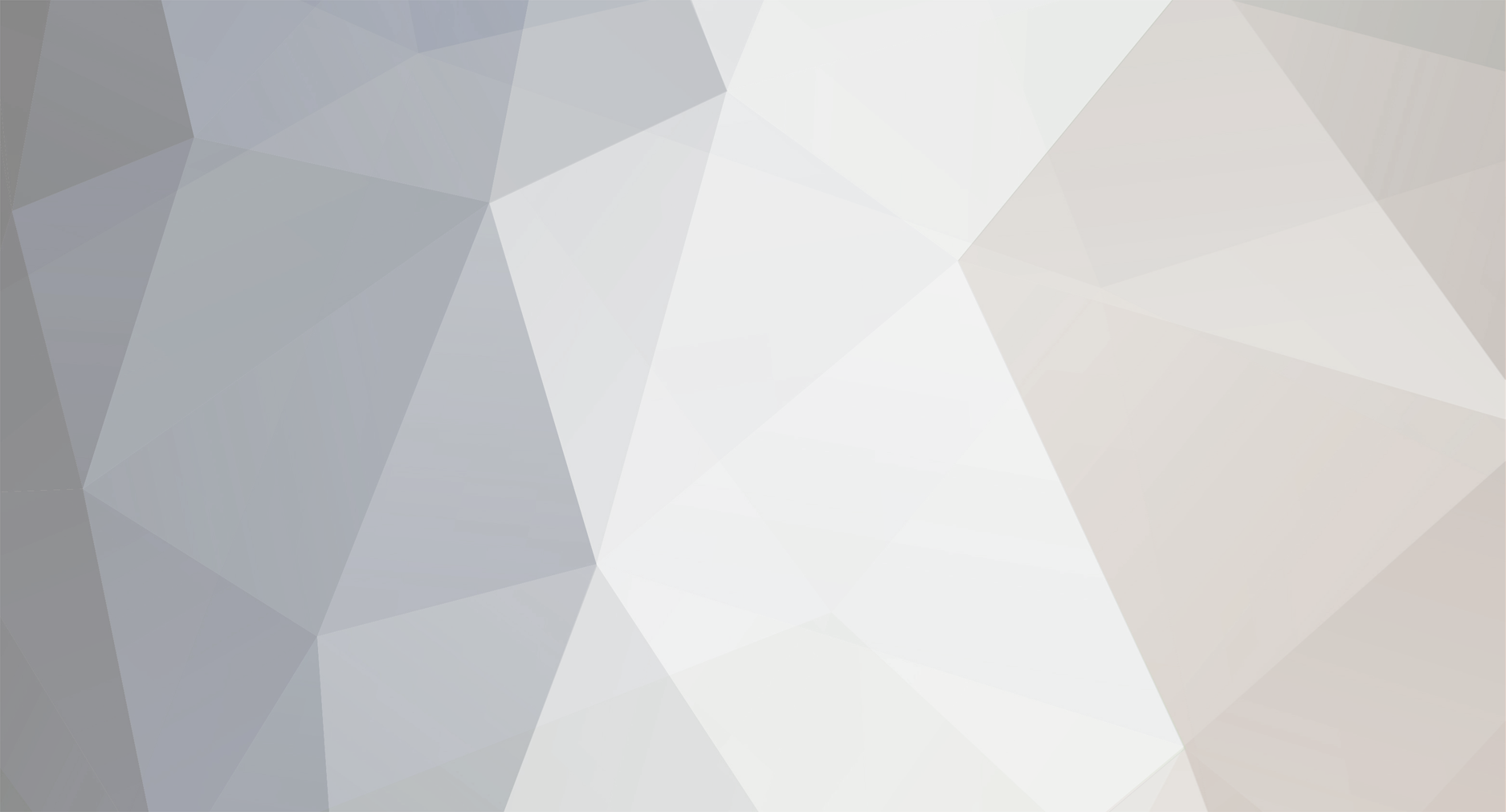
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
This cannot be done because variable names must be strings, they can't be arrays, that just doesn't make any sense. That's like trying to do this: $arr = array('something'); $$arr = 'something'; // Is the same as.. $array('something') = 'something'; Which makes no sense at all.
-
Forgot a closing ), sorry. $class = (strpos($_SERVER['PHP_SELF'], 'about.php')) ? 'active' : null;
-
When in doubt check the manual
-
You can't have nested PHP tags.. <?php $file = file('data.txt'); if(strpos($lines[4], 'about') !== false) { $class = (strpos($_SERVER['PHP_SELF'], 'about.php') ? 'active' : null; echo "<li class='$class'><a href='about_us.php'>About Us</a></li>"; } ?>
-
Given the choice I'd choose Java, primarily because if taught correctly you should learn a great deal about OOP.
-
There's no error there, post your entire code.
-
$file = file('data.txt'); if(strpos($file[4], 'about') !== false) { echo "<li>About</li>"; } file strpos
-
Although it is being stereotypical, I don't really see a problem with it. There are stereotypes for a reason. Typically the first thing anyone joining a forum community would see is your posts, not your profile, or age if you happen to have it public. I'm 16 years old and I've been part of many forum communities for many years, and I'd like to think I maintain a respectable reputation. Even though there might be stereotypes in this situation, the most important thing is how you represent yourself. You're not going to be type-casted into a group in which you do not belong.
-
$file = file('filename.txt'); $key = array_search('weruewu|dsifgsd|ewrtet', $file); if($key) unset($file[$key]); file_put_contents('filename.txt', implode("\n", $file)); file array_search unset file_put_contents
-
The benefit of escaping your $_POST variables, or any other user input that will go into a mysql query for that matter, with mysql_real_escape_string is to prevent SQL injections which can be very harmful to your website.
-
You start by rethinking your logic. Having to use exit, die or any functions alike usually tells you that you have a problem with your coding logic. First off, I wouldn't suggest trying to keep your entire website within 1 page, this is just going to clutter thing without benefits. If you must, why don't you just use a switch? switch($_GET['something']) { case 'this': break; case 'that': break; default: // Invalid page ID or whatever.. break; } // Footer
-
Preform mysql_real_escape_string on all data before it's put into the mysql query.
-
edit: Lol, it appears you found a helper from another framework (I was gonna suggest that as well, codeigniter has one, but I checked it out and it would hard to edit to your needs). It's okay, no harm done, had nothing else to do anyway . -- I couldn't find it :-\ I decided to make a basic Form generator class anyway (didn't have anything better to do), maybe it'll something to start with. With something this simple it's hard to find a balance between making versatile enough and still making it easier than just manually writing out the entire form, but hopefully this does that for you. I'm sure there's room for improvement, it's just something that I whipped together really quick. Class: class Form { private $_form, $_xhtml, $_elements = array(); public function __construct($action, $method, $other = array(), $xhtml = true) { $this->_xhtml = $xhtml; foreach(array_merge(array('action' => $action, 'method' => $method), (array) $other) as $attrib => $val) { $attribs[] = "$attrib='$val'"; } $this->_form = "<form " . implode(' ', $attribs) . ">\n"; } public function add() { $elements = func_get_args(); $this->_elements = array_merge($this->_elements, $elements); } public function output() { $this->build(); echo $this->_form; } private function build() { foreach($this->_elements as $element) { $attribs = array(); foreach($element as $attrib => $val) { $attribs[] = "$attrib='$val' "; } $xhtml = ($this->_xhtml) ? '/' : null; $this->_form .= "<input " . implode($attribs) . $xhtml . "><br " . $xhtml . ">\n"; } $this->_form .= "</form>\n"; } } Examples: #1: Basic usage $form = new Form('', 'post'); $form->add(array( array( 'type' => 'text', 'name' => 'username', 'value' => 'default value' )) ); $form->output(); When instantiating the Form object you pass the action and the method, then you have 2 optional arguments. The first optional argument is an array that will hold additional attributes you want to apply to the form tag (the same way you list attributes for the add() method). If you want to leave this out but still pass the 4th argument simply pass null. The last optional argument defaults to true and just tells the class whether or not to use XHTML (Just changes things like <input ... > to <input ... /> and <br> to <br /> to comply with XHTML standards). output: <form action='' method='post'> <input type='text' name='username' value='default value' /><br /> </form> #2: Adding multiple elements at once: $form = new Form('', 'post'); $form->add( array( 'type' => 'text', 'name' => 'username', 'value' => 'default' ), array( 'type' => 'text', 'name' => 'password' ), array( 'type' => 'submit', 'name' => 'submit', 'value' => 'Submit!' ) ); $form->output(); Optionally if you wish to add multiple inputs at the same time you can simply by adding other parameters each containing an array to represent each element (the same way as in the first example). Output: <form action='' method='post'> <input type='text' name='username' value='default' /><br /> <input type='text' name='password' /><br /> <input type='submit' name='submit' value='Submit!' /><br /> </form> -- Now this gives you a decent amount of control, you can add attributes like classes to elements to format them using CSS, but if you want even more control like adding wrappers you can also add that functionality.
-
I actually made a class specifically for this when I was bored one day. I guess it turned out not to be so useful in the long run because I don't use it anymore, but I suppose that could be because I don't use too many forms, and even when I do on larger projects I work with my design partner who does all of the html anyway.. I'll do a search and see if I can find it..
-
Rising of oceans now apparently caused by god's tears..
Alex replied to 448191's topic in Miscellaneous
When it comes to interacting with our environment and the impact that we have on the earth we're much more than just ants. -
Taking another look over your code, I noticed some other errors. So I decided to rewrite it (somewhat) for you and explain it a little better. Hopefully this will teach you something for future use. <html><head><title>Adding a User </title></head> <body> <?php if(!isset($_POST['submit'])) { echo <<<FORM Please enter all new user details <form action="" method="post"> First Name: <input type="text" name="firstname"><br> Last Name: <input type="text" name="lastname"><br> UserName: <input type="text" name="username"><br> PassWord: <input type="text" name="password"><br> <input type="submit" name="submit" value="Submit"> </form> FORM; } else { $conn = mysql_connect("mysql4.000webhost.com")or die("Try Again Punk"); $db = mysql_select_db("a3213677_snorre",$conn)or die("NOOPE"); $sql = "insert into `User Table` (first_name,last_name,user_name,password) values('{$_POST['firstname']}','{$_POST['lastname']}','{$_POST['username']}',password('{$_POST['password']}'))"; $result = mysql_query($sql,$conn) or trigger_error(mysql_error()); if($result) { echo("New User $username ADDED DUH!"); } } ?> </body></html> It's much easier to write a block of html using the heredoc syntax. You had a problem with a missing " which is why it wasn't working before. You most likely missed this because it's hard to see when you're escaping tons of quotes. This way you can write the form like you normally would in any html document. I also removed all the value="..."s because assuming this works you'll never be submitting data in a way that it'll be redisplayed on the form (Unless you add validation, which you don't currently have, but I'd suggest). I also removed the $PHP_SELF, because using this leaves you open to XSS attacks. It's a better idea to just leave the action attribute empty, or just type in the name of the file (if you want it to validate) if you want to submit a form back to the same page. Finally I gave the submit button a name of 'submit', this way you can just use if(!isset($_POST['submit'])) { ... } to see if the form was submitted. ( isset ). Hopefully this helps. ( And for future reference it's a better idea to keep this inside of the help topic, and not over pms ).
-
how do I write to the end of the first line of a flat text file
Alex replied to husslela03's topic in PHP Coding Help
file_put_contents is only available in PHP 5+, instead you'll have to use fopen, fwrite and fclose. $fp = fopen('somefile.txt', 'w'); fwrite($fp, $text); fclose($fp); -
Sorry, I left an extra ( when I edited your code. <html><head><title>Adding a User </title></head> <body> <?php if( !isset($_POST['username'], $_POST['password'], $_POST['lastname'], $_POST['firstname']) ) { $form ="Please enter all new user details"; $form.="<form action=\"$PHP_SELF\""; $form.=" method=\"post\">First Name: "; $form.="<input type=\"text\" name=\firstname\""; $form.=" value=\"$firstname\"><br>Last Name: "; $form.="<input type=\"text\" name=\"lastname\""; $form.=" value=\"$lastname\"><br>UserName: "; $form.="<input type=\"text\" name=\"username\""; $form.=" value=\"$username\"><br>PassWord: "; $form.="<input type=\"text\" name=\"password\""; $form.=" value=\"$password\"><br>"; $form.="<input type=\"submit\" value=\"Submit\">"; $form.="</form>"; echo($form); } else { $conn = mysql_connect("mysql4.000webhost.com")or die("Try Again Punk"); $db = mysql_select_db("a3213677_snorre",$conn)or die("NOOPE"); $sql = "insert into `User Table` (first_name,last_name,user_name,password) values('{$_POST['firstname']}','{$_POST['lastname']}','{$_POST['username']}',password('{$_POST['password']}'))"; $result = mysql_query($sql,$conn)or die ("NOT EVEN"); if($result) { echo("New User $username ADDED DUH!"); } } ?> </body></html>
-
By just reloads the page do you mean that it's still displaying the form? That would mean that it's not even getting to the mysql insert, which could be why it's not working. $username, $password, won't even be set unless register_globals is enabled on your server (which is a bad idea..). Try this: <html><head><title>Adding a User </title></head> <body> <?php if( (!isset($_POST['username'], $_POST['password'], $_POST['lastname'], $_POST['firstname']) ) { $form ="Please enter all new user details"; $form.="<form action=\"$PHP_SELF\""; $form.=" method=\"post\">First Name: "; $form.="<input type=\"text\" name=\firstname\""; $form.=" value=\"$firstname\"><br>Last Name: "; $form.="<input type=\"text\" name=\"lastname\""; $form.=" value=\"$lastname\"><br>UserName: "; $form.="<input type=\"text\" name=\"username\""; $form.=" value=\"$username\"><br>PassWord: "; $form.="<input type=\"text\" name=\"password\""; $form.=" value=\"$password\"><br>"; $form.="<input type=\"submit\" value=\"Submit\">"; $form.="</form>"; echo($form); } else { $conn = mysql_connect("mysql4.000webhost.com")or die("Try Again Punk"); $db = mysql_select_db("a3213677_snorre",$conn)or die("NOOPE"); $sql = "insert into `User Table` (first_name,last_name,user_name,password) values('{$_POST['firstname']}','{$_POST['lastname']}','{$_POST['username']}',password('{$_POST['password']}'))"; $result = mysql_query($sql,$conn)or die ("NOT EVEN"); if($result) { echo("New User $username ADDED DUH!"); } } ?> </body></html>
-
Are you getting your success message? If it's not updating the database try this: $result = mysql_query($sql,$conn) or trigger_error(mysql_error()); Is your table named 'User Table' (It is case-sensitive)?
-
You had some mistakes with your quotes when defining your $sql variable. Try: $sql = "insert into User Table(first_name,last_name,user_name,password) values('$firstname','$lastname','$username',password('$password'))"; I'd also suggest you don't use the MySQL PASSWORD() function to store your passwords. It has changed in the past and it's a possibility that it will change again, and if it does it would break your application.
-
That's because the port isn't open. To check to see if it's actually online to eliminate your code as the problem use a port checker
-
What's the format that data is stored in the two files? Can you post an example? I'd also suggest you use a select input for hardback/paperback instead of having them enter h or p, it's much more user-friendly.
-
Rising of oceans now apparently caused by god's tears..
Alex replied to 448191's topic in Miscellaneous
I hate when people talk about global warming like this. "It's colder than last year, GLOBAL WARMING MUST BE FALSE". Global warming doesn't (for the most part) happen at a rate so that you can notice it from year-to-year. You have to look at the trends from the last couple hundreds of years. If it was happening at such a rate that you can really say it's noticeably warmer overall this year than last year (and not just from normal temperature variations) we would already be dead. -
lmao. I've had the same thing happen to me before. Not all clients are bad, but some are hell. One client in particular that I worked with was a nightmare. We (my partner and I) had a client complain about how the space between two letters in the logo was too big. Meanwhile it was just the normal spacing of that font (that he suggested). He had us actually manually move the letter in the image over 1px. There were tons of other ridiculous things that he had us do, but that's the one that sticks out in my head.