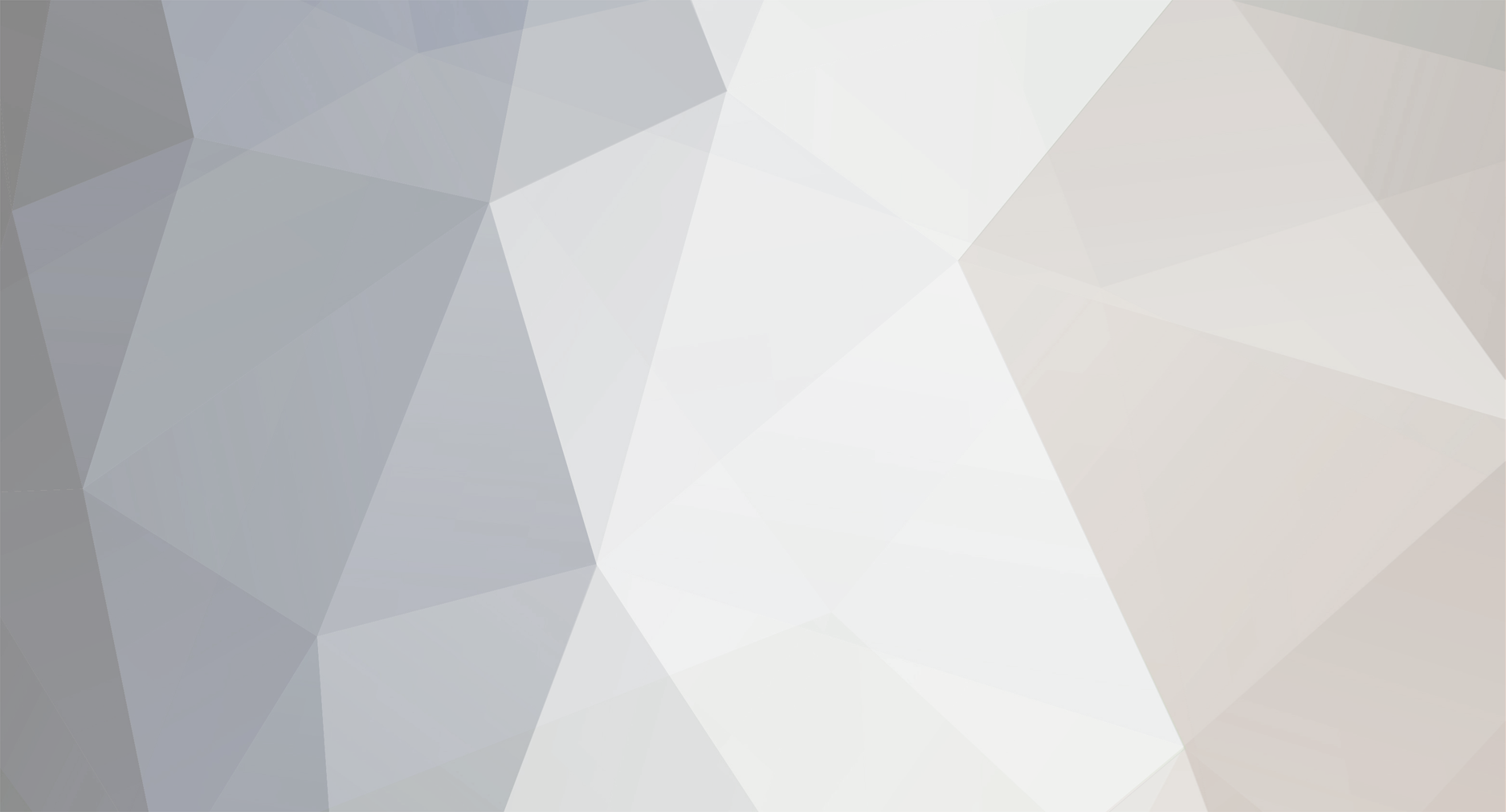
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
[SOLVED] Incorrect Activation Code - Needing help
DavidAM replied to Irresistable's topic in PHP Coding Help
It looks like you sent an email with the activation code. Was that a link that they clicked to get to this page, or is this page from a form where the user typed it? If it was a link, did you urlencode() the email and/or activation code in the <A> tag? If you did, you need to urldecode() them here, if you didn't, then you probably should. If it was a form, did you use GET (or POST) as the method? Also, a couple of notes: 1) your if(!isset) is using AND, so the exit there will only occur if BOTH are blank, I would use OR. 2) use mysql_real_escape() before sending any $_GET or $_POST data to the database otherwise you ar leaving yourself open to sql attacks. As it is if I provide an email of ' OR 'a'='a that query will return every row in your database. -
The code you posted looks fine except I do not see that you have included the error test. The error message you posted says: 1) find line 21 of edit.php, look on it or near it for a call to mysql_num_rows, make sure the parameter is a variable returned by mysql_query. 2) immediately after the call to mysql_query, add the if test from my last post. This should produce some new output. Post it and the code so we can see what the problem is. Also, the query you posted has a space before the word SELECT. This should NOT matter unless it is some other non-printing character.
-
[SOLVED] Quering Multiple Databases for a single field.
DavidAM replied to Errant_Shadow's topic in MySQL Help
Separate queries might be the easiest solution. You can still use a UNION with different data, by supplying null fields in the appropriate place of each query and then looking at only those fields of interest in the result: Example: Table red.subscriptions ID Name Title StartDate ExpireDate Table blue.subscriptions ID Title PaidDate IssueCount SELECT 'Red' as Source, R.ID, R.Name, R.Title, R.StartDate, R.ExpireDate, NULL as PaidDate, NULL as IssueCount FROM red.subscriptions AS R WHERE R.ID = 4 UNION SELECT 'Blue' as Source, B.ID, NULL, B.Title, NULL, NULL, B.PaidDate, B.IssueCount FROM blue.subscriptions as B WHERE B.ID = 4 I think this will make the code more difficult to follow when it comes to maintaining it. It would probably be best to use separate queries, even if you store them in a single array: Note: This is an example, it is not intended to replace your code. Use it to develop code that fits your process. $ID = 4 $Data = array(); // Get subscriptions from "red" $res = mysql_query("SELECT 'Red' AS Source, R.* FROM red.subscriptions AS R WHERE R.ID = " . $ID); if ($res) { while ($row = mysql_fetch_assoc($res)) { $Data[] = $row; } } // Get subscriptions from "blue" $res = mysql_query("SELECT 'Blue' AS Source, B.* FROM red.subscriptions AS B WHERE B.ID = " . $ID); if ($res) { while ($row = mysql_fetch_assoc($res)) { $Data[] = $row; } } This should produce something along the lines of: $Data = array ( [0] => array ('Source' => Red, 'ID' => 4, 'Name' => John Doe, 'Title' => PHP Weekly, 'StartDate' => 2009-10-01, 'ExpireDate' => 2010-09-30 ), [1] => array ('Source' => Blue, 'ID' => 4, 'Title' => PHP Weekly, 'PaidDate' => 2008-09-12, 'IssueCount' => 12 ) ) While the UNION example would produce something very similar but with empty fields in each: $Data = array ( [0] => array ('Source' => Red, 'ID' => 4, 'Name' => John Doe, 'Title' => PHP Weekly, 'StartDate' => 2009-10-01, 'ExpireDate' => 2010-09-30, 'PaidDate' => , 'IssueCount' => ,), [1] => array ('Source' => Blue, 'ID' => 4, 'Name' => , 'Title' => PHP Weekly, 'StartDate' => , 'ExpireDate' => , 'PaidDate' => 2008-09-12, 'IssueCount' => 12 ) -
[SOLVED] Getting single field from table in a field
DavidAM replied to nvee's topic in PHP Coding Help
Not until it is done processing the entire file. -
[SOLVED] Getting single field from table in a field
DavidAM replied to nvee's topic in PHP Coding Help
SELECT DISTINCT year FROM course_student Make note of the fact that you could end up with gaps if there are no students registered for any course for a particular year (highly unlikely with this data, I think, but something to keep in mind if you try this approach with other data). by the way, you do not need to connect to the server and database for every query. You can connect once at the beginning of the script and use the same connection throughout. -
In my post to provide the answer, I neglected to say: "This does NOT look like a good design." However, without knowing why it was designed that way, or if, in fact, this was just an example pulled from the air, to explain the question, I did not condemn it immediately. @eugene2009 If this is the design of your database, you may need to re-think it. If you'd like us to review the design and provide insight, you may want to start a new topic. If you are working on an admin-type page to let you review databases, tables, and data (as I have done on occasion), you might want to look at the SHOW and DESCRIBE commands of mySql. They can provide much information about the database and it's contents. Good Luck!
-
[SOLVED] Quering Multiple Databases for a single field.
DavidAM replied to Errant_Shadow's topic in MySQL Help
Ignore the fact that the tables are in separate databases for a moment, and design a query to get the data from the separate tables, then you can add the database qualifications. The first query you suggest: SELECT * FROM `red`.`subscriptions`, `green`.`subscriptions`, `blue`.`subscriptions` WHERE `account_id` = "$num" is not going to work, because "account_id" (in the WHERE clause) is ambiguous; that is, it exists in more than one table and has not been qualified to indicate which table should be used. The second WHERE clause is closer: SELECT * FROM `red`.`subscriptions`, `green`.`subscriptions`, `blue`.`subscriptions` WHERE `red`.`subscriptions`.`account_id` = "$num" OR `green`.`subscriptions`.`account_id` = "$num" OR `blue`.`subscriptions`.`account_id` = "$num" since you have now qualified the table names, but using OR without JOINing the tables will result in a cartesian product; that is, the specified row in the first table matched to EVERY row in the second table matched to EVERY row in the third table. And then the specifed row in the second table matched to EVERY row in the first table matched to EVERY row in the third table. And then, the same for the third table. I ran this query on a table with 6 rows and received 91 rows in the resultset! Using AND would be much closer to the desired result: SELECT * FROM `red`.`subscriptions`, `green`.`subscriptions`, `blue`.`subscriptions` WHERE `red`.`subscriptions`.`account_id` = "$num" AND `green`.`subscriptions`.`account_id` = "$num" AND `blue`.`subscriptions`.`account_id` = "$num" assuming that there is an entry in each table with the specifed account_id. But if any one table does not contain the specified value, you get nothing. An outer JOIN could help here, but will get complicated with more than two tables. Also, using a single SELECT, will create a wide row with data from ALL THREE tables across, not a list. I think the best approach would be to use a UNION: SELECT 'Red' AS Source, R.* FROM red.subscriptions AS R WHERE R.account_id = "$num" UNION SELECT 'Green', G.* FROM green.subscriptions AS G WHERE G.account_id = "$num" UNION SELECT 'Blue', B.* FROM `blue`.`subscriptions` AS B WHERE B.account_id = "$num" ORDER BY 1 Which will return one row from each table if it is present. I added the literals ('Red', 'Green', 'Blue') as "Source" to the SELECT so you can tell which database/table a particular row came from if need be. That could be anything, or nothing at all if you don't need it. Of course, for the union to work properly, the columns returned by each SELECT must be the same. The column names from the first select will be returned (you can see I did not use "AS Source" in the subsequent SELECTs). And these columns should be the same data type throughout. The ORDER BY 1 indicates that the first column in the returned result is to be used to sort the results. You can use column names from the first SELECT (i.e. ORDER BY Source) to accomplish this as well. Each query in the UNION should be sufficient to stand on its own. So if you need additional data from the "globals" database for each row, you can add a join in each of the SELECTs to accomplish this: SELECT 'Red' AS Source, R.*, M.MoreData FROM red.subscriptions AS R JOIN globals.subscriptions AS M ON R.account_id = G.account_id WHERE R.account_id = "$num" UNION SELECT 'Green', G.*, M.MoreData FROM green.subscriptions AS G JOIN globals.subscriptions AS M ON R.account_id = G.account_id WHERE G.account_id = "$num" UNION SELECT 'Blue', B.*, M.MoreData FROM `blue`.`subscriptions` AS B JOIN globals.subscriptions AS M ON R.account_id = G.account_id WHERE B.account_id = "$num" ORDER BY M.MoreData, Source I had to use "M" as the alias for the globals database/table since I already used "G" for green. -
[SOLVED] Quering Multiple Databases for a single field.
DavidAM replied to Errant_Shadow's topic in MySQL Help
To reference a table in a database you qualify the table name with the database name. SELECT ID, Name FROM database.tablename WHERE ID = 3 -
SHOW TABLES will return a list of all tables in the database. At least the tables that you have priviledges to see. It returns a resultset just like any other query.
-
That message indicates that the query failed. When mysql_query() fails, it returns false, which is not a valid resource for futher mysql functions. Try adding the following to edit1.php and see what you get: $result=mysql_query(" SELECT * FROM shop WHERE reference='$record'"); if (!$result) { echo mysql_error() . PHP_EOL; exit; } $num=mysql_num_rows($result);
-
You have short tags ("<?") around the queries but full tags ("<?php") around the other code. It may be that your server is configured not to allow short tags. In that case, they are being ignored by the PHP processor and sent to the browser. The browser will ignore them because they are an unknow HTML tag (since they start with "<"). Always use full tags ... <?php Also, turn on error reporting so you can see any errors you get. So the beginning of every php file should be: <?php error_reporting(E_ALL);
-
I'm glad you got it. And thanks for posting your solution. The next person searching the forums for a similar situation may be able to use your solution to learn something. Now, if you could mark the topic as solved, that would help others, too. There's a tab at the bottom of the page you can click to mark it. Only YOU can mark your topic as solved. Good luck.
-
@Neil I do not see how your query is more efficient than the one I submitted. In fact, it looks less efficient. Without the limitations on the latitude and longitude, this query will have to calculate the distance for every auction in the table. SELECT a.description, a.zipcode, z.city, z.state, SQRT(POW(69.1 * (z.latitude - ".$latitude."), 2) + POW(69.1 * (".$longitude." - z.longitude) * COS(latitude / 57.3) , 2)) AS distance FROM jos_bid_auctions a INNER JOIN zipcodes z ON (a.zipcode=z.zipcode) HAVING distance < ".$distance." ORDER BY distance ASC The query I submitted, on the other hand, can take advantage of indexes on both tables to reduce the number of rows to be processed. If Latitude and/or Longitude is indexed on the zipcode table, then that will limit the number of zipcodes to be checked. Once a zipcode is found that fits the limits, an index on zipcode in the aution table can be used to retrieve the record. SELECT A.description, A.zipcode, Z.city, Z.state FROM jos_bid_auctions A JOIN zipcodes Z ON A.zipcode = Z.zipcode WHERE A.description like '%auction%' AND Z.latitude BETWEEN 36.286982858078 AND 35.997537141922 AND Z.longitude BETWEEN -95.699526863467 AND -96.058611136533 ORDER BY Z.state, Z.city, Z.zipcode$ Granted, having the distance calculation in the query could be handy. But that could be done in PHP against the resultset. I'm not sure which process there might be more efficient, I'm not sure it would make much difference. And I will admit I do not know the internals of mySql; but I do know that a table scan looses to an indexed read in almost every case. Also, I have never used a float for an index, but I don't see how it could cause significant problems. In fact, I might personally be inclinded to make the lat and long DECIMAL columns with 5 or 6 digits on the right. @johnathan Use BETWEEN instead of <= AND >=. I think the server can make better use of the indexes since it will know both limits in one clause. At any rate, it should not be less efficient. Just keep in mind that BETWEEN is inclusive (both limits also satisfy the condition).
-
How would I convert this into code using an if statement?
DavidAM replied to kaveman50's topic in PHP Coding Help
1) Sorry about the missing parenthesis, I really have to learn to count. 2) Since you did not state the varaible name for the exam score, I just picked up a spare one laying on my desk, it was called $ES. Now that you have posted some code, I see that yours is called $exam_score, so you need to change all those $ES to $exam_score. 3) Where is what .5? There's one in there for the B and one for the C. If you are asking about the calculation for the F, you don't really need it because if it is not an A, B, C, or D then it has to be an F; right? 4) Your original post simply asked how to make the IF statement for the different grade rules. So, that's what I wrote. You said you already had some code so I just put 'echo' in there where the grade would be. I assumed you would change the 'echo' to whatever it needed to be to fit with the rest of your code (which we could not see). -
[SOLVED] Requires your assistance desperately!
DavidAM replied to Modernvox's topic in PHP Coding Help
Did you read the error message? -
<td align="left" class="main"><?php echo '<a href="javascript:popupWindow('' . (HTTP_SERVER . DIR_WS_CATALOG . FILENAME_ORDERS_PRINTABLE) . '?' . (tep_get_all_get_params(array('order_id')) . 'order_id=' . $HTTP_GET_VARS['order_id']) . '')">' . tep_template_image_button('button_printorder.gif', IMAGE_BUTTON_PRINT_ORDER) . '</a>'; ?></td> Immediately after the "popupWindow(" there is a single quote, which closes the literal being echo'd. This is immediately followed by another single quote which would START a new literal except there is no operator to combine the strings. I suspect you are trying to put a literal single-quote in the string, if so, you have to escape it (also escape the one at the end of the parameter list): <td align="left" class="main"><?php echo '<a href="javascript:popupWindow(\'' . (HTTP_SERVER . DIR_WS_CATALOG . FILENAME_ORDERS_PRINTABLE) . '?' . (tep_get_all_get_params(array('order_id')) . 'order_id=' . $HTTP_GET_VARS['order_id']) . '\')">' . tep_template_image_button('button_printorder.gif', IMAGE_BUTTON_PRINT_ORDER) . '</a>'; ?></td> [ code ] tages are a marvelous thing. See how the red shows literals and the blue shows variables (and green shows functions)?
-
How would I convert this into code using an if statement?
DavidAM replied to kaveman50's topic in PHP Coding Help
if ($ES >= ($mean + (1.5 * $total)) { echo 'A'; } elseif ($ES >= ($mean + (.5 * $total)) { echo 'B'; } elseif ($ES >= ($mean - (.5 * $total)) { echo 'C'; } elseif ($ES >= ($mean - (1.5 * $total)) { echo 'D'; } else { echo 'F (what ever happened to "E")'; } -
I don't know what to say. Usually, my psychic powers of fixing code I can't see to remove errors I haven't been told about are perfect. Did you read what I said in my last post?
-
//I need to concatenate each topic with each sentence foreach($dataArray->topic as $eachTopic) { foreach(dataArray2->sent as $eachSent) { $resultArray[] = $eachTopic." ".$eachSent; } } echo $result; 1) $dataArray is an array not an object. The loop should be foreach($dataArray['topic'] as $eachTopic) 2) $dataArray2 should be handled in the same manner 3) 'echo $result' makes no sense because nothing was ever assigned to it. The sentences are in $resultArray while will have to be echo'd in another for loop
-
//Insert Into pwUser Table $sql="INSERT INTO pwUsers (userName, password, email, acctType, activationKey, active, createDate, loggedIn, lastLoggedIn, firstLogin) VALUES ('$userName','$md5pwd','$email',23,'$activationKey',0,CURDATE(), 0, CURDATE(),0)"; $result1 = mysql_query($sql) or die ("Error in query: $sql. " . mysql_error()); $aID=mysql_insert_id(); // **** HERE YOU ARE EXECUTING THE QUERY A SECOND TIME // **** I THINK YOU WANT TO TEST $result1 if (!mysql_query($sql)) { die('Error: ' . mysql_error()); }
-
If you insist on using two separate queries, your logic should work with a couple of minor changes: while($row_topics = mysql_fetch_array($topics)) { $topicid = $row_topics['topicid']; $topic = $row_topics['topic']; //echo $topicid.'<br \>'; //echo $topic.'<br \>'; } you are assigning each row to the same variables, so in the end, the variables, $topicid and $topic, will contain only the last row from the database. You can turn those into arrays by adding the square-brackets to them in the assignment: while($row_topics = mysql_fetch_array($topics)) { $topicid[] = $row_topics['topicid']; $topic[] = $row_topics['topic']; } you will need to do the same with the sentence loop later.
-
You are specifically excluding the search zip code with these two lines: AND (latitude != $lat1 AND longitude != $lon1) since $lat1 and $lon1 are the values you retrieved from the database for the search zipcode, you are telling the query NOT to return that record. I'm not sure why it would be including 74133. Check the latitude and longitude for that record, make sure there is only one record for that zip, echo the sql and look to see what is causing it to be included.
-
Those two queries are NOT the same. The first one: is not even a valid query. The start of the WHERE clause is missing. Even with the WHERE clause (from your previous post) the query is not correct: $query = "SELECT description, zipcode, zip FROM jos_bid_auctions, zip_code WHERE jos_bid_auctions.description like '%$description%' AND zip_codes.latitude BETWEEN $latN AND $latS AND zip_codes.longitude BETWEEN $lonE AND $lonW AND zip_codes.latitude != $lat1 AND zip_codes.longitude != $lon1 ORDER BY jos_bid_auctions.zipcode"; There is no JOIN and nothing to relate the two tables, so you will get a cartesean product. The query you echoed from "my" code SELECT A.description, A.zipcode, Z.city, Z.state FROM jos_bid_auctions A JOIN zipcodes Z ON A.zipcode = Z.zipcode WHERE A.description like '%auction%' AND Z.latitude BETWEEN 36.286982858078 AND 35.997537141922 AND Z.longitude BETWEEN -95.699526863467 AND -96.058611136533 AND Z.latitude != +36.142260 AND Z.longitude != -095.879069 ORDER BY Z.state, Z.city, Z.zipcode$ looks like it will return the rows you indicated you were looking for. This is NOT the same as the first one you showed in your last post. What do you get when you execute this query?
-
retrieving images from mysql database using php
DavidAM replied to developr's topic in PHP Coding Help
First, use the code tags, which is not the typewriter, it's the # button. Yeah, I know, it's not intuitive. Took me a while to find it, but it is worth the effort. Since you used addslashes() on the data before you inserted it into the database, you are going to have to stripslashes() to remove them before you echo the data. I would recommend using mysql_real_escape() instead (you don't have to undo that when you retrieve the data). But if you already have data in the database, you can't switch without updating all existing data. As for the "Access denied" you are either using the wrong user, password or database; or you do not have access to the database. You'll have to talk to your webhost about that. -
If all you want is the total, and you are not going to do anything with the actual balances, let the database do the work: SELECT SUM(bal) AS TotBal FROM assets WHERE term = 'C'