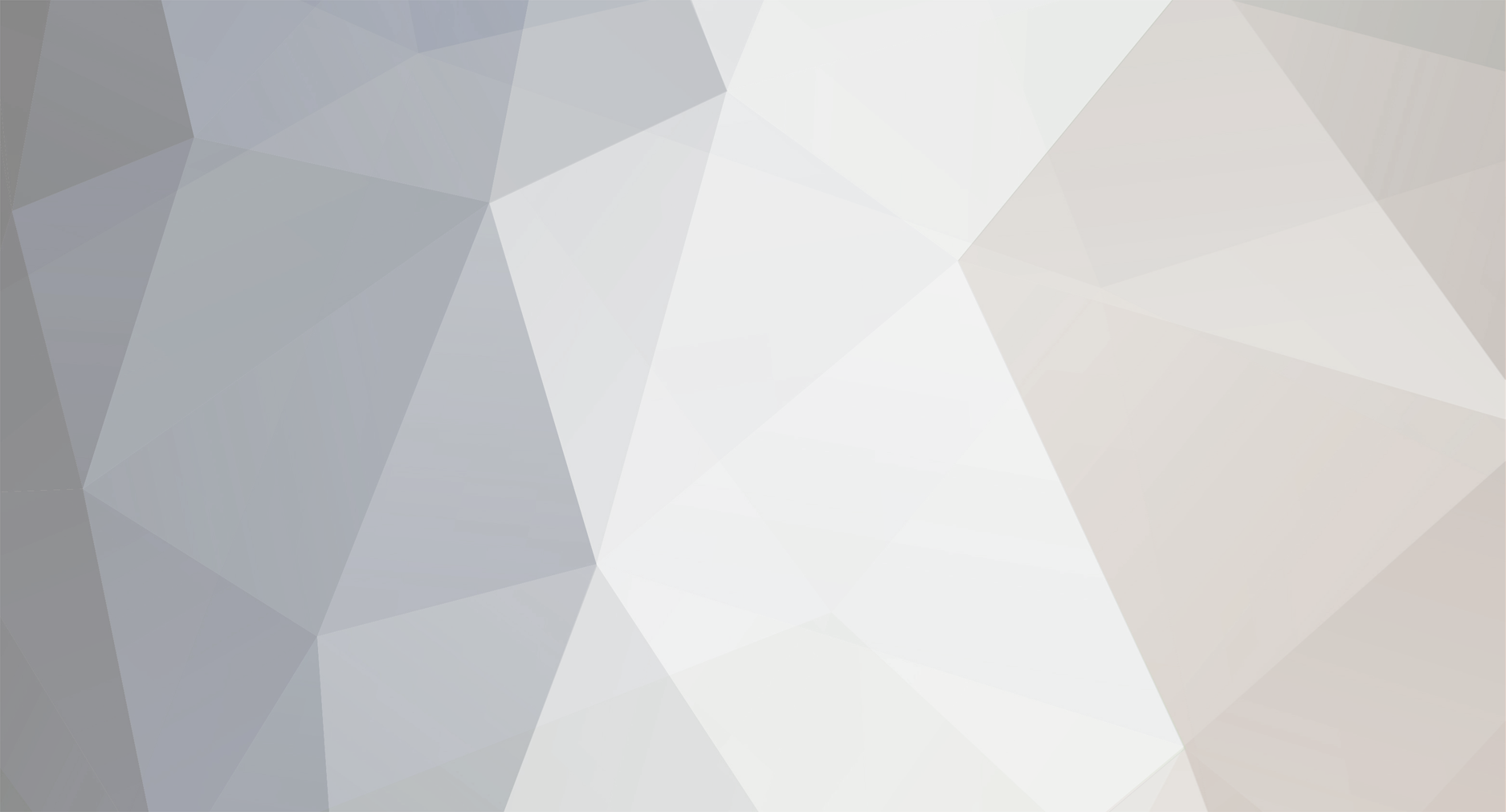
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
If you want to comment out the insertion then just move that if statement to the next line so it's not affected.
-
Because the insert line also has this on it: if ($add_member = mysql_query($insert)) {
-
When to use isset() and when to use empty()
Alex replied to dennismonsewicz's topic in PHP Coding Help
You use them for just what they sound like they should be used for. isset is used if you want to see that a variable is set, and empty is to see if a variable isn't null. isset should be used when checking for variables that have a possibility to be unset (like when a form is submitted), because it's the only way to test if a variable is set without getting a notice if it isn't. $var = ''; if(isset($var)) // true { // ... } if(!empty($var)) // false { // ... } -
Did you view the source? If you're just echoing it out normally you won't be able to see the tags..
-
You have a logic problem with your function. You're never keeping the change, so only the last replaced value is actually being kept. You can fix it by doing this: function modifier($vars, $html) { foreach($vars as $key=>$value) { $search = "%%".strtoupper($key)."%%"; $html = str_replace($search, $value, $html); } return $html; } Or alternatively: function modifier($vars, $html) { return str_replace(array_map(create_function('$x', 'return str_pad(strtoupper($x), strlen($x) + 4, "%", STR_PAD_BOTH);'), array_keys($vars)), array_values($vars), $html); }
-
Why getting Parse error: syntax error, unexpected T_OBJECT_OPERATOR?
Alex replied to ghurty's topic in PHP Coding Help
What version of PHP are you running? That syntax isn't valid below PHP 5. -
<?php $current = 14; for($i = $current - 1;$i >= $current - 5 && $i > 0;$i--) { echo $i . "<br />\n"; }
-
Replace a string, the code does not go well written but
Alex replied to pibebueno's topic in PHP Coding Help
Use str_replace instead. -
By the way, there's a "topic solved" feature. It's appreciated if you mark a topic as solved once it has been. There's a button on the bottom left of the page to do so.
-
The reason you're getting that result is because | in regular expressions means the literal "or". So you'd need to escape that character ( #\|# ). But in this specific case I wouldn't suggest using regex. PCRE functions consume a lot of memory and should be avoided when possible. Unless you need match complex patterns you should find an alternative. In your case you can just use str_replace $replace = str_replace('|', '","', $content);
-
:: is the scope operator. As RussellReal said, it's used for calling static methods. Which basically means the method belongs to the class itself, rather than an instance of it. Depending on how high your error reporting is set you may not get an error when trying to access a non-static method using the scope operator, so be careful.
-
Is it possible to use one of those conditionals in a for loop?
Alex replied to aeroswat's topic in PHP Coding Help
It's called the ternary operator, and yea that's perfectly valid. Why don't you just try it? -
I can't believe I just looked through like 10 pages of that.. [ot]You should really fix your signature. It's pretty annoying when there's only half of an interesting fact shown :-\[/ot]
-
Or alternatively.. $words = str_word_count($text, 1); print_r(array_count_values($words)); And to limit to only the top 3: $words = array_count_values(str_word_count($text, 1)); arsort($words); $words = array_slice($words, 0, 3);
-
You're missing a closing ) on this line: if( $anglerId != $_SESSION['anglerId'] OR !isset($_GET['currentpage']) should be: if( $anglerId != $_SESSION['anglerId'] OR !isset($_GET['currentpage']))
-
I could've sworn there was a built in function that would return an associative array containing each string and how many times it occurred. Maybe I'm just going crazy though. You could use something like this: function count_word_occurrences($str, $case_sensitive = true) { $str = $case_sensitive ? $str : strtolower($str); $occurrences = array(); foreach(str_word_count($str, 1) as $word) { $word = $case_sensitive ? $word : strtolower($word); if(!in_array($word, array_keys($occurrences))) { $occurrences[$word] = preg_match_all("~\b$word\b~", $str, $matches); } } return $occurrences; } $text = <<<TEXT The Law, as quoted, lays down a fair conduct of life, and one not easy to follow. I have been fellow to a beggar again and again under circumstances which prevented either of us finding out whether the other was worthy. I have still to be brother to a Prince, though I once came near to kinship with what might have been a veritable King and was promised the reversion of a Kingdom army, law-courts, revenue and policy all complete. But, to-day, I greatly fear that my King is dead, and if I want a crown I must go and hunt it for myself. TEXT; print_r(count_word_occurrences($text)); Output: Array ( [The] => 1 [Law] => 1 [as] => 1 [quoted] => 1 [lays] => 1 [down] => 1 [a] => 6 [fair] => 1 [conduct] => 1 [of] => 3 [life] => 1 [and] => 6 [one] => 1 [not] => 1 [easy] => 1 [to] => 6 [follow] => 1 [i] => 6 [have] => 3 [been] => 2 [fellow] => 1 [beggar] => 1 [again] => 2 [under] => 1 [circumstances] => 1 [which] => 1 [prevented] => 1 [either] => 1 [us] => 1 [finding] => 1 [out] => 1 [whether] => 1 [the] => 2 [other] => 1 [was] => 2 [worthy] => 1 [still] => 1 [be] => 1 [brother] => 1 [Prince] => 1 [though] => 1 [once] => 1 [came] => 1 [near] => 1 [kinship] => 1 [with] => 1 [what] => 1 [might] => 1 [veritable] => 1 [King] => 2 [promised] => 1 [reversion] => 1 [Kingdom] => 1 [army] => 1 [law-courts] => 1 [revenue] => 1 [policy] => 1 [all] => 1 [complete] => 1 [but] => 1 [to-day] => 1 [greatly] => 1 [fear] => 1 [that] => 1 [my] => 1 [is] => 1 [dead] => 1 [if] => 1 [want] => 1 [crown] => 1 [must] => 1 [go] => 1 [hunt] => 1 [it] => 1 [for] => 1 [myself] => 1 ) If you're wanting to only get the top occurring words in the string you can use arsort and only take the first few elements of the array. @teamatomic: There's a few problems with that method. Most notably that substr_count won't count only full words. So if you were searching for the word "a", it would also count all the a's that are parts of other words. Even if you padded spaces onto the string you're searching for that doesn't take into account other word barriers (like ",", ";", etc). However, the \b modifier in regex does.
-
That really doesn't help. Can you post the source to the two files?
-
Are you including first.php in second.php or what?
-
You're using the wrong header. It should be: header('Content-type: image/png');
-
If you want to use the contents of temp.php there use file_get_contents: Imagettftext($im, 25, 0, $start_x, $start_y, $black, 'impact.ttf', file_get_contents('temp.php'));
-
$var = '$filedir = "/file/path/$username";';
-
if(strtolower(substr($string, 0, 3)) == '/me') { // ... }
-
It depends on many factors. Generally speaking, there has to be a balance between the two. In most cases you won't want to have everything run on ajax/javascript.
-
A better alternative would be to use ctype_alpha, preg_match uses a lot more resources and it's unnecessary in this case. Example: if(!ctype_alpha($string)) { // Contains illegal characters } [ot]In your example you did this: $available = array('a', 'b', 'c', 'd'); //write a-z If you actually needed to create an array of a-z you could do: $arr = range('a', 'z'); [/ot]