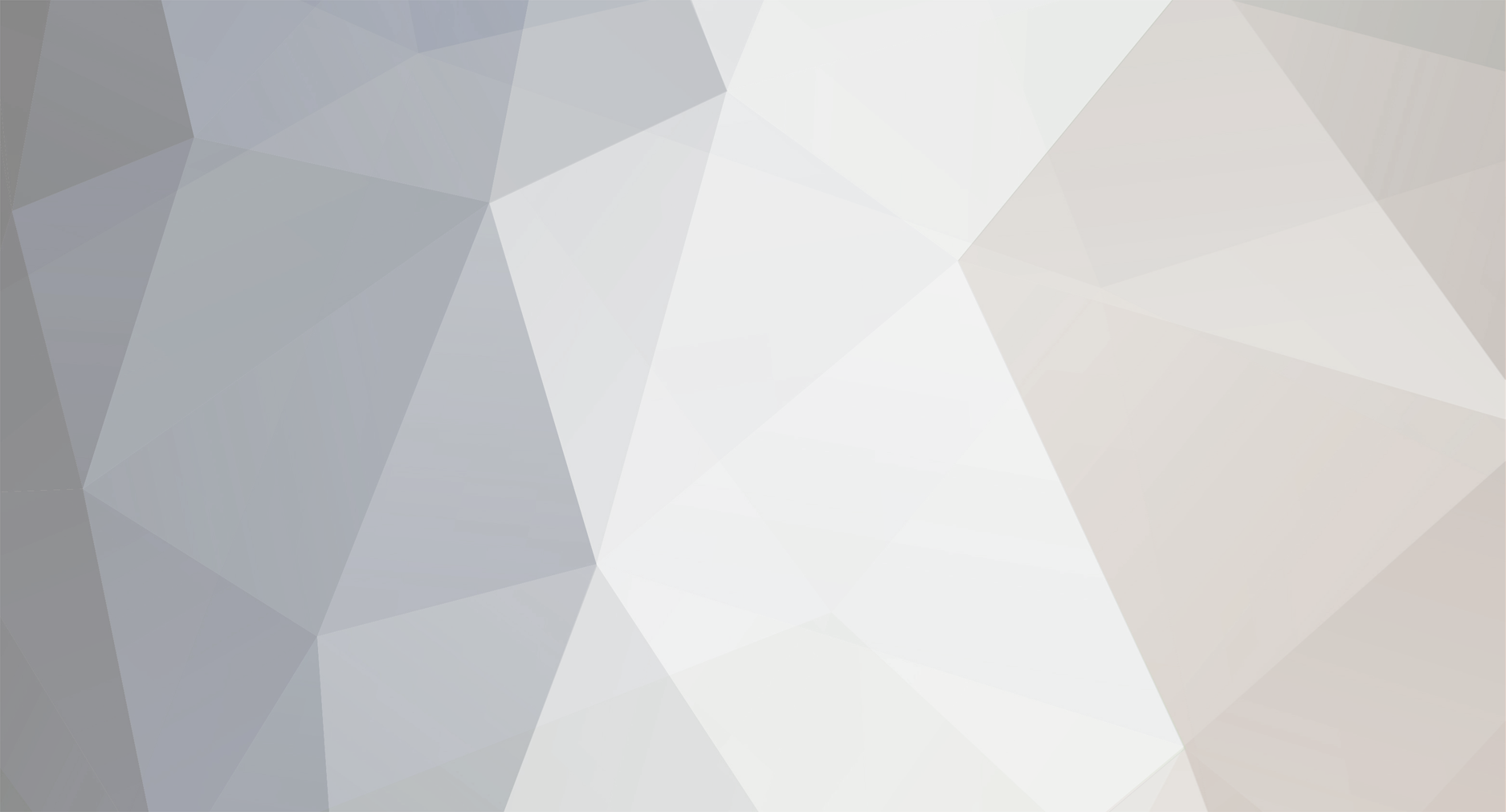
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
Probably should. I would not insert the information into the database until I have verified that the file is valid, and stored it in it's final destination. Otherwise, you may end up with database entries that reference files that do not exist. Around line 233 might be a good place. if ($success) { // INSERT INTO DATABASE HERE ... $result = "$thumb_name and $workout inserted sucessfully."; } I didn't walk through the entire script, so there may be some issues to workout when you move it (and what you move), but I think that is where it needs to be.
-
Actually, the "WHERE 1" seems to work on my server, although I don't recommend it. It looks like it was put there so the rest of the statements building up the SQL don't have to worry about whether the WHERE has already been added and can just always use AND. I would make it "WHERE 1=1 " because it makes it more clear to me that it was done on purpose. Where are you trying to add the ORDER BY? I think it would work if you added it after the last if test. function search() { //base sql $sql = "select * from behan WHERE 1"; //get the values from the form if ((!empty($_POST['beha']))&&($_POST['beha'] != 'all')) { $sql .= " and beha like '". addslashes($_POST['beha'])."%' "; } if ((!empty($_POST['omraede']))&&($_POST['omraede'] != 'all')) { $sql .= " and omraede like '". addslashes($_POST['omraede'])."%' "; } if ((!empty($_POST['pr']))&&($_POST['pr'] != 'all')) { $sql .= " and pr = '". addslashes($_POST['pr'])."' "; } // ADD ORDER BY $sql .= ' order by total_value DESC '; On the other hand, your error message says: where did that first set of ?'s come from? The code you presented is using column names there. You need to echo $sql before you execute it, and possibly at various other places, to find out where this is coming from.
-
Quick & Simple question. [PHP with Javascript]
DavidAM replied to Joshua4550's topic in PHP Coding Help
Make sure you include the array brackets on the name when you name it using Javascript or it will not be part of the array: <FORM action="" method="POST"> <INPUT type="text" name="txtData[]"> <INPUT type="text" name="txtData[]"> <INPUT type="text" name="txtData[]"> </FORM> When POSTed this will produce an array in PHP: foreach ($_POST['txtData'] as $field) { echo $field . PHP_EOL; } When you add the field in Javascript, you need to make sure you set the name to "txtData[]" so it will be part of the array. Note: I've never actually done this, but my experience scanning through the form elements in Javascript seem to bear this out. -
If this page is on the internet, and you have no other code protecting what file is being removed, it will be VERY easy to erase your entire website once this script gets fixed. I REPEAT: THIS IS A DANGEROUS SCRIPT!!!! The action in your form is using PHP_SELF, which does NOT include the GET variables that were passed in to the script. You will have to add the querystring on the end of that to get the filename passed when the form is posted. OR since you are POSTing the form, you could add a hidden field that contains the file name and then pull it from the POST array. Note: your comment just before unlink() says Rename file, but unlink() deletes the file. I REPEAT: THIS IS A DANGEROUS SCRIPT!!!!
-
You are going to need to use preg_match_all() to do this; at least, that is probably the cleanest solution. I am not very good with regular expressions, but I think it would be something like this: $html = '{POST = test} {GET = testtwo} <html> <title>This is a test</title> <body> Test Body. </body> </html>'; $regEx = '/\{(.*?) ?= ?(.*?)\}/'; $found = array(); $data = array(); if (preg_match_all($regEx, $html, $found, PREG_SET_ORDER)) { // Found at least one foreach ($found as $entry) { $data[$entry[1]] = $entry[2]; } } // now remove the stuff $html = preg_replace($regEx, '', $html); Someone in the PHP RegEx Section may be able to give you a better (correct?) regular expression; as I said, I'm not very good with it. Disclaimer: This code is untested, and may be completely wrong.
-
If you are referring to this insert statement (around line 118) $insertSQL = sprintf("INSERT INTO workout (Name, Type, Hyperlink, `Description`, Image, Profit) VALUES (%s, %s, %s, %s, %s, %s)", GetSQLValueString($_POST['Name'], "text"), GetSQLValueString($_POST['Type'], "text"), GetSQLValueString($_POST['Hyperlink'], "text"), GetSQLValueString($_POST['Description'], "text"), GetSQLValueString("images/".'$thumb_name', "text"), GetSQLValueString($_POST['Profit'], "double")); I see two problems with it. First, $thumb_name has not been defined at this point in the program, so it has no value. Second, you have surrounded $thumb_name with single-quotes, so it will not be evaulated, but will be inserted as literally '$thumb_name'. There is no need to put quote marks around the variable in this particular statement. I did not really go through the rest of the code, so there may be other issues as well.
-
How about this: $idSELECTED = 8; $query = "SELECT * FROM videos WHERE ID IN ($idSELECTED, (SELECT MAX(ID) FROM videos WHERE ID < $idSELECTED), (SELECT MIN(ID) FROM videos WHERE ID > $idSELECTED) )"; The second two SELECT statements are inside of the IN () phrase. Hopefully, your ID field is indexed (Primary Key?) so these will run quickly. Of course, if your ID field will ALWAYS be sequential, with NO gaps (from DELETES or so forth) then you can simply do: $idSELECTED = 8; $idBEFORE = $idSELECTED - 1; $idAFTER = $idSELECTED + 1; $query = "SELECT * FROM videos WHERE ID IN ($idSELECTED, $idBEFORE, $idAFTER)";
-
SELECT DISTINCT cat1 FROM table UNION DISTINCT SELECT DISTINCT cat2 FROM table something like that, I'm not sure if all of the DISTINCTs are needed
-
2 databases simultaneously update in one script?
DavidAM replied to vijdev's topic in PHP Coding Help
Unless you are using mysqli and multi_query, you cannot put a use statment in with the query to be executed i.e. this will not work mysql_query('USE database1; UPDATE table ...'); You could select the database just before executing each query mysql_select_db('database1'); mysql_query('UPDATE table ...'); mysql_selectdb('database2'); mysql_query('UPDATE table ...'); but it is not strictly necessary. As long as every table reference is qualified by a database name, you don't have to specify a default database. Also, if you do specify a default database, the is nothing preventing you from access data in another database (on the same server). mysql_select_db(database1); mysql_query(SELECT a.Name, b.Name FROM Users a JOIN database2.Users b ON a.ID = b.ID -
2 databases simultaneously update in one script?
DavidAM replied to vijdev's topic in PHP Coding Help
you will have to have two different connections $cn1=mysql_connect(server, user, password, true); $cn2 = mysql_connect(server, user, password, true); mysql_select_db(database1, $cn1); mysql_select_db(database2, $cn2); mysql_query('UPDATE ...', $cn1); mysql_query('UPDATE ...', $cn2); If the databases are on the same server, you can use a single connection and qualify the table names with the database name: mysql_query('UPDATE database1.tablename ...'); mysql_query('UPDATE database2.tablename ...'); -
MySQL Error where there was never one before...
DavidAM replied to SquattingDog's topic in PHP Coding Help
In line 79, $catQuery is the result of executing an INSERT statement. The mysql_query() function returns a boolean in this case (true or false). There are no rows to fetch so you should not be calling mysql_fetch_array() here. -
Try wrapping the separate tests in parenthesis: if ( ($_POST['user'] == $realuser) && (md5(md5($_POST['pass']) . "rs-ps") == $realpass) ) {
-
INSERT INTO tablename VALUES (...) requires you to provide a value for every column in the table, in the order they exist in the table. It looks like you tried to take care of that by adding the '' as the first value. Unfortunately, an empty string is not a valid value for a numeric field (which is what your UID is). So you get an error. If you insist on not listing the columns in the input statement, then put the word NULL there (not in quotes). Personally, I always name the columns so the code is clearer when I review it later. Also, if the table structure changes later, the insert will not fail because the column count is always correct. If you name the columns, you do not have to provide a value for the auto_increment column: INSERT INTO mytable (column1, column2, column3) VALUES (25, 'something', 'end of line.');
-
Any database is going bog down if you don't maintain it. Every now and then the data and index pages need to be rebuilt just to keep things running optimum. Access has a command to Compact the database. You should run this command to optimize space. Even if you move it to mySql, or any other database, you will need to run maintenance commands periodically or it will begin to degrade as well. Make sure you have indexes that make since for your queries. If you often query by Serial Number, you might want to consider adding an index on it. Indexes do not have to be unique, and they can be composite (i.e made up of multiple fields). Indexes take up additional disk space, so you have to balance speed for space (but space is cheap these days). Define foreign keys on your tables: The Customer Number in the Machine table is a foreign key (to the Customer table). Depending on your version of Access (or any other database), this automatically indexes the column; which makes sense. Think about this carefully, you can define a foreign key to prevent deletion of the parent (Customer) record if there are child (Machine) records. However, you can also define the foreign key to Cascade Deletes - if you delete the Customer it automatically deletes the Machines. Having said that, do you really want to delete customers or machines. You lose history that way. Consider adding a flag "InActive" to the tables rather than deleting.
-
replace $userid with whatever variable holds the id of the user that is currently logged in. This tells the query not to look at the current user's record, we expect the icq to match on that record and we don't care that it does because it is the same record we are about to update.
-
In SQL if you do not name the columns in an INSERT statement, the data is applied to the columns in the order they are defined in the table. So you first example INSERT INTO TABLE1 VALUES('James','Japan') would try to put 'James" into the ID column and 'Japan' into the Name column. Of course this will fail because the ID column is an integer and 'James' is not. So, yes, you would have to name the columns as in your second example: INSERT INTO TABLE1 (Name,City)VALUES('James','Japan') (by the way, you need a space before the VALUES clause). As an alternative, you could order the columns in the table putting ID last, and then not have to name the columns in the INSERT (your first example would work). Since there are only two values being inserted, the third (and any remaining columns) would be ignored and receive their default value. I am not advocating this table layout. I have never seen it done and it does not "feel" right to do it this way. But in theory it should work. I recommend ALWAYS naming the columns in the INSERT statement so when you are reading the code you can see exactly what is happening without having to refer to the table layout when you (or someone else) has to modify the code later.
-
add AND id != $userid in place of $userid put whatever variable you have the current user's id stored in.
-
or even WHERE IFNULL(folder, '') = ''
-
WHERE folder = NULL will never be true. Because NULL means unknown, nothing is equal to it including another NULL. You have to use WHERE folder IS NULL to find rows that have a NULL value in the folder column.
-
You should add a session_destroy(); after the session_unset();.
-
PHP newbie, small question about putting line break in form
DavidAM replied to JOERI840's topic in PHP Coding Help
Oh, got it. Thought you might be looking for rich ones that don't wear underwear. -
The SELECT would examine more than MAX_JOIN_SIZE rows
DavidAM replied to miniu's topic in PHP Coding Help
1) Did you read the error message and do what it says? You'll also want to check the JOINS The implication here is that you are trying to run a query that is going to have to process and lot of rows; maybe you left out a JOIN or WHERE condition causing a cartesian product. If you echo the query does it look right? Can you run it from the control panel? Do you get the results you expect. I looked at the big query (the first two in the code), and the only problem I see is that you are using "at" as a table alias; my editor flags that as a SQL keyword, is that causing a problem? The last three selects (referee names) look OK as well. You chose not to use a JOIN there but you have the tables joined in the WHERE clause. Without knowing the layout of your tables and the number of rows in each, I don't see a problem with the queries. But I would recommend double checking them before you circumvent a protective measure that could result in some long running queries. Also check "admin.session.php" to see if there are any SELECT statements there. Since we really don't know which SQL it is complaining about. If it turns out that all the SELECTs are fine, then you may want to review your indexing on the tables. -
Are you sure that "B Pack" is in the data? Maybe it is there are "B Pack " -- particularly since you replaced the newlines with a space, change your str_replace to replace with nothing: $data = str_replace($remove, ' ', $data); // change that line to this $data = str_replace($remove, '', $data); see if that works.
-
I'm not as fluent in JavaScript, but I think it would be something like: document.getElementById("DIVid").style.display = ""; at least that's what I had to use in an app I'm working on now. When I tried display="block" it did not work. So I'm guessing that because it is currently set to "none" clearing it causes the default action which is to show the DIV. DIVid is the ID you assign to the DIV in HTML <DIV id="DIVid">...
-
There are a couple of problems with your login page: if ($_POST['password'] == 'guest' && isset($_POST['username'])) { session_start(); /* $username has not been defined yet so you are not going to get a value here, also, you have $username inside of single-quotes, this will NOT evaluate the variable so you are assigning the literal string '$username' to the session. Remove the single quotes and assign $_POST['username'] ***** YOU REALLY NEED TO SANITIZE THAT VALUE FIRST!! **** */ $_SESSION['username'] = '$username'; header("location: index.php"); /* ALWAYS - put an exit(); after a header() redirection. PHP will continue executing code while the browser is deciding what to do. Make this change in your index page as well */ exit(); }