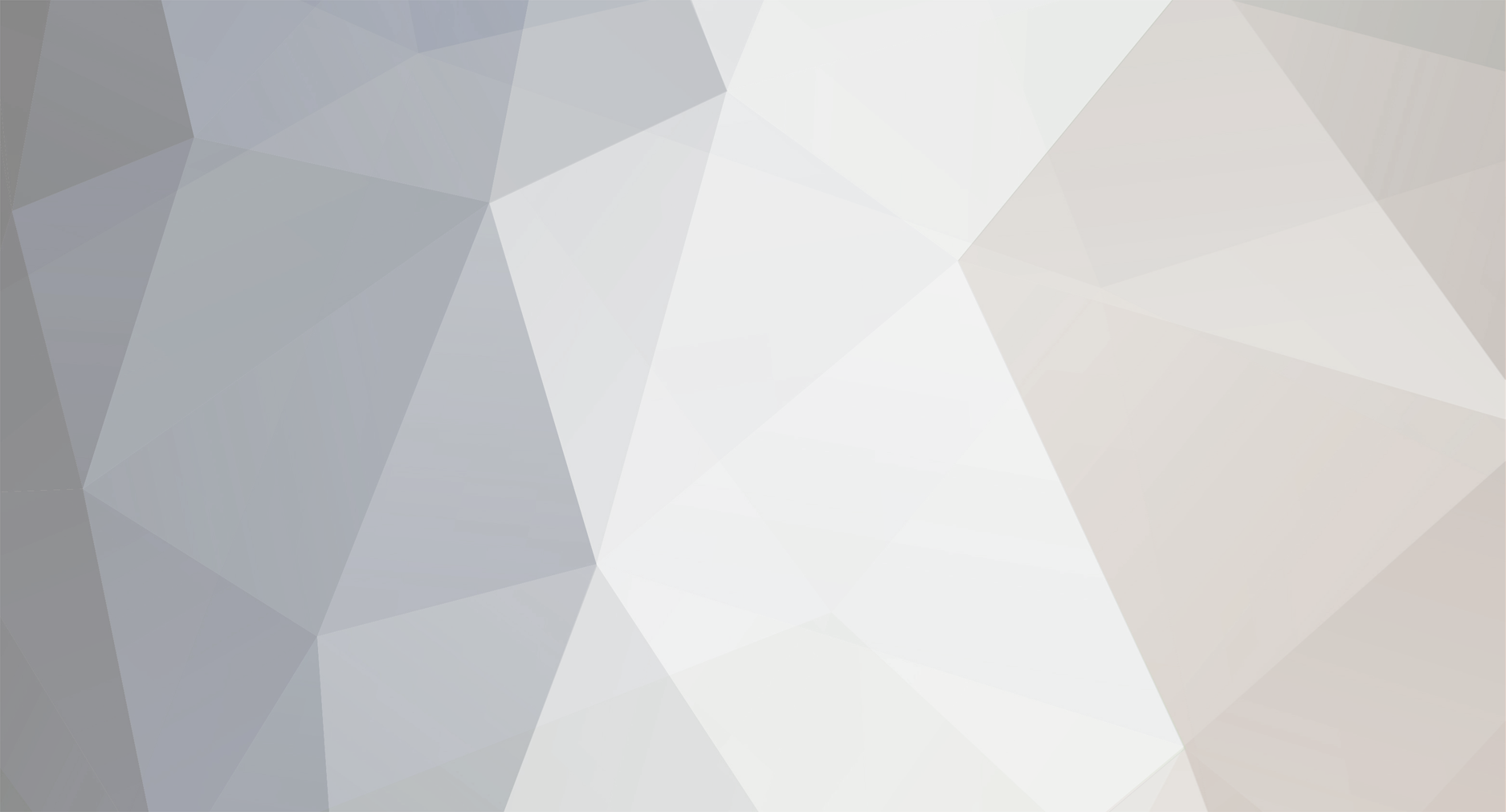
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
mysql_fetch_array returns the next row from your query. Since you are only calling it once, you are only looking at the first row. Since mysql_fetch_array() will return false if no row is returned, you don't need to use a counter for the loop. Consider something like this: <div = "reviews"> <?php $sqlrev = "SELECT * FROM rev WHERE rev_name = '$member' ORDER BY id DESC"; $revresult = mysql_query($sqlrev) or die(mysql_error()); $revrows = mysql_num_rows($revresult); // Don't really need this any more, either # $revname = mysql_fetch_array($revresult); MOVED THIS TO REPLACE THE FOR LOOP ?> User reviews for <? echo $member;?> <br> <br> <table border="0"> <?php // PROCESS EACH ROW RETURNED BY THE QUERY while ($revname = mysql_fetch_array($revresult)) { ?> <tr> <?php echo "Review by: " . $revname['usr_name']; echo "<br>"; echo $revname['text'] ."<br>"; echo "<br>"; ?> </tr> <?php } ?> </table> </div>
-
Display data from database !Confused!HELP Please
DavidAM replied to ckerr27's topic in PHP Coding Help
The only error I see in the code as posted, is a missing semi-colon on the first echo. However, the example display you showed indicates that you used a single-quote to open the first echo, and did not close that string. (by the way, please use [ code] tags or [ php] tags when posting code). The example display looks like your code actually is: echo '<b><u>Event Name:</b></u> $eventname echo "<b><u>Event Date:</b></u> $eventdate<br>"; } ?>' Note that PHP will not interpret variables inside of single-quoted strings. That block should be: echo "<b><u>Event Name:</b></u> $eventname"; echo "<b><u>Event Date:</b></u> $eventdate<br>"; } ?> -
1) Do you have error reporting turned on? Put these to lines at the beginning of your PHP code in newsfeed.php. error_reporting(E_ALL); ini_set("display_errors", 1); 2) Forget you ever heard about the "@" operator. All it does is hide errors. Take it out and fix whatever errors, warnings, notices that code produces. 3) When you get the blank page, use the "View Source" feature of the browser to see if there is any hidden output -- if an open angle bracket is sent to the browser, the browser considers it an HTML tag and will not display the contents until it comes to a closing angle bracket. 4) Are header strings case-sensitive? I can't remember, but I always write it as header('Location: somewhere.php'); Question: If you post an empty news item, do you get sent back to admin-news.php as programmed, or do you get left at a blank newsfeed.php page? If the header is not working then you probably have output being sent somewhere, and the header error is not being displayed because you don't have error reporting on.
-
The header error usually tells you the file and line number where the output started. This would be the place to start looking. Post the entire error message that you are getting.
-
Look at the first line and last 2 lines. $dbQuery contains the data you are retrieving from your SELECT on the events table. You are trying to execute that value (i.e. BBQ) in the next-to-last line here. I don't see the sense in that if statement being there at all. It would be more useful if you tested the result of mysql_query immediately after calling it (the first line). However, since you have nested it inside of a call to mysql_result, there is no way to test it. I recommend breaking up that first line into several lines: $innerSql = "SELECT ..."; $innerResult = mysql_query($innerSql); if ($innerResult === false) // some error stuff here for each ($innnerData = mysql_fetch_assoc($innerResult)) { // handle data here ...
-
Can a server answer a GET request with a POST response?
DavidAM replied to jhsachs's topic in PHP Coding Help
For clarification, the solutions I offered would not ordinarily be my first choice either. However, I have often come to a situation where the design and system requirements required a "non-standard" solution. In this case, the OP has indicated that he can not or will not reveal detail design issues. That may be a valid decision based on proprietary issues and / or corporate mentality; or it may be that the OP just thinks he knows the best approach and just wants an answer. Either way, I decided to provide workable solutions. -- By the way, there is a third solution, which would be my personal last-ditch approach, and that would be to use flash for the whole thing. 1) JavaScript is not reliable. I'm not saying it does not work, I'm saying you can not depend on anything that the user has control over. A savy user (i.e. most of the people answering questions on this board) can intercept your JS and make it do whatever we want. 2) The browser is not reliable. Again, just about anybody can manipulate the "hidden" form that I recommended. 3) Cookies are not reliable (see 1 and 2 above). In Short, JS should never be used as a security or validation technique. Always design and develop the site to work entirely without JS. Then add JS to enhance the user's experience. It is fine to validate data in the browser with Javascript, as long as it is validated at the server when it gets there. Validating with JS is only a convenience for the user, saves time and band-width; it is NOT part of the application's data validation. PFMaBiSmAd is also correct. I have spent many many hours writing code to circumvent problems because that was what the managers said to do. I almost lost my job (working for a major consulting company, at the time) because I told the project manager we needed to fix a design conflict between two modules (designed and in development by a competitor) instead of writing and executing code every-other day to fix the data that the two modules were updating inconsistently. In the end, they had to address the design conflict, but not before the customer had spent lots of dollars fighting it. (By the way, it was the customer who refused to address the issue.) -
Can a server answer a GET request with a POST response?
DavidAM replied to jhsachs's topic in PHP Coding Help
We went "off the rails" trying to help you solve a problem that, perhaps, we don't completely understand. The literal answer to your question is, as Adam said in response #1, there is no such think as a "POST response". If you want to turn all of your links into POSTs (at the browser), and if you consider JavaScript as part of the solution set -- keeping in mind that the user can turn off JavaScript and thereby defeat your protection -- you can place a form on the page with a hidden field and no visible elements, and apply an onClick event to your <A> tags. Then have the onClick event change the Action of the form to the destination of the link, set the hidden field to whatever value you want passed to the new page, and then POST the form from JavaScript. As an alternative to POSTing a hidden form, you could have the JavaScript event put the value into a cookie and then allow the browser to issue the GET request. Of course this requires the user to have JavaScript AND Cookies enabled. You will, likely, want to have your PHP script verify that the Request Method is POST (in option 1) or that the COOKIE exists (option 2). I'm not terribly good with JS, and would probably choose option 1, since I find working with cookies in JS to be tedious. In either case, I would be using JQuery to simplify my life as much as possible. -
I'm surprised that with everything else PHP tried to do to "help" developers -- magic quotes, register globals, etc -- they did not have the setcookie() function go ahead and create the $_COOKIE entry. However, the reason it does not work is that setcookie() queues up a header to be sent to the browser when the webserver is ready to send. The browser sends cookies when it requests a page. So, at the time that this script was requested (by the browser), the browser did not have a cookie to send, and PHP did not have anything to put in the $_COOKIE array
-
You probably left out the most important part. If you are using setcookie to set the cookie, it will NOT be available to PHP until the next page load -- it is NOT available to the current script. Maybe you could "fake" it by setting $_COOKIE after the setcookie in that code -- I don't know, I've never tried; but I don't see why not.
-
trying to avoid session variables being destroyed
DavidAM replied to imsewhi's topic in PHP Coding Help
Since you have not posted any code, we have to shoot in the dark Are you saying the index.php page has a form - that form is posted to a third-party - and you want the values from that form (the form on your index.php page)? Or is there another form before that one. The POST (from index.php) went to the third-party script NOT to your ThankYou page. And, most likely, they are sending you a GET, and sending it WITHOUT your POSTed values. If you want those values, you will have to find a different way to get them. 1) Post to your own processing script and use curl to POST to the third-party 2) Create an intermediate script to collect your data and present another form to post to the third-party. -
Can a server answer a GET request with a POST response?
DavidAM replied to jhsachs's topic in PHP Coding Help
After reading through both posts, I get the impression you require the user to visit every page in the "course". Or do you require that they visit every page in sequence? If you want to make sure they go in sequence then I would have the last action of each script be to set $_SESSION['LastPage'] = $MyPageNumber. Then have the first action of each script be to compare $MyPageNumber to the session and emit message if they are out of sequence. If you just want them to visit/complete every page, I would add an array to the session at the start of the "course": $_SESSION['Pages'] = array(1 => false, 2=>false, 3=>false ...); then as each script decides that the user has satisfied the requirement of "visiting the page", you could mark that page's value to true. When you get to the end page, you check the array for any false values and tell the user to go back and complete that page. Rats! someone beat me again! PFMaBiSmAd's suggestion can be merged with mine (which is of course a better suggestion ), so I'm going to post it anyway. -
When a system() call works from the command-line (in putty) but does not work from a webpage, the problem usually has to do with who and what is running. When run from the command line, the script is running as you (or whoever you logged in as) with your access rights and your environment. When run from a webpage, it is running as the webserver NOT as you. On a well configured system, the webserver user has tightly restricted privileges and environment. You have to figure out the difference between these two environments and work around it. Try creating a script with JUST a call to phpinfo(). Run it from putty and redirect the output to a file. Then run it from a webpage with the output redirected. Compare the two files. Look especially at the ENV PATH values and the PHP include paths. Add a call to system("id"); to the script. That should tell you the user running the script as well as the groups it belongs to. Check the permissions on the directories and files you are trying to access in the system() call -- the webserver user (or group) will have to have read access to the script you are running and will have to have write access to any directories and files it needs to write to, it will need execute access to any directories and sub-directories it needs to traverse (access).
-
It is possible the the PATH environment variable for the webserver does not include the path for the php command-line executable. Find out where it actually is and change the command you execute to include the full path for php /usr/bin/php myphpscript.php ...
-
Deleting multiple rows from mysql table in php using checkboxes
DavidAM replied to ggw's topic in PHP Coding Help
<input name="delete_these[]" type="checkbox" id="checkbox[]" value="<?php echo $row['del_id']; ?>"> Is there really a column named del_id in your table? I think that should be the msg_id (the primary key on the table). Because it looks like your $_GET['delete_these'] array is full of empty values. -
You are looking for Heredoc $message = <<<EOM This all goes into the message variable Right up until we get to the (in this case) EOM but it has to be ALONE on the line with NO whitespace EOM echo $message;
-
You didn't mention the quantity field in your first post. If you want the total of all quantities for a product, you would use SUM(quantity) instead of COUNT(product). Use the same GROUP BY, and you should be fine. SELECT productname, SUM(quantity) FROM daysales GROUP BY productname
-
Multiple Instance of PHP - running php file in n times concurrently
DavidAM replied to tullies's topic in PHP Coding Help
I mis-spoke. The shutdown process does NOT send a SIG_HUP. It sends SIG_TERM to all running processes. Then it will kill any that don't exit. I would still implement the two signals the way I described in my previous post. I just wanted to correct my mistake for the record. (It doesn't count as a real mistake if you catch it yourself, right? -
Multiple Instance of PHP - running php file in n times concurrently
DavidAM replied to tullies's topic in PHP Coding Help
The example from kicken is pretty much the way I would do it. In your case, I think you would add your scheduler check here: while (count($runningPIDs) < MAX_PROCESSES){ /* Check the schedule table here and sleep if nothing to do The checkSchedule() function should return the database ID of the task to be executed or FALSE if there are no tasks waiting */ while (($IDtoRun = checkSchedule()) === false) sleep(##); echo "Launching worker process."; if (($pid=pcntl_fork()) == 0){ RunProcess($IDtoRun); } You have to make sure the checkSchedule() marks the record as being sent to the scheduler somehow. Otherwise, you could launch several processes for the same ID before the first one gets to the point of marking it. While the child process "differs from the parent process only in its PID and PPID", it seems to me that your database connection (and other resources) do NOT get properly replicated. In fact, it seems I had to close the connection before calling fork() and then re-open the connection in both the child and the parent. When you fork(), the process is replicated and the new process starts running in the same spot. This is the reason for the if test in kicken's code. The fork() returns the child's PID to the parent. So the if(($pid=pcntl_fork()) == 0) statement will be TRUE in the CHILD and will be FALSE in the PARENT. I think this was the most confusing point when I first used fork() (in a C utility). -- The CHILD does NOT start at the beginning. kicken's example also includes a check for a file to stop the process. This is a good idea, as just killing the task can leave things in a questionable state. You also need to consider, what would happen if multiple launchers are started. Most *nix services like this, check for a "pid" file at start-up. If this file already exists, the service will print an error message and exit. If it does not exist, the file is created and the PID is placed in it. When the service exits, it will remove the file. Since the file contains the PID (process ID) of the running service, if you need to kill it from the command line, you can look at the contents of this file to get the PID and issue your KILL. You should consider using pcntl_signal to install a signal handler that will intercept the SIG_HUP and SIG_TERM and exit cleanly. Otherwise, the PID file will not be removed and child tasks will become zombies on exit. I would implement SIG_HUP as a command to not start any new tasks. This allows all running children to exit normally and when there are no more children, the parent would exit normally. (This is how kicken's code behaves when it finds the stop file. I would implement SIG_TERM to have the child tasks exit immediately but still have the parent wait and do the clean up. I believe that *nix servers will send SIG_HUP to all processes when a shutdown is issued (i.e. they are rebooting the server). Then a few seconds later a SIG_TERM will be sent to all processes that have not yet exited. Eventually, it sends a SIG_KILL, which you cannot intercept so you just die. For your scheduled tasks, you need to consider a couple of flags in the database. One for "Started" which is set just before forking the process to run it. And one for "Completed" which is updated by the child process just before it exits. When the main process starts, it can update any tasks that are "Started" and NOT "Completed" as NOT Started, so they will be run again. This state will exist if the server dies during a process, or someone kills the child that is running it, or the child ab-ends for some reason. I'm sure there were other lessons learned, but I think that covers the high points. -
You are not actually collecting the data from the form: //This is only displayed if they have submitted the form $searching = (isset($_POST['searching']) ? $_POST['searching'] : ''); if ($searching =="yes") { $nervai = "<h2>Results</h2><p>"; //If they did not enter a search term we give them an error $find = (isset($_POST['find']) ? $_POST['find'] : ''); if ($find == "")
-
Multiple Instance of PHP - running php file in n times concurrently
DavidAM replied to tullies's topic in PHP Coding Help
You are looking for pcntl_fork. This is advanced material, here. If you do it correctly, it can work nicely. If you do it wrong, your host may ask you to leave. You need to keep a count of fork'ed children, so you can limit how many run concurrently; collect them as they exit, to avoid zombies; and not overload the system. If there are no queued tasks, you can sleep, which I think does not use any resources. Or you can exit and have a cronjob scheduled every 5 minutes (or whatever) to start a new scheduler if there is not already one running. I personally prefer this method to avoid memory leaks. -
First, you need to put quotes around the value you are searching for: SELECT * FROM tsue_members WHERE upper(membername) LIKE '%Rastaman46%' But note that you are using the UPPER() function on the column which means you are comparing "RASTAMAN46" (from the column) to 'Rastaman46'. I'm not sure if the that will work even if you are using a Case Insensitive character set on the table. Also, if you are looking for an EXACT match, you don't want to use LIKE. SELECT * FROM tsue_members WHERE upper(membername) = UPPER('Rastaman46')
-
while($row = mysqli_fetch_array($r)) { if($showHeader) { //Display category header $showHeader = false; } while($row = mysqli_fetch_array($r)) { You are never actually displaying the data from the FIRST fetch before you start a NEW loop with a second FETCH. There is no need for the nested while loop. Move the <TABLE> tag and everything else you want to do before the first row up into the if($showHeader). Then remove the second while() statement (and it's closing curly-brace). Note: your closing TABLE tag is going to have to come out of the loop, as well as the closing DIV
-
Just for fun, I decided to test this on a 64-bit Win7 machine. At 18x18 it is almost instantaneous. At 19x19, well ... it's still running. Consider changing your generator. It looks like you are looking for six numbers between 1 and 38, without any duplicates. I suspect it is getting hung up in all those loops looking for unique numbers. But try this. ... still going ... function stargate_address_generator() { $chevrons = range(1,38); shuffle($chevrons); $stargate_address = implode('-', array_slice($chevrons, 0, 6)); return $stargate_address; } The shuffle randomizes the list of numbers. Then we can just take six off the top and have six random numbers without any of them being the same. ... still going ... I just tested that at 35 x 35 and it only paused for half-a-second. ... still going ... I don't think the first one will ever finish. ... still going ... This one actually pukes at around 40 x 40. Are we getting close to the limit here? How many unique combinations are there? Actually, I'm betting it is a problem with the Windows random number generator. I bet everyone else that reported testing it was using Linux (which I prefer myself). ... still going ... --- I'm not waiting anymore -- CTRL-C Well, I see someone else offered a different solution. But, you might want to optimize your generator anyway.
-
Did you try using the GLOB_NOESCAPE flag? See the manual for glob
-
Logical Sequence Mistake or Variable Assignment Mistake
DavidAM replied to cosmic_sniper's topic in PHP Coding Help
<form action="output.php" method="post" name="a"> req1: <input type="text" name="a_req_1"> req2: <input type="text" name="a_req_2"> . . . <input type="submit"> </form> $form_name = $_POST['a']; if($form_name = "a") { //variables for a goes here The name attribute of the FORM tag is not posted with the form. I have always thought this is a slight failing in the specifications, but they didn't ask me. To determine which form was posted. Add a hidden field to each form using the same name but with different values: <form action="output.php" method="post" name="a"> req1: <input type="text" name="a_req_1"> req2: <input type="text" name="a_req_2"> <INPUT type="hidden" name="whichForm" value = "a"> . . . <input type="submit"> </form>