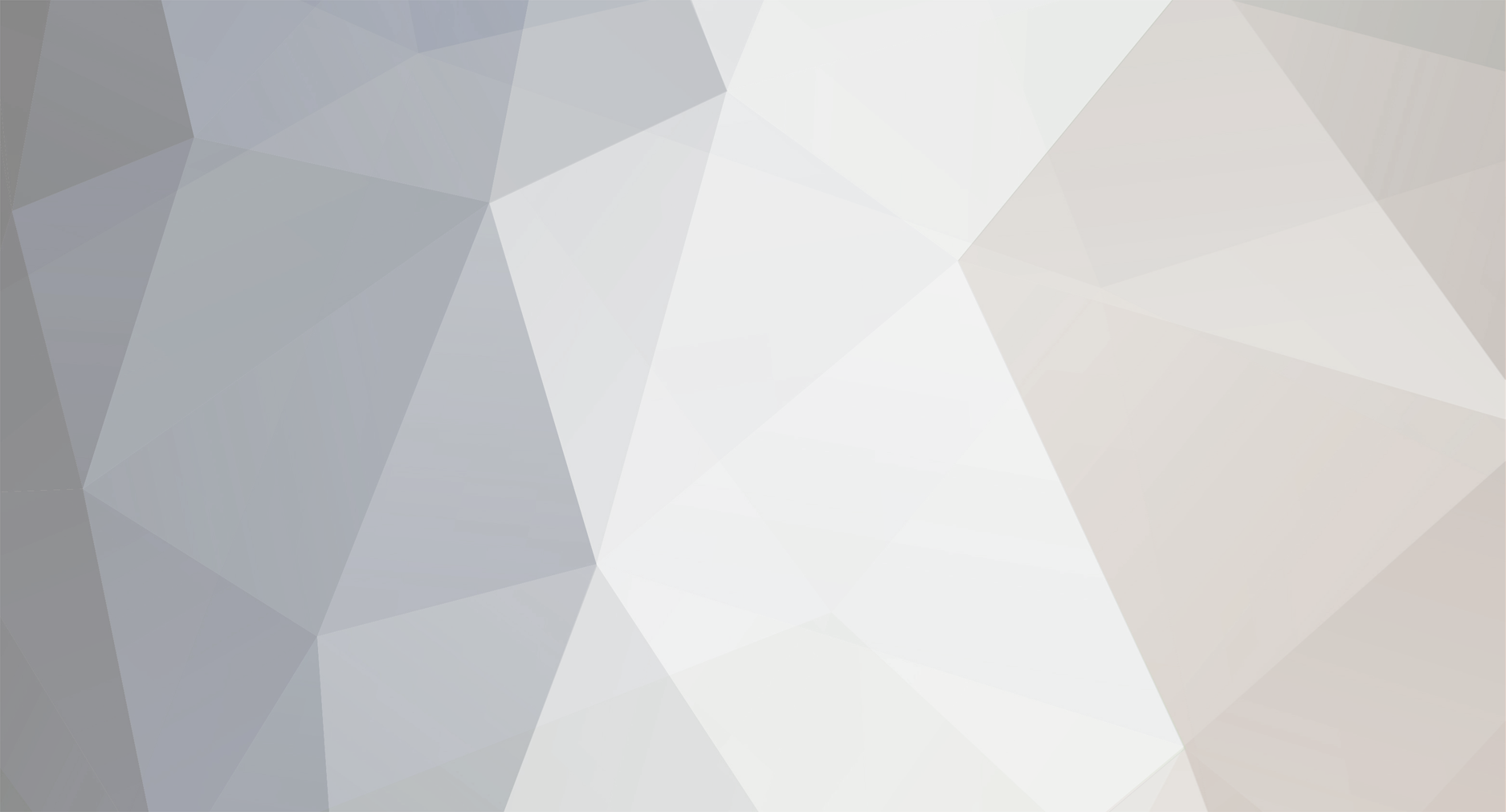
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
Why don't you just use a for loop? Example: $loops = 8; for($i = 0;$i < $loops;$i++) { // ... }
-
Parse error: syntax error, unexpected T_CONSTANT_ENCAPSED_STRING
Alex replied to phpnewbieca's topic in PHP Coding Help
I think he's trying to point out the line where the error message says the error is. The actual problem is on the line before it: if ($AddName'] == "Add Name") { You have '] which shouldn't be there. if ($AddName == "Add Name") { -
Here's one way you can do it with percentages: $items = array( array('lint', 40), array('copper coin', 30), array('silver coin', 20), array('gold coin', 10) ); function pickItem($items) { $hat = array(); foreach($items as $item) { $hat = array_merge($hat, array_fill(0, $item[1], $item[0])); } return $hat[array_rand($hat)]; } Example: $picks = 500; for($i = 0;$i < $picks;$i++) { $picked[] = pickItem($items); } $counts = array_count_values($picked); arsort($counts); foreach($counts as $item => $freq) { echo "$item was picked $freq times (" . ($freq / $picks * 100) . "%)<br />\n"; } Output: lint was picked 195 times (39%) copper coin was picked 155 times (31%) silver coin was picked 96 times (19.2%) gold coin was picked 54 times (10.8%)
-
Of course you can. The browser doesn't care if it's hard-coded HTML or dynamic HTML spit-out by PHP, it's all the same.
-
Parse error: syntax error, unexpected T_STRING
Alex replied to phpnewbieca's topic in PHP Coding Help
Your error is on this line: if($Graphic Card) { You have a space there that shouldn't be. The variable you're trying to use is $GraphicCard -
Very interesting topic, I'm looking forward to seeing some of the responses. #1: I tend to go with method #1a, I just format it a bit differently. I tend to do something like this (especially when there are a lot of properties): class Foo { public $myVar1, $myVar2; .... } #2: I generally stick to #2a and follow the naming conventions, but I have to admit I sometimes do deviate from this just because I don't like it that much, and I don't find it to be too helpful. I tend to only do this on quick classes/projects, though. #3: I always follow #3a and never omit the visibility keyword when declaring public methods, it would look too sloppy and inconsistent for my coding OCD to handle. :-\ #4: I follow #4a and name them correctly because it's just more trouble not to, and it's really just a habit now so it's not really something that I would even consider not doing.
-
You just want to get the contents of a file and echo it out? Use file_get_contents: echo file_get_contents('filename.txt');
-
I'd like to follow the Zend standard, but I'm really stubborn about a few things, in particular, this. I wouldn't have a problem changing the way I do some things to adhere to the standard, like the space before the parenthesis after if. But I figure there's no point in following only some of the standard. I guess the reason I'm so stubborn about this is because when I program in lower level programming languages like C++ I find this use of (the second method) bracket formatting especially helpful in terms of readability. I guess there's 2 main reasons: 1) I find that it just keeps things less crowded, easier to read in general. 2) It makes matching opening an closing brackets much easier. I find it's easier to visually match the opening and closing brackets when they're both at the same horizontal position. I've seen, and worked on a few projects for some larger companies that actually required small things like this. For example they might have something like (Just an example in PHP, because the projects that I'm actually referencing happened to be in C++): if ( $variable == $anothervar ) { $this->something ( $variable ); } I just don't happen to see how those spaces really improve readability, but that's just my opinion.
-
Yes, it's important that you're spelling the super-global correctly. It is also important that you place session_start at the top of every page that you wish to access the $_SESSION super-global from. Example: page 1: session_start(); $_SESSION['something'] = 'val'; page 2: session_start(); // You need this echo $_SESSION['something']; // val
-
For me it's the second method all the way. I just think it's much easier to read and nicer to look at, especially when things get complicated and involved.
-
I think the left looks better.
-
You can change this line: document.getElementById("inputs").innerHTML += '<input type="text" name="name" /><br />'; To something like this: document.getElementById("inputs").innerHTML += '<input type="text" name="name_' + i + '" value="' . i + '" /><br />'; To make the names like: name_0, name_1, and the value would be the number.
-
If you're only wanting to dynamically create input elements there's no need for PHP at all. Here's an example: <script type="text/javascript"> function makeInputs(number) { document.getElementById("inputs").innerHTML = ''; for(var i = 0;i < number;i++) document.getElementById("inputs").innerHTML += '<input type="text" name="name" /><br />'; } </script> <form method="" action="" onsubmit="return false"> <select id="number" name="number" onchange="makeInputs(this.value)"> <option value="1">1</option> <option value="2">2</option> </select> </form> <div id="inputs"> </div>
-
You can use preg_split to split a string by multiple delimiters. Example: <?php $str = "john + 10/20/2008 - 10/30/2008"; $var = array_map('trim', preg_split('~\+|\-~', $str)); echo $var[0]; // john echo $var[1]; // 10/20/2008 echo $var[2]; // 10/30/2008 ?>
-
Try: echo $result_array[0]->salt;
-
Why would you create a function like that? All it does is use mysql_real_escape_string.. Why not just use it directly? That's a really pointless function. Anyway, your problem is probably because of your html syntax errors. You're missing a bunch of equal signs (=). Example: class"clearfix" .. name"firstname"
-
Check out this page Basically, if the methods are public/protected they will be inherited, but if they're private they will not.
-
First off, if you're using the keyword private in your user class any class that extends it will not inherit it. Instead, for this purpose, you should use protected. To add to the profile array in the classes that extend user you can user array_merge. Example: class user { protected $profile = array ('userID', 'firstname', 'surname'); } class primaryUser extends user { public function __construct() { $this->profile = array_merge($this->profile, array ('a', 'b')); print_r($this->profile); } } Output: Array ( [0] => userID [1] => firstname [2] => surname [3] => a [4] => b )
-
Didn't really answer my question. How are players eliminated? If they lose once they should be removed or what?
-
Each set of matches that gets played there should be half as many players right? How do you decide who gets removed if each player has 2 matches per round?
-
You could do something like this: $targets = array("Andy","Jan","Alex","Sue"); $available = $targets; foreach($targets as $target) { do { $rand = $targets[array_rand($available)]; } while ($rand == $target || $pairs[$rand] == $target); $pairs[$target] = $rand; $index = array_search($rand, $available); unset($available[$index]); } foreach($pairs as $player1 => $player2) { echo "$player1 matched with $player2<br />\n"; } Example output: Andy matched with Sue Jan matched with Alex Alex matched with Andy Sue matched with Jan
-
You're forgetting a period: ...<img src=\"" . amazon_display_thumbnail($prodID) . "\">"...
-
Or $next = end(array_keys($arr)) + 1; This will not work as you want if the element that you unset is the last one though (I assume this isn't a problem for what you're doing), same with Daniel's.
-
You forgot $row[...] $ttname = $row['tname']; $ttpreview = $row['preview']; $ttprice = $row['price'];
-
Well just doing $name = "array[0][1][1]"; doesn't make $name an array, it's just a string. This might be totally in the opposite direction from what you're looking for, but if you want to have a variable that you can edit that will also edit $array[0][1][1] you can pass that by reference. Example: $array[0][1][1] = 'value'; $name = &$array[0][1][1]; $name = 'Something'; echo $array[0][1][1]; // Something