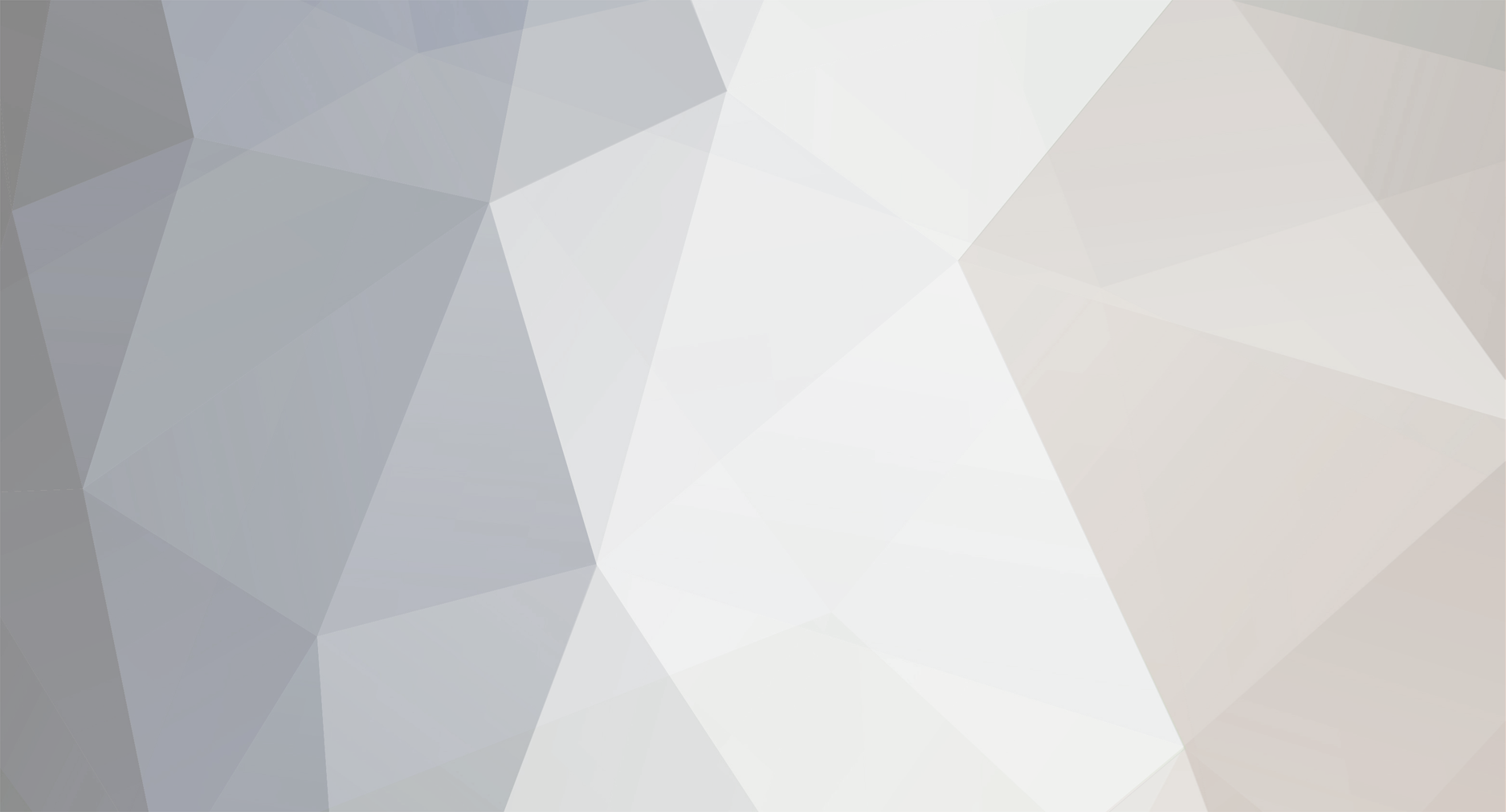
RussellReal
-
Posts
1,773 -
Joined
-
Last visited
Posts posted by RussellReal
-
-
no doubt
mark as solved
THANKS!
-
try adding hidden fields to each form you create called 'godate' that will allow your first php block to execute
-
lol I'm trying to unpack it, but I'm having no luck
maybe someone here whose done more work with unpack can get it to work
you can probably use substr to manually get the bytes you need
-
what do you mean.. like a timer? inside or outside of a php script?
inside of your script you'd need to just throw yourself into an infinite loop..
outside of a php script.. you can use cronjobs
-
show us an example file?
-
I'd love to help you.. but you made like no sense
also use
tags please
-
why are you using savefile() 3 times just for if and elseif statements.. why not save file ONCE and then store that in a variable THEN do the checks
-
you could do a sub query
like..
SELECT (SELECT AVG(ratings.score) FROM ratings WHERE ratings.article_id = articles.id) As average FROM articles;
a join will not really work
-
flash would be your best bet.. but you say you don't want flash? I know of a guy who made an actual playable version of Super Mario Brothers in JavaScript, but that must be such an outstanding amount of code involved.. if he had made it in javascript it'd have been s much less code, more likely more efficient, and could have built more, with less
you will not find any "arcade style" games written in any other language except Java and Flash for playing within the browser
-
php oop is worthless for this type of thing, php classes for this type of precedure is basically to make this code includeable into multiple files..
php oop is GOOD for creating multiple instances and have them independant of the other instances..
for this purpose classes aren't going to give you an "oop" feel, it will give you a function feel with a nifty wrapper..
-
just make 1 sold image.. when it gets sold.. create the image and display that image instead of the first one..
unless you want to add the "sold" like ontop of the image (in html - layered) in which case.. you can just set a div with the image as it's backgound.. and with javascript display "SOLD"
-
hey shes an orangutan so her tastes are a bit different! I think it could look alright if the menu was a little less.. err.. long..
and yeah if you want to use that logo atleast match some colors to it
but if you're gonna keep the current color scheme.. you should go with a blue color logo not a very emo looking logo
-
basically the query is bad
-
things like this make mysql kind've obsolete, basically mysql being a relational database, needs things to relate to other things.. basically to have data work together you need to bounce back and forth..
the correct way to do this with mysql would be to have a "like" table..
with the structurelike so:
id | like | dislike | user_id | media_id | comment_id
and use this table for everything..
the drawbacks of this.. is you will run outta available ids in the long run.. even though its like some super large never to be reached number on 64bit servers.. ( I don't have this number off the top of my head
)
but.. you could do it the WRONG way.. and have it comma seperated... in the particular table
for example..
id | media_name | media_source_path | media_description | media_likes | media_dislikes | voters
and pull a result.. say I'm voting on video with the id number 37
SELECT * FROM media WHERE id = 37;
we now have the row.. pretend I do all the php to get that result set..
if (in_array($_SESSION['id'],explode(',',$resultSet['voters']))) { // hes in here screw him! } else { // hes not!! WOO HOO! // set voters to include his id.. $x = explode(',',$resultSet['voters']); $x[] = $_SESSION['id']; $x = implode(',',$x); if ($_GET['like']) { // 1 or 0 expecting lets say $set = "media_likes = media_likes + 1"; } else { $set = "media_dislikes = media_dislikes + 1"; } mysql_query("UPDATE media SET {$set}, voters = '{$voters}' WHERE id = '{$_GET['media_id']}"); // update that row! }
but the WRONG way has some drawbacks aswell.. there is a limit on text fields aswell, but that is a couple gigabytes for large blobs.. its really up to you what you want to do.. those are two ways to go about doing it
-
in MOST php applications (excluding COMET or PUSH implementations) after the php has finished being executed, php exits.. meaning you cannot continue to modify the output the user sees already..
php is for the most part server side, with another select few exceptions, but for most WEB APPLICATIONS it is 98% server side, with like I said before, COMET and PUSH applications..
JavaScript, however, is a CLIENT SIDE language with another few exceptions.. but for the most part this is the language you will want to utilize to manipulate information already sent to the client (the browser).
-
if (strlen($searchTerms) < 3) {
haha.. you made a heart
aside from your beautiful heart
your crazy } needs to be removed for sure, and your error is probably because that crazy } belonged to an if statement which was to see if the form had been submitted
which you removed..
-
register shutdown function will run when php ends.. however, it will not end when a window is closed.. you can set expiration dates to your cookies.. which will be a good idea anyway
-
If you really have "®" in your db, you might need to html_entity_decode() twice to get the actual character. Keep in mind that ® and ™ are not going to map to ascii characters though ..
Instead of decoding, you might want to just replace "&...;" sequences with a single dummy charater, so you can get the length count correct. Then use the original string for displaying.
thats what I do in my first example with "X" but yes he does make a point about the ascii characters, I'm not a real guru with charsets or anything I just know regex n stuff
-
curl_exec will block until the transfer is complete..
curl_exec_multi will not block.. but unfortunately php doesn't really utilize events like it should therefore exec_multi should only be used when needed..
for NOW what you should worry about is why the transfer is failing.. you received http status code 301.. which is permanently moved, you need to tell php to follow redirects
you do this with the curloption CURLOPT_FOLLOWLOCATION
-
u don't need preg_replace..
you could just copy/paste the symbols into replace() and replace them as needed
-
try this regex..
preg_replace("/&[a-z]{2,5};/i",'X',$title);
that SHOULD work..
however.. y ou could just pass over with html_entity_decode
-
why not try this:
obj.value += text1 + text2 + obj.value.substr(end, obj.value.length);
also note, I really don't see a problem with the code above, if anything the substr is giving you a pain in the balls because it has no data to manipulate
oh sorry.. edit #2.. scratch the above.. just add in a clause..
if (obj.value.length) { obj.value += text1+text2; } else { //do ur code }
-
setopt CURLOPT_RETURNTRANSFER true
-
Help with Registration Class
in PHP Coding Help
Posted
oop is for like..
if you wanted to have more than 1 instance of the same code..
I'll write up a little class for you
thats an example of a little game.. you can like make it an actual game with a server connection and stuff.. basically Player is an instantiable object.. to keep data specific to that Player
the Game object is the object which indexs all of the players, its more of a package than an object..
but if you did make it an actual game, you would add connectivity functions to the Player class.. not the Game class, because each player needs to test connectivity individually.. etc..
a class for the MOST PART is just to have a batch of values and functions that work independantly and can be reused..
heres a script I made a while ago, its a server->client script.. it is long long ago so I don't know how good it is anymore, but it is a great example of using OOP
http://lovinglori.com/newServer.phps
I also wrote a connection class.. which you can use to create multiple connections.. wrapped in along with lots of easy to use functions..
this is the version from an older project I've worked on, I've updated this class since.. however, the beauty of this being an object.. is I don't need to remember to specify an mysqlresult (which you get from mysql_query) in mysql_fetch_assoc and stuff.. since its an object you'll really only run 1 query at a time.. but in the event you do want to hold 1 result set and process another before the prior.. you can specify an optional field $result
these are all 3 good examples of proper oop..
making a class just for 1 page which really doesn't NEED oop.. is just gonna give you a function feel to classes, classes are more powerful than just for that
, although they lack in alot of ways in PHP compared to other languages, they're st ill really handy 