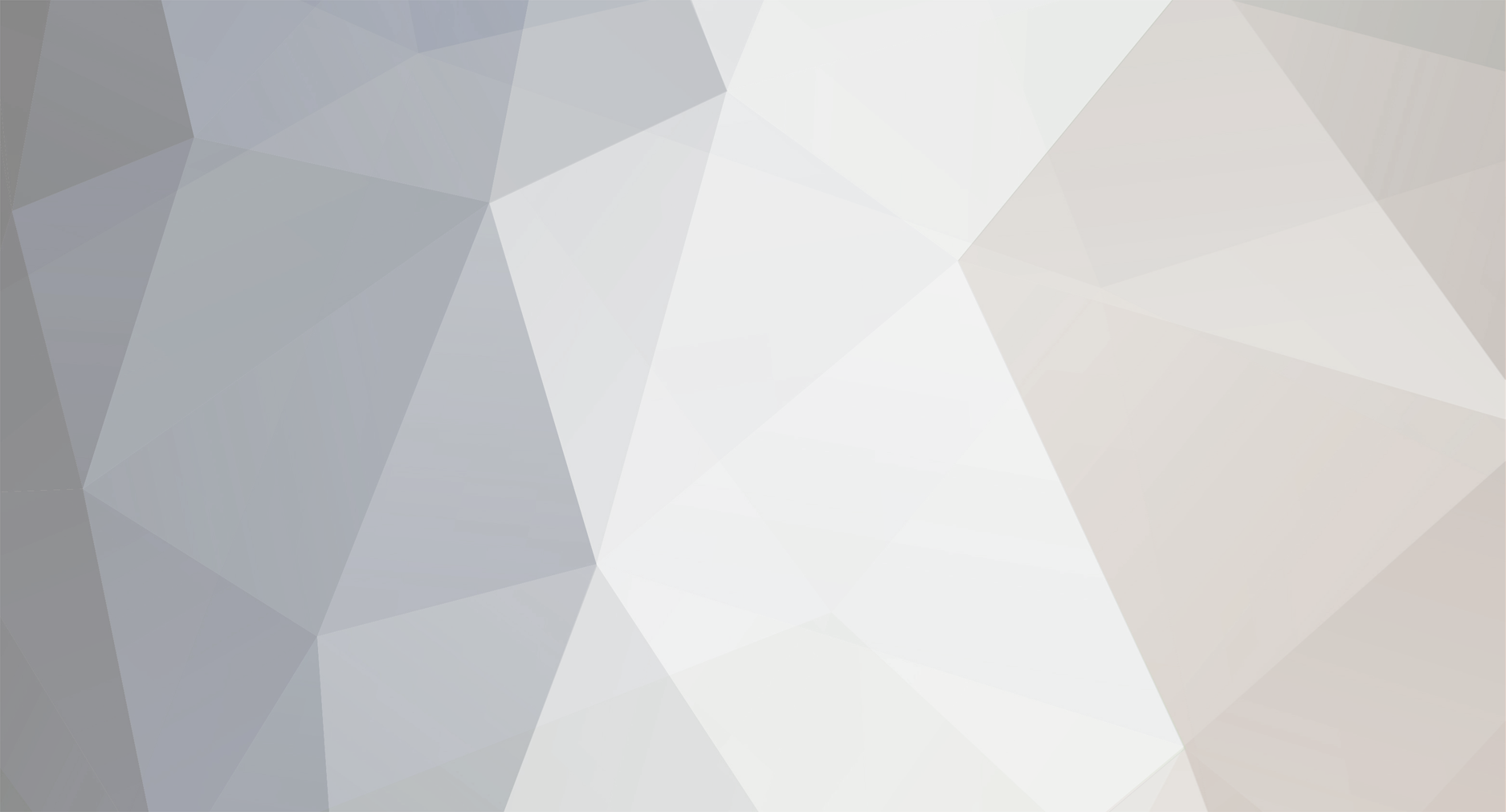
RussellReal
-
Posts
1,773 -
Joined
-
Last visited
Posts posted by RussellReal
-
-
after this line
$query = mysql_query($sql);
add this line:
echo mysql_error();
I suspect that the error is telling you that the table you're trying to access doesn't exist
SIDE NOTE (edit):
You will probably want to change your mysql credentials when your site goes live, as it is now public information and if you're going to be dealing with money you're gonna want to have your mysql info not as public
aswell as your table name, because that is the big "?" when sql injecting
-
<?php $total = 0; foreach ($data as $v) { $total += $v['score']; } ?>
-
show some code man
- Russell
-
I think your code is structured completely wrong
You should not be looping through the return results from a mysql query if you already have the ids, that is redundant at best..
Try something like this..
<?php mysql_query("UPDATE upload SET uploaded = 'yes' WHERE id IN (".implode(',',$usersids).")"); ?>
This is basically saying.. set all the rows that's id is in $usersids to show uploaded = yes..
-
thanks for the quick reply and excuse my ignorance but I don't understand what exactly you're saying. I did a copy paste into my page and it didn't work, and actually broke the whole site.
Having said that, and taking your comments about security with the gravity it deserves, what would be the CORRECT way to go about doing this ignoring the code that I wrote which is clearly the wrong way to approach this problem?
Again thanks for you assistance!!
-Carl
the code I gave you should work with your current way of handling urls.. but instead of having a url like:
http://yoursite.com//lala.php?category=includes/lala
it will need to be
http://yoursite.com/lala.php?category=lala
-
this isvery dangerous.. and very susceptible to an XSS attack.. I would suggest you to prepend a directory or something to the included file at the least.. or to be more secure toss the values thru a switch statement..
or at even a lower level of security.. remove "include/" from the urls and put: "include/" as the initial value to $url..
and your problem is.. when there is no category or page values you don't default to any categories
..
try this..
$url = 'include/'; if (!empty($_GET['category'])) { // do your thing here $url .= $_GET['category']."/"; } else { // ahhhh now we're gonna handle what happens when no category is given.. $url .= "global/"; } if (!empty($_GET['page'])) { // do your thing here $url .= $_GET['page'].".php"; } else { // ahhhh now we're gonna handle what happens when no page is given.. $url .= "new1.php"; }
note this could be done in 3 lines.. but to keep it within your code I just copy/pasted and edited
-
you if you have X amount of field names you need that same amount of values
for example
INSERT INTO table (field1,field2) VALUES (value1, value2)
if they don't match you get that error
-
anytime:)
-
very good catch.. you're using filesize as a variable
I know its possible to do that but in this case you'd want to drop the $
EDIT: For anybody who looks @ this what I meant by filesize as a variable:
http://www.php.net/manual/en/functions.variable-functions.php
for example:
<?php
$filePath = '....';
$filesize = 'filesize';
$filesize($filePath);
?>
but since you didn't specify the function to the variable it won't work lol
but disregard the above if you aren't interested <33
-
temporary tables..
CREATE TEMPORARY TABLE temp SELECT * FROM file As f1 INNER JOIN file As f2 ON (f1.topic = f2.topic AND f1.id != f2.id) ORDER BY f1.topic
^^ that will create a temporary table called 'temp' which will hold all of your duplicate rows..
then you get the total number like this:
SELECT COUNT(*) As num FROM temp
then you do your pagination like this:
SELECT * FROM temp LIMIT $start, $limit
-
not sure why it would corrupt, but with the content disposition it SHOULD force download either way, you could try setting the content-type as image/png because google chrome has a weird database type security system, and it encrypts everything it receives, and if it receives it as something it isn't it could be causing the corruption, (the db structure I'm referring to is it's cache, not sure why it would affect a download.. but, try it anyway doesn't hurt
)
-
<?php $nextMonth = date("m") + 1; $daysInNextMonth = date("t",mktime(0,0,0,$nextMonth)); echo $daysInNextMonth; ?>
this should do, I wasn't knocking you alex, people are lucky to have you here
I have been away from this site for a couple months now, the more coders helping people the more the community grows
Sorry if I sounded offensive I was just tryna help out
-
The next month is August, which has 31 days. If you want to test it out, try doing this as the first line:
$thisMonth = date("m",mktime(0,0,0,NUMBER OF MONTH HERE,1,YEAR HERE);
For example, if we pretend we are now in August, and want to know how many days in September, try this:
$thisMonth = date("m",mktime(0,0,0,8,1,2010));
EDIT:
Sorry, I had a few missing capital letters for variables, try this:
<?php $thisMonth = date("m",mktime(0,0,0,8,1,2010)); //gets the 2 digit expression of the current month if($thisMonth == 12) //If we are in Dec { $nextMonth = "01"; //Make the next month Jan } else { $nextMonth = $thisMonth + 1; //Otherwise add one the the current month } $daysInNextMonth = date("t",mktime(0,0,0,$nextMonth,1,date("Y"))); //set the time to the 1st of the next month of the current year (as the only time that the number of days change is in Feb, using current year is OK echo $daysInNextMonth; ?>
ALSO (and sorry for the double post) if you are in month december.. when it turns to january for $nextMonth which would be 1 you would be going from december 2010 to january 2010 instead of december 2010 to january 2011 which could tamper with the days of the week.. for example 1 year the first of january will be a monday, the next year it could be a wednesday or whatever, you're better off letting it go to 13 and it will push the year up aswell
-
oooo you should order by f1.topic.. although the problem could be with the limit if you're forgetting to set $start and $limit
-
Take a look at this:
<?php $thisMonth = date("m"); //gets the 2 digit expression of the current month if($thisMonth == 12) //If we are in Dec { $nextMonth = "01"; //Make the next month Jan } else { $nextMonth = $thismonth + 1; //Otherwise add one the the current month } $daysInNextMonth = date("t",mktime(0,0,0,$nextmonth,1,date("Y"))); //set the time to the 1st of the next month of the current year (as the only time that the number of days change is in Feb, using current year is OK echo $daysInNextMonth; ?>
All you need to know can be found at http://php.net/manual/en/function.date.php
As for part two, I dont really understand what you are looking for.
mktime for months if you specify 13 it will go back to january
The next month is August, which has 31 days. If you want to test it out, try doing this as the first line:
$thisMonth = date("m",mktime(0,0,0,NUMBER OF MONTH HERE,1,YEAR HERE);
For example, if we pretend we are now in August, and want to know how many days in September, try this:
$thisMonth = date("m",mktime(0,0,0,8,1,2010));
EDIT:
Sorry, I had a few missing capital letters for variables, try this:
<?php $thisMonth = date("m",mktime(0,0,0,8,1,2010)); //gets the 2 digit expression of the current month if($thisMonth == 12) //If we are in Dec { $nextMonth = "01"; //Make the next month Jan } else { $nextMonth = $thisMonth + 1; //Otherwise add one the the current month } $daysInNextMonth = date("t",mktime(0,0,0,$nextMonth,1,date("Y"))); //set the time to the 1st of the next month of the current year (as the only time that the number of days change is in Feb, using current year is OK echo $daysInNextMonth; ?>
for your second quote you'd want to use the date format character 't' not 'm' t will return the number of days in the current month
-
what is the error.. I am pretty sure it should work
-
for question part 1.. just add it to the code
and for question part 2.. you could insert all the rows to a temporary table...
and then count the results from the temporary table.. then output them
that way you don't need to do the same query twice
-
not sure, but I'll be honest with you, no1 will be able to give you a proper answer the code shown is very vague.. I can only give you an assumption and say that the value you're using to represent your url really isn't the full url and is just a relative path to the file '/page/home.html' not the absolute path which you are expecting
-
try this
SELECT * FROM file As f1 INNER JOIN file As f2 ON (f1.topic = f2.topic AND f1.id != f2.id)
-
use sessions..
<?php // top of php files include('monitor.php'); ?> <?php // monitor.php session_start(); // turn the session into an object to avoid strict notices (unset keys) $user = (object) $_SESSION; if (isset($temp->logged_in)) { if ($temp->logged_in <= (time() - (24 * 60 * 60))) { $temp = null; } } else { $temp->logged_in = time(); $temp->posts = 0; } $_SESSION = (array) $temp; ?>
and then whenever the user posts a post increase $_SESSION['posts'] and check if its greater than 5.. if its greater than five don't continue with the comment..
-
@OP
You're arguing against very good points (Asside from the google not being the dominant search engine, the british vs american english aswell is a stupid point, google doesn't correct those searches, you just place yourself in a different result set, probably better for you that way in any event.)
but some of his other points like a fallback.. or maybe PLACE the QUICK CONTACT button in WITH javascript, that way any1 who disable javascript will naturally not even see that button even exists..
you can't come here and ask for feedback and get defensive.. thats not the right way to respond to the requested information, its constructive criticism not ridicule. learn from the information, accept it, go with it.. but don't argue against it.
What alot of people who come to these forums forget is that most of us don't get paid for providing you our time, so value the time we invest in you, because we give you advice or we help you because we want you to succeed at whatever the endeavor may be.
-
Cool, thanks for all the feedback everyone, Its still being built so elements like the click here for a quote arent linked yet..
And why should we take out the freephone? Its so potential clients can call us without being charged? Also the website uses a 4 colum layout so thats where a lot of the whitespace is coming from at the moment, Thanks again everyone!
I'm not saying remove the PHONE NUMBER I'm saying remove 'freephone' put up Customer Service Number or like.. just put the number there, 'freephone' makes you sound like you don't understand english too much lolz
-
second site looks alright.. the logo space is too tall.. for nothing going on in there to begin with..
and the footer.. what is up with all that left-side whitespace.. its actually kinda annoying..
'freephone' what is that about? take that out..
and those links are freaking giant man.. tone them down a bit.
'click here to request a free quote' that could be made a hell of a lot better with an image map..
'our latest work' should probably be a little bit bigger than the other text below it.. it is technically a title..
^^ that seems to be about it
-
Thanks Rooput18.
I will try with corrected code later.
But just for yr info, the array consist of 40 to 50 'records', each of which has 10 'fields'.
All fields are 'text' & not special characters are used. Just alpha-numerical characters. Short fields have 4 or 5 characters, whereas longest one has 25 characters.
Do I need to use session for this purpose?
I'd use sessions for ANYTHING <3
how to break if something doesn't exist (This should be easy)
in PHP Coding Help
Posted
try this..