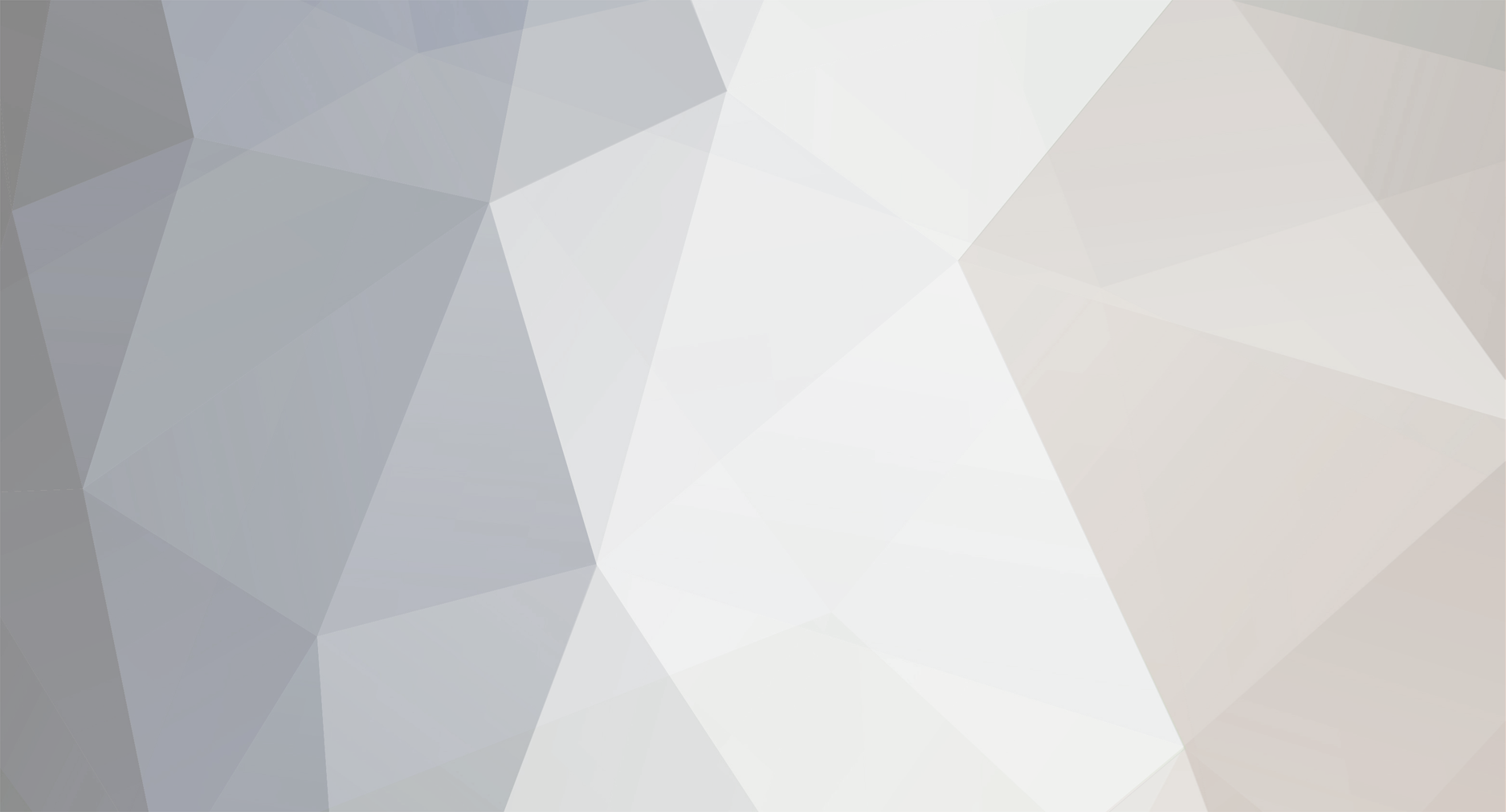
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
There is a setting in the mySQL configuration file that affect this. It will either truncate to the defined (maximum) length and issue a warning; OR it will raise an error (and not insert the data at all). See the mySql manual [EDIT] It is good to be aware of these things, since the setting may be different between your development platform and the production server; which could cause errors to start occurring when moving from one server to the other. [/EDIT]
-
In your echo statement, where you are building the IMG tag, you used a comma between the $site and $row['picture'] variables. But you were using periods everywhere else. In echo, this works so: $var1 = 'Hello, '; $var2 = 'World'; // These two statements produce the same output echo $var1, $var2; echo $var1 . $var2; // However, the comma will not work in other places $statement = $var1, $var2; // NOT valid $statement = $var1 . $var2; // Valid print($var1, $var2); // NOT valid print($var1 . $var2); // Valid Mixing the two in that long statement, made it difficult to read (or maybe I'm just not used to seeing them). It is just usually better to be consistent. If you only have one row, you can leave the while out and just retrieve the data. $query = "SELECT * FROM shows"; $result = mysql_query($query) or die(mysql_error()); $row = mysql_fetch_array($result); // No WHILE loop here echo " ... <a href='". $site ."show_page.php?". $row['id'] ."' name='show_id' value='". $row['id'] ."'> <img src='". $site,$row['picture'] ."' height='180' width='180'></img></a> ... "; // Took out the closing brace for the while we took out
-
Unless I am reading the code wrong, you are not generating a valid HTML link in the show_list() function. In the code you have (I chopped part of it out): // Just some values for the example $site="http://www.mysite.com/" $row['id'] = 4; $row['picture'] = 'theshow.jpg'; // Your code echo " ... <a href='". $site ."show_page.php?". $row['id'] ."' name='show_id' value='". $row['id'] ."'> <img src='". $site,$row['picture'] ."' height='180' width='180'></img></a> ... "; I think you will get: <a href='http://www.mysite.com/show_page.php?4' name='show_id' value='4'> <img src='http://www.mysite.com/theshow.jpg' height='180' width='180'></img></a> which is not generating a link that will help you at all. I think you need: // Just some values for the example $site="http://www.mysite.com/" $row['id'] = 4; $row['picture'] = 'theshow.jpg'; // Your code echo " ... <a href='". $site ."show_page.php?show_id=". $row['id'] ."'> <img src='". $site . $row['picture'] ."' height='180' width='180'></img></a> ... "; which should look like: <a href='http://www.mysite.com/show_page.php?show_id=4'> <img src='http://www.mysite.com/theshow.jpg' height='180' width='180'></img></a> Also, when you are using a query string (the "?" on the link), you get the values from $_GET not from $_POST. So, your show_page() function should be using $_GET instead of $_POST. Other things in that code you might want to think about: 1) For the most part, you are using concatenation (i.e. "A" . "B") , but in a couple of places you use the comma (like in the image source). The comma works fine in an echo statement, it just separates variables to be echoed. But anywhere else, you would want concatenation there as well. It confused me for a while (with all of the quotes and stuff). It is generally a good idea to pick one method or the other and be consistent. 2) You are only using a couple of fields from your select statement. For performance, it is best NOT to use "SELECT *" (unless you need every field in the table). You should list the fields you want in the select statement ("SELECT id, picture, show, `description-short` ..."). 3) Using a dash in a column name is not a good idea in SQL. The server will think it is a subtraction statement unless you use the back-ticks (as I did in #2 above). Use an underscore ("description_short") or camel case ("descriptionShort"). 4) In show_list(), you are generating an HTML table for each and every show. Unless there is a reason to have that many tables, I would start the table before the while-loop and close it after the while-loop, and then create a row ("<TR>...</TR>") for each record inside the while-loop. 5) I don't know why you have a while-loop in the show_page() function. I would think there would be only one row with the specified show_id. It doesn't hurt, much; but for a single-row result, it is completely unnecessary. If you leave it in, see #4 above. 6) AyKay47's responses are also valid (just substitute $_GET in place of $_POST).
-
I am not an OOP expert. But one point seems to have been missed in this conversation. A Class constant, as in your original example, is a member of the CLASS and is NOT a member of the OBJECT. This is the reason for the different way that you reference it. Your second code sample would more closely resemble your first if it were: <?php //Classes// class Circle { static $pi = 3.141; public function Area($radius){ return self::$pi * ($radius*$radius); } } //Program Variables// $circle = new Circle; //Program Code// echo $circle->Area(100); ?> The static keyword makes the $pi property a class property. It belongs to the class and not to the object. If the variable's value were changed by a program, it would change for ALL of the objects instantiated from the class. Since it is a member of the class it is referenced using the self:: construct (or using Circle::$pi, in this case). So, the answer to your underlying question as to why use $this-> vs. using self:: is not because of the constant. It is because of the scope or ownership of the element being referenced. Perhaps one of the OOP guys can explain it better. And, by the way, there is no way (in PHP) to create an "Object Constant". Constants (in a class) always are static class constants.
-
HTML is, by definition, output sent to the browser. That <DIV> tag just before your include, is being sent to the browser, as is the "title, css and what not ..." before it. IF this was working on another site, it may have had output buffering on, which would capture the output (and buffer it) until the page was done. I don't consider that to be a solution, but it might be an explanation.
-
The error message tells you where the output started: Have a look at index.php, somewhere around line 10. Some kind of output was sent to the browser there. You cannot have any output to the browser before a session start (which has to send headers). Anything in your code that is not between php open and close tags is sent to the browser. Also, you should avoid using the short tags ("<?"), instead, use the full tags ("<?php"). Also, there is no real reason to jump in and out of PHP like that: <?php // Some PHP code here ?> <?php // more PHP code here // ... and nothing between the close and open tags above ?> You should just stay in PHP. Otherwise, that blank line between the close tag and the open tag will be sent to the browser <?php // Some PHP code here // more PHP code here ?>
-
You are checking for the error before you execute the query; and then executing the query in the elseif. Try rearranging it a bit: $con = mysql_connect("localhost"); if (!$con) { die ('Could not connect: ' . mysql_error()); } mysql_select_db("subscribe", $con); $sql="INSERT INTO subscribe_init (id, notes) values ('$_POST[id]','$_POST[notes]')"; if (!mysql_query($sql,$con)) if (mysql_errno() == 1062) echo 'You are already subscribed.'; else die ('Error: ' . mysql_errno() . mysql_error()); else else echo "Thank you for signing up!"; mysql_close($con) or something like that. Disclaimer: There are other issues with that code. This answer applies only to the original question.
-
mysql_fetch_array returns an array of data representing each row selected. So your code: while( $cred = mysql_fetch_array($r) ) { if ($cred >= '1' ) is not correct. $cred is an array and the if test will never be true. Looking at the rest of your code, you should probably using: if ($cred['user_credit'] >= 1) The user_credit column really should be numeric so you don't need to use the quotes around the number 1.
-
If the ID column is a numeric type, the answer is rather simple: $ids = array(2, 4, 8, 16); $sql = 'SELECT columnName from TableName WHERE ID IN (' . implode(',', $ids) . ')'; If the column is a string type, you just have to add a couple of quotes in the right places: $ids = array('I2', 'D4', 'T8', 'Q16'); $sql = 'SELECT columnName from TableName WHERE ID IN ("' . implode('","', $ids) . '")';
-
function BBCodeToHTML($text) { $text = trim(htmlentities($text)); The first line of your BBCode function is converting all HTML to HTML Entities; which is proper. So the <IMG > tag is changed to be displayed rather than interpreted. You should probably be using the BBCode tag for an image ( .
-
$sql = "SELECT * FROM players WHERE ('$search_by') LIKE ('$search') "; If you echo out your SQL, you should see a problem. You have quotes around the $search_by which tells the database it is a literal string NOT a column name. And why do you have parenthesis around that and the search value? $sql = "SELECT * FROM players WHERE $search_by LIKE '$search' ";
-
Php problem- Syntax error, unexpected t_string line 3
DavidAM replied to Weakwill's topic in PHP Coding Help
Let me rephrase: Two single-quote marks do not a double-quote make. Look at your code. There are three separate lines in the code you just posted where you have used two-single-quote marks as double-quotes (twice in each line). -
What is the best way, of replacing array values?
DavidAM replied to diablo3's topic in PHP Coding Help
$non_rand_arr = array(1, 2, 3, 4); $rand_arr = $non_rand_arr; shuffle($rand_arr); $team1 = array (3, 4, 3, 4, 1, 2); foreach ($team1 as $key => $value) { $newKey = array_search($value, $non_rand_arr); if ($newKey !== false) $team1[$key] = $rand_arr[$newKey]; } This looks at each value in the $team1 array; searches for it in the NON Random array and gets it's key from there; then sets the $team1 element to the value from the RANDOM array with the same key. If a value in $team1 is NOT in the non random array, its value does not change. Although, if the values in the non-random array are always consecutive, you could just shuffle that array, and use the value returned by array_search (plus one) as the new value for the team array. -
Actually, you would want to normalize this into two separate tables: OrderHeader - order_id, customer_id (FK), store_id(FK), order_date, order_status, etc. OrderLines - order_id (FK), line_no, product_id (FK), line_qty, line_status, etc. There is no sense in repeating all of the "header" data multiple times. And for the record: Anytime you are thinking about having multiple tables with the same layout, you almost certainly need to re-think the design. The database server (and your application) will handle one very large table much more efficiently that multiple small ones.
-
AS expression contains an IF statement - not working
DavidAM replied to dmirsch's topic in MySQL Help
You don't really need the calculation in the SELECT clause. And you don't need the LEFT Join (as that will return Venues that have NO performance records -- although the WHERE clause is going to remove them). I think the query below will work, I don't have a system to test it on here. SELECT DISTINCT venues.VenueCode, venues.VenueName FROM venues JOIN Performance ON venues.VenueCode = Performance.VenueCode WHERE venues.VenueCode!="VV" AND Performance.startDateTime >= DATE_SUB(NOW(), INTERVAL 1 DAY) ORDER BY venues.VenueName Be aware that NOW() returns a DateTime, so the result may be different at different times of the day. You might consider using CURRENT_DATE() instead. -
1) Using SELECT * will return all the columns from both tables for every matched row. The PHP engine has to buffer this data, so if there are a lot of columns, this could reduce through-put. It is almost always best to select only the columns that you will need. 2) Run the query directly in the database and see if the "slow down" shows up there. 3) Is postcodes.Pcode indexed? Is cars.Location indexed? Are both of those columns the same datatype? If one or the other is not indexed, you will end up doing a table scan to find all of the records. If the datatypes are different, the server is having to do a bunch of data conversions. 4) Use EXPLAIN to see what the query is doing -- what indexes are used, how many rows are scanned, etc. Post the results and we can talk about it. 5) It could be that your PHP processing of the results is causing a problem (array copies, etc). Post a little more of the code and we can see if we can help.
-
Column count doesn't match value count at row 1
DavidAM replied to smproph's topic in PHP Coding Help
Yeah, I was just showing an example. Last time I provided an example using the OP's code, with the $_POST intact, I got blasted for not sanitizing the inputs. So, I thought I would just give an example and hope the "programmer" could understand the concept and translate to his/her needs. By the way - It is NOT a good idea to use $_POST (or $_GET or even $_COOKIE) directly in a query without sanitizing them first . So, to expand the example: $values = array(); foreach ($_POST['participant_name'] as $pName) { $values[] = sprintf("('%s', %d, '%s')", mysql_real_escape_string($pName), int_val($_POST['team'], mysql_real_escape_string($sport)); /* UNLESS the team_no column is a VARCHAR or CHAR or TEXT in which case it would be: $values[] = sprintf("('%s', '%s', '%s')", mysql_real_escape_string($pName), mysql_real_escape_string($_POST['team']), mysql_real_escape_string($sport)); */ } $sql5="INSERT INTO participants (participant_name, team_no, sport) VALUES " . implode(',', $values); I'm guessing we are using mySql here. If not, use the appropriate escaping mechanism for your database of choice. -
Column count doesn't match value count at row 1
DavidAM replied to smproph's topic in PHP Coding Help
It is definitely your implode function call. It took me a while to figure out what you were trying to do. And when you come back to this script in a few months to make changes, it will take you a while to figure out how to do that. Just off the top of my head, a cleaner way to write that would be: $team = 4; $pnames = array('DavidAM', 'smproph', 'Pikachu2000', 'xyph'); $sport = 'programming'; $values = array(); foreach ($pnames as $pName) { $values[] = "('$pName', '$team', '$sport')"; } $sql5="INSERT INTO participants (participant_name, team_no, sport) VALUES " . implode(',', $values); There may be an even more elegant way of doing it, but that's worth more than twice what you paid me for it. -
AND takes precedence over OR so basically, that statement says: SELECT * FROM users WHERE username LIKE '%$idea%' OR fname LIKE '%$idea%' OR lname LIKE '%$idea%' (OR tags LIKE '%$idea%' AND private = '$priiv') So it only checks the private column when checking the tags column. You need to use parenthesis to group the OR's all together: SELECT * FROM users WHERE (username LIKE '%$idea%' OR fname LIKE '%$idea%' OR lname LIKE '%$idea%' OR tags LIKE '%$idea%') AND private = '$priiv' or, rearrange it to something easier to read (IMO) SELECT * FROM users WHERE private = '$priiv' AND (username LIKE '%$idea%' OR fname LIKE '%$idea%' OR lname LIKE '%$idea%' OR tags LIKE '%$idea%')
-
You can use the Referrer, BUT the information it contains was SENT BY THE VISITOR. If I was going to spam somebody's registration page, I would set the referrer to their site so it would not think it was spam. Captcha is a good way to reduce this. You can also generate a hash and store it in the session and in a hidden form field. If the hash you receive with the form does not match the hash in the session, they probably submitted a modified or counterfeit form. You can also store a timestamp in the session, and check that no less than 5 seconds and no more than 60 seconds passed between sending out the form and receiving the post. This can help prevent automated processes, which would be real fast; and copied forms, which would be a real long time. You would, obviously have to fine-tune the number of seconds on both ends based on the amount of information the user has to enter and how tightly you want control. If you ever figure out a way to prevent it 100% ... patent it, copyright it, and sell it. In other words, as long as we accept the anonymous nature of the Web, there will always be people hacking our sites.
-
You can also use the pre-defined constant PHP_EOL, which contains the proper line ending for the platform PHP is running on. fwrite($fh, PHP_EOL);
-
Oh, boy! The ads are back. Thank God, I was suffering from withdrawal.
-
unable to print distinct dates and display in a specific format..
DavidAM replied to php_begins's topic in PHP Coding Help
That looks like a unix timestamp not a datetime (which is an SQL data type). Hopefully, you have that defined as an integer and not a varchar (or other string type). There are a couple of issues with your code that I would cleanup. Do not use 'select *' and mysql_num_rows() to determine how many rows exist, use 'select count(*)' instead. You are wasting a lot of time and resources that way. Also, do not run queries in a loop if you can avoid it. I would suggest writing that (first) process along these lines: $sql = "SELECT DATE_FORMAT(FROM_UNIXTIME(dateline), '%Y-%m-%d')) AS FmtDate, COUNT(postid) AS PostCnt FROM post WHERE dateline >='$limit' GROUP BY DATE_FORMAT(FROM_UNIXTIME(dateline), '%Y-%m-%d')) DESC"; $res = mysql_query($sql); if ($res !== false) { while ($row = mysql_fetch_assoc($res)) { echo 'Date: ' . $row['FmtDate'] . ' Posts: ' . $row['PostCnt']; } } That's just off the top of my head. You will have to add all of your HTML and stuff where the "echo" is; but that should give you an idea. I don't know why you were using ">=", unless it was because of the TIME portion of the timestamp. The query above should give you post counts by date, regardless of time. Also, I do not see where you are using the $acronym variables to limit the queries; so I'm not sure how you would incorporate that. This concept should work for your other tables, look into JOINs and aggregate functions (in mySql) to see how to work that. If you get stuck on the other queries, post your work and we can take a look. -
$_FILES is a global array that is created when a file is uploaded from a web page using a <FORM> tag. This array is not going to exist in a cron job. Since you are using if ( ! empty($_FILES)) { at the beginning of the script, the code inside the IF is not going to execute.
-
In every configuration I have ever used, $_GET is always defined, so your IF statement will always be true. You need to test for a specific index in the $_GET array. I usually use isset: if (isset($_GET['page'])){ /* NOTE: The double quotes around the entire $_GET[] expression are not needed; however, single (or double) quotes are needed around the index */ switch($_GET['page']) { case "news": include "news.php"; break; case "is-it-for-me": include "for_me.php"; break; } }