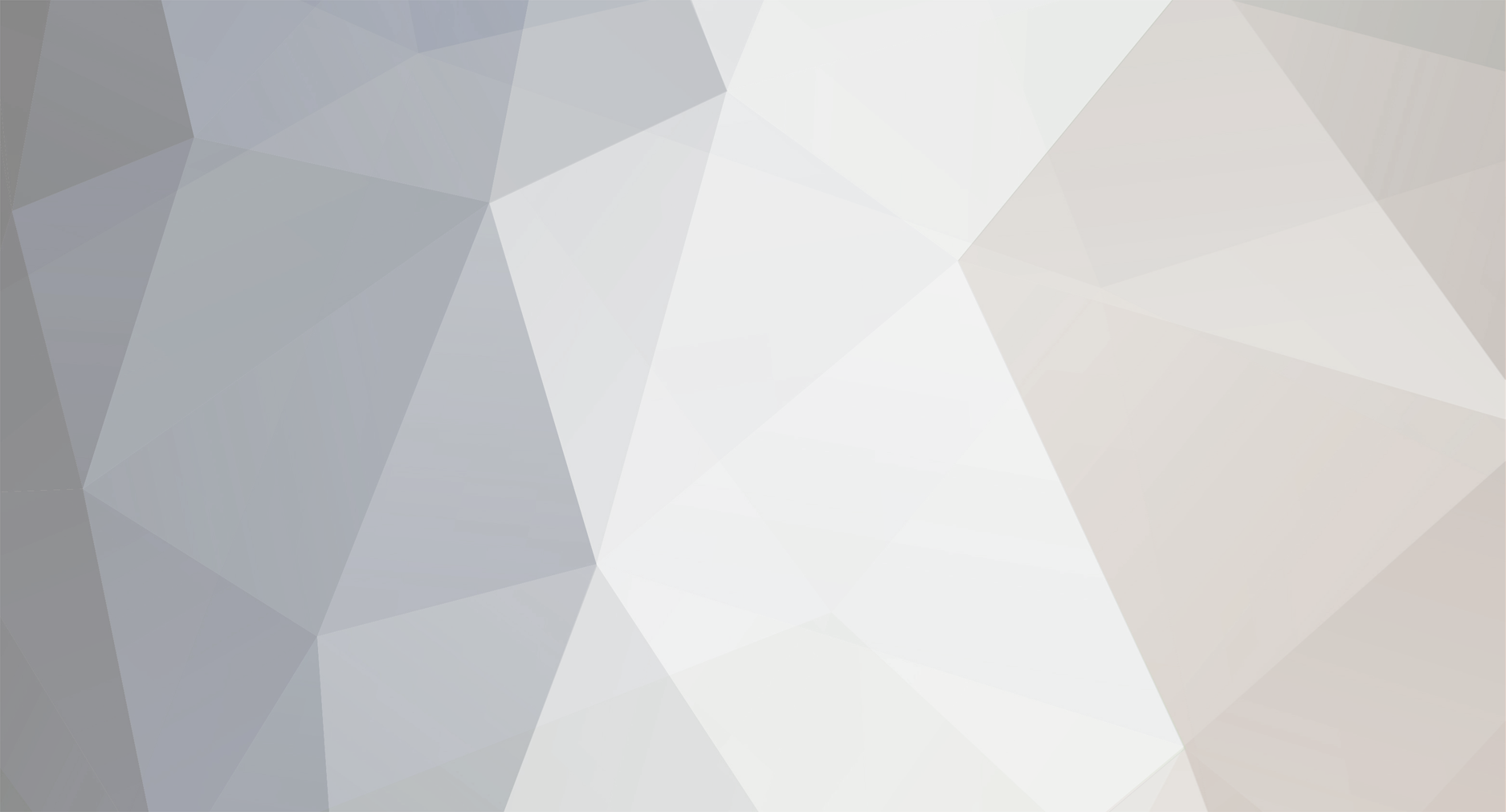
RussellReal
-
Posts
1,773 -
Joined
-
Last visited
Posts posted by RussellReal
-
-
foreach ($dropDowns as $name => $v) { echo "<select name='{$name}'>"; foreach ($v as $opHTMLValue => $optValue) { echo "<option value='{$optHTMLValue}'>{$optValue}</option>"; } echo "</select>"; }
this will expect an array structured like this..
array( [places] => array( [myroom] => My Betroom!, [bathroom] => The Bathroom! ), [names] => array( [names1] => Tanya, [names2] => Jane ) )
-
no php required.. all javascript
-
Show us what you get and what you expect to get.. seperating by \n SHOULD be enough to get you lines
-
and it is dangerous to allow end user to add JS to your website, we spend a lot of time stopping JS includes. JS can give then an open window to ur server.
allowing js doesn't give you a window to your server anywhere other than pages that are requestable from the outside anyway
.. JS never reaches to the backend.. the only thing you need to look out for is server-side javascript..
what it DOES do is give them access to cookies on other users' computers, and that is a huge security flaw.. but saying it is a window to your server is a very big exaggeration
-
oh I seeeeeeeeeeeee yeahhhhhhhhhhhh
.... ... .. .
oh right.. javascript..
lemme ask you.. do you eval() this code at all? do you put it into a file then include this page? because if you for instance..
echo a string which contains "<?php echo 'hi'; ?>" (well technically it wouldn't work because ?> would kill it, but I'm assuming you're pulling it from a DB so the technically doesn't affect you now does it?) it will basically echo it as a string.. not evaluate it
so you posting here technically is a waste of time.. if you aren't building a file then including it.. and another side note, if you look around I'm the only one here who posted to help you so its probably in your best interest to not get all sarcastic and stuff on a public board, where you are the one here asking for help, and I am the good guy trying to help you
..
Have a good morning in any event
-
htmlEntities() the output
should fix everything
-
ofcourse there is,however, it is a little tricky.. you see, mouse position is not very cross-browser (interms of the properties you're using from the MouseEvent object), therefore its probably a good idea to use a cross-browser solution (jQuery
however, if you do NOT want to use a cross-browser solution you will need to calculate and manipulate the data you receive from the event
in IE6-8 'x' and 'y' will represent the cursor position INSIDE the firing element, where as.. Chrome and Firefox will use layerX and layerY to do/represent this..
you will also need to know the width of the content within the overflowing div.. its usually a good idea to structure this like this:
<div id="container" style="overflow: hidden; width: 500px; height: 100px;"> <div id="content"> <!-- enter all your content here! --> </div> </div>
this way you can use the element #content to get the width of the entire content.. ALSO you can just slide this div left and right using css
OR you can use the .scrollLeft() method of #container
Whichever is clever
last thing you'll need to do is calculate your mouse's position within an element against your content div's width..
for example.. you will want to use this formula..
(MouseX / ContainerWidth) * ContentWidth
and that will give you the amount to move the #content element from the right..
using this javascript line:
document.getElementById('#content').style.right = (MouseX / ContainerWidth) * ContentWidth;
-
why not just go to jquery.com, build your theme with jquery the way you want it, then download the correct accordion version aswell as the tabs
that will solve your problem.
-
add me to MSN I want to discuss me joining this project of yours
RussellonMSN@hotmail.com
Are you sure that couldn't have been sent via a PM? Hope your spam box is nice and roomy.
this email is always getting spammed, it makes me feel alive, thank you for the concern though thorpe
I sent you a PM you never answered that 1 though
-
oh yeah..
how about you follow this guideline here to K.I.S
(Keep It Simple)
#1 instead of using all ids, use class names.. thats simple enough?
then just do like this
<style type='text/css'> .userEdit { display: none; } .userInfo { display: none; } .show { display: block; } </style> <input type="text" class="userEdit" name="x" /> <span class="userInfo show">The Infoz</span>
#2 now that you have a specific piece of information to tie together to your inputs.. you can simply do something like this:
<script type="text/javascript"> edit = false; function toggleData() { edit = !edit; /* flips edit over E.G. the Toggle */ inputs = document.getElementsByTagName('input'); for (i in inputs) { if (inputs.className.split(' ')[0] == 'userEdit') inputs[i].className = 'userEdit'+((edit)? ' show':''); } spans = document.getElementsByTagName('span'); for (i in spans) { if (spans[i].className.split(' ') == 'userInfo') spans[i].className = 'userInfo'+((!edit)? ' show':''); } } </script>
#3 now that you have this function, dw about the traversing the elements on the page, it really doesn't matter js doesn't make it noticeable that its doing that kinda work unless you have 5,000 spans on the page
which even still wouldn't be that noticeable
, anyway,
now that you have the function you just need to use it!
document.getElementById('toggle').onclick = toggleData();
and there you are! All set!
-
add me to MSN I want to discuss me joining this project of yours
RussellonMSN@hotmail.com
-
document.getElementById('left').style.width = '0px';
document.getElementById('right').style.width = '0px';
-
http://dev.mysql.com/doc/refman/5.0/en/update.html
UPDATE table SET col = 'value' WHERE col = 'value';
haha you following me JCB? <3
-
anytime man
you got my username, if there is ever a question that takes too long to get answered just send me over a PM I've got a bit of freetime every now and then (the life of a developer)
-
since you've placed the dll in the ext dir and enabled it in the ini.. what error have you been getting?
EDIT: I took a look @ the installation requirements, which states that they do not have a .dll for the extension, and PECL is down for windows XP+ unless you have visual basic 98 or visual studios 98, you need the compiler bundled with that.. then you'd also need to install pear and then go thru the command line to install the pecl extension..
In other words.. you're more than likely not gonna get this extension for windows
Sorry man <3
-
does it have... asp? can you request the host install php for you? you will need a backend script somewhere.. maybe get a free hosting site that supports php and put geolocation software on that server.. then AJAX request where they live from that server..
also help on a certain library/opensource code will more than likely need asking in the opensource forum here on phpfreaks.
-
paragraph*
more to your question..
a parent element is an element which contains another element
<div> <span></span> </div>
span's parent is div..
traversal is basically moving from element to element..
it basically says javascript makes it easy to traverse element to element even from a certain point.. E.G. getting <span>'s parent would be as simple as..
document.getElementsByTagName('span')[0].parentNode;
so when you read that paragraph just think traversal as moving from one element to another.. or between, outward and inward..
-
it is not a stupid question it is nearly impossible unless they don't protect against sql injection, and even then you'd need to know the table name to get any data about the table to be output..
your best bet is to not worry about their table structure.. and build your database to accommodate data output that their site outputs..
for example.. if their website outputs information like:
artist name, song name, song length, song bit rate, song genre..
then you know those fields are going to be needed
if you need any help feel free to MSN me RussellonMSN@hotmail.com
I won't do any code for you but I can give you advice
-
select value, class from tablename where (class = 'total' OR class = 'coupon') and id=$id ORDER BY class
I took the liberty to add in 'class' to the list of values retrieved from the table, because if you are combining the two of them into the same result set you will probably want to sort them.. I sort them also in the query by class so all the totals will be together with the totals and same for the coupons..
but you will still need to put an if statement in there..
if ($row->class == 'total') { /* do for total */ } else { /* you're only selecting either total or coupon so else will obviously be coupon */ }
-
memcached?
-
I'm not exactly sure you're updating by deleting a row then re-adding the row.. why not just UPDATE?
-
are all the fields named and assigned appropriately?
-
if you scan the directory every time the page is loaded, that is most likely not efficient to begin with.. But if it must be done, you only need to record the list as it goes thru.. and record the name of the image before the current one.. the current one.. and the next one after the current..
for example..
$getNext = (boolean) ($curr = ($prev = ($next = ''))); foreach ($images as $filename) { if ($getNext) { $next = $filename; break; } if ($filename == $_GET['requestedImage']) { $getNext = true; $curr = $filename; continue; } $prev = $filename; } // $next, $prev, $curr are what you're lookin for
-
Some apache configurations limit the post size and file sizes also, aswell as enforce a timeout for the connection.. That is number one..
use set_time_limit and set the time limit to 0(unlimited)..
for the first one.. you probably don't have to worry, but you can talk to your hosting provider to update your configuration for you
and the second one you already handle in your php ini but it shouldn't hurt to try it
all in all I don't see why there would be a problem
Click a button to open 'custom' URLS
in PHP Coding Help
Posted
you don't, it'll be moved