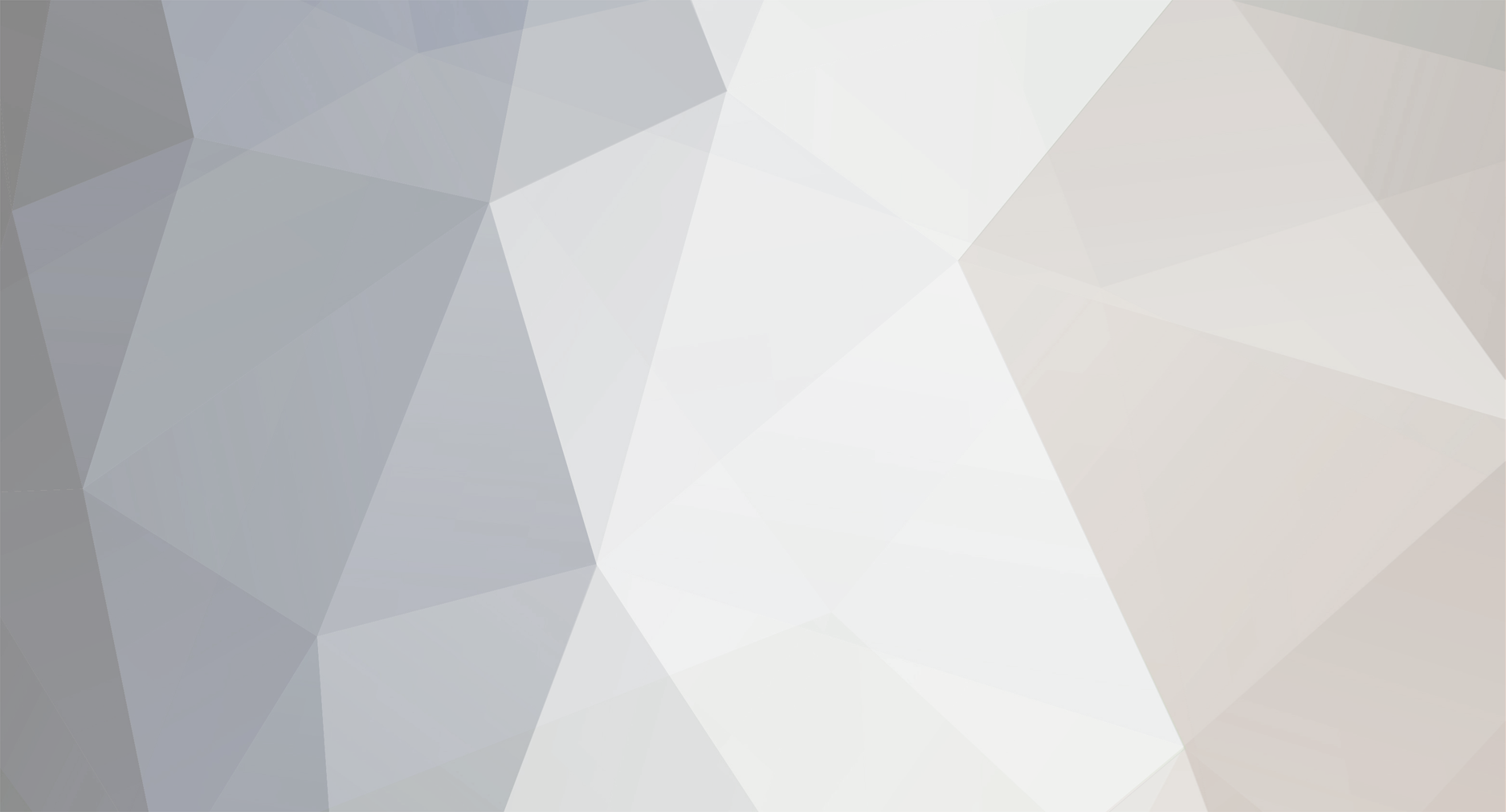
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
The reason that the three-character codes were deprecated is because they are not representative of the entire regions that they (supposedly) cover. Even in America, there are some places that do not recognize Daylight Saving Time, and some that use a different offset.
-
Converting Values Inside An Array Into A String
DavidAM replied to pioneerx01's topic in PHP Coding Help
Variables are not interpreted inside single-quoted strings. You would use double-quotes here. However, you do not need quotes there at all. -
When the callback function returns false, array_filter will discard the value being processed. In your case, the value being processed is one of your nested arrays; not the scalar value with "too many decimals". You need to run array_filter on each of your nested arrays. Also, review that test condition; I don't think it is doing what you want/think.
-
Variables are not interpreted in single-quote delimited strings. Use double-quotes header("Content-Disposition: attachment;filename=$today.csv");
-
Checkboxes are only POSTed if they are actually checked. Since you have it as an array, you can get a list of the values from checked checkboxes using implode: if (isset($_POST['i_have'])) $i_haveField = implode(', ', $_POST['i_have']); else $i_haveField = '';
-
shuffle does not return the shuffled array. It modifies the array passed as a parameter. $maximum_integer = strip_tags(trim($_REQUEST['maximum_integer'])); $range = range(0,$maximum_integer); shuffle($range); $random_range_imploded = implode(', ', $range); print $random_range_imploded;
-
You should definitely use a single query with a JOIN and a single loop. SELECT players.id, players.username, players_killed.players_killed FROM players JOIN players_killed ON players.id = players_killed.playerid WHERE players_killed.players_killed > 100 LIMIT 50 You may want to through ORDER BY players_killed.players_killed DESC in there between the WHERE clause and the LIMIT clause.
-
You could try glob as in: $ArrayOfFileNames = glob('/path/to/images/dir/2012/*.{jpg,gif,png}', GLOB_BRACE); which might not be disabled, and eliminates the need for the preg_match. Then use foreach to walk the array of files. I can't remember right off if the filenames in the array will include the full path or just the names. Also, remember glob() will return false if it does not find any files that match
-
Paginate A Table In Multiple Databases (Php/mysql)
DavidAM replied to FishSword's topic in PHP Coding Help
Before you even think about implementing kicken's solution (which is correct, by the way); this question really must be answered. If it is a business requirement, then so be it; we've all had to work with less than ideal databases. But if it is a design flaw, it should be addressed as soon as possible. -
The OP is using fputcsv() so there would be a little bit more to do than just implode() and file_put_contents(). I agree though, the file open should be moved to before the do loop and the file close should be moved to after the do loop. I don't see any reason in that code to open and close it each time. I also do not see any reason to call clearstatcache or gc_collect_cycles. Since we don't know what "leads_query()" is doing, I could be wrong, but I see nothing in the presented code implying the need for it. Also, sleep requires an integer, you are passing it a floating point value. I don't see a need to sleep here, but if you want it, you need to use a 1 (one) not .5 (one-half). Most of those times are negative, but you don't show how you generated that value, so I don't understand what is going on there. I would be concerned about the consistent increase in memory use. By the way, you do realize that if leads_query() returns false, you are still going to try to process it as an array of data, right? I think I would rewrite that as: $fp = fopen($file_path, 'a'); $total_rows = 0; $start = memory_get_usage(); while ($rows = leads_query($total_rows)) { $total_rows += sizeof($rows); echo "Currently at Row: " . sprintf('%07d',$total_rows); foreach ($rows as $row) { fputcsv($fp, $row); } echo "\t\t".'Memory used ' . memory_get_usage() . "\t\t Change:" . (memory_get_usage() - $start) . "\n\r"; } fclose($fp); You might try profiling the leads_query() function to see if there is a bottleneck there. Does it open and close the database connection each time? (it should not). Do you free the query resource after retrieving it? (you should).
-
You are missing a quote mark after $Loses_Items. There may be others, it is difficult to read the line.
-
I did not see anything in the OP's posts about selecting a different image if the profile image is not present. If there are other images that need to be chosen when the "profile" image does not exist, we would need to know the structure and selection criteria in order to make a suggestion.
-
Move the AND condition into the LEFT JOIN LEFT JOIN tutor_images AS timg ON timg.tutor_id = tcs.tutor_id AND timg.image_type = 'profile' WHERE s.subjects LIKE '%business%'
-
1 - Turn on error reporting. Line 16 ($inc++) should be throwing an error unless you have it defined somewhere else. 2 - Initialize $inc before the while loop: $inc = 0; 3 - Why are you appending a newline onto the biblionumber and then imploding with another newline? That will put two newlines between each number, is this what you want? I would just do the implode with two newlines if that's what you want. Although the way you have it, there will be a newline after the last number, that my suggestion will not include. 4 - The implode is accessing $row, which is not an array at this point, so this line (#13) is throwing an error as well. I think you want $data here. At this point (after line 13), $bib is undefined, so it is useless to put it into a query. #4 ADDRESSES YOUR PROBLEM STATEMENT
-
The only thing in that script that jumps out at me, is that you are not escaping the username before you put it in the query string. This leaves you open to SQL injections, and query failures if someone uses a single-quote in their username. Build the query as: $query = "SELECT * FROM users WHERE username='" . mysql_real_escape_string($username) . "' AND password='$password'"; Note: I did not escape the password because it has been run through MD5 and cannot possibly contain any special characters. Otherwise, this script looks OK to me. The problem, I think, is at the beginning of your OTHER scripts. You said they all start with: Here you are retrieving the $username from $_POST which (probably) does not exist. You need to retrieve it from $_SESSION where you stored it in the login script.
-
Basically, the different pages (scripts) will each have to start a little differently. First, this needs to be at the top of every page-script (at least during development): error_reporting(E_ALL); ini_set('display_errors', 1); Second, on the script that is validating the login page; you check to see if the credentials are valid, then $_SESSION['username'] = <<The username of your logged in user>> Third, on pages that should only be accessed by a logged-in user: if (!isset($_SESSION['username'])) # kick them out Of course, the session_start() call needs to appear before you reference any of the $_SESSION variables.
-
Unless every page is POSTing the username from a form field, you are not getting the username on subsequent pages. You need to turn on error reporting, that line should throw a warning about an undefined index. Basically, in the script handling the login form, you need to collect the username (from POST) and store it in the session. On all other pages, you should collect the username from the session not from a POST field.
-
@Myddy_Funster: This code will not modify the contents of $topArray. In later versions of PHP (5.2+, I think), you can use a reference on $topValue to allow the modification of the array contents. i.e. foreach($topArray as $topKey => &$topValue){ Actually, it is get_magic_quotes_runtime that affects data read from a file. The _gpc version affects GET, POST, COOKIES, and FILES. I missed this in my earlier post; but I would put this check earlier, immediately after reading from the file, rather than after manipulating the values.
-
$sql = "INSERT ... VALUES (" . $array[0] . ", " . $array[1] . ", " ...; You have to make sure you put quotes around string data, and escape string data to protect against breaking the sql. Be advised that running one million rows this way will take a long time. You will likely need to remove or adjust the time limit (set_time_limit). You can make it faster, if you are using mySql, by inserting multiple rows at one time. I don't know what other database engines support this, but in mySql, you can do: INSERT INTO tablename (column1, column2, ...) VALUES (row1Column1, row1Column2, ...), (row2Column1, row2Column2, ...), (row3Column1, row3Column2, ...); Here is an example (I'm not going to type out your whole INSERT): $file = fopen("data.txt", "r") or exit("Fail!"); $insert = "INSERT INTO mytable (ID, FirstName, LastName, Age) VALUES "; $counter = 0; $values = array(); while(!feof($file)){ $string = fgets($file); $array = explode('|',$string); $values[] = sprintf("VALUES(%d, '%s', '%s', %d)", $array[0], mysql_real_escape_string($array[1]), mysql_real_escape_string($array[2]), $array[3]); $counter++; if ($counter >= 100) { $sql = $insert . implode(',', $values); mysql_query($sql); $values = array(); $counter = 0; } } ## Oops, almost forgot. If the file does not have a multiple of our check count (100) ## There will be values left in the array that need to be written: if ($counter > 0) { $sql = $insert . implode(',', $values); mysql_query($sql); } fclose($file); Of course, it looks like you have a delimited file there. If the string values are already quoted, you can import it directly into mySql just like you would a CSV file, by specifying the delimiter and quote characters. Also, if the string data is already quoted in the file, you are going to have to be careful. The example above added single quotes around the string data, so the quotes in the string data you read will be inserted into the database (probably not what you want). And depending on where the file came from, the quoted-string data my have been escaped for the quotes that were used, which may then be escaped by mysql_real_escape_string(), resulting in the escape character becoming part of the data in the database. As you write this, you should stop after the first 100 rows and thoroughly check the database to be sure you get what you expect. I would do the same check after the first 1000 records.
-
At the time that that Javascript executes, the SUBMIT button does not exist (yet), so there is nothing to attach that function to. You need to wait until the document is finished loading. I usually use: /* When the page has finished loading, initialize any Javascript */ $(window).load(pageInit); function pageInit() { // Put your page initialization code here $('input#submit').on('click', function() { alert(1); )} }
-
Glad to hear it. Please click the Topic Solved button so others will know it is solved. (Is it bad form to quote yourself?) I really feel like I should clarify the "not too big of a deal" statement: PHP has functions that let you retrieve the arguments passed to a function as an array, even if the function definition does not specifically name those parameters. As good programming practice, this is something that should be avoided, unless you have a need for unlimited parameters (see sprintf). I said "not too big of a deal" because it is not an error condition, and the code will run, but in this particular case, there was obviously a mismatch in parameters.
-
Yeah, I went through the "too many splitters" issue a couple of years ago. At that time there were two. The guy then re-routed one of the cables and there is now only one splitter; its a 4-way splitter in the house. It did make a difference at the time, but things have progressively gotten worse; both with the on-demand and the Internet. I don't know much about the hardware side of things, but everything he said makes sense. I've got a guy at work that knows the cable side pretty well, he's been out all day on a job, so I haven't been able to talk to him, yet.
-
You gotta watch those tax collectors. ... While three times zero is always still zero, they will somehow figure a way to make 35% of zero be some big positive floating-point value! Congratulations, Barand!
-
Have a look at the function definition and then look at where you are calling it: function user_active($username, $mysqli) { if(!user_active($username, $data, $mysqli, $command)) { The function definition has two parameters: Username and the mySql Object. However, when you call it, you are passing four parameters (which is not too big of a deal) but the second parameter you pass is not your mysql object (unless you are using some strange naming convention).
-
Thanks for the suggestions. I wasn't sure if there was some "magic" C library somewhere that could read something from the NIC card. I think I'll just stick with the DNS lookup. I've given it an array of domains I use regularly, and are not likely to go away. I rotate through the list, mostly because I wasn't sure if the router would cache anything. I don't think it does though. If I disconnect the router from the modem, it fails immediately. I am running dig +short domainname. Which prints a single line containing the IP address, and exits with a status of zero. I've noticed that if I disconnect the router, it still exits with zero, but does not output anything. If I shutdown the modem, it exits with a status of 9, and prints a message about the failure. I had the cable guys out today. They replaced several of the "F" connectors throughout the house, and told me that the cable in the wall that the modem is plugged into has some noise on it. They offered to replace it, at a cost of $50. I told them I'd do it myself. I'm pretty sure we have a spool of RG-6 at the shop, and one or two of my guys are capable of crimping it. Pulling it down the wall will not be a problem. The network is still bouncing up and down more than I like -- 8 times in the past 6 hours for a total of 50 times today (since midnight). Well, we will see what happens when I replace the cable tomorrow.