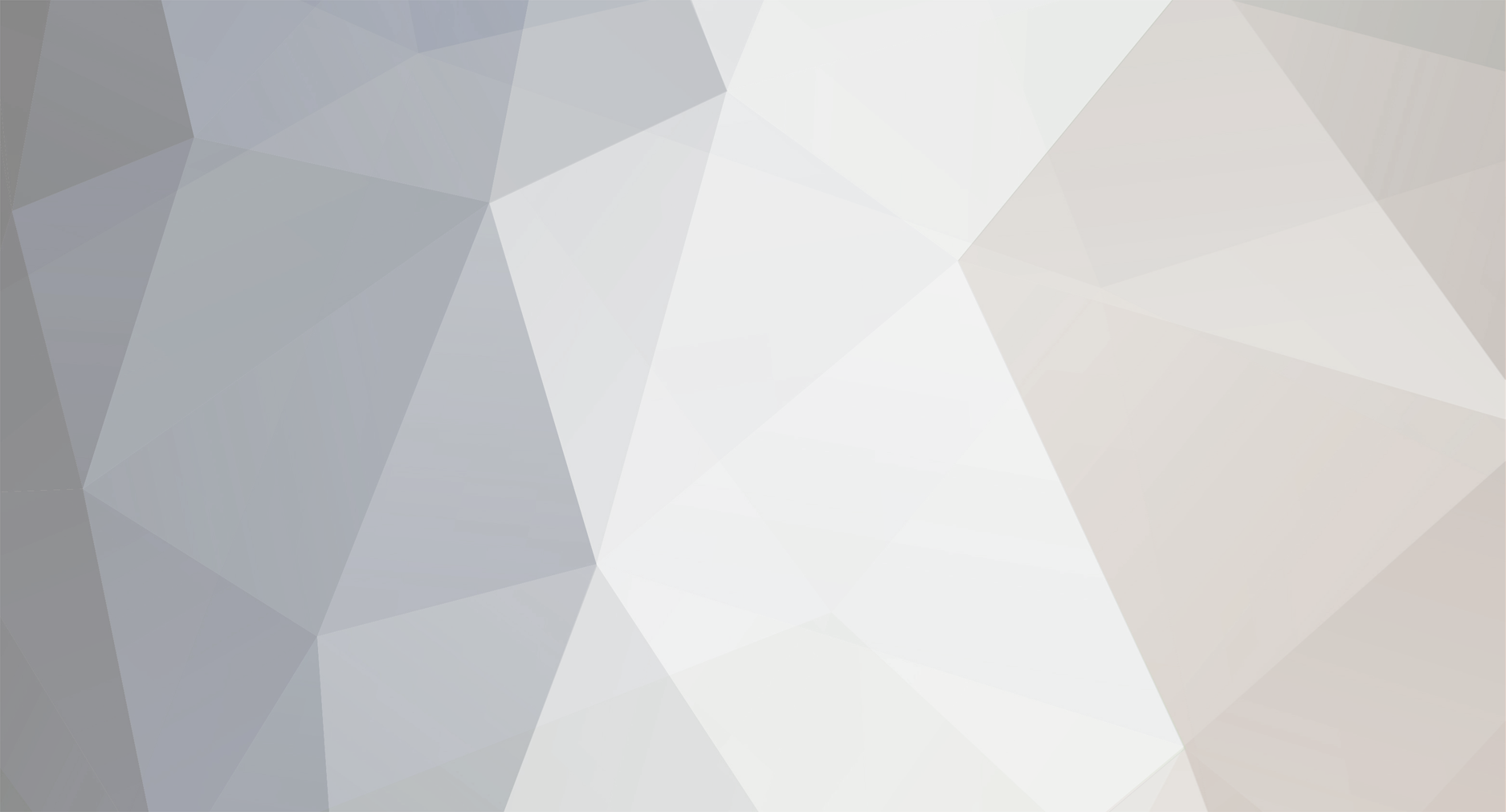
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
Damn Timezones! We have to treat them all as UTC times to get this to work correctly. You might want to (eventually) look at the PHP DateTime class. // Assuming we have a timezone set ... date_default_timezone_set('America/Chicago'); $var1 = '2012-02-24 06:38:22'; $var2 = '02:00:00'; $t1 = strtotime($var1 . ' UTC'); // CHANGED THIS $t2 = strtotime('1970-01-01 ' . $var2 . ' UTC'); // AND CHANGED THIS (don't know why zero wouldn't work here) $t3 = $t1 + $t2; $var3 = gmdate('Y-m-d H:i:s', $t3); // AND CHANGED THIS print($var3 . PHP_EOL); // Output: 2012-02-24 08:38:22
-
If you are pulling these from a mySql database, you can add them in the SELECT query using the ADDTIME() function SELECT StartTime, Duration, ADDTIME(StartTime, Duration) AS EndTime ... If you really need to add them in PHP, you can use the strtotime function on each of them, add the results together, then use the date function to reformat them. $var1 = '2012-02-24 06:38:22'; $var2 = '02:00:00'; $t1 = strtotime($var1); $t2 = strtotime($var2, 0); // NOTE the 0 (zero) so we don't get the current date $t3 = $t1 + $t2; $var3 = date('Y-m-d H:i:s', $t3); print($var3 . PHP_EOL); // Output: 2012-02-24 08:38:22
-
Your query is grouping on referrer rather than the date, so I think you are getting the number of times for each unique referrer and NOT the number of different referrer's. If your date column is a DATE or DATETIME column I would think the query you want is: SELECT MONTH(date), COUNT(DISTINCT referrer) 'referrer' FROM app_analytics WHERE appid = $userid AND date BETWEEN $y_month AND $t_month GROUP BY MONTH(date)
-
Yeah, that's about how it is done. I use a function to handle this for me: function quoteStringNull($value, $delim = "'") { if (empty($value)) return 'NULL'; else return $delim . mysql_real_escape_string($value) . $delim; } $sql = 'UPDATE aTable SET aColumn = ' . quoteStringNull($newValue) . ' WHERE ID = ' . $theID; The function actually does a little more than that, but that is the idea. I have a second function called quoteString(), which returns an empty string (instead of NULL) when the value is empty. I call the appropriate function depending on whether the column allows NULL or not. The key is, the value returned by these functions has the delimiters I need for the SQL statement inside the returned value, so I don't have to put them in the SQL string. That way, the function will return the string NULL, without the delimiters around it. Obviously, the quoteStringNull() is not useful for the WHERE clause, only for the VALUES of an INSERT or UPDATE.
-
You are only providing snippets of code, so it is impossible for us to find the problem. For instance, is there any other database interaction between executing the INSERT and retrieving the ID? If so, that could be causing a problem. mysql_insert_id Also, you have to check every step of the way for any errors. $maincon = mysql_connect('local', "root", "root"); if ($maincon === false) { print "Unable to connect to server. I don't know what to do so ..."; die(); } if (mysql_select_db("root") === false) { print "Unable to select database. So ..."; die(); } $query = "INSERT ..."; $success = mysql_query($query); if ($success === false) { print "The query failed: " . $query . " with error: " . mysql_error(); die("so"); } $ref_id = mysql_insert_id($maincon); Note: The die() function is only suggested here as a debugging tool. The script should do something intelligent when an error condition prevents future action. Calling the die() function will cause the script to exit, leaving your user with a blank white screen. Not very user friendly.
-
$message = wordwrap($message, 900, "\n", true); The "true" parameter forces the break at 900 (in this case). If this parameter is "false" it will find a space to break on. So, it could be breaking in the middle of a tag name or attribute name or value. (whitespace inside a tag is not significant unless it breaks a name or value) The RFC specifies <CRLF> as the line break. Most mail systems probably handle <LF> alone, but there may be some that do not. You might consider wrapping it with "\r\n". When generating HTML for a mail message (or for a web page, for that matter), I always stick in line-feeds at the end of blocks and so forth -- so, after </P>, </TR>, </DIV>, even </TD> if the rows are long), then I wordwrap() to about 70. wordwrap() seems to honor the line-feeds that are already present and just adds new ones (like for long paragraphs). Other Considerations: You really need to take a look at the source of the message -- either write it to a file or CC yourself on the email. Find out what mail client is being used when this happens, is it always the same one or does this happen across multiple clients? Is it possible that a value in a cell actually contains HTML special characters? Be sure to run the values through htmlspecialchars() or htmlentities() to prevent data from scrambling the page.
-
The COUNT function should be part of your SELECT list: SELECT name, AVG(overall) AS overall, COUNT(*) AS counted FROM $tbl_name GROUP BY name ORDER BY AVG(overall) DESC";
-
I think the code where you are parsing the CSV needs to change as well. Try this: while (($row = fgetcsv($handle, 0, ',', '"')) !== FALSE) { foreach($row as $key => $value) { if ($line === 1) { $keys[$key] = $value; } else { $out[$line][$keys[$key]] = $value; // <<< We want the heading as the index } } $line++; } unless I am terribly mistaken, the $key value in the foreach will be just an integer index (i.e. 0, 1, 2, 3 ...). This is (I guess) why the first line is captured in the $keys array. So, you need to use those collected key names in loading the line. That is why you were getting the undefined indexes (I think). So you will need to change back to $values in the code I gave you before: $LOCATION = $values['LOCATION']; $LocationName = $values['Location Name']; $AssetNumber = $values['Asset Number'];
-
<a href=profile.php?ID=>break</a> -- Look Here ---------^ The member's ID did not get output. So apparently $row['ID'] does not exist or does not have a value. Check the column name. The array index (ID) is cAsE SeNsItIvE.
-
View the source of the member list in your browser to see what the links look like. Either the ID is not getting put there, or it is being ignored. Are you sure the column name is ID (uppercase) or is it id (lowercase)? Also, you really should have quotes around the entire href value. echo "<tr><td>" . '<a href="profile.php?ID=' . $row['ID'] . '">' . $row['user_name'] . "</a>" .
-
You can use mysqli_insert_id(), you just have to use it twice. It gets the auto_increment value from the last insert statement on the connection. I'm not as familiar with mysqli so here is an example using mysql. You should be able to make the necessary adjustments. $sql = "INSERT INTO pets(pet_name) VALUES('$pet_name')"; mysql_query($sql); $pet_id = mysql_insert_id(); $sql = "INSERT INTO members(member_name, pet_id) VALUES('$member_name', $pet_id)"; mysql_query($sql); $member_id = mysql_insert_id(); $sql = "UPDATE pets SET member_id = $member_id WHERE pet_id = $pet_id"; mysql_query($sql);
-
Your variable names have spaces in them. -- i.e. {$Location Name} -- This is NOT allowed. Besides, I don't see anywhere that you have defined these variables. Also, your foreach($out as $key => $value) { is not consistent with the way you loaded the data. I'm guessing here, but I think that section of code should be something like this: if (!empty($keys) && !empty($out)) { $conndb = mysql_connect("localhost", "root", ""); mysql_select_db("master", conndb); // foreach($out as $key => $value) { foreach ($out as $lineNo => $values) { $LOCATION = $values['LOCATION']; $LocationName = $values['Location Name']; $AssetNumber = $values['Asset Number']; $sql = "UPDATE `master` SET `LOCATION` = '{$LOCATION}', `Location Name` = '{$LocationName}' WHERE `Asset Number` = {$AssetNumber}"; mysql_query($sql); } mysql_close($conndb); $message = '<span class="green">File has been uploaded successfully</span>'; }
-
This is a BAD idea. This will NOT work. First, if two users insert records at the same time, they will both get the same value for MAX(id). Second, you already have the ID of the record you just inserted from the call to mysqli_insert_id(), so this is not even necessary. This will not work. You just created the record with pet_id = $id, so the value will most likely not be in the table. If it is already there, you have bigger problems. Why don't you just get the current user's account_id and insert it with the original INSERT?
-
Well, I rarely use lambda functions in my code. I just don't personally like the look of it. So I would write the binding as: $(".del a").bind("click",delClick); // Pulled this out of your original BIND function delClick() { var batch = $(this).attr( 'href' ); $("#confirmdel").html('Are you sure you want to delete batch ' + batch + '? <a href="#" id="confirmdelete">Yes</a> <a href="#" id="cancel">Cancel</a>'); $("#confirmdel").show('slow', function(){ $("#confirmdelete").click(function(){ $.post('../scripts/deletebatch.php', {batchid: batch}); $("#confirmdel").hide('slow'); $('#batches').fadeOut('fast'); $('#batches').load('index.php #batches',false,function(){ $('#batches').fadeIn('slow'); }); // Now BIND the rows we just added $(".del a").bind("click",delClick); return false; }); }); return false; } I think the BIND method only binds to existing elements at the time that you call .bind(). However, I seem to remember reading something on the jquery site about the ability to define a bind that would automatically bind to new instances of the selector.
-
Typically, I do this by "forcing" the array index value. The code you have <INPUT TYPE="TEXT" NAME="particpation_points[]" > will create an array: $_POST['particpation_points'][seq#] -- where the number is just an incrementing value from zero. You will have no way to really "know" which array element goes with which "user_id". I would write it something like this: <INPUT TYPE="TEXT" NAME="particpation_points[12]" > Here, the "12" represents the database ID value of the user's record I will update with the value provided. So the code to generate the form would be something like this: // Database query to get the students of the class ... while ($row = mysql_fetch_assoc($resource)) { print('<SELECT NAME="attendance[' . $row['id'] . ']"> <OPTION VALUE=""> </OPTION> <OPTION VALUE="1"> Present </OPTION> <OPTION VALUE="0"> Absent </OPTION> </SELECT> <INPUT TYPE="TEXT" NAME="particpation_points[' . $row['id'] . ']" >'); } print('<INPUT TYPE="SUBMIT" VALUE="Submit" >'); Then to handle the data after posting: foreach ($_POST['attendance'] as $ID => $value) { $sql = "INSERT INTO $class (oasis_id, attendance_date, attendance, particpation_points) values ($ID, '$attendance_date','$value', " . $_POST['participation_points'][$ID] . ")"; // Execute Query } Or something along those lines. Since we "forced" the array index, we only need one loop. You need to sanitize the inputs before shoving them into the query -- This is just a example of the process flow You can, and probably should, build a single insert statement for all of the data, so you can do a single trip to the database.
-
I don't know how much I can help, but I see a couple of things that might create issues: 1) the "id" attribute in HTML is supposed to be unique on the page (well, in the DOM). So, creating multiple rows with the same "id" could lead to issues with jquery. You should probably use a class instead of the id here. 2) You have deleted the DOM element that you bound the click event to. Then you created new DOM elements. You will (most likely, I think) have to BIND the click event to these new DOM elements (after the load). It seems to me that there is a way to do the BIND that will cause jquery to bind the event to ALL present and future elements that satisfy the selector, but again, since you are selecting by ID and the ID is supposed to be unique, jquery may not honor the "future" part or the multiple future elements.
-
p0-mad:~$ php -r "echo number_format(150 + 20.95 + .5, 2);" 171.45 works for me, too. Are you using variables in your code? Maybe they are not what you think they are.
-
Read the error message carefully, and you will find that the variable name is in it ($memberID). Variables are not interpreted inside single-quote marks. Use double-quotes to assign the the "old" name: $new = "memberFiles/$memberID/logo.jpg"; The error message could be a little clearer; since it shows only one of the two parameters, it lead me to believe that you had them backwards. But, I suspect the error indicates that the directory "$memberID" does not exist and therefore you cannot copy a file into it.
-
1) You need to turn on error reporting so you can see the errors that are occurring and fix them. Put this at the beginning of your php script error_reporting(E_ALL); ini_set("display_errors", 1); Do NOT ignore any WARNINGS or NOTICES. They are the result of LOGIC errors and need to be fixed. 2) It looks like you may have incorrectly modified the connection data: // FROM YOUR SCRIPT define("*******", "YOUR HOST"); define("*********", "YOUR USER"); define("******", "YOUR USER PASSWORD"); define("**********", "YOUR DATABASE"); If you have *'d out the connection parameters, you may have done it backwards. Your connection information needs to be the SECOND parameter there NOT the first. // SOMETHING LIKE THIS define("HOST", "????"); // Replace the ??'s with your database server (probably localhost) define("USER", "????"); // Replace the ??'s with your database user login name define("PASSWORD", "????"); // Replace the ??'s with your database user's login password define("DB", "????"); // Replace the ??'s with your database name
-
I, too, recommend sprintf(). However, be advised that the "%10s" will not stop the string at 10 characters. It will pad the string if $digitString is less than 10 characters long; but if it is more than 10 characters long, it will include the entire string. So, it would be safer as: sprintf("%c%10s ", $firstChar, substr($digitString, 0, 10));
-
Your problem is due to "variable scope" in labelprocessor.php. $senderName = $_POST["first"]." ".$_POST["last"]; $senderPhone = $_POST["phone"]; $senderStreet = $_POST["street"]; $senderLine2 = $_POST["line2"]; $senderCity = $_POST["city"]; $senderState = $_POST["state"]; $senderZip = $_POST["zip"]; $senderCountry = "US"; // THEN LATER function addShipper(){ $shipper = array( 'Contact' => array( 'PersonName' => 'Sender Name', //doesn't process at all if at least this one isn't a text value 'PhoneNumber' => $senderPhone), 'Address' => array( 'StreetLines' => array($senderStreet, $senderLine2 ), 'City' => 'Arlington', 'StateOrProvinceCode' => $senderState, 'PostalCode' => $senderZip, 'CountryCode' => 'US') ); return $shipper; } Here you have defined the variables (such as $senderPhone) in the "Global" scope of the script. Then you try to access the variable in the scope of function addShipper. Those variables do not exist inside of the function. You need to pass them in as parameters to the function (or -- not recommended -- refer to them as globals).
-
You can get whatever you assign as the "value" attribute of the OPTION selected. <SELECT name="cmbChoose"> <OPTION>Me</OPTION> <OPTION>Her</OPTION> </SELECT> Using this example, you will get either "Me" or "Her" from $_POST['cmbChoose'] <SELECT name="cmbChoose"> <OPTION value="1">Me</OPTION> <OPTION value="2">Her</OPTION> </SELECT> Using this example, you will get either "1" or "2" from $_POST['cmbChoose']
-
I've never used autoload, but the way I understand it, the code in your first block should fire it: // setup the sessions handler $session = new Session($member); and should autoload the Session class (in class.Session.php) creating an object which is stored in the variable $session (lower case name). You could just as easily call it $abc it should not matter. However, you are referring to it in the second code block as <?=$Session->isLog();?> using an uppercase name ($Session). The error message says which is saying that the variable $Session (uppercase name) does not exist. If you change that to be the lowercase name ($session), you should not get the same error message -- does it then tell you that $session (lowercase name) does not exist? We don't see enough of the code to know if there is a scope issue here. The object you created exists in whatever scope (Global or function or class) that it is instantiated in. So your reference to it must be in the same scope; otherwise, the variable does not exist (in scope). Have you tested to see if the object is, in fact, being instantiated? // setup the sessions handler $session = new Session($member); if (! is_object($session)) trigger_error('Session Object Not Instantiated', E_USER_ERROR); although, I would expect you to get an error at that point if it did not.
-
$session (lowercase name) is not the same as $Session (uppercase name).
-
The GROUP BY phrase is used to limit the results to a single row for each value of GROUP BY field(s). It is intended for use with aggregate functions. For instance: SELECT l.email, COUNT(q.id), MAX(q.value), MIN(q.value) FROM ... GROUP BY l.email would give you the Count, Maximum Value and Minimum Value of all quotes for each lead. The ORDER BY (which appears after the GROUP BY) controls the sequence of the returned rows and is not affected by the GROUP BY at all. In your case, I think you want to remove the GROUP BY, and move the field you have there into the (beginning of) the ORDER BY. SELECT ... FROM leads l ... ORDER BY l.email, qr.id DESC