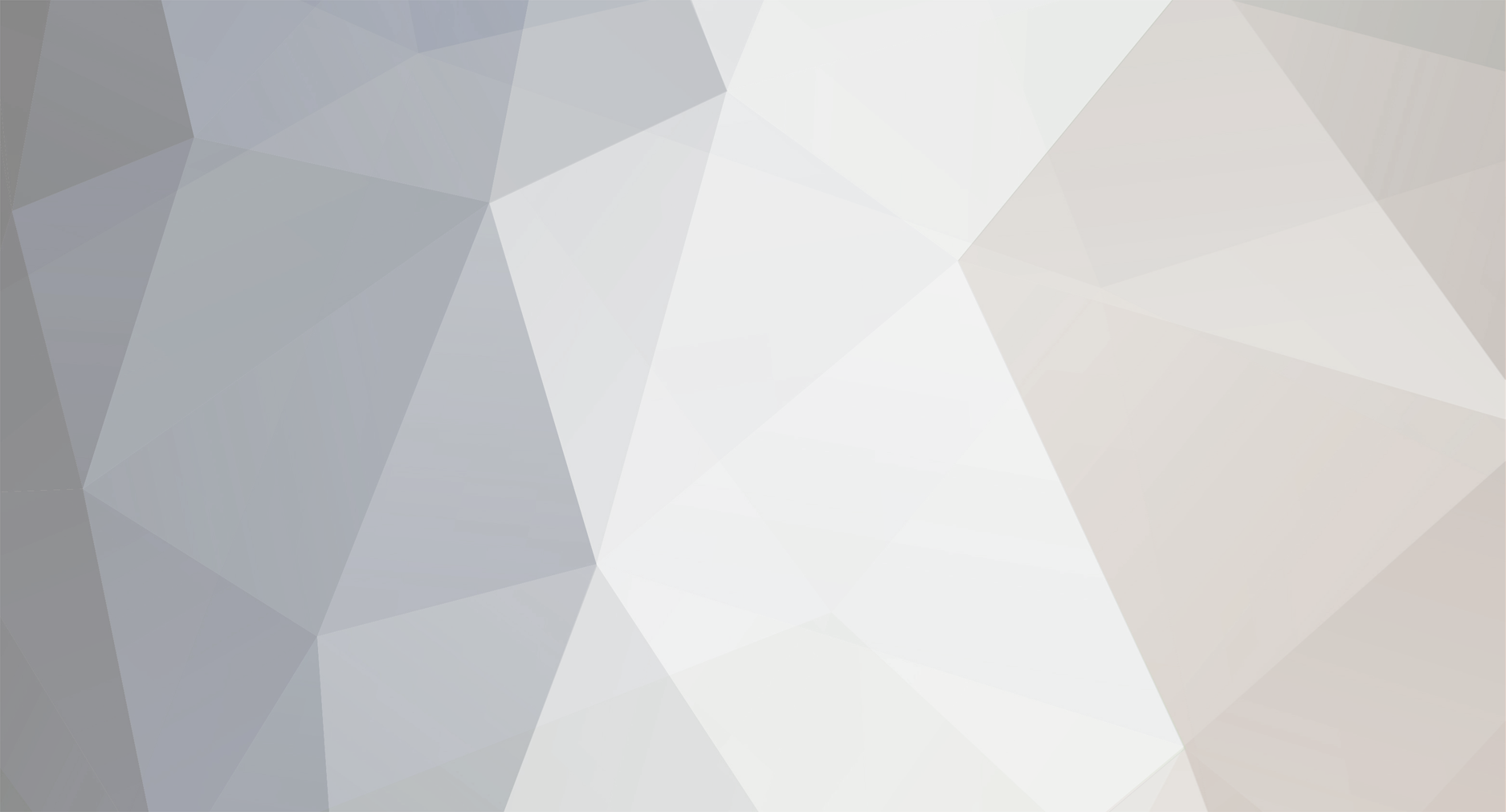
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
Are you saying that you want to create a table with 1.6 million columns? MySQL column limit explained.
-
OK, here is where we are. First I would like to say, there has to be a better way, but it is way to late for me to think clearly. This works, but I'm sure someone could clean it up abit. Secondly, you MUST have an end date in the database, or the query will not return THAT row. <?php include 'config.php'; $sql = "SELECT eventName, DATE_FORMAT(startDate,'%m/%d/%Y') AS eventStart, DATE_FORMAT(endDate,'%m/%d/%Y') AS eventEnd, DATE_FORMAT(CURDATE(),'%m/%d/%Y') AS today, DATE_FORMAT(DATE_ADD(CURDATE(), INTERVAL 7 DAY),'%m/%d/%Y') AS endWeek FROM events WHERE "; $clause = array(); for( $i = 0; $i < 7; $i++ ) { $clause[] = "DATE_ADD(CURDATE(), INTERVAL $i DAY) BETWEEN DATE(startDate) AND DATE(endDate)"; } $sql .= implode(' OR ', $clause); $sql .= ' ORDER BY startDate'; $result = mysql_query($sql) or trigger_error($sql . ' has falted. <br />' . mysql_error()); //pull data from database. $dates = array(); //define dates array. for($i = 0; $i < 7; $i++) { $dates[] = date('m/d/Y', strtotime("+$i days")); //fill dates array with every date from now until 7 days from now. } foreach($dates as $days) { //go through dates array. $data[$days] = NULL; //fill data array with keys for every date from now until 7 days. } if(mysql_num_rows($result) > 0) { while($r = mysql_fetch_assoc($result)) { //while data exists in the database result resource. if(strtotime($r['eventStart']) < strtotime($r['today'])) { //if the event started before today. $r['eventStart'] = $r['today']; //make it's startdate today. } if(strtotime($r['eventEnd']) > strtotime($r['endWeek'])) { //if the event ends after this week is over. $r['eventEnd'] = $r['endWeek']; //make the end of the week, it's end. } //echo '<pre>' . print_r($r,true) . '</pre>'; //de-bugging. $started = false; //define a false variable. foreach($data as $key => $value) { //loop through our data array. if($key == $r['eventStart'] && $started == false) { //if the event starts at the present key, put it in the array. $data[$key][] = $r['eventName']; $started = ($key == $r['eventEnd']) ? false : true; //set started to true. } elseif($key == $r['eventEnd']) { //if the eventEnd is the present key, put it in the array, $data[$key][] = $r['eventName']; $started = false; //set started to false; } elseif($started == true) { //if started is true, then put the event in the array. $data[$key][] = $r['eventName']; } } } foreach($data as $date => $v) { //loop through the data array. echo $date . '<br />---------<br />'; //echo the $date, followed by a line. foreach($v as $event) { echo $event . '<br />'; //each event is then echo'd to the page, followed by a break rule. } echo '<br />'; //after all of the events on this day, print another break rule, double spacing before the next date. } } else { echo 'No rows to show!'; } ?> edit: fixed code, please re-run.
-
Pik's code added to mine: <?php $sql = "SELECT eventName, DATE_FORMAT(startDate,'%m/%d/%Y %H:%i:%s') AS eventStart, DATE_FORMAT(endDate,'%m/%d/%Y %H:%i:%s') AS eventEnd FROM events WHERE"; $clause = array(); for( $i = 0; $i < 7; $i++ ) { $clause[] = "DATE_ADD(CURDATE(), INTERVAL $i DAY) BETWEEN DATE(startDate) AND DATE(endDate)"; } $sql .= implode(' OR ', $clause); $sql .= ' ORDER BY startDate'; $result = mysql_query($sql) or trigger_error($sql . ' has falted. <br />' . mysql_error()); if(mysql_num_rows($result) > 0) { while($r = mysql_fetch_assoc($result)) { echo $r['eventStart'] . ' until ' . $r['eventEnd'] . ' => ' . $r['eventName'] . "<br />\n"; } } else { echo 'No rows to show!'; } ?>
-
Welp, I just plugged the startDate in, and didn't even put anything doing with the endDate. So, it would only pull dates that Started in the next week. Your query is probably more what the OP is wanting, as you tend to think much to much for me... But, in reality, I understand what your query does, and yes, it would fit this problem better.
-
That is correct. Try this: <?php $sql = "SELECT eventName, DATE_FORMAT(startDate,'%m/%d/%Y %H:%i:%s') AS eventStart FROM events WHERE DATE(startDate) BETWEEN CURDATE() AND DATE_ADD(CURDATE(), INTERVAL 7 DAY) ORDER BY startDate"; $result = mysql_query($sql) or trigger_error($sql . ' has falted. <br />' . mysql_error()); if(mysql_num_rows($result) > 0) { while($r = mysql_fetch_assoc($result)) { echo $r['eventStart'] . '=> ' . $r['eventName'] . "<br />\n"; } } else { echo 'No rows to show!'; } ?> Post back with the results please!
-
Why am i receiving blank emails from my contact form?
jcbones replied to queketth's topic in PHP Coding Help
Replace: $email = (isset($_POST['Email'])) ? strip_tags($_POST['Email']) : NULL; //same as above. With: $email = (isset($_POST['Email']) && preg_match('~^([a-zA-Z0-9_\-\.]+)@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.)|(([a-zA-Z0-9\-]+\.)+))([a-zA-Z]{2,4}|[0-9]{1,3})(\]?)$~',$_POST['Email'])) ? $_POST['Email'] : NULL; Test that pattern, as I got it off of the RegEx Library. -
You're welcome!
-
Or just view the output in the HTML source code (i.e. right-click View Page Source). That seems to soar over the heads of newbies!!!
-
You should have a form that gets the info, then passes it to a script that collects the data, and does calculations. After that, put out a confirmation page, clicking 'yes' goes to paypal, clicking 'no' clears the order.
-
include 'db.config.php'; $sql = "SELECT u.username FROM follow AS f JOIN users AS u ON f.follower = u.userid WHERE f.followid = '$profileuserid'"; $result = mysql_query($sql) or trigger_error($sql . ' has an error.<br />' . mysql_error()); if(mysql_num_rows($result) > 0) { while($row = mysql_fetch_row($result)) { echo $row[0] . '<br />'; } } else { echo 'No followers'; } UN-Tested.
-
Why am i receiving blank emails from my contact form?
jcbones replied to queketth's topic in PHP Coding Help
I would suggest something along the lines of: <?php if(isset($_POST)) { $name = (isset($_POST['Name'])) ? strip_tags($_POST['Name']) : NULL; //if name is set, strip html tags, and return it, otherwise set the string as NULL. $email = (isset($_POST['Email'])) ? strip_tags($_POST['Email']) : NULL; //same as above. $telephone = (isset($_POST['Telephone'])) ? preg_replace('~[^0-9\-]~','',$_POST['Telephone']) : NULL; //if telephone is set, remove any characters that are not a number, or a dash, othewise set as NULL. $type = (isset($_POST['Type'])) ? strip_tags($_POST['Type']) : NULL; //strip tags. $message = (isset($_POST['Message'])) ? strip_tags($_POST['Message']) : NULL; strip tags. if(empty($name) || empty($email) || empty($message)) { //name, email, and message are required fields, if they are empty, tell the user to go back and fill them in. echo 'Please go back and fill in all required lines!'; } else { //if the fields are NOT empty, proceed with the mailing. $formcontent=" From: $name \n Type: $type \n Message: $message \n Telephone: $telephone"; $recipient = "enquiries@hqandco.com"; $subject = "Contact Form"; $mailheader = "From: $email \r\n"; if(mail($recipient, $subject, $formcontent, $mailheader)) { //if mail is sent to the SMTP server successfully, echo 'thank you'. echo "Thank You!"; } else { //otherwise, tell the user it did not go through. echo 'There was an error completing your request! Please try again!'; } } ?> -
When you use pring_r() or var_dump(), inclose them inside <pre> tags, to get the desired output. echo '<pre>' . print_r($var,true) . '</pre>';
-
Most likely you are missing a curly brace ({ OR }) somewhere.
-
Here is a listing of all the PayPal variables. You could send a lot through the 'custom' variable, but you will have to keep it less than 255 chars.
-
Well, how do you propose removing one's they *think* they need, vs ones the *know* they want?
-
You wouldn't put the escape into the string when typing it in, would you? So the string should be: $string = "Is your name 0'reilly?";
-
If you would have followed my advice, you would have removed the equal (=) sign from your query.
-
It will delete every file in your directory that you give it.
-
Your query has no 'ids' in it. That is the problem. You need to make sure you are getting them. echo '<pre>'; print_r($_POST['check']); echo '</pre>';
-
uploading a small file but errors returned
jcbones replied to freelance84's topic in PHP Coding Help
move_uploaded_file() will not create the folder for you, you must create the folder, and then give it the proper permissions. -
Pik is saying that the delete query should be: $q = "DELETE FROM pictures WHERE pic_id IN($ids)"; If you want to delete the image file, then use unlink().
-
Is $selectList the resource, or an array derived from another call to the resource? ala. mysql_fetch_assoc...
-
Try this one: <form name="input" action="" method="post"> Make: <Select name="make"> <option "Input" value="<?php echo $_POST['make']; ?>"><?php echo $_POST['make']; ?></option> <option value="All Makes">All Makes</option> <option value="Ford">ford</option> <option value="BMW">BMW</option> <option value="Honda">Honda</option> <option value="Lexus">Lexus</option> </select> Model: <Select name="model"> <option "Input" value="<?php echo $_POST['model']; ?>"><?php echo $_POST['model']; ?></option> <option value="All Models">All Models </option> <option value="Civic">Civic</option> <option value="3 Series">3 Series</option> <option value="Fiesta">Fiesta</option> <option value="IS200">IS200</option> </select> Fuel: <Select name="fuel"> <option "Input" value="<?php echo $_POST['fuel']; ?>"><?php echo $_POST['fuel']; ?></option> <option value="Any">Any</option> <option value="Petrol">Petrol</option> <option value="Diesel">Diesel</option> </select> Location: <Select name="location"> <option "Input" value="<?php echo $_POST['location']; ?>"><?php echo $_POST['location']; ?></option> <option value="UK">UK</option> <option value="London">London</option> <option value="Kent">Kent</option> </select> <input type="submit" name="searchCars" value="Search Cars" /> </form> <?php if(!isset($_POST['searchCars']) || $_POST['searchCars'] != 'Search Cars') { exit(); } include 'db.inc.php'; //variables from selection form $cars['Make'] = mysql_real_escape_string($_POST['make']); $cars['Model'] = mysql_real_escape_string($_POST['model']); $cars['Fuel'] = mysql_real_escape_string($_POST['fuel']); $cars['Location'] = mysql_real_escape_string($_POST['location']); //make sure all $cars variables hold a value; //set it to an array if it does. foreach($cars as $key => $value) { if(!empty($value) && (substr($value,0,3) != 'All') && (substr($value,0,3) != 'Any')) { $query_string[] = "$key = '$value' "; } } //default query, with added parameters. $query = "SELECT * FROM `cars` "; if(is_array($query_string)) { $query .= 'WHERE ' . implode('AND ',$query_string); } /*paganation starts =================*/ $per_page = 5; $page_query = str_replace('*','COUNT(*)',$query); $page_result = mysql_query($page_query) or trigger_error(mysql_error()); $count = mysql_fetch_row($page_result); $row_count = $count[0]; //add echo before dollar pages below if you want to show count of rows or pages $pages = ceil($row_count / $per_page); $page = (isset($_GET['page'])) ? (int)$_GET['page'] : 1; $query .= ' LIMIT ' . (($page - 1) * $per_page) . ',' . $per_page; //echo $query;//query built from values above depending on selections $result = mysql_query($query); //check if results if(!$result){ echo "No Results <br />"; } else { echo "<table class='ex1' border='0' width='120%' style=text-align:center; cellpadding='6' cellspacing='0'> </tr>"; while($row = mysql_fetch_array($result)) { echo "<tr style=font-family:verdana;font-size:80%;>"; echo "<td width=13%>" . $row[""] . "<img src=\"" . $row["Photo"] . "\"></a>"; echo '<td width="14%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Make'] . '</a></td>'; echo '<td width="5%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Model'] . '</a></td>'; echo '<td width="4%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Fuel'] . '</a></td>'; echo '<td width="4%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Location'] . '</a></td>'; echo "</tr>"; } echo "</table>"; } //show number of pages at footer if ($pages >= 1 && $page <= $pages) { for ($x =1; $x<=$pages; $x++ ){ echo ($x == $page) ? '<strong><a href="?page='.$x.'">'.$x.'</a></strong> ' : '<a href="?page='.$x.'">'.$x.'</a> ' ; } } ?>