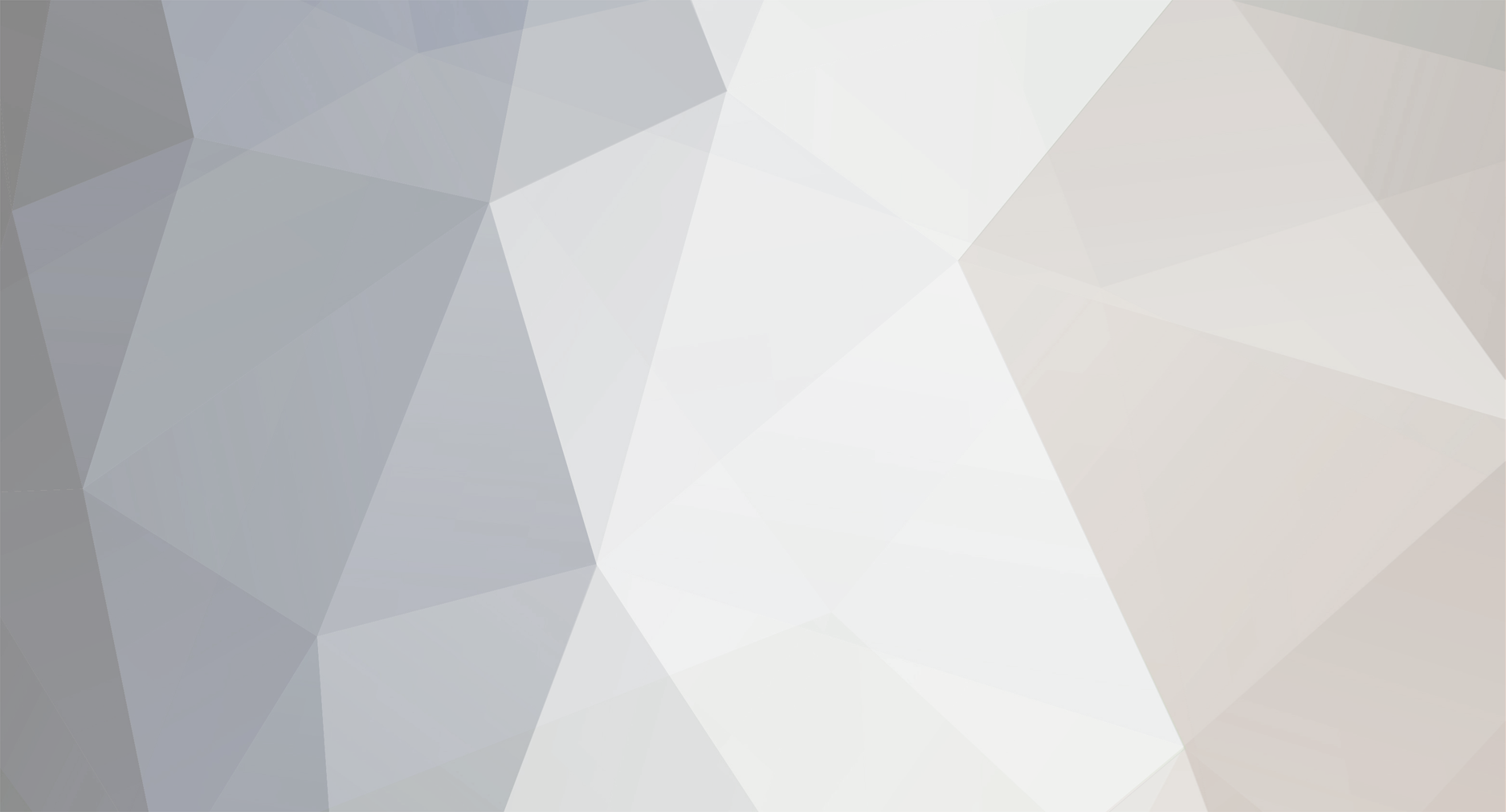
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
The SELECT SUM will work if you want to make another trip to the database. However why not just count them while you are outputting them to the table? $open_count = 0 while ($row = mysql_fetch_assoc($result)) { if ($row['case_open'] == 'yes') $open_count++; }
-
Your $insert = array ... statement does not look correct. I'm guessing you cut some code out or re-typed it. Usually when I do something like this, I put the product_id (or whatever) as the key to the array in the form field, this way everything related to that product has the same array key. for example (this is not real code, just off the top of my head) <input type="text" name="color[1]" value="Black"><input type="text" name="qty[1]" value = 1> <input type="text" name="color[2]" value="Blue"><input type="text" name="qty[2]" value = 3> <input type="text" name="color[3]" value="Cyan"><input type="text" name="qty[3]" value = 1> This way I don't need a hidden field for the product_id and if there are check boxes, the returned array is indexed by the product_id since the ones that are not checked (were not selected) are not sent to the action script. The indexes do not have to be numeric, they just have to be valid for an array key; however, if they have certain special characters they may get mangled (urlencoded?) when POSTed. I do it this way because I do not trust browsers. If for some weird reason it sent the fields out of sequence, the numerically assigned array keys could get mixed up. Using the product ID insures that the fields related by product have the same unique index. Call me paranoid, but hey, as long as M$ is has a browser out there, I'm going to protect myself from their idiocy. Besides, checkboxes are not sent unless checked and the numeric array would not tie to the other fields for that same line.
-
Yeah, you have to watch out for reserved words and ambiguous names. Always try to use different names for database, tables, columns, etc. On the other thing, it sounds like you are retrieving the full name from the database, and only part of it is showing up in the link. In the example you gave "myaddress.php?name=Bob" instead of "myaddress.php?name=Bob+Saget", it looks like you need to encode the name: $name="Bob Saget"; $link = "myaddress.php?name=" . urlencode($name); that should put the '+' in there for you. Also, in myaddress.php you will (probably) have to decode that: $name = urldecode($_GET['name']); If this is not the problem, or doesn't solve it, post some code so we can see what is happening.
-
Left Join Extremely slow - Any way to remedy? (16,000 lines)
DavidAM replied to JChilds's topic in MySQL Help
Almost definitely. Since your where clause is limiting on trans_id, an index here will help find the sales records quicker. Once the sales records are found, we need a quick way to find the items records. That means an index is needed on items.barcode (unique or not). The index on sales.barcode may or may not help with this query. Do you rebuild (or refresh, or whatever its called on your server) your indexes periodically? Considering the size of the items table and how long you have been collecting data, the table (and indexes) may be fragmented. Refreshing them will help make searches quicker. Some servers might just ignore the index if it is too badly fragmented and do a table scan anyway. Of course, I'm speaking from experience that is years old, but the concepts apply. Indexes are (usually) faster to search than a table scan. Fresh indexes are in order and can be searched even faster. Also, if all of the data (from a particular table) that is needed by a query is contained in a single index on that table, the server may scan only that index and never look at the actual data blocks. This is one reason to avoid using SELECT *. For instance, if you have a single index on sales.trans_id, sales.barcode, and an index on items.barcode, and all you were returning is the barcode, this query could run totally against the indexes. Since there are fewer columns in the index, there is more "data" per block so fewer disk reads are required. The values are the same as in the data segment, so there's no sense in looking there. Again, I'm speaking from years-old knowledge of the internals of a particular database server (almost definitely NOT the one you are using) and your server may behave differently. But the logic makes sense. Give the server as much opportunity to find the data as quickly as it can and it will be your friend. Most database servers provide a way to view the plan that is generated for a query. That should show you what indexes are being used, intermediate sorts, etc. Sometimes the plan is different for SELECT *, don't know why, except to say that once the server sees the *, it KNOWS it will have to read the data, so if some index is big enough it may decide a table scan would be faster anyway. Also, the plan may change as the table size changes (and indexes fragment). Also, and I don't mean to preach, SELECT * means more data read, more data extracted, more data sent to the client ... it chews up resources like mad (database-server cpu, database-server memory, bandwidth, client memory, client cpu). -
No, you don't have to SELECT before you UPDATE. I don't think using the same name for the database and table is a problem. If it is a reserved word, you'll have to put it in backticks; i.e. UPDATE `database` SET $time ... The error message indicates that your UPDATE statement has the word "database" just before the SET phrase. Is the code you showed exactly what you are running or did you try to simplify it? Try changing the update to: $sql = "UPDATE my_db SET '$time' = '$name' WHERE '$time' = 'OPEN' LIMIT 1"; echo $sql . "\n"; if (! mysql_query($sql)) echo mysql_error(); That will output the sql statement before sending it to the server. Post the result and let's see what we're telling the server to do. You might also cut-and-paste this part of the code exactly as is without the connection specifics (login, password, etc). I think we are loosing something in the translation.
-
Can you define "24 hours later since the 1st submit" ? Is it the first (or do you mean last) time submitted from this computer-user, or submitted with the exact same data, or submitted by the user logged into your site (I don't see a user login but maybe it's there). Give more detail on what you are trying to restrict and we might be able to give you some suggestions.
-
at the top of your script add error_reporting(E_ALL); ini_set("display_errors", 1); This should cause all PHP errors to be output. It will not output mySql errors so change the UPDATE code to: if (! mysql_query("UPDATE my_db SET '$time' = '$name' WHERE '$time' = 'OPEN' LIMIT 1")) echo mysql_error(); post any errors that are displayed so we can see what is happening.
-
[SOLVED] Perform advanced searches in SELECT statement
DavidAM replied to ktsirig's topic in MySQL Help
WHERE ID BETWEEN 56 AND 67 However, BETWEEN is inclusive: i.e. 56 <= ID <= 67. So if they are integers your example would be WHERE ID BETWEEN 55 AND 66 or you could do it the long way WHERE (ID > 56 AND ID < 67) -
kevin has a good point. Or are the "16 Slots" time slots and you are passing the column name in the $time variable. The second point I see is UPDATE my_db SET ... is my_db actually the name of the table you are trying to update?
-
<?php include 'db.php'; $sql = "SELECT * FROM pirerp WHERE status = '2'"; $result = mysql_query ($sql); $cargo = 0; while ($row = mysql_fetch_array($result)) { $cargo += $row['cargo']; } echo $cargo; ?>
-
Left Join Extremely slow - Any way to remedy? (16,000 lines)
DavidAM replied to JChilds's topic in MySQL Help
[*]Create an index on sales.Trans_ID [*]Create an index on sales.barcode [*]Create an index on items.barcode [*]Avoid using SELECT * unless you absolutely need every column in the table; list the columns individually Are the sales.barcode and items.barcode columns defined using (exactly) the same datatype? What are the row counts on these two tables? Is the LEFT JOIN necessary? Is it possible to have a sale without an item? If not, remove LEFT and use a straight JOIN. Post the table descriptions and row counts and let's see what we can see -
You can't do that. The user does not get the page until PHP is through with it. However, you can use an HTML refresh command in the HEAD section: <meta http-equiv="refresh" content="2;url=index.php" /> the 2 in the line above is 2 seconds. So for 3 minutes it would be 180
-
The function you are looking for is "glob". It will return an array of filenames that match a specified pattern. http://us3.php.net/manual/en/function.glob.php
-
Maybe this has already been suggested but, what about adding another link at the top of the page. You currently have why not add "Show MY 23 UNSOLVED Topics"? Maybe make it red or bold or standout in some other way. This might remind people that they have topics that need to be marked Solved. Also, what about an automatic email, maybe once a week, saying "Hey, you still have 23 unsolved topics ..."? Maybe it should only include topics that received a new post during the week since, I guess, not ALL problems get solved. Hmm, what about posts that aren't actually questions but are just informational? I guess there would have to be a way to mark a New Topic as a question vs. information, that way we could determine which should be marked solved and which do not need to be "solved". Dang, why does every little change have to lead to more and more change?
-
Code isn't working, yet is practically copied out of a PHP book...
DavidAM replied to jackwh's topic in PHP Coding Help
OK, let's think about what we see here: We're using a loop to process all of the results from the query [quote]while ($row = mysqli_fetch_array($result)) // LINE 18[/quote] and the output you showed us (it would have helped if you showed all of it in the first post) It looks like the while loop runs once, so the error occurs on the second time through the loop. It would appear that your include include($_SERVER['DOCUMENT_ROOT'] . "/fetchtweetfromdb.php"); is changing one of the variables used in the conditional part of the loop, specifically $result. -
Add chmod after you rename the file ... $newfilename = $ImageDir . $lastpicid . $ext; rename($ImageName, $newfilename); chmod ($newfilename, 0755); note the leading zero on the permissions, this makes it an octal literal, if you use 755 (without leading zero) it will not be the correct permissions.
-
[SOLVED] Object help please - foreach not working.
DavidAM replied to spires's topic in PHP Coding Help
I would guess, that for this to work, the column names in the database would have to be the same as the attribute names in the object. Your example of something that works shows: $object->id = $record['photo_id']; -- 'photo_id' is the column name in the database but 'id' is the attribute name in the obect; so 'has_attribute('photo_id')' is going to fail. Disclaimer: I haven't upgraded to PHP5 yet, so I haven't worked with objects in that version. If there is something special about "attribute" that I don't know, then I could be wrong ... (I thought I was wrong once before, but I was mistaken). -
[SOLVED] combining two WHILE statements into one
DavidAM replied to dachshund's topic in PHP Coding Help
Your $userresult is just going to be the user that is logged in, not the user that left the comment. Why not have the comment query join to the users table and get all that information in a single result: $commentsql="SELECT * FROM comments JOIN users ON comments.userid = users.userid WHERE articleid ='$id' ORDER BY id DESC LIMIT 10"; then you have all of the information in a single while loop -
[SOLVED] Counting the number of bids made on a tender
DavidAM replied to debuitls's topic in PHP Coding Help
Your SQL is close, but if I understand your question correctly it should be something like this: SELECT proposal.proposalid, proposal.subject, proposal.budget, proposal.category, count(bid.proposalid) AS bids FROM proposal LEFT JOIN bid ON proposal.proposalid = bid.proposalid GROUP BY proposal.proposalid"); You might also have to include all of the non-aggregate columns in the GROUP BY: GROUP BY proposal.proposalid, proposal.subject, proposal.budget, proposal.category depending on which server and version you are using. This will get you a list by proposal ID (including subject, budget and category) with the total number of bids placed for that proposal. -
your assignment in the javascript is openning a php block, but you are in the middle of an echo in php. I think you need to just close the string, call the function, then open the string back up. function random_ad() { echo " <script language='JavaScript'> images = new Array(2); images[0] = '" . display_adsense() . "'; images[1] = '<img src=wp-content/themes/wp-symisun/images/ad_test_1.PNG></img>'; index = Math.floor(Math.random() * images.length); document.write(images[index]); </script>"; }
-
Output a repeated value only once in a PHP drop-down list
DavidAM replied to Fabrizzio PHP's topic in PHP Coding Help
I have to agree with Mark, you should normalize the database, especially if it will contain lots of data. Performance will (should) be better and updates simpler. That being said, to get the results you want with what you have try: //** Instead of SELECT * FROM subjects ORDER BY id ASC $list=mysql_query("SELECT DISTINCT borough FROM subjects ORDER BY borough ASC"); Of course you can't order by id now since you are not returning it and there would be multiple id's for a single borough, but I would think you want it ordered by borough for this list anyway. If you want to be really fancy, you could do something like this: SELECT borough, count(*) AS ucount FROM subjects GROUP BY borough Then you could show the number of universities in your drop down i.e. Westminster (2). Of course, you would have to strip off the count part in result.php. Note: you don't need an ORDER BY in this example because the server sorts it for the GROUP BY (might not need it for the first example either). -
[SOLVED] Retreving Large amounts of data from database
DavidAM replied to Peggy's topic in PHP Coding Help
Glad to help. I've never actually used that approach, but answering your question got me to thinking about how it might be useful. Just be sure that your column names are valid for variable names and that they don't collide with existing variables. If the value in $key is not a valid variable name, you should get an error message (I think). If it is the same as an existing variable name, it will just replace the contents of that variable (without warning) which might be just what you want. You might want to look at the extract function (http://us3.php.net/manual/en/function.extract.php). It does the same as the foreach example but allows you to add a prefix to the resulting variable names, prevent collisions, etc. -
$_SERVER['REQUEST_URI'] should give you that information without having to do rewrites, I think.
-
How to print a file's content so it won't erase empty lines
DavidAM replied to Shantar's topic in PHP Coding Help
How are you showing the file to the user? If you are retrieving a text file and displaying it in a web page, HTML is going to discard all "extra" whitespace (spaces, carriage-returns, new-lines, tabs, etc) and replace them with a single space. If this is the way you are presenting it, put it inside of <PRE> tags (that tells HTML that the text is "pre-formatted" and it should not be modified). echo "<PRE>" . $output . "</PRE>"; -
[SOLVED] Retreving Large amounts of data from database
DavidAM replied to Peggy's topic in PHP Coding Help
In your code, $row is the array the data is written to. I don't think there is a Super Global (like $_POST) that the data goes to. However, you can use your $row just like you did $_POST: foreach ($row as $key => $value) { /* even use "variable-variables to do the assignments (as long as the column names are all valid variable names */ $$key = $value; } Notice the two dollar-signs on key ($$key = $value). That will use the value in $key as the variable name. So it's the same as the last three lines of code in your example: $applicant_telephone = $row['applicant_telephone']; $applicant_email = $row['applicant_email']; $applicant_id = $row['applicant_id'];