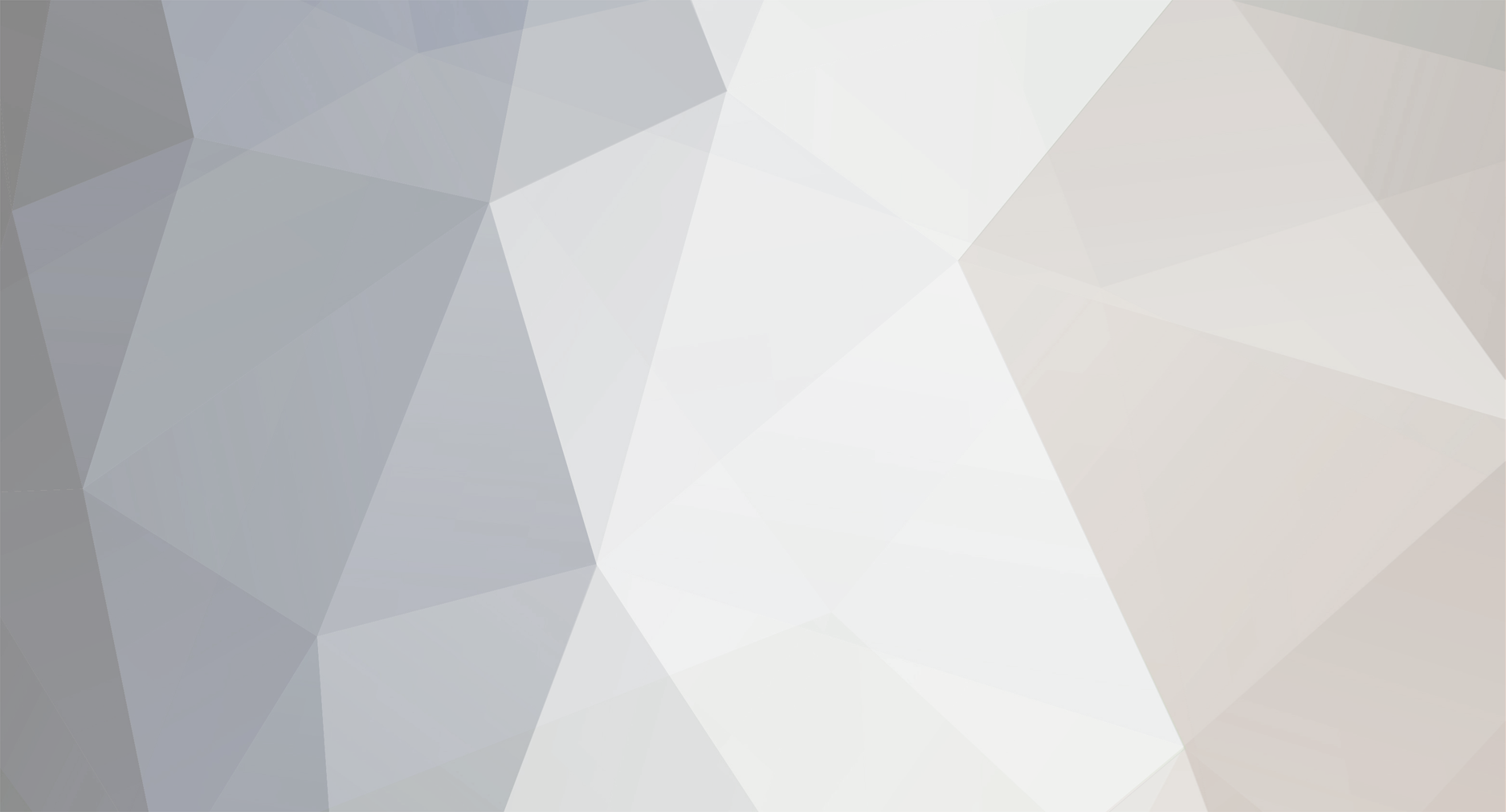
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
$count=mysql_num_rows($result); This line appears before you execute the query, in fact, it is before you have even connected to the database. This statement needs to be after you have executed the query. $sql="SELECT * FROM $tbl_name WHERE username=`$myusername` and password=`$mypassword`"; The backticks, `something`, indicate a column name to mySql, you need to use quotes (single or double) around the values (variables) for username and password.
-
Any given query will use only one index per table. So an index on domain will limit the number of rows to be read. It is not likely that any indexes that do not have domain as the first component will help in this situation. Just FYI. Fields used in the ON clause of a JOIN matter as well. Since those are usually FOREIGN KEYS, they are usually indexed anyway; but it bears mentioning here.
-
What did you change? Show your revised code and tell us what it is doing? Try echo (or var_dump()) on $anexo to see if it is what you expect.
-
You have to join the table twice. Once it is acting as a "Vendor" table and once it is acting as a "Restaurant" table: SELECT products.*, Vendors.name AS Vendor, Restaurants.name as Restaurant FROM products INNER JOIN companies AS Vendors ON products.vendor = Vendors.id JOIN companies AS Restaurants ON products.restaurant = Restaurants.id WHERE vendor='$id' -- AND companies.type='$type' I'm not sure what to do with that last line. I'm guessing it would be something like: AND Vendors.type = 'Vendor' -- or whatever code you use for vendor records AND Restaurants.type = 'Restaurant' -- or whatever code you use for restaurant records but, if the id in companies is unique, I don't think you need to check the type at all
-
My Internet connection seems to be problematic right now. So, I decided to write a script to run continuously and periodically check to see if the Internet is available. The script checks every five minutes (which is configurable, and I might want to change to 1 minute or 15 minutes). So, my question is, what is a good way to check to see if the Internet is available. My first thought was to do a DNS lookup. So I tried using dig +short domainname which works, and responds pretty quickly. I'm just concerned that a DNS lookup every minute is going to get me "on the radar", so to speak. It does not seem excessive from a network standpoint, but I have no real experience on that side and don't know what Network Admins might be watching for; or if DNS results might be cached in the router or modem (it does not appear to be, but I am guessing). I am also wondering if there is a different command I can (or should) use. The configuration is: Debian Linux <----CAT5----> NetGear (Wireless) Router <----CAT5----> Cisco Cable Modem <----Cable----> Internet So checking to see if the network is up will always return success (unless the router is down). But I want to know if The Internet is available. As long a I am doing this, I wouldn't mind adding a check on the router as well, so if there is a good way to do that, I would be interested to know. For now, the script is written in PHP; I can switch to bash or even a C program if I need to (although, I would like to keep it as a script).
-
Actually, data from a database or a file is affected by magic_quotes_runtime. While magic_quotes_gpc affects GET, POST, COOKIES, and FILES
-
Also, if (false) will always be false. It looks like the validate function should be returning TRUE or FALSE. Then you capture the return value and process accordingly: $rtc = validate($email, 1); if (! $rtc) { setcookie('error', 'Invalid email or password', 0); echo $_COOKIE['error']; } Jessica is correct in saying that the parameter should make sense. But I would use defined constants for it: define('C_VALIDATE_EMAIL', 1); define('C_VALIDATE_PASSWORD', 2); $rtc = validate($email, C_VALIDATE_EMAIL); ## And then in the function switch($type_of_validation){ case C_VALIDATE_EMAIL: ## ... It's a matter of personal preference, but it prevents the problem of mistyping the string (spelling or case) in some place and then wondering why it does not work. If you spell the constant wrong, PHP will issue a warning. You are developing with error reporting on, right?
-
If you did not even get the WARNINGs I put in that last code, my guess is that they have a custom error handler which is trapping and hopefully logging the errors somewhere. You might try to find a log file from the app and see if it contains any recent messages. The code you just posted is checking for the "no write" result, but not for the "no lock" result. So, it would appear that the flock call is failing. However, the file_put_contents() version should not have been affected by that. Of course, we are assuming that you were correct when you stated that this function is the one causing the problems. I can't tell you how many times I've narrowed the problem down to a particular function, only to discover later that I was wrong about where the problem was. Try this version of the function. I changed the trigger_error() calls to print() and added a print of the filesize on entry and exit. See what we can see. // Lock a file, and write if lock was successful function lock_and_write($file, $body, $append = 0) { error_reporting(E_ALL);## ini_set('display_errors', 1);## print('Enter lock_and_write. Filesize = ' . filesize($file) . '<BR>');## ignore_user_abort(1); $mode = ($append == 1) ? "a" : "w"; if ($fp = fopen($file, $mode)) { if (flock($fp, LOCK_EX)) { ##fwrite($fp, $body); if (! fwrite($fp, $body)) print('fwrite failed!<BR>');## ##flock($fp, LOCK_UN); if (! flock($fp, LOCK_UN)) print('flock failed (unlock)!<BR>');## fclose($fp); ignore_user_abort(0); print('Exit lock_and_write (OK). Filesize = ' . filesize($file) . '<BR>');## return ""; } else { print('flock failed (lock)!<BR>');## fclose($fp); ignore_user_abort(0); print('Exit lock_and_write (no lock). Filesize = ' . filesize($file) . '<BR>');## return "no_lock"; } } else { print('fopen failed!<BR>');## ignore_user_abort(0); print('Exit lock_and_write (no write). Filesize = ' . filesize($file) . '<BR>');## return "no_write"; } }
-
Look at your original code: function email($msg) { extract($msg); $this->load->library('email'); $this->email->from($to); $this->email->to($from); $this->email->subject($sub); $this->email->message($msg); $this->email->send(); if ( ! $this->email->send()) { echo $this->email->print_debugger(); } } Six months or a year from now, when you come back to make some change to this function, it will not be immediately clear where $to, $from, and $sub come from. And, in fact, $msg is not what it looks like at first. Coming in to the function $msg is an array, but it gets overwritten by the "'msg'" element of that array when you extract() it. My first response to your original question was that $sub was not defined and did not have a value. This was because I did not see the extract() and did not see where the variables were coming from. Also, consider what happens if you decide to use this function in another area of your application. But in that area, you have loaded the $msg array with a whole lot more data, maybe even including an element called "'this'". What happens when you extract that? I really don't know if it will damage the object's reference to itself, or if PHP protects against it. But it would be a difficult bug to track down if it did. I agree that the extract() function seems to be a handy function, and I used to use it when I first started writing PHP. But, as I said, coming back to the code later left me confused. If you are going to use extract, have a look at the third parameter, and make it a point to use it. This puts a prefix on the variable names so you 1) don't overwrite existing variables, and 2) have an indicator of the source of the variable's definition: extract($msg, EXTR_PREFIX_ALL, 'EX_'); echo "To: $EX_to";
-
When you get a blank white page, use the View -> Source feature of the browser to see if there is any "hidden" content. A malformed HTML tag could cause a problem. If there is anything there (in the view->source) show it to us. The function is returning a string indicating success or failure, I wonder what is happening to that string, more specifically, I wonder what string it is returning. Can you show us the code that calls that function in the script you are having trouble with? fopen with the "w" flag will truncate the file, so the indication is that one of the following functions is failing (flock or fwrite). There are no tests to see if the write or subsequent unlock is failing. Just for debugging purposes, try adding some error triggers in the code. See the lines below that are marked with "##": // Lock a file, and write if lock was successful function lock_and_write($file, $body, $append = 0) { error_reporting(E_ALL);## ini_set('display_errors', 1);## ignore_user_abort(1); $mode = ($append == 1) ? "a" : "w"; if ($fp = fopen($file, $mode)) { if (flock($fp, LOCK_EX)) { ##fwrite($fp, $body); if (! fwrite($fp, $body)) trigger_error('fwrite failed', E_USER_WARNING);## ##flock($fp, LOCK_UN); if (! flock($fp, LOCK_UN)) trigger_error('flock failed (unlock)', E_USER_WARNING);## fclose($fp); ignore_user_abort(0); return ""; } else { trigger_error('flock failed (lock)', E_USER_WARNING);## fclose($fp); ignore_user_abort(0); return "no_lock"; } } else { trigger_error('fopen failed', E_USER_WARNING);## ignore_user_abort(0); return "no_write"; } } This should print one or more error (warning) messages.
-
$this->php_self might be unsafe, depending on what that class is doing with it. There are known exploits with using $_SERVER['PHP_SELF'] directly, but if the class is handing that, then it might be safe. Your script needs to pick up the page value that is requested using $_GET['page'] and use that to select the data for the page. Without seeing some code, we can't really tell where you are going wrong.
-
As a side note, once the permission issue (or whatever, is resolved); I would consider rewriting that to use file_get_contents and file_put_contents. Which, unless I am mistaken, are atomic and would not require the LOCKing strategy that is being used. Something like: if ($append == 1) { $body = file_get_contents($file) . $body); } file_put_contents($file, $body); Hmmm, well, file_put_contents() has a flag for APPEND and a flag for LOCK_EX. So, depending on the PHP version you are using, it could possibly be done without the file_get_contents() call. Having said all that; keep in mind, once you start making changes to third party software, upgrading to a future release can become problematic.
-
That is exactly what you told it to do if $anexo is not equal to "sim". My guess is that that variable is not getting set to what you think it should be. Also, see my comment in the first line below --PHP-mixed-<?php ## NOTE: This should be INSIDE the IF below if ($anexo=="sim") { echo $random_hash; ?> Content-Type: application/zip; name="<?php echo $file_name; ?>" Content-Transfer-Encoding: base64 Content-Disposition: attachment <?php echo $attachment; } ?> --PHP-mixed-<?php echo $random_hash; ?>--
-
It is possible that the software is changing the setting in one of the configuration scripts. Try adding the following, as the first lines inside of that function, just long enough to test. error_reporting(E_ALL); ini_set('display_errors', 1);
-
I would avoid the use of extract(), it makes your code much more difficult to understand. ## Your code, with MY comments and SOME changes function email($msg) { ## extract($msg); ## Avoid the use of extract() $this->load->library('email'); /* ## change the following $this->email->from($to); $this->email->to($from); $this->email->subject($sub); $this->email->message($msg); ## the extract overwrote your original $msg, I think? */ ## to the following $this->email->from($msg['to']); $this->email->to($msg['from']); $this->email->subject($msg['sub']); $this->email->message($msg['msg']); $this->email->send(); ## This line is sending the email (probably) if ( ! $this->email->send()) ## This line is sending the email (probably) AGAIN!! { echo $this->email->print_debugger(); } } You are sending the email twice. I would remove the first call, since there is no error checking on it.
-
Reading Unix Timestamp Into A Regular Date Expression
DavidAM replied to svgmx5's topic in Other RDBMS and SQL dialects
There is a mySql function (UNIX_TIMESTAMP) that will convert a (mySql formatted) date into a unix timestamp. SELECT * FROM myTable WHERE CreateDate > UNIX_TIMESTAMP('2012-10-12') PHP can convert (strtotime) most formatted dates into a unix timestamp to be used in the query. $minTime = strtotime('10/12/2012'); $sql = 'SELECT * FROM myTable WHERE CreateDate > ' . $minTime; I have an internal site that stores unix timestamps, and I really, really wish I had not done that. Ad-hoc queries against the database are a pain, because you can't see the dates/times, unless you add the functions to do the conversion. If I was starting that project today, I would want to use DATETIME or TIMESTAMP. -
Converting And Saving Multiple Html Files To Databasr
DavidAM replied to Tufanayd's topic in PHP Coding Help
Yes, (although I don't see a need for JQuery). -
1) Instantiate an object of the class KeywordsGenerator (it is not a function. 2) Call a method of that class (looks like it should be generateKeywords()) 3) Capture (or echo) the return value from that method call.
-
If the executable name or any parameters that will be passed are supplied by the user, then you have potential security issues. You must validate all user supplied data to make sure it is expected and acceptable. Keep in mind that your hosting provider may put restrictions on you that either prevent running executables, or require them to be in a "safe directory" or limit the CPU or memory resource usage.
-
You don't move_uploaded_file to your website URL; you move it to a filesytem directory. In this case, you might want to use $_SERVER['DOCUMENT_ROOT'] as the path. By the way, why do you have the same code repeated over and over?
-
Exactly! Session variables are created and stored server-side. The client has no access to them. The server sends a token (usually a cookie) that contains the session id. The client sends this back with each request. So, when the login is successful, you store the user's ID in the session. Then when a request is made, you can check the client-provided user id with the session-stored user id. Actually, there is little reason to have the client provide their own ID. Since it is stored in the session, the server already knows it. A Session ID is usually a hash or some other "random" string. So, a malicious user cannot just subtract 1 from the session id and get another valid session id.
-
Hashes are not guaranteed to be unique, so there is still the potential for a name collision. And since there may be collisions with different data, you cannot be sure that a duplicate hash means you have received a duplicate image. All in all, hashing the image data and using it as the filename is not such a good idea. Also, consider that a user may add (or remove) tag data to (or from) an image, and it would produce a different hash even though the visual image is the same. Hashes are designed to "prove" that the data has been changed. You could store the hash of a (image) file in the database; then, before displaying the image, run a hash on the file and compare the two hashes. If they are different, then someone changed the file; if they are the same, then the file has probably not been changed. Since hashes are not guaranteed to be unique, it is conceivable that the file could be changed in such a way as to cause the hash to be the same. Using a salt helps prevent someone from purposefully making such a change; since without the salt they don't know what hash value they are working toward. And the odds of it happening accidentally are very slim.
-
I don't see any HTML. I'm guessing it is in the description in the database. Have a look at the manual entry for strip_tags
-
Is Margaret really going to sit there for 10 DAYS (240 hours) waiting for the balloon to drop? At 0% humidity, it will never leave the ground. At 100% humidity, it comes back in 17 hours. So Margaret is not going to starve to death after all. 20 lines of code, without comments or range checks (I was bored --- and really worried about Margaret)
-
See, to me that is not "it didn't work". That is "the inline styling isn't working". Keep in mind, if you are reading the emails with a browser, the HTML in your message is being placed inside of the HTML for the mail page. So the BODY tag you supplied is inside another BODY tag. That is invalid HTML and may cause rendering problems. However, you can't really leave it out, can you? I mean, if the user uses a mail client instead of a browser, I'm guessing that stuff needs to be there. I have not experimented with it a lot, so I don't know the "correct" way to handle this. I typically include the full HTML, HEAD, BODY, etc. Mostly because I include the TITLE (in the HEAD) for the Subject when displayed in a mail client. That being said, your BODY tag is probably being ignored, which means that background-color is being ignored. I would move the background-color down to the TABLE tag --- or wrap everything inside your BODY in a DIV and put the background on the DIV. The last two links don't have an address, it is just "#"; so they won't go anywhere. You might try putting the CSS in a STYLE sheet in the HEAD or the BODY, instead of inline. I think it looks cleaner, but I don't know how widely supported it is. It seems most people recommend inline styling for HTML emails.
- 3 replies
-
- html email
-
(and 1 more)
Tagged with: