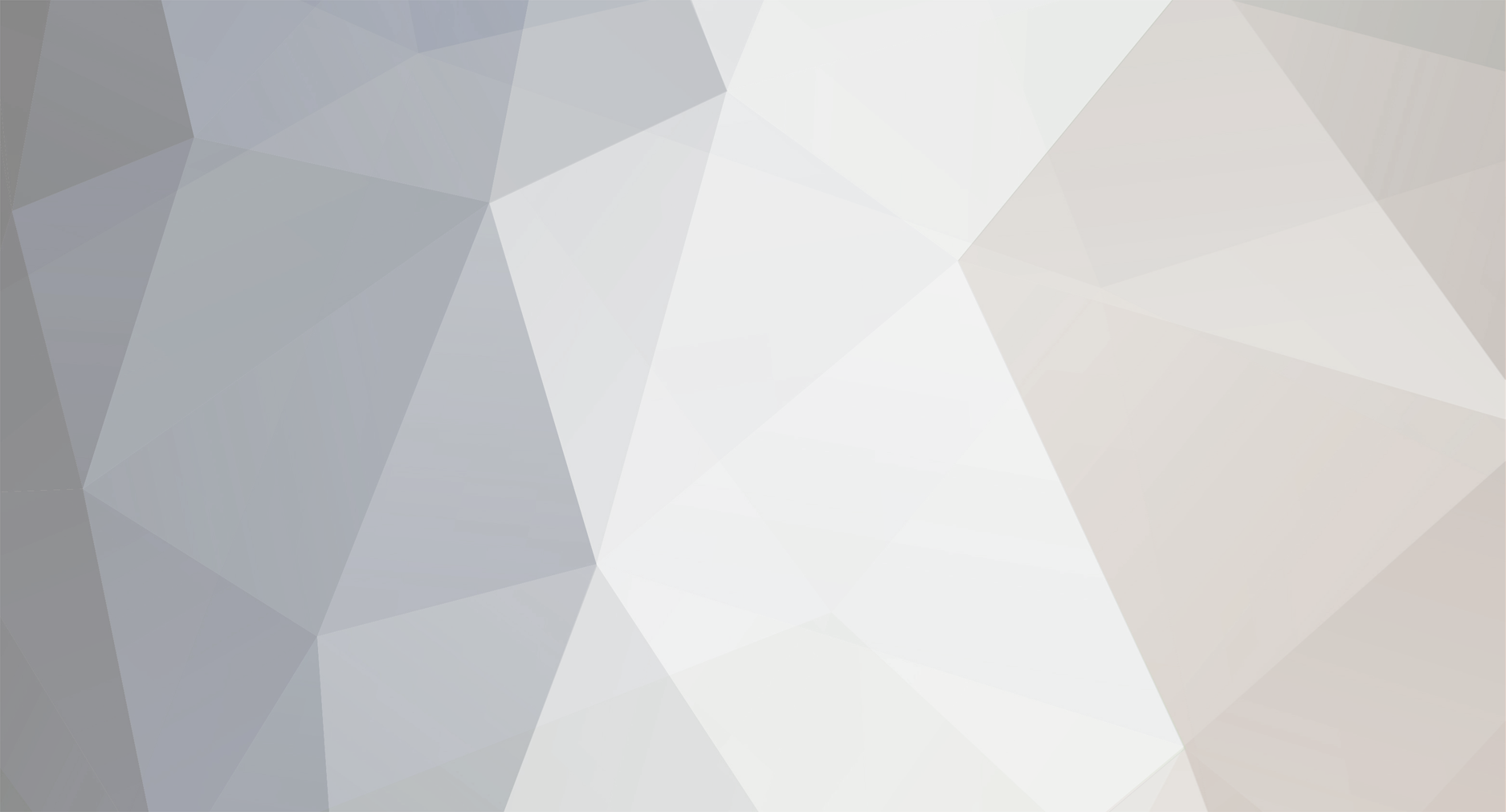
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
It would read the file from the computer that PHP is running on. You can't access files on a client's computer.
-
mod_rewrite + mysql = useful, but is it efficient?
Alex replied to flyinavacuum's topic in Application Design
The size of the actual file, or the code doesn't matter. The user isn't downloading that code from your website, only the output. You can have a small file containing the following: <?php for($i = 0;$i <= 1048576;++$i) { echo '.'; } Sure, the size of the file on your server is small, but they're still downloading a megabyte of data from your server. -
Echo out the variables and make sure they contain what you want (what you were hard-coding into the script to make it work).
-
Sorry I didn't explain the code in my first post better, it was like 4AM and I was about to go to sleep. Anyway, you can build the pot array directly within the while loop, no need to repetitious code. $pot = array(); while($row = mysql_fetch_array($result)){ $user_name = $row['user_name']; $numTickets = $row['numTickets']; echo("<p>User name: $user_name"); echo("<p>No. of tickets: $numTickets"); array_merge($pot, array_fill(0, $numTickets, $user_name)); } echo $pot[array_rand($pot)];
-
Here's a quick example on one way to do it: <?php $data = array( 'user1' => 12, 'user2' => 1, 'user3'=> 43, 'user4' => 7 ); $pot = array(); foreach($data as $user => $tickets) { $pot = array_merge($pot, array_fill(0, $tickets, $user)); } shuffle($pot); echo $pot[0];
-
Sure. echo file_get_contents('C:\path\to\file\file.txt'); file_get_contents
-
You're missing a ) on this line: if(!isset($_SESSION["list_score"]){ Should b: if(!isset($_SESSION["list_score"])){
-
Using cookies would not be effective at all. You could just set your browser not to store cookies and press the button as many times as you want. There will be no method that will work perfectly for something like this. If you use cookies they can be cleared. If you base it on ip address they can use a proxy. If you use sessions they can just restart their browser and the session is cleared from the web server, etc.. The best way would be to require registration so that they can click only one time per account, but if you're in a situation where you need to allow guests then I'd say the next best thing is to go off ip address, or perhaps even a combination of the methods mentioned above.
-
Hardcore oop enthusiast looking for appropriate framework
Alex replied to andrewgauger's topic in Other Libraries
Kohana? -
Select id, name, contact_name, street_address, city, state, zipcode, country, phone_number, email, website FROM hair_loss_clients WHERE name LIKE 'A%' ORDER BY name ASC
-
<?php echo $display_block = "<a href=\"add_new_product3.php?id=".$_GET['id']."=success&show_image=".$_FILES["image_upload_box"]["name"]."\">PREVIOUS TO EDIT</a>"; ?>
-
That's part of the output of var_dump. If you don't want that then just echo the variable. echo $section;
-
You got that error because you're attempting to call it publicly. When a method or property is protected it means you can access it from within the child class, not outside of it.
-
This will make it very difficult to download the video: if(rand(0, PHP_INT_MAX) == 73) { // Display video }
-
You should run all data through mysql_real_escape_string before inserting it into the database.
-
Just by including or echoing something that output isn't sent to the browser. In the script you provided there would only be 1 output to the browser (at the end of execution) as is the case in most situations unless you're using output buffering.
-
You can use output buffering and replace what you want before you actually output any content. <?php ob_start(); require_once ("../inc/config.inc.php"); require_once ('../inc/cookie.php'); include_once (DOC_ROOT . "/inc/functions.php"); require_once (MEMBERS_TEMPLATES_PATH . "/member_header.php"); require_once (MEMBERS_TEMPLATES_PATH . "/member_index.tpl"); require_once (MEMBERS_TEMPLATES_PATH . "/member_footer.php"); echo str_replace('%%%SPONSOR%%%', $_SESSION['sponsor'], ob_get_contents()); ?> ob_start ob_get_contents
-
You don't need preg_replace for this because you don't require the use of regular expressions. Simply use str_replace. $str = str_replace('%%%SPONSOR%%%', $_SESSION['sponsor'], $str);
-
If they're set to appear as invisible they don't want to appear as online.. I doubt that Yahoo! is going to give you any way to check if they're only invisible and not offline, that would completely void the purpose of the invisible status in the first place.
-
I moved it to the right section, then you edited your post :-\ Anyway, to answer your question you need to use $2 for the second variable.
-
@GetFreaky: Why would you do that? You can do just do this: a.php <?php $variable = 'Some value'; b.php <?php include 'a.php'; echo $variable There are almost no cases where you should use the global keyword. As for what the OP wants, I think he's after something like this: $var = '<a href="somefile.php">Link</a>';
-
You need to test your code to find which part of it is slowing down your website. Then you can figure out how to optimize it. It's not about size as much as efficiently. You can have 10 inefficient lines of code that take longer to execute than 10,000 lines of efficient code. The reason that large websites like facebook need so many servers to operate is because of the sheer size of their user-base.
-
Getting things to appear after the if...die command
Alex replied to Seaholme's topic in PHP Coding Help
Alternatively you should redesign your program so you don't need to use the die function in that manner. That would be optimal. -
No, the user can not edit session data which is stored on the server, as opposed to cookies which are stored client side. From what you've described there is nothing insecure there.
-
Check to see if when divided by 2 there is a remainder, using the modulus operator. if($num % 2 == 0) { // even } else { // odd }