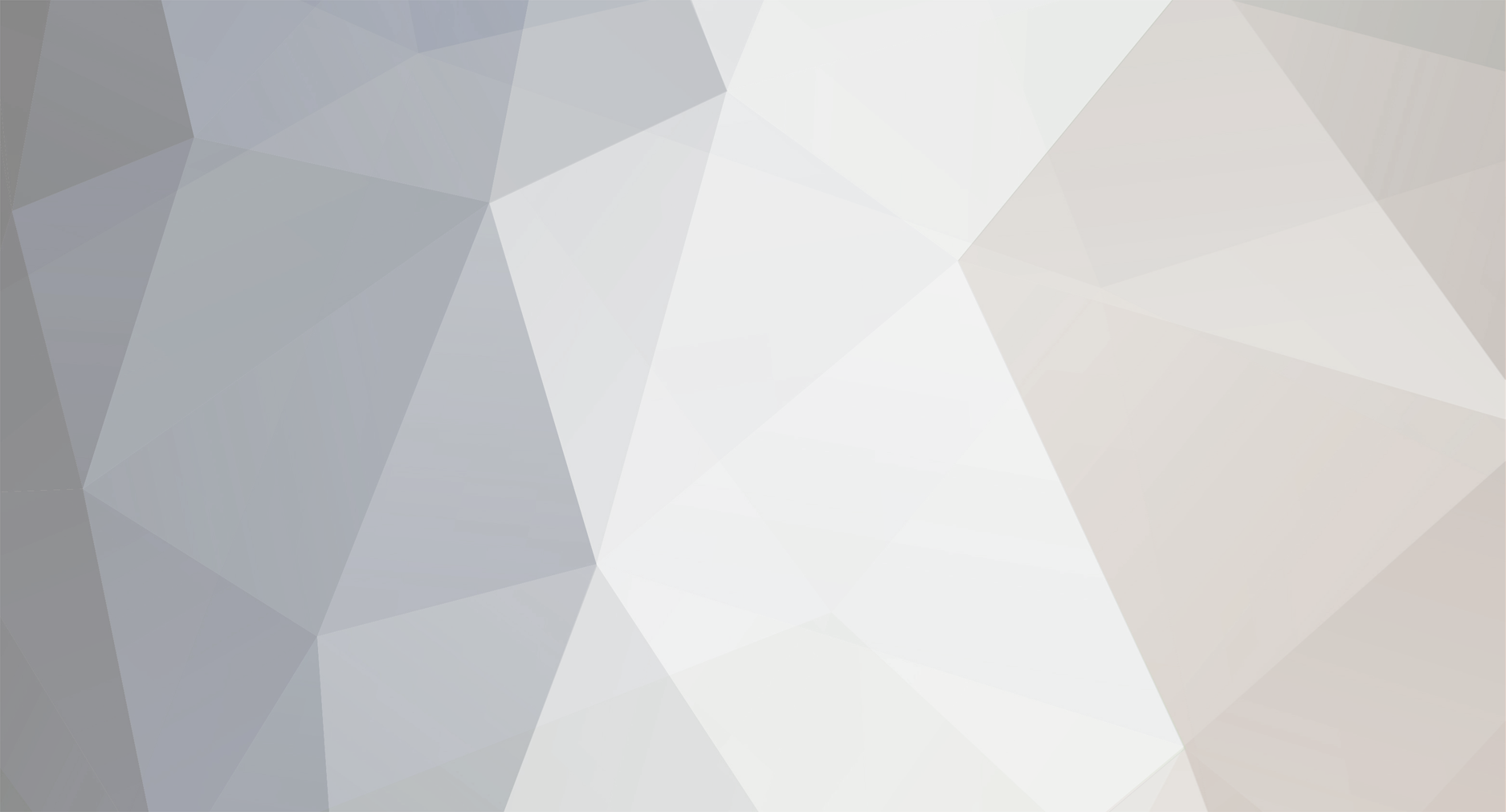
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
<?php // get Current pri value $db = mysql_connect("ip","user","pass") or die("Couldn't connect"); mysql_select_db("database",$db) or die("Couldn't select database"); $query="SELECT `priority` FROM `calls` WHERE `worksheet` = '$worksheetnum'"; $result=mysql_query($query); $num=mysql_numrows($result); $i=0; while ($i < $num) { $priority=mysql_result($result,$i,"priority"); $i++; }; $checked = ($priority == 1) ? 'checked="checked"' : NULL; echo '<input name="priority" type="checkbox" id="priority" value="1" ' . $checked . '>'; ?>
-
You shouldn't have session_start() twice. And since there's one inside of header.php which you're including this is causing your error.
-
Wrong, session_start() must be placed on every page that you wish to access the super-global $_SESSION.
-
Yes, it's completely possible and it's been done many times. There are many open-source versions available and I've even made a one myself before.
-
Sounds like you need what mattal999 suggested along with some regex to parse the file and get exactly the part you want out of it.
-
GetVar isn't a standard PHP Function, and $item_id isn't even used (from what I can see) in that code at all.
-
thanks jackass! I wasn't trying to be rude; you can't ask a question that would be specific to your code then not post any code..
-
How would we know if a missing ' or } could be throwing everything off if you haven't posted any code?
-
Just replace like '$_POST[username]', with '{$_POST[username]}', Do that for all of the arrays.
-
Need rapidshare account for 1-2 weeks (You WILL be paid back)
Alex replied to Cory94bailly's topic in Miscellaneous
Oh, well I thought you were planning on doing it another another way. Either way I doubt these forums would appreciate this type of thing. -
You're messing up your quotes. Anything inside of single quotes (' ') will parsed as a string, not a variable. echo '<OBJECT ID="MediaPlayer" WIDTH="192" HEIGHT="190" CLASSID="CLSID:22D6F312-B0F6-11D0-94AB-0080C74C7E95" STANDBY="Loading Windows Media Player components..." TYPE="application/x-oleobject"> <PARAM NAME="FileName" VALUE="' . $imageurl . '"> <PARAM name="autostart" VALUE="false"> <PARAM name="ShowControls" VALUE="true"> <param name="ShowStatusBar" value="false"> <PARAM name="ShowDisplay" VALUE="false"> <EMBED TYPE="application/x-mplayer2" SRC="' . $imageurl . '" NAME="MediaPlayer" WIDTH="600" HEIGHT="450" ShowControls="1" ShowStatusBar="0" ShowDisplay="0" autostart="0"> </EMBED> </OBJECT>';
-
Need rapidshare account for 1-2 weeks (You WILL be paid back)
Alex replied to Cory94bailly's topic in Miscellaneous
I'm fairly certain that you can't do this; otherwise they're securing their files stupidly, which I doubt since they're such a big website. -
This is a PHP question, not HTML. And that's not very secure, but to do what you asked it would be like this: <?php if($_POST['password'] == 'somepassword') header('Location: mywebsite.com/hiddenpage4569.php'); ?>
-
You should use an AUTO_INCREMENT field
-
There are timers in JavaScript that are easy to use. Read more here You might need AJAX as well; depending on if you meant that a .php file needs to be ran when the update image is pressed or when the page loads.
-
That's not a match.
-
I haven't noticed much of a change.
-
$finfo = finfo_open(FILEINFO_MIME_TYPE); $mime = finfo_file($finfo, $_FILES['file']['tmp_name']); finfo_close($finfo); $mime will then contain the mime file type (ie. application/msword for .doc) To see what the other mime types for the other file types you want to allow here's a reference: http://www.w3schools.com/media/media_mimeref.asp
-
<?php $phrases = Array('Phrase A', 'Phrase B', 'Phrase C', 'Phrase D', 'Phrase E', 'Phrase F', 'Phrase G'); $out = Array(); for($i = 0;$i < 5; $i++) { $key = array_rand($phrases); if(!in_array($phrases[$key], $out)) { $out[] = $phrases[$key]; unset($phrases[$key]); } } echo implode('<br />', $out);
-
Here's a useful class I wrote: class Image { private $image, $ext, $file; const CENTER = 0, CENTER_LEFT = 1, CENTER_RIGHT = 2, TOP_CENTER = 3, TOP_LEFT = 4, TOP_RIGHT = 5, BOTTOM_CENTER = 6, BOTTOM_LEFT = 7, BOTTOM_RIGHT = 8; public function __construct($im=NULL) { if(empty($im)) return true; $info = @getimagesize($im); $backtrace = debug_backtrace(); if(!$info) return die('<b>Fatal error:</b> \'' . $im . '\' is not a valid image resource on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $ex = explode('/', $info['mime']); $this->ext = $ex[1]; $func = 'imagecreatefrom' . $this->ext; $this->image = @$func($im); $this->file = $im; return true; } public function setImage($im) { $info = @getimagesize($this->file); $backtrace = debug_backtrace(); if(!$info) return die('<b>Fatal error:</b> \'' . $im . '\' is not a valid image resource on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $ex = explode('/', $info['mime']); $this->ext = $ex[1]; $func = 'imagecreatefrom' . $this->ext; $this->image = @$func($im); $this->file = $im; return true; } public function resize($width, $height, $porportional=FALSE, $percent=NULL, $max=NULL) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->resize, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $info = Array(imagesx($this->image), imagesy($this->image)); if($porportional) { if(empty($max) && empty($percent)) return false; if(empty($max)) { $new_width = $info[0] * ($percent/100); $new_height = $info[1] * ($percent/100); } else { if($info[0] < $max && $info[1] < $max) return false; $new_width = ($info[0] > $info[1]) ? $max : ($info[0]/$info[1]) * $max; $new_height = ($info[0] > $info[1]) ? ($info[1]/$info[0]) * $max : $max; } } else { $new_width = $width; $new_height = $height; } $new_image = imagecreatetruecolor($new_width, $new_height); imagecopyresampled($new_image, $this->image, 0, 0, 0, 0, $new_width, $new_height, $info[0], $info[1]); $this->image = $new_image; return true; } public function crop($width, $height, $x, $y, $position=NULL) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->crop, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $info = Array(imagesx($this->image), imagesy($this->image)); if($width > $info[0] || $height > $info[1]) return false; if(!empty($position) && $position >= 0 && $position <= { switch($position) { case CENTER: $x = ($info[0] - $width)/2; $y = ($info[1] - $height)/2; break; case CENTER_LEFT: $x = 0; $y = ($info[1] - $height)/2; break; case CENTER_RIGHT: $x = ($info[0] - $height); $y = ($info[1] - $height)/2; break; case TOP_CENTER: $x = ($info[0] - $width)/2; $y = 0; break; case TOP_LEFT: $x = 0; $y = 0; break; case TOP_RIGHT: $x = ($info[0] - $width); $y = 0; break; case BOTTOM_CENTER: $x = ($info[0] - $width)/2; $y = ($info[1] - $height); break; case BOTTOM_LEFT: $x = 0; $y = ($info[1] - $height); break; case BOTTOM_RIGHT: $x = ($info[0] - $width); $y = ($info[1] - $height); break; default: return false; break; } } $new_image = imagecreatetruecolor($width, $height); imagecopyresampled($new_image, $this->image, 0, 0, $x, $y, $width, $height, $width, $height); $this->image = $new_image; return true; } public function save($loc, $compression=100) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->save, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $func = 'image' . $this->ext; return $func($this->image, $loc, $compression); } public function output() { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->output, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); header('Content-type: image/' . $this->ext); $func = 'image' . $this->ext; $func($this->image); } } My class can do a lot of different things, so you'd need to be specific for me to help you on exactly what you need to image to be resized to (proportional, max width, max height, whatever..). Unless you can look at the resize function and figure it out yourself. Here's an example of something you can do: //include my class $image = new Image($_FILES['file']['tmp_name']);// $_FILES['file']['tmp_name'] is the file being uploaded $image->resize(width, height); $image->save('path/to/save/location');
-
My name is Alex, I live on Long Island right outside of NYC and I'm 16 years old (Since Sunday!). I got interested in programming (more specifically, web development) a couple years ago through a friend who was into that kind of thing. I originally started with HTML and CSS, but I never really liked that too much; soon after I moved onto PHP & MySQL. Over the past few years I've dipped into a bunch of other programming languages like C++, JavaScript and AS3 (Which I actually just got back into more seriously). I'm constantly studying programming languages and always trying to learn more. Currently it's just a hobby, although I do make money from freelancing here and there I'm currently still in high school, I'm a junior (11th grade). I have no idea where I'm going to college yet, or even what I want to major in. I'm extremely indecisive which doesn't help at all and it's getting close so that's pretty stressful. Recently I've been considering a job in computers, and studying for a degree in computer science; but I see programming more of just a hobby for me at the moment.
-
$checkbox = 1; while ($row = mysql_fetch_assoc($query)){ $subject = $row['subject']; $message = $row['message']; $from = $row['from']; $when = $row['when']; echo "<tr><td>$checkbox - $subject - $message</td></tr>"; $checkbox++; } $var++; is used to increment a number.
-
It's always good to read the guide when uncertain, header()
-
Just add http:// in front of what I posted before. <?php echo "<a href='http://{$row_Recordset1['website']}'><img src=http://www.entertainfortwayne.com/images/home/".$row_Recordset1['logo'] ."></a> "; ?>
-
What's an example of what the website row in the database contains?