ) { $query = "INSERT INTO tablename(item_id, column2_id) VALUES ('$item_id', '$val')"; mysql_query($query) or die( mysql_error() ); // echo $query . '<br />'; } if ($result) { echo 'items have been added'; } } else { echo 'system error. Not added'; echo '<p>' . mysql_error() . '<br><br>query:' . $query . '</p>'; } mysql_close(); } ?> <body> <FORM style="border: 1px dotted red; padding: 2px" action="" method="post"> item id field:<br> only enter numbers here<br> //this will be a hidden passed variable in finished form <input type="text" name="item_id" value="<?php if(isset($_POST['item_id'])) echo $_POST['item_id']; ?>" /> <br><br> characteristics:<br> <input type="checkbox" name="column2_id[]" value="1" > one <br> <input type="checkbox" name="column2_id[]" value="2" > two <br> <input type="checkbox" name="column2_id[]" value="3" > three <br> <input type="checkbox" name="column2_id[]" value="4" > four <br> <input type="checkbox" name="column2_id[]" value="5" > five <br> <input type="checkbox" name="column2_id[]" value="6" > six <br> <input type="checkbox" name="column2_id[]" value="7" > Seven <br> </fieldset><br><br> <input type="hidden" name="submitted" value="TRUE"> <input type="submit" /> </form> </body> </html> -
I'd like opinions on the overall look
Pikachu2000 replied to Pikachu2000's topic in Website Critique
In which browser and what OS are you seeing the width of the bar as not consistent?