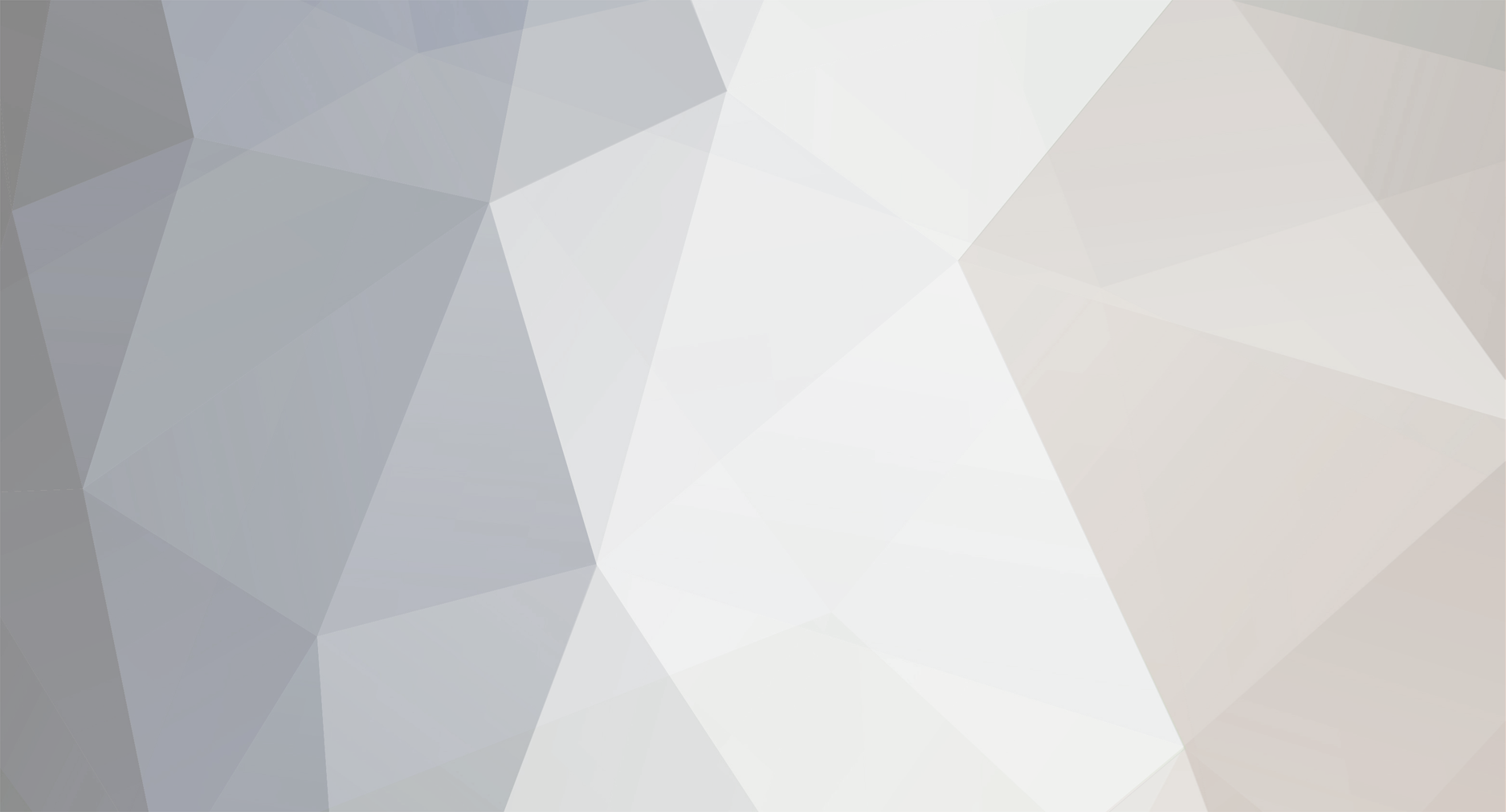
RussellReal
-
Posts
1,773 -
Joined
-
Last visited
Posts posted by RussellReal
-
-
why would you want to use ajax for this, just click a link and direct them to the page..
page.php?email=1/2/3/4
etc and do it that way and in page.php for 1 make it xxx@bla.com for 2 make it yz2@blabla.com
etc etc
but if you absolutely want to do it with ajax. (I really don't see a point)
you can just request a page via POST OR GET and send a value to it..
here for some pointers..
-
sorry buddy, I'm out of it today =\
-
I don't really understand the point =\ I'd love to help but I don't know what you're trying to do..
from what I read you're trying to do -topicname- etc.. BUT..
I really think you should be using ids instead of topic names.. because mysql is a relational database, and using indexs to relate to data is alot better than using text in a non-unique index to index topics in a 'read' table..
ids can never be duplicated in an auto increment field
-
you should never need to do this..
unless you're delivering browser dependant plugins or operating system specific..
the popup can work solely on position: absolute; across every browser..
you just need to declare the popup in html source OUTSIDE of your content div.. for example..
<body> <div>LALALALA</div> <div id="popup"> </div> </body>
then code it the wya you normally would
however, I also suggest you make #popup width: 100%; and inside have a popupContainer.. that is X wide or whatever.. position: relative; margin: auto;
that will position it in the center aslong as #popup has text-align: center; specified
good luck
-
it is most likely a frameset, but you point 1 frame to your site and 1 frame to the other site
look up <frameset>
-
what do you mean.. insert the rows from that table to another one?
INSERT INTO otherTable SELECT fieldname1 as field1, fieldname2 As field2 FROM transactionalTable WHERE id > 10;
-
there really isn't a way to do this "the right way", you know?
if the quicktime plugin that you're utilizing gives you events to use for the time updating..
but if they're simple variables you can't check the variable with an event-based fashion.
You will need to just update the times/positions every N miliseconds..
for example:
function updateTime() { document.getElementById("positionHolder").innerHTML = QT.position; } setTimeout('updateTime'),500);
SORRY I just realised I messed up a bit.. you'd need to constantly set the timeout..
you'd do this:
function updateTime() { document.getElementById("positionHolder").innerHTML = QT.position; setTimeout('updateTime'),500); } setTimeout('updateTime'),500);
-
there really isn't a way to do this "the right way", you know?
if the quicktime plugin that you're utilizing gives you events to use for the time updating..
but if they're simple variables you can't check the variable with an event-based fashion.
You will need to just update the times/positions every N miliseconds..
for example:
function updateTime() { document.getElementById("positionHolder").innerHTML = QT.position; } setTimeout('updateTime'),500);
-
browscap is an ini file full of browsers and information about them, if you want to include a huge chunk of unattractive if statements for user agents than you'd do it your way
browscap is the best alternative to this.. and probably better than what you've got going because new browscap.ini files come out whenever a new browser comes out.. + you only check for what 10 browsers? most browscap.ini variations have pretty much every distributed browser besides homemade ones from kids with visual basic..
ALSO your checks are pretty redundant in the way that if it alrdy matches "windows 7" why would you check for linux.. you feel me?
-
you should look into browscap, I never worked with it before but I have actually ran into it a couple times, it should actually help you alot, and there are TONS of browscap.ini downloads across the internet
-
if id is an auto incrementing field you don't need to show it.. and like the other poster said you're still using mysql_db_query
-
Why is create an integer, why not a date? with it as a date you will be able to use date functions..
namely:
http://dev.mysql.com/doc/refman/5.0/en/date-and-time-functions.html#function_datediff
-
is this for like a bus route or sumfin lol
but either way..
you'd be better off having ur array somethinfg like this:
[Monday] => array( [0] => 12:32, [1] => 03:33, ), [Tuesday] => array( [0] => 12:32, [1] => 03:33, ), etc
then you can loop thru the times comparing..
for example...
<?php $times = array( 'Monday' => array( '1:30pm', '2:30pm', '3:30pm', '4:30pm', '5:30pm', '6:30pm', '7:30pm', '8:30pm', '9:30pm', '10:30pm', '11:30pm' ) ); function getClosestTime($day,$timestamp) { global $times; $current = 150000; $d = false; foreach ($times[$day] as $key => $time) { if (($x = (strtotime($time) - $timestamp)) >= 0) { if ($x < $current) { $current = $x; $d = $day." {$time}"; } } } return $d; } echo getClosestTime(date('l'),time()); ?>
tested and works..
note: timezone from the server is what is used, so if your server is in Tokyo and your client is in New York.. obviously it won't be EST time it'll be like god knows what time lol!
-
to talk to php thru the game is a simple URLLoader then you handle it just like you would handle a form or whatever in php
in order to keep it secure however you're probably gonna want to add in some sort of ticketing system.. that way someone with firebug won't abuse the url request
but the concept is still rather simplistic and shouldn't be very hard
-
ok I Just read most of that article and that doesn't help me, how would I do this WITH A TRIGGER to achieve the same effect I am looking for
Thanks for the help though much appreciated
well... seems that you didn't read enough or you don't have a good understanding about how triggers work... which is ok.. we all started in some point
(no offense intended)
lets see...
what you wrote was:
DELIMITER ~ CREATE TRIGGER updTrgr BEFORE INSERT ON tester FOR EACH ROW BEGIN UPDATE tester SET tester.order = tester.order + 1 WHERE new.order <= tester.order; END;~ DELIMITER ;
just look the code... and ask yourself
- why if what I wrote is a BEFORE INSERT trigger I'm trying to UPDATE a row that is not there? (you are just inserting that row)... this doesn't make sense.
seems that what you want to do is
DELIMITER ~ CREATE TRIGGER updTrgr BEFORE UPDATE ON tester FOR EACH ROW BEGIN IF NEW.order <= OLD.order THEN SET NEW.order = OLD.order + 1; ENDIF; END;~ DELIMITER ;
nooo you misunderstand man <3 I didn't read too far you're right but I'm not trying to do what you're doing
I'm trying to update ALL OTHER rows in the table?
for example..
order
1
2
3
4
5
I add a row with the order "2"
I want it to do this..
order
1
2
2 -> 3
3 -> 4
4 -> 5
5 -> 6
understand?
-
ok I Just read most of that article and that doesn't help me, how would I do this WITH A TRIGGER to achieve the same effect I am looking for
Thanks for the help though much appreciated
-
basically I had something like this:
DELIMITER ~ CREATE TRIGGER updTrgr BEFORE INSERT ON tester FOR EACH ROW BEGIN UPDATE tester SET tester.order = tester.order + 1 WHERE new.order <= tester.order; END;~ DELIMITER ;
now this works (or the one that I used last time did)
but I get an error message on inserting.. it says something like..
#1442 - Can't update table 'tester' in stored function/trigger because it is already used by statement which invoked this stored function/trigger.so how do I do this? :S
-
probably
-
rl=links/start&a=open&category=15">Znanost</a> Datum: 21.08.2010
They do infact show dates.. and they all should show dates...
-
yes.. so why not have 3 images.. top image.. middle image.. bottom image (assuming all the comments are the same width..)
then all you do is something like this
<div class="comment">
<div class="comment-top"> </div>
<div class="comment-middle">
comment goes here!
</div>
<div class="comment-bottom"> </div>
</div>
and give the images accordingly to the divs
-
sure..
instead of
"DELETE FROM tbl WHERE name LIKE '%bob%'"
do
"SELECT COUNT(*) As affected_rows FROM tbl WHERE name LIKE '%bob%'"
-
might I ask even why you'd want to do this this way?
why not just make the borders or w.e u r tryna do with css..
nothing needs to be dynamic here.. it just needs to be positioned correctly with css
-
lol these guys are clownin with ya, don't let em bully you, everybody comes along phpfreaks one way or another, its not your fault you came across us after you came across another site
thats google's fault!
-
you need to be more clear, you're very vague and I don't think any1 here can understand what you want
loading a secured link...
in PHP Coding Help
Posted