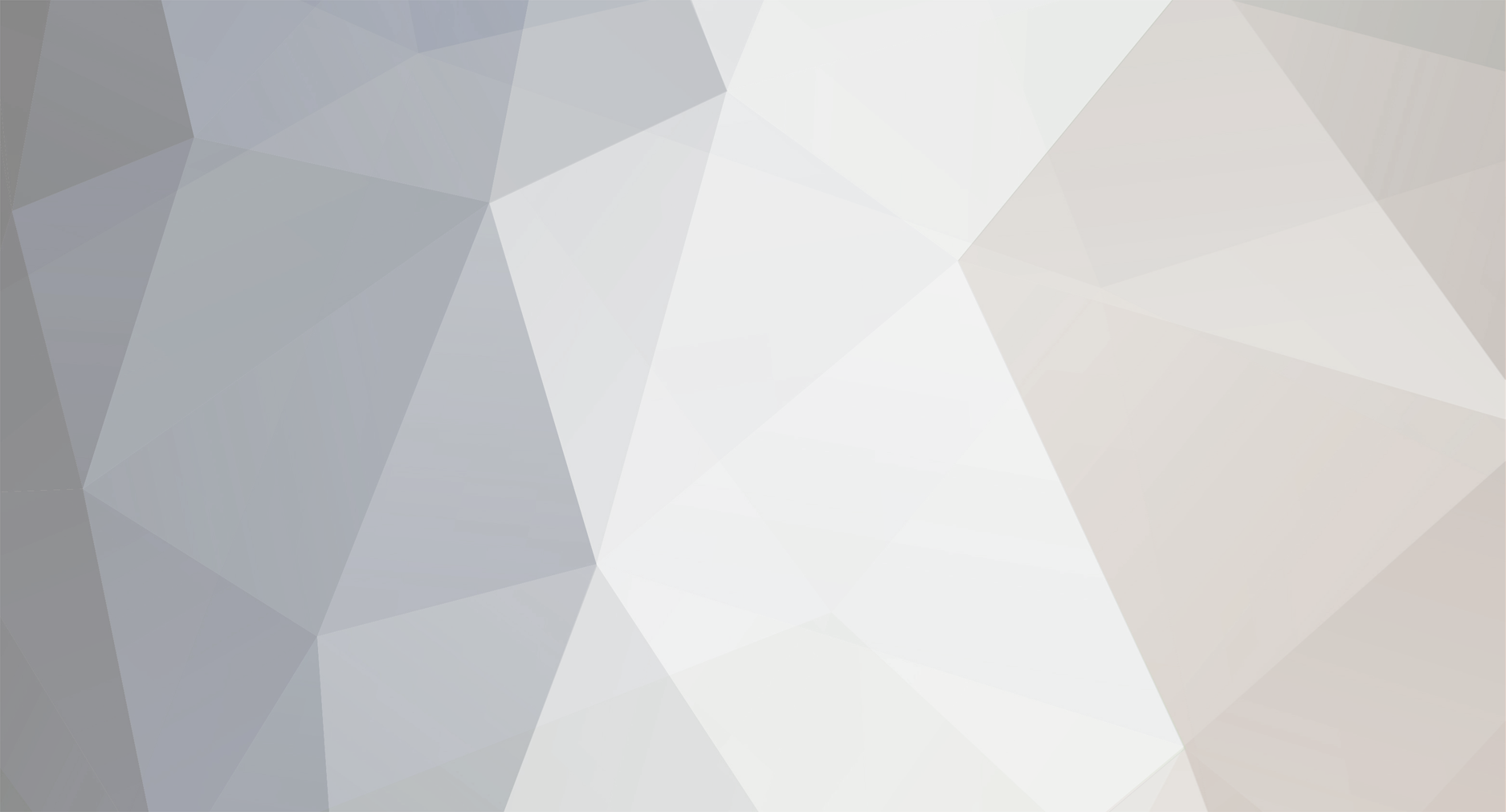
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
Remove the gallerybefore. and galleryafter. from your indexes. Your dump shows that they are NOT in your array index. "SELECT gallery_before.photo_id, gallery_before.photo_filename, gallery_before.photo_title, tbl_before_after.id, tbl_before_after.before, tbl_before_after.after, gallery_after.photo_id AS after_photo_id, gallery_after.photo_filename AS after_photo_filename FROM tbl_before_after INNER JOIN gallery_before ON tbl_before_after.before = gallery_before.photo_id INNER JOIN gallery_after ON tbl_before_after.after = gallery_after.photo_id" Now you should have a couple more indexes in that array.
-
Let me know if this doesn't work: It is Un-tested! <?php include 'db.inc.php'; //variables from selection form $cars['Make'] = mysql_real_escape_string($_POST['make']); $cars['Model'] = mysql_real_escape_string($_POST['model']); $cars['Fuel'] = mysql_real_escape_string($_POST['fuel']); $cars['Location'] = mysql_real_escape_string($_POST['location']); //make sure all $cars variables hold a value; //set it to an array if it does. foreach($cars as $key => $value) { if(!empty($value)) { $query_string[] = "$key = '$value' "; } } //default query, with added parameters. $query = "SELECT * FROM `cars` WHERE " . implode('AND ',$query_string); /*paganation starts =================*/ $per_page = 5; $page_query = str_replace('*','COUNT(*)',$query); $page_result = mysql_query($page_query) or trigger_error(mysql_error()); $count = mysql_fetch_row($page_result); $row_count = $count[0]; //add echo before dollar pages below if you want to show count of rows or pages $pages = ceil($row_count / $per_page); $page = (isset($_GET['page'])) ? (int)$_GET['page'] : 1; $query .= ' LIMIT ' . (($page - 1) * $per_page) . ',' . $per_page; //echo $query;//query built from values above depending on selections $result = mysql_query($query); //check if results if(!$result){ echo "No Results <br />"; } else { echo "<table class='ex1' border='0' width='120%' style=text-align:center; cellpadding='6' cellspacing='0'> </tr>"; while($row = mysql_fetch_array($result)) { echo "<tr style=font-family:verdana;font-size:80%;>"; echo "<td width=13%>" . $row[""] . "<img src=\"" . $row["Photo"] . "\"></a>"; echo '<td width="14%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Make'] . '</a></td>'; echo '<td width="5%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Model'] . '</a></td>'; echo '<td width="4%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Fuel'] . '</a></td>'; echo '<td width="4%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Location'] . '</a></td>'; echo "</tr>"; } echo "</table>"; } //show number of pages at footer if ($pages >= 1 && $page <= $pages) { for ($x =1; $x<=$pages; $x++ ){ echo ($x == $page) ? '<strong><a href="?page='.$x.'">'.$x.'</a></strong> ' : '<a href="?page='.$x.'">'.$x.'</a> ' ; } } mysql_close($con); ?>
-
What you need to do, is create a function: <?php $food = 500; $peasants = 50; $food_per_peasant = 10; $laborers = 10; $food_per_laborer = 10; function availableTraining($food, $required_persons, $food_per_persons) { return (($total = ($food / $food_per_persons)) > $required_persons) ? $required_persons : intval($total); } echo availableTraining($food, $peasants, $food_per_peasant) . ' laborers can be trained!<br /> OR, <br />' . availableTraining($food, $laborers, $food_per_laborer) . ' farmers can be trained!'; ?> Here you can see, that you can plug in the necessary variables to make the function work with different values. You could change food_per_laborer to 15, and it will still calculate it out correctly.
-
Join is a lot faster, with less overhead, and with less problems to crop up in the future.
-
Does your server have imageMagick installed? This would be much faster, with a lot less overhead than the GDLibrary.
-
Is there any reason you cannot join the two queries together? $sql = "SELECT name.ump_id, name.first_name, name.last_name FROM ump_names AS name JOIN ump_priority AS up ON name.ump_id = up.ump_id WHERE up.ump_id IN ( $values ) ORDER BY up.priority DESC ";
-
What is your results now? Before, you were only getting 1 result, so the order by was non existent.
-
ORDER BY doesn't reflect on the query going TO the database, it reflects on the results COMING from the database. BTW, remove the single quotes from around the $values, as you are passing them as integers, Those single quotes are saying that you want to pass ALL of those numbers as 1 string parameter.
-
I have no way of knowing how $userstats3['food'] or $userstats['peasants'] is defined in this script. Did you add that snippet to your code? If I was to run this snippet as/is, I would get some undefined errors, and a 'cannot divide by 0' error. Cast your strings to integers when you pull the data back from the database. $food = intval($userstats3[food]); $peasants = intval($userstats3[peasants]); Post your full script.
-
You can play with the numbers in my code snippet. It should calculate the max_trainable depending on the available food, or the cost_per_peasant.
-
If you are using a subquery inside of the IN() function, you can ONLY call ONE column, you are selecting it to return 2 and 3 columns in each. In each one of those subqueries, you should be only selecting the 'ump_id', since that is the column you are checking against.
-
Where does $max_train come from? <?php $food = 100; $peasants = 50; $food_per_peasant = 10; $max_train = (($total = ($food / $food_per_peasant)) > $peasants) ? $peasants : $total; echo $max_train; ?>
-
So something like: $date = date('Y-m-d H:i:s',strtotime('Wed Jun 01 09:37:13 +0000 2011')); I believe that strtotime() will parse that date, post back if it doesn't work.
-
1. Don't use $_REQUEST, instead use the appropriate $_POST, $_GET, or $_COOKIE. 2. Change the filename to a unique one on. Some just append a time() to the end of the filename (before the ext. of course). 3. You may want to add a WHERE clause to that sql query, unless you want every row in the database updated. 4. You should be checking for $_FILES['userfile']['error'], to see if there are any errors in the image upload. 5. You shouldn't rely on MAX_FILE_SIZE to limit the file size uploaded, that can be spoofed easily. Check that in your script also. 6. I would wrap an if() statement around the move_uploaded_file. Then echo either 'file stored' or 'file failed to store' based on the return of 'true' or 'false'. Think on these, then post back.
-
Whats the error in this Insert Statement...???
jcbones replied to genext.brite's topic in PHP Coding Help
Try this: <html> <body> <?php $con=mysql_connect("localhost","root",""); if(!$con) { die ('Can not connect to :' .mysql_error()); } mysql_select_db("brite",$con); $sql="insert into subjects VALUES('{$_POST['subname']}','{$_POST['submarks']}','{$_POST['subgrade']}','T')"; mysql_query($sql) or trigger_error( mysql_error() ); mysql_close(); header('Location:subject.php'); exit(); ?> You had an extra bracket (]) around one of your $_POST variables. -
Can't believe I missed it either.
-
Or, it's to late, and I didn't really look at it to good!!! :'(
-
Try: if (trim($avalue[0]) == trim($itemToAdd[0])) Or, even: if (strtolower(trim($avalue[0])) == strtolower(trim($itemToAdd[0])))
-
Some people will tell you that calling a class inside a class breaks encapsulation as well, although, I don't share that train of thought. The argument is that by calling that PDO class, you are tying a specific database type to the class, on the other hand, writing a query inside a class does the same thing. The only thing that I see is, you may want to move your database details to the variable definitions, that way you don't have to change anything in the function, to change database's (TBL_PREFIX as well).
-
Have you tried: if ($avalue[0] == $itemToAdd[0])
-
You can do that. Just make sure the heredoc statement is included after you have defined the variables. I know I can, just don't understand why HE can't.
-
Fortunately, there is a ton of features that Paypal offers, that would let you do everything you want to do, plus more. Un-fortunately, there is so much, it would take someone that can look at all of you code, and help you integrate it, in a one on one setting. You could look at Paypal Integration, or post this in the freelance section. If you make a go of it on your own, post back if you run into a problem. PS. I really hope that is not the total contents of those files.
-
Without seeing how the array is built in $_SESSION, we really can't help to much. However, if you provide a dump of the session array, it would greatly help us in helping you. echo '<pre>'; print_r($_SESSION); echo '</pre>';
-
The reason that we say 'Don't use global' is because you have now tied the function to a particular script, for a particular purpose. Take for instance. $num = .10; function discount($price) { global $num; return $price - ($price * $num); } We have now global included a fairly commonly used variable into a function. Why there is not a problem with it now, being that I defined the variable as .10 for a 10 percent discount. Pretty straight forward. I did this so that I would only have to change the discount in one place in the script. Why would there be anything wrong with that? Here is why. So, I am looking through my library one day for a discount function. I find this one, I'll just drop it into my script and call it. It only has one argument, $price. for($num = 0; $num > 10; $num++) { echo discount($prices[$num]); } While this may be a simple function, and one that you would instantly see the problem with, there are lots of times the problem will not be this evident. Now why would my included function start returning all these crazy numbers? I've just caused myself some troubleshooting that would have been prevented by listing $num in the arguments.
-
Now, to post all details that are NOT null, I would use something like: while ($row = mysql_fetch_assoc($query)) { //loop the query results echo '<table><tr><th colspan=2>Model#:' . $row['model'] . '</th></tr>'; //build a table, with a header. unset($row['model']); //destroy the model value, so we don't get it in the next loop. unset($row['id'],$row['prod_cat']); //instead of unsetting this value, I would just not call it from the database. foreach($row as $column => $value) { //loop the remaining results. if(!empty($value)) { //if the value is NOT empty, run the next line. echo "<tr><td>$column</td><td>$value</td></tr>"; //echo the column name, with the value in the table. } //end if. } //end foreach. echo '</table>'; //end the table. } //end while.