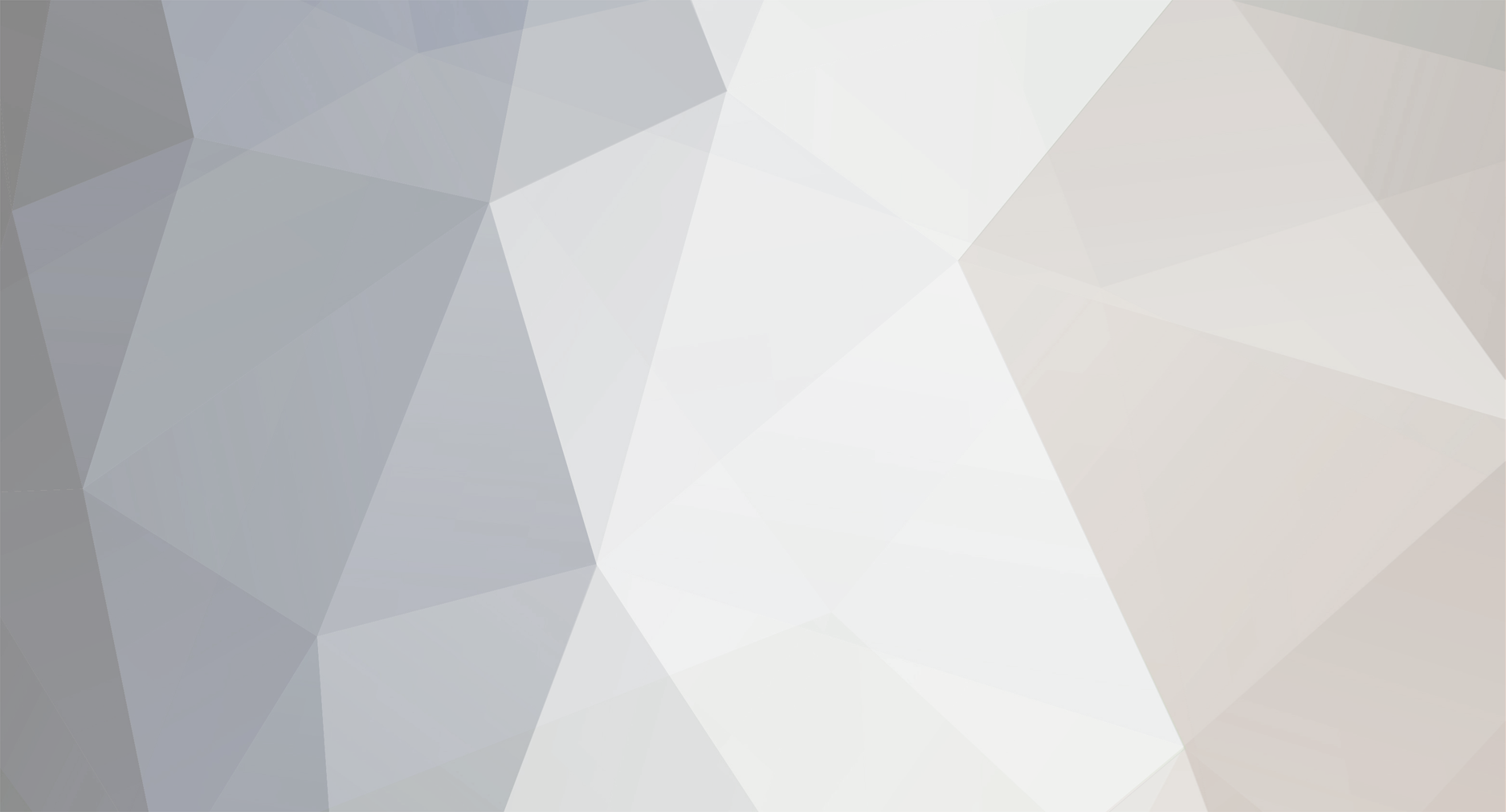
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
As PFMaBiSmAd said in Reply #6, that hash from the database is TOO short. An MD5 hash is 32 characters and that DB hash is only 20. So you are never going to match it. Second, there is a lot of stuff going on in that Registration Processing script that is screwing with the password. 1) Around line 27: $arVals[$key] = (get_magic_quotes_gpc()) ? $val : addslashes($val); This could modify the Password value. Since it will add slashes to "escape" certain characters. If you insist on keeping this code here, you need to use the exact same code in your login so that the password is modified in the same way. 2) Around line 35: if ($key != "access_period" && $key != "passwd") $arVals[$key] = "'".$arVals[$key]."'"; Since the password key is spelled incorrectly in the IF statement, this line is definitely changing the password value entered by the user before you run md5 on it (around line 100). As a side note, not directly related to this problem. The manner in which you are using get_magic_quotes and addslashes "hinky" to me. Since you are using mysql, you should really be using mysql_real_escape_string instead of addslashes, anyway.
-
Looking at that code, I have no idea where $user_id and $password are coming from. So it is difficult to determine what it should be doing. However, let's take this approach: In your login processing page, add these lines just before testing the password if ($row = mysql_fetch_assoc($result)) { // LET'S SEE WHAT WE HAVE printf("dbHash: %s<BR>\n", $row['password']); printf("enrtyHash: %s<BR>\n", md5($password)); printf("should be: %s<BR>\n", md5('HARD CODE THE PASSWORD YOU ARE TESTING WITH HERE')); if (strcmp($row['password'], md5($password)) != 0) { That should give you three hashes. The last two should be exactly the same if $password is coming from the form. If they are and the database one is different, then we need to see the code that puts the entry in the database. I notice in your first post, you were inserting from $arVals[] but there is no indication of how the values got into that array and what might have been done to the password before it was hashed. Typically, a salt is used when hashing, and if one is used before the INSERT, we have to use the same salt in the login page. By the way, turning off register_globals is not just for security. Your development platform should match your intended production platform as closely as possible. Especially in this respect. If you develop with register_globals ON you could end up writing code that "works perfectly" on your development platform, but fails miserably on your production platform. -- Search this forum for "works perfectly" and you will find many, many posts that have that problem.
-
And they let you inadvertently set variables to something you don't want them set to. $_SESSION['user_id'] = $user_id; $_SESSION['password'] = $password; With register_globals ON, the first time you executed this statement, the password from the POST data was placed in the session. Every time after that, register_globals put the SESSION password value into $password (overwriting the POST value). So, regardless of what you type in the form, you are testing the same password value over and over (from the SESSION data). 1) Clear out your session -- delete the cookie and if you have access, delete the server file 2) Do NOT store the user's password in the session. Session files, especially on shared hosts, are typically stored in /tmp which is world readable. Note: You may have had the same problem on the page that registered the user. So, the value that was hashed and stored as the user's password may not have been what you think you set.
-
Your function definition is inside an ELSE clause. PHP will NOT define that function until that section of code executes (since the function definition is conditional). Move the function definition outside of your IF clause (recommended), or move the call to after the definition. Also, indent your code, so you can easily see things like that.
-
You can make the entire decimal part optional $regExp = '~^[0-9]{1,10}(\.[0-9]{0,2})?$~'; $values = array('123.45', '123', '123.', '123.5', 'abc', '123a21'); foreach ($values as $ind => $value) { $match = preg_match($regExp, $value); printf("%d: %s -- %d\n", $ind, $value, $match); } output 0: 123.45 -- 1 1: 123 -- 1 2: 123. -- 1 3: 123.5 -- 1 4: abc -- 0 5: 123a21 -- 0
-
I don't think you can do it in a single query with any kind of JOIN. But you can do it with a UNION using two LEFT JOINs -- All Rows in Table 1 -- SELECT TABLE1.x, TABLE1.y, IFNULL(TABLE2.z, 0) AS z FROM TABLE1 LEFT JOIN TABLE2 USING TABLE1.x = TABLE2.x UNION -- All Rows in Table 2 with no matching X in Table 1 SELECT TABLE2.x, 0, TABLE2.z FROM TABLE2 LEFT JOIN TABLE1 ON TABLE2.x = TABLE1.x AND TABLE1.y IS NULL ORDER BY 1
-
A proper query only has the WHERE keyword once. DELETE FROM temp_users WHERE condition_1 AND condition_2 AND condition_3 The book_stage condition looks OK (although if it is an integer field, I would not use quotes around it). For the time condition, you need to use mySql functions, or do the calculation in PHP and insert the value. Using the mySql functions would be the preferred method. CURRENT_TIME > DATE_ADD(creation, INTERVAL 60 MINUTE)
-
The mysql_query function returns a resource when a SELECT statement succeeds. When an action query (INSERT, UPDATE, DELETE) succeeds, it returns true (a boolean), when any query fails, it returns false (a boolean). When a mysql_whatever() function issues that message, it generally means the query failed. To figure out why a query failed, you can do this: mysql_select_db("product", $con); $sql = "SELECT * FROM 'tblt' WHERE 'UID' =('$ids')"; $sqlout = mysql_query($sql); if ($sqlout === false) { print('Query: ' . $sql . ' failed with error: ' . mysql_error()); exit; } while($sqlres = mysql_fetch_array($sqlout)) Of course, in a production environment, you would do something more user-friendly than just exiting -- and you really don't want to display that kind of information to potential hackers. The problems I see with that query are: 1) You have the table name in quotes -- that is not valid 2) You have parenthesis aroud the $ids value -- I really don't know if that hurts or not, but I don't see any need for it.
-
I'm sorry. Is there some reason you suggest running the same query twice instead of using SQL_CALC_FOUND_ROWS and SELECT FOUND_ROWS()? I mean, returning all those rows, twice, is very inefficient. At least, just use a SELECT COUNT() instead of selecting all the data. Did I miss something there?
-
In the provided code, the WHILE loop contains a single statement. It is an IF statement. Since it is a single statement, the curly-braces are not technically required. Just like an IF statement without braces will execute a single statement, so will a WHILE. The reason that the ELSE part is never getting executed is that the statements inside the example loop never execute when mpid != $mpid. Since the SELECT statement will not return any rows, the condition on the WHILE loop is false, and the IF is not executed. To get the desired results, try something like this: $resultc = mysql_query("SELECT * FROM table where mpid=$mpid"); if (mysql_num_rows($resultc)) { while($rowc = mysql_fetch_array($resultc)) echo '<p>' . $rowc['vpcomm'] . '</p>'; } else { echo "No Comments Available"; } again, without the curly-braces, it is a bit confusing, so: $resultc = mysql_query("SELECT * FROM table where mpid=$mpid"); if (mysql_num_rows($resultc)) { while($rowc = mysql_fetch_array($resultc)) { echo '<p>' . $rowc['vpcomm'] . '</p>'; } } else { echo "No Comments Available"; }
-
Most likely, that is the problem $year = 2012; $sql = "select year, force, homicide from crime where year = $year "; echo $sql; $sql = 'select year, force, homicide from crime where year = $year '; echo $sql output would be select year, force, homicide from crime where year = 2012 select year, force, homicide from crime where year = $year The second query is invalid because "$year" is not a valid number to compare against the year column; and, since it is a string, the server will either look for a column named "$year" or complain about an illegal character (or something).
-
1) Test to see if the value is an array and don't process it foreach ($_POST as $key => $value) { if (is_array($value)) continue; // ... } Of course, then strtotime will choke on the submit button. I would recommend changing the field names in your HTML: <INPUT name="dates[$ID]" ...> # Where $ID is whatever ID or Key you are using now # or, if that's not an ID and just a sequential array index <INPUT name="dates[]" ...> # use empty brackets Then process only the dates foreach($_POST['dates'] as $id => $value) { // Here we get only the dates } [edit] Guess I type too slow [/edit]
-
I'm not familiar with using SQL Server with PHP, but that error usually indicates that the query failed: $describeQuery='select year, force, homicide from crime where year = $desiredyear '; 1) PHP will not interpret variables inside a string that is delimited with single-quotes. This is likely the problem. Use double-quotes around the string or use concatenation. 2) Is "year" a reserved word in SqlServer? (It is not in mySql.) 3) Is the YEAR column an integer (it should be). If it is not, you are going to need quotes around the value.
-
500 server error after specific number of cURL executions
DavidAM replied to Kurrel's topic in PHP Coding Help
For development, I usually use error_reporting(-1); (or E_ALL) so I see ALL of the messages. On the memory issue, you can use memory_get_usage, which "Returns the amount of memory, in bytes, that's currently being allocated to your PHP script. " Now that I think about it, though, there is a specific error that is printed when PHP exceeds the memory limit. But you could check the value before and/or after each loop cycle to see how much it is jumping. I would think that a 500 error would put something in the Apache error log. This may be an issue with PHP/Apache not releasing the socket right away when the curl session is closed. This might be causing you to exceed the allowed number of open file descriptors (which is an O/S level setting). See if there is a "Keep Alive" setting (or something) in the curl options and whether you can reduce the time or turn it off. Have you considered/tried moving the curl_init() and curl_close() functions outside the loop? I don't know how much rewrite this would require (or if it would even work), but I wonder if using the same curl handle would help. -
creating tables with columns and values from values in other tables
DavidAM replied to orise's topic in MySQL Help
INSERT INTO destinationTableName (columnName1, columnName2, ...) SELECT dataForCol1, dataForCol2, ... FROM sourceTableName WHERE conditions If you can build the SELECT statement to return the columns and rows you want to INSERT into the other table, it is pretty much just a matter of combining the two statements. I'm sure there are some limitations (see the manual) -
The isset call returns true if the variable is defined (has been "set" to a value), and returns false if it has not. The following would accomplish about the same thing, but it would throw an error (actually, a NOTICE) about referencing an undefined variable (or, in this case, an undefined index in the array): $apples = $_POST['apples']; The ternary operator condition ? trueExpression : falseExpression; is a shortcut for an if ... else ... if (isset($_POST['apples'])) { $apples = $_POST['apples']; } else { $apples = ''; } // is exactly the same as $apples = (isset($_POST['apples']) ? $_POST['apples'] : ''); Don't start thinking that your dropdown menu values (<SELECT> fields) are safe. They can be very easily hacked to send you a value you are not expecting. If you use the value directly from the POST array, you must validate and/or sanitize. I don't sanitize my variables until I put them in an SQL statement. Consider: // Values from the form $_POST['apples'] = "I'm here"; $_POST['donuts'] = "What's a \ for?"; // Quick and dirty sanitize $fromUser = array_map('mysql_real_escape_string', $_POST); // Now I want to display what the user typed print "Apples: " . $fromUser['apples'] . "<BR>"; print "Donuts: " . $fromUser['donuts'] . "<BR>"; The output displayed by those statements will be: Apples: I\'m here Donuts: What\'s a \\ for? mysql_real_escape_string() puts backslashes in to protect the database. The database knows to treat these backslashes in a special way. But to the browser, they are just characters to be displayed. This is why I don't "escape_string" until they go into an SQL statement.
-
500 server error after specific number of cURL executions
DavidAM replied to Kurrel's topic in PHP Coding Help
While you're waiting for a curl expert ... check the basics: Did you check the Apache error log? Could there be a memory leak in curl causing the php memory use to continuously increase? Do you have error_reporting on so you can see any PHP warnings, notices, or errors? -
Is the column for the current academic year actually called "election_id" or do you have the wrong column name in that INSERT statement?
-
$_POST (there is an underscore in there) is where PHP holds the values POSTed from a form, so that your script can get to them. If you are running an old version of PHP and/or you have register_globals turned on, then the first two statements are redundant. However, having register_globals on is a major security risk! The "best practice" is to run with register_globals off and do something like this: $apples = (isset($_POST['apples']) ? $_POST['apples'] : ''); $donuts = (isset($_POST['donuts']) ? $_POST['donuts'] : ''); /* Verify that the values for "apples" and "donuts" are valid (whatever "valid" means to your application) */ // Prepare for SQL (assuming mysql here) $apples = mysql_real_escape_string($apples); $donuts = mysql_real_escape_string($donuts); $sql = "INSERT INTO myTable (appleColumn, donutColumn) VALUES ('$apples', '$donuts')"; I have over-simplified that example. The point is, Test to see if the values were provided; Validate the values to be sure the are acceptable; Sanitize the data to prevent SQL injection attacks; Build the SQL statement separately (to make debugging simpler); Send it to the database (not shown in the example).
-
thumbnail script stopped working after upgrade to PHP version 5
DavidAM replied to daveyp2004's topic in PHP Coding Help
Glad to help. Make sure you turn off error reporting on your production server. Mark the topic Solved Have fun! -
thumbnail script stopped working after upgrade to PHP version 5
DavidAM replied to daveyp2004's topic in PHP Coding Help
Interesting. If I request the script without the im parameter -- http://www.jetski.ukwebad.com/uktn.php -- I get the following However, if I add the im parameter -- http://www.jetski.ukwebad.com/uktn.php?im=forms/user_uploads/68f00fc57c0fc71030fb53cd44f67d28.jpg -- I get an image which is a line of text that says: It is actually an image of the text and not an html document. That is strange. I've never seen it before. On to the problem. The only thing I can see is the call to imagepng. The third parameter is called "quality", but seems to actually be compression level. The valid range is 0 - 9. Try changing that line to: imagepng($img, null, 9); Note that the higher compression levels take longer to compress but produce smaller files for download. -
VALIDATE E-MAIL IN EXISTING PHP SCRIPT - 03.19.12
DavidAM replied to mrjap1's topic in PHP Coding Help
It looks like you got it. If you put those two IF statements in place of the one in your first post, it should work fine. As long as you keep in mind that this does not mean that the email address is "valid" (whatever that means). Even if you write THE perfect test script to check the email address (the one I currently use is probably around 100 lines of code) you can't tell if the email address is a working email address without contacting the SMTP server (for that address) and asking if it can deliver to it. This is one of the reasons that most sites require you to respond to an email in order to complete the registration. Your response to the email is the closest proof they can get that the address is valid and it belongs to the person submitting the form. -
And you shouldn't. At the very least export these rows to an archive table. If the application calls for a DELETE, then you delete. You just need to design your application properly so you don't delete valuable data. From the standpoint of table fragmentation, an UPDATE is just as bad as a DELETE. Unless ALL of your columns are fixed length, and NONE of your columns allow NULL; then the row length varies, and it is not possible to do an update-in-place. The row is typically "moved" to another location in the "table space" and the table fragments. Also, if any of your indexed columns are being updated, the indexes are (likely) getting fragmented as well. A database, like a car, requires periodic maintenance.
-
VALIDATE E-MAIL IN EXISTING PHP SCRIPT - 03.19.12
DavidAM replied to mrjap1's topic in PHP Coding Help
Verifying that a string is a valid email address is not a simple matter. Email addresses can be very convoluted. If you search for it in Yahoo, Google, or any other search engine you will find many, many entries. If you just want to check for the "@" and the "." you can use strpos and strrpos. $posAt = strpos($email, "@"); $posDot = strrpos($email, "."); if ( ($posAt === false) or ($posDot === false) or ($posDot < $posAt) ) { // Bad Address I used strrpos() because there can be full-stops before the "@" as well as after. You may want to improve these checks since there must be at least one character before the "@" and at least one between the "@" and the "." and the "." cannot be at the beginning or the end. ... as I said, this testing gets very complicated. Note: My business email address is 26 characters. So I guess you don't want me at your website. The maximum length of an email address is not clearly defined, although there are limits on the various components of the address. I usually set my database field to VARCHAR(255), which is still less than the "legal" limit. Note: The full-stop (".") is not required. There are valid email addresses at the TLD's (i.e. someone@com); although you are not likely to see many of these at your site. -
move_uploaded_file($_FILES['usr']['tmp_name'],"/learning/".$_FILES['usr']['name']); The leading slash on your destination "/learning/" indicates the root of the file system. This would be equivalent to specifying "C:\learning\...". This is most likely NOT where you are trying to put the file. The error message is indicating that that directory does not exist. You need to either take off the leading slash --- which will put it in the "current" directory, wherever that is --- OR (the preferred approach) define a variable that holds the destination directory name: Using parts from your original post: $dir = "C:\\xampp\\htdocs\\learning\\"; // ... checking and processing code move_uploaded_file($_FILES['usr']['tmp_name'], $dir . $_FILES['usr']['name']);