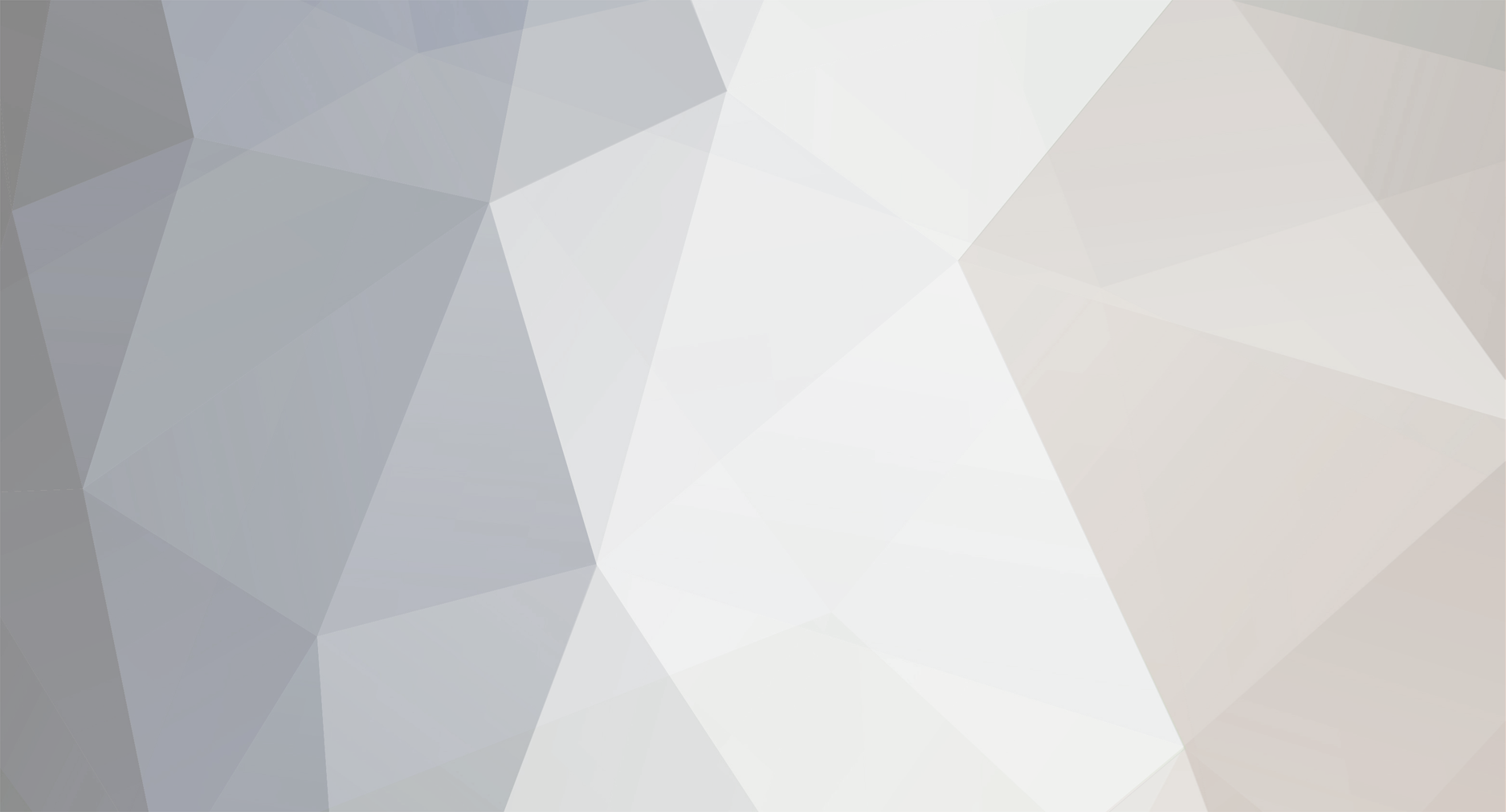
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
converting strings from foreach into a single array
QuickOldCar replied to tarquino's topic in PHP Coding Help
I did it for complete words and trying to find close matches within the multiple words. Hopefully this helps you closer to what you need. <?php if (isset($_GET['s']) && trim($_GET['s']) != '') { $search = strtolower(trim($_GET['s'])); } else { $search = ''; } ?> <form action="" method="get"> <input type="text" name="s" value="<?php echo $search; ?>"/> <input type="submit" value="GO" /> </form> <?php $cakes = array( "jade" => array( "Chocolate Cake" => array( "ingredients" => "Chocolate, eggs, flour" ), "Cookie Dough Cake" => array( "ingredients" => "Cookies, Double cream, flour, eggs" ) ), "jessica" => array( "Lemon Cheesecake" => array( "ingredients" => "Lemon, eggs, flour" ), "Belgian Chocolate Cake" => array( "ingredients" => "Dark belgian chocolate, eggs, flour" ) ), "stephanie" => NULL ); $string = ''; //keep blank $levenshtein = array(); $exploded = array(); //find each word from the array values foreach ($cakes as $val) { if (is_array($val)) { foreach ($val as $key => $val2) { if (is_array($val)) { //explode multiple words $exploded = explode(" ", strtolower(trim($key))); if (!empty($exploded)) { //loop each exploded word foreach ($exploded as $explode) { //get each words weight $lev_weight = levenshtein($search, $explode); //if any of the words within range, add complete words to array if ($lev_weight >= 0 && $lev_weight <= 3) { $levenshtein[$key] = $lev_weight; } } } } } } } //only show if not an empty array if (!empty($levenshtein)) { asort($levenshtein, SORT_NUMERIC); //show raw results echo "<pre>"; print_r($levenshtein); echo "</pre>"; //loop items from array foreach ($levenshtein as $word => $weight) { echo $word . "<br />"; echo $weight . "<br />"; } } ?> results for chocolate Array ( [belgian Chocolate Cake] => 0 [Chocolate Cake] => 0 ) Belgian Chocolate Cake 0 Chocolate Cake 0 -
The best place to start is php.net You can try simple basic things and see if you can do it, a combination of those can make a larger script or project and become something useful.
-
Some help needed about one variable in different links
QuickOldCar replied to 684425's topic in PHP Coding Help
I did this to be a little safer using parameter values in an include <?php //creates the links array $links = array( "link1", "link2", "link3" ); //loop as href links foreach ($links as $link) { echo "<a href='?x=$link'>$link</a> "; } //GET from address bar //only allow one that's in links array if (isset($_GET['x']) && in_array(trim($_GET['x']), $pages)) { $location = trim($_GET['x']); } else { $location = 'link1';//default location } //assign the files name to link switch ($location) { case "link1": $filename = "x"; break; case "link2": $filename = "y"; break; case "link3": $filename = "z"; break; } //so can see it work echo "<h2>" . $location . "</h2>"; //include a page $script = dirname(__FILE__) . DIRECTORY_SEPARATOR . $filename . ".php"; if (file_exists($script)) { include($script); } else { echo "$script does not exist."; } ?> -
Lockout user after failed log on attempts
QuickOldCar replied to NotionCommotion's topic in PHP Coding Help
Totally agree with Jacques, constantly looking through ip's in a db or long lists takes lots more resources as well. -
I guess this depends on how are actually doing it. $_SERVER['HTTP_REFERER'] can be spoofed You can do a check what the ip is though $remote_ip = $_SERVER['REMOTE_ADDR']; if (strstr($remote_ip, ', ')) { $ips = explode(', ', $remote_ip); $remote_ip = $ips[0]; } if($remote_ip == "192.168.1.2"){ //use servers ip echo "from my server"; }
-
Filtering is still ok to use in forms, it will let users know it's required or something not correct with it. What part of the form was wrong. But as Jacques explained is not something you should rely on, all input should be escaped and also the data you expect it to be. Look into constraint validation for forms You can mess around with this form setting minimum and maximum values. <form action="" method="post"> Name: <input type="text" user="" pattern=".{3,10}" required placeholder="3 to 10 characters"> <input type="button" name="submit" value="Go"> </form> min="3" max="10" could also work
-
converting strings from foreach into a single array
QuickOldCar replied to tarquino's topic in PHP Coding Help
You could remove this section and not do ingredients. if (is_array($val)) { foreach ($val2 as $key => $val3) { if ($val3 != NULL || trim($val3) != '') { $string .= str_replace(",", " ", $val3) . " "; } } } -
As kicken stated, update each a new order in the database upon doing the submit Query all your users and their orders Change the selected users order to an insane high number would never use Sort the new array by lowest order and update them all with an update set query but using the order ranges you want. My first impression looking at this was to use a timestamp/date based system. Update the timestamp to the current timestamp the selected user. The biggest difference in time the database compared to the current time would be lowest order.
-
Ha, I thought the same thing, including the question mark. Edit: I wondered the purpose for checking that length. To limit the max characters allowed?
-
Basically you need a cms (content management system) I see 3 choices here. 1. Hire someone to do this for you. 2. Learn a lot and try to do this on your own.(This won't be easy, Is a lot to it and will take a while, providing do it correctly as well) lots more out there if look html5 htmldog html-5-tutorial udemy css htmldog csstutorial echoecho php php.net javascript htmldog ajax mozilla tizag jquery jquery learn Those would be the common things to learn in addition to databases (mysql is popular) and their connection types like mysqli or pdo, xml - tizag tutorial, json or whatever else may need If actually making a cms there is also frameworks could also learn and may make it easier and better for you. laravel symphony2 You can use php as a template engine if are slick enough, is also twig and smarty which are the popular ones. 3. Use a premade cms system, is many opensource ones out there for free. If you don't have the desire or time to learn, this is what you should do. wordpress (a wealth of plugins and themes, free and paid) joomla (a wealth of plugins and themes, free and paid) drupal (modules and themes, free and paid) ezycardealer (get what it is) jt carframework (get what it is) magneto (while the cms is free, most every addition have seen for this cost some money, but some may be worth it) Browse the net and shop around, might be one out that there suits your needs better I'm all for people learning and do on their own, but all you have is a few simple html pages that won't even be of use the proposed new site.
-
converting strings from foreach into a single array
QuickOldCar replied to tarquino's topic in PHP Coding Help
This isn't the greatest thing to do as arrays can get huge, more work just to check for a typo. Lucene has this built in using fuzzy searches <?php if (isset($_GET['s']) && trim($_GET['s']) != '') { $search = strtolower(trim($_GET['s'])); } else { $search = ''; } ?> <form action="" method="get"> <input type="text" name="s" value="<?php echo $search;?>"/> <input type="submit" value="GO" /> </form> <?php $cakes = array( "jade" => array( "Chocolate Cake" => array( "ingredients" => "Chocolate, eggs, flour" ), "Cookie Dough Cake" => array( "ingredients" => "Cookies, Double cream, flour, eggs" ) ), "jessica" => array( "Lemon Cheesecake" => array( "ingredients" => "Lemon, eggs, flour" ), "Belgian Chocolate Cake" => array( "ingredients" => "Dark belgian chocolate, eggs, flour" ) ), "stephanie" => NULL ); $string = ''; //keep blank //find each word from the array values foreach ($cakes as $val) { if (is_array($val)) { foreach ($val as $key => $val2) { $string .= $key . " "; if (is_array($val)) { foreach ($val2 as $key => $val3) { if ($val3 != NULL || trim($val3) != '') { $string .= str_replace(",", " ", $val3) . " "; } } } } } } //remove whitespace and multiple spaces $string = preg_replace('/\s+/', ' ', strtolower(trim($string))); //explode the spaces in string and create an array $suggestions = array(); $suggestions = explode(" ", $string); $levenshtein = array(); //loop the suggestions array against levenshtein foreach ($suggestions as $suggestion) { $levenshtein[$suggestion] = levenshtein($search, $suggestion); } if (!empty($levenshtein)) { //sorted by lowest number of changes asort($levenshtein, SORT_NUMERIC); //show all weighted results echo "<pre>"; print_r($levenshtein); echo "</pre>"; //show only the closest results foreach ($levenshtein as $suggestion => $weight) { //weights of 1 or 2 usually the closest matches, zero is an exact match if ($weight > 0 && $weight < 3) { echo $suggestion . "<br />"; } } } ?> searching for coookie returns Array ( [cookie] => 1 [cookies] => 2 [cake] => 4 [chocolate] => 5 [double] => 5 [lemon] => 6 [dark] => 6 [dough] => 6 [cream] => 6 [flour] => 6 [belgian] => 7 [eggs] => 7 [cheesecake] => 8 ) cookie cookies -
Creating queries from a form dynamically
QuickOldCar replied to pcristian43's topic in PHP Coding Help
You could start by finding all the databases,tables,columns. SHOW DATABASES SHOW TABLES SHOW COLUMNS Loop each to get all the results, easier to make functions for them. Do normal queries using the data you discovered. -
array_push() not adding anything to array
QuickOldCar replied to pcristian43's topic in PHP Coding Help
Are you aware POST is already an array? print_r($_POST); -
As you described, if your complete article is on another site there would be no need to go anywhere else. Those are things you should try to prevent. People copying your content should be getting permission from you, if they don't you can make them remove it. If they have automatic scrapers and you don't want them to copy it...block their server ip through htaccess. https://support.google.com/webmasters/answer/66359 It's a big issue others copying content, plagiarism checkers have been made. http://smallseotools.com/plagiarism-checker/ The idea is to find some unique content in your article, then uses the search engines to see if there was any results. I found this same article by doing an exact google search with "around 100 likes. Has anyone tried that? Does it work? Because" http://forums.devshed.com/search-engine-optimization-108/moronic-seo-questions-965892.html Search engines do not always follow the same rules, depends on the content, the site posting it, where the sources come from. Take news articles as an example, I read the same articles a lot from news sites, they aggregate them from news api's. Search engines still index them all even though they are all similar. You can try and keep up with SEO and supposed algorithms, to me the most important about SEO is having metadata within the head of your page. Normal meta data, opengraph or oembed even. Every so often I see these "tricks" to rank higher or better SEO, some may work for a short while, but usually the search engines catch on and will make changes. Creating pretty seo-friendly links helps more for sharing them other places, search engines can also find them other places and associate the link to the hyperlinked title as well. An example On your site the link may be Pet Hotel You or someone else can share this link as Dog Grooming Service Both links are the same, but now you added more to the title that could end up in a search if someone looked for dog grooming. Adding additional data into the alt of the link could also help The best thing you can do SEO purposes is to use the most important words the beginning of your titles and articles Always have new and original content. Try to prevent duplicate content and similar links within the same site. I'll tell you a story my experience aggregating articles and duplicate content and how it reflects on search results. I aggregated thousands of websites. Every time websites made a new post or even edited it would update on my site. Because my site was ranked higher than theirs 9 out of 10 times I would be before them in the search results, even ones paying google for rank and seo tools. Usually a site like I had would be penalized, but since I was letting them crawl my new articles all the time and had many thousands new a day they let it go. At the time I was helping search engines find content. My site categorized and tagged articles automatic. I was an extremely popular site and ranked high at the time. I did not try to claim or own the articles and always posted the titles back to the originating site. The content creators liked the traffic I gave them and would not want to ban my server.(pretty much what search engines do, except mine was a website) I would truncate the articles content as to just give the reader a brief overview, to read the entire article would require them going to the original site. So in the end, it did not matter if they clicked the link through the search engine or my site, they both took the person to the original location.
-
array_push() not adding anything to array
QuickOldCar replied to pcristian43's topic in PHP Coding Help
An example saving them into a session <?php session_start(); ?> <!DOCTYPE html> <html> <head> <title> </title> </head> <body> <div class="site"> <div class="menu"> </div> <div class="head"> </div> <div class="content"> <form action="" method="post"> <input type="text" value="" name="add"/><br/> <input type="submit" value="Add" name="submit"/> </form> <?php //create array if(!$_SESSION['list']){ $_SESSION['list'] = array(); } //check is set and not blank if (isset($_POST['add']) && trim($_POST['add']) != '') { //assign variable $field = trim($_POST['add']); //see if doesn't already exist in the array if (!in_array($field, $_SESSION['list'], true)) { //add it to the array //array_push($_SESSION['list'], $field); //or $_SESSION['list'][] = $field; //check if it added to array if (!in_array($field, $_SESSION['list'], true)) { echo "not added <br />"; } else { echo "added <br />"; } } else { echo "already existed <br />"; } } //loop and show results from array if not empty if (!empty($_SESSION['list'])) { echo "<h2>List</h2>"; foreach ($_SESSION['list'] as $values) { echo "$values <br />"; } } ?> </div> <div class="footer"> </div> </div> </body> </html> -
array_push() not adding anything to array
QuickOldCar replied to pcristian43's topic in PHP Coding Help
if(isset($_POST['add']) && trim($_POST['add']) != '') { That should be sufficient to ensure is not empty data Any data type checking or filtering might be nice You may even want to check that a value doesn't already exist in the array Can use the same method to ensure is now added to the array. if(!in_array($field,$list, true)){ array_push($list, $field); } Additionally you don't echo an array, you need to loop it foreach($list as $values){ echo "$values <br />"; } The new array will always be set empty and a new single value added in the form unless saved into sessions or a database, did something else with it. So all together <!DOCTYPE html> <html> <head> <title> </title> </head> <body> <div class="site"> <div class="menu"> </div> <div class="head"> </div> <div class="content"> <form action="" method="post"> <input type="text" value="" name="add"/><br/> <input type="submit" value="Add" name="submit"/> </form> <?php //create array $list = array(); //check is set and not blank if (isset($_POST['add']) && trim($_POST['add']) != '') { //assign variable $field = trim($_POST['add']); //see if doesn't already exist in the array if (!in_array($field, $list, true)) { //add it to the array array_push($list, $field); //check if it added to array if (!in_array($field, $list, true)) { echo "not added <br />"; } else { echo "added <br />"; } } else { echo "already existed <br />"; } } //loop and show results from array if not empty if (!empty($list)) { foreach ($list as $values) { echo "$values <br />"; } } ?> </div> <div class="footer"> </div> </div> </body> </html> -
Try to do a repair on your database, it may have gotten corrupted somehow. Using something like phpmyadmin can do it Visit http://nfspolicehq.com/phpmyadmin/ log in with your credentials click your database, should see a pile of tables to the right select "Check All" then to the right is a "With Selected" select the "Repair table" If you have more tables than shown do this process as many times as need to. You can also do through command line with mysql mysqlcheck --repair --all-databases http://dev.mysql.com/doc/refman/5.7/en/rebuilding-tables.html
-
The in your face contact info up top should go, you have all that in your contact page and easy to find. Remove the underlines text-decoration: none; I feel you should do full dates and place them under the article titles. Having 3 fields just 2 numbers can be confusing, depending where people live would interpret them in different orders http://en.wikipedia.org/wiki/Date_format_by_country d-m-y m-d-y y-m-d How about when is the 8th day of June for an example 08-06-14 06-08-14 14-06-08 Don't make users have to interpret the date, make it absolutely no question as to what the date is. Along with that remove the here and make the title a hyperlink. I myself would add a source link to the main domain versus the mixed sentences as you have and the here with the hyperlink. source: youtube.com yours: Gold Will Take The Rocket Ship Back Up 18-11-14 Long time Gold advocate Peter Schiff was interviewed by Kitco News and discussed amongst other things his views on the Gold Market. He was as bullish as ever and the ten minute interview can be viewed here proposed suggestion:(remove the underline) Gold Will Take The Rocket Ship Back Up November 18th 2014 Long time Gold advocate Peter Schiff was interviewed by Kitco News and discussed amongst other things his views on the Gold Market. He was as bullish as ever in the ten minute interview. source: youtube.com
-
Search engines like original unique content and will base the reliability of the source content off that. If you were to copy content from another site it would be best to pass a canonical link to the other site as to not lower your score. <link rel="canonical" href="http://originalsite.com/copied-content"/> This should help your site not get penalized as having duplicate content or being a content aggregator. In that same respect it's not helping your site either as the link to your article gets passed on to the original site. Value as to your visitors is a bit different, if that's why they come to your site then have to weigh your options which benefits you more. As for the facebook and likes, I've seen people pay for lesser methods to try and gain traffic to their sites, try it out, won't hurt.
-
Edit Microsoft Word file on website
QuickOldCar replied to NotionCommotion's topic in PHP Coding Help
Some sort of csv conversion may be better. Then could edit it in a form on site or manipulate the data in other ways.. -
Since nobody ever replied to this will try to help a little. You don't want to change the class, instead you want to use your already scraped data with a search and pagination. What if was more than 90 results for that specific search term? If instagram api has a from startrow or a way to query by date can take advantage of that From what I see is a max 90 results anything you searched for, such as your kitten The $i variable is used as a count but not really doing anything. It could potentially be used as a pagination on just the results got back from the live api, but in this instance you are saving them all to a database so is worthless. You can call to the script using single GET requests...coming from an array or lists you create somehow and here is a way can do that //add whatever want to the array $fetch_terms = array("kitten","dog","cat","funny","animal","penguin","horse","donkey"); shuffle($fetch_terms);//randomize it if(isset($_GET['fetch']) && trim($_GET['fetch']) != ''){ $fetch_term = trim($_GET['fetch']); } else { $fetch_term = $fetch_terms[0]; } then edit this line to $photos = $instagram->getTagMedia($fetch_term); now if call to the script with something like mysite.com/instagram.php?fetch=kitten it will retrieve kitten results if don't use the fetch query parameter in the url it would use a random one from the $fetch_terms array to handle ensuring getting them all, well unless you can query instagram a certain date, will just get latest ones, I never personally looked at their api but you could just run it as often as like, and set unique constraints in your database on 1 or more columns to ensure duplicates are not added $link = $post->link; looks like a good item to make it unique the command to do this for mysql ALTER TABLE userfeeds ADD UNIQUE (link); Unless you wanted just kittens,you need to run this many times a day on every fetch term you used in the script. $caption data could be used for your search later on, but you could also make a new column and use the $fetch_term you specify as a category/tag system This way is no need to search but instead can make the categories and a click will get you all those results...or have both. A combination search and pagination is very possible once you have the data stored mysql. Make a simple search form I suggest looking at this old mysql_ function pagination tutorial Mac_Gyver was so nice as to make a combo mysql/mysqli one http://forums.phpfreaks.com/topic/291074-php-mysli-pagination-with-items-per-page-select-option/?do=findComment&comment=1491152 Depending what you do with categories or can use fulltext search (which takes a bit of work,such creating an index in mysql) or do a LIKE in the sql query Since people do not do single words all the time, you would need to explode them by spaces For fulltext is a few different types of advanced searches and operators, take a look at boolean mode Example fulltext in boolean mode $sql = "SELECT * FROM userfeeds WHERE MATCH (caption) AGAINST ('$search_term' IN BOOLEAN MODE) Limit $offset, $rowsperpage"; Example LIKE sql $sql = "SELECT * FROM userfeeds WHERE caption LIKE '%$search_term%' LIMIT $offset, $rowsperpage"; The count sql also needs to be modified..minus the limit with offset and rows per page $sql = "SELECT COUNT(*) FROM userfeeds WHERE caption LIKE '%$search_term%'"; Now that's all fine for the search aspect and pagination, but should also make multiple sql queries if nothing was searched for so can do all results $search_term needs to exploded by spaces if multiple words, LIKE needs multiple where/and/or clauses while fulltext are spaced or use the + operator or others Untested code using my search integrated into mac_gyvers pagination with some changes <?php //configuration define('SOURCE','mysqli'); // the method/type of data source - mysql, mysqli //database $db_server = "localhost";//sever name, usually localhost $db_user = "username";//mysql username $db_pass = "password";//mysql password $db_database "database_name";//mysql database $tablename = "userfeeds"; //set table name for query $column = "caption"; //set column name for query $fulltext_search = false; //like or fulltext ...determine what type of search method, fulltext requires creating an index //end configuration $search_query = '';//leave blank switch(SOURCE){ case 'mysql': // database connection info $conn = mysql_connect($db_server,$db_user,$db_pass) or trigger_error("SQL", E_USER_ERROR); $db = mysql_select_db($db_database,$conn) or trigger_error("SQL", E_USER_ERROR); break; case 'mysqli': // database connection info $conn = mysqli_connect($db_server,$db_user,$db_pass,$db_database) or trigger_error("SQL", E_USER_ERROR); break; } if(isset($_GET['search']) && trim($_GET['search']) != ''){ $explode_search = array(); $search = trim($_GET['search']); $search = preg_replace('/\s+/', ' ', $search); $explode_search = explode(" ",$search); if($fulltext_search == true){ $trimmed_words = ''; foreach ($explode_search as $trim_words) { if (substr($trim_words, 0, 1) != "-" || substr($trim_words, 0, 1) != '"') { $trim_words = trim($trim_words); $trimmed_words .= " +$trim_words"; } else { $trim_words = trim($trim_words); $trimmed_words .= " $trim_words"; } }//end loop $trimmed_words = trim($trimmed_words); $trimmed_words = preg_replace('/\s+/', ' ', $trimmed_words); case 'mysql': $trimmed_words = mysql_real_escape_string($trimmed_words); break; case 'mysqli': $trimmed_words = mysqli_real_escape_string($conn, $trimmed_words); break; } //assembled search query $search_query .= "WHERE MATCH ($column) AGAINST ('$trimmed_words' IN BOOLEAN MODE)) "; } else { $multiple_like = array(); foreach($explode_search as $search_word){ switch(SOURCE){ case 'mysql': $search_word = mysql_real_escape_string($search_word); break; case 'mysqli': $search_word = mysqli_real_escape_string($conn, $search_word); break; } $multiple_like[] = "`$column` LIKE '%" . $search_word . "%' "; }//end loop if(count($multiple_like) > 0 ){ //assembled search query $search_query .= "WHERE " . implode (' AND ', $multiple_like); } }//end fulltext if }//end GET search switch(SOURCE){ case 'mysql': // find out how many rows are in the table $sql = "SELECT COUNT(*) FROM $tablename $search_query"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); $r = mysql_fetch_row($result); $numrows = $r[0]; break; case 'mysqli': // find out how many rows are in the table $sql = "SELECT COUNT(*) FROM $tablename $search_query"; $result = mysqli_query($conn,$sql) or trigger_error("SQL", E_USER_ERROR); $r = mysqli_fetch_row($result); $numrows = $r[0]; break; } // number of rows to show per page $rowsperpage = 10; // (default value when using dynamic rows per page) // dynamic rows per page, handling and form $per_page = array(1,5,10,25,50); // choices for select/option menu. also used to limit (min, max) the submitted value $rowsperpage = isset($_GET['perpage']) ? (int)$_GET['perpage'] : $rowsperpage; // get submitted value or the default $rowsperpage = max(min($per_page),$rowsperpage); // limit to the minimum value $rowsperpage = min(max($per_page),$rowsperpage); // limit to the maximum value // produce rows per page form $rpp_form = "<form method='get' action=''>\n<select name='perpage' onchange='this.form.submit();'>\n"; foreach($per_page as $item){ $sel = $rowsperpage == $item ? 'selected' : ''; $rpp_form .= "<option value='$item' $sel>$item</option>\n"; } $rpp_form .= "</select>\n<noscript><input type='submit'></noscript>\n</form>\n"; // find out total pages $totalpages = ceil($numrows / $rowsperpage); // get the current page or set a default if (isset($_GET['currentpage']) && is_numeric($_GET['currentpage'])) { // cast var as int $currentpage = (int) $_GET['currentpage']; } else { // default page num $currentpage = 1; } // end if // if current page is greater than total pages... if ($currentpage > $totalpages) { // set current page to last page $currentpage = $totalpages; } // end if // if current page is less than first page... if ($currentpage < 1) { // set current page to first page $currentpage = 1; } // end if // the offset of the list, based on current page $offset = ($currentpage - 1) * $rowsperpage; switch(SOURCE){ case 'mysql': // get the info from the db $sql = "SELECT * FROM $tablename $search_query LIMIT $offset, $rowsperpage"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); $rows = array(); while ($row = mysql_fetch_assoc($result)) { $rows[] = $row; } // end while break; case 'mysqli': // get the info from the db $sql = "SELECT * FROM $tablename $search_query LIMIT $offset, $rowsperpage"; $result = mysqli_query($conn,$sql) or trigger_error("SQL", E_USER_ERROR); $rows = array(); while ($row = mysqli_fetch_assoc($result)) { $rows[] = $row; } // end while break; } // while there are rows to be fetched... foreach($rows as $row) { // echo data echo $row['id'] . " : " . $row['number'] . "<br />"; } // end foreach // display dynamic rows per page form echo $rpp_form; /****** build the pagination links ******/ // range of num links to show $range = 3; // if not on page 1, don't show back links if ($currentpage > 1) { // show << link to go back to page 1 $_GET['currentpage'] = 1; $qs = http_build_query($_GET, '', '&'); echo " <a href='{$_SERVER['PHP_SELF']}?$qs'><<</a> "; // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page $_GET['currentpage'] = $prevpage; $qs = http_build_query($_GET, '', '&'); echo " <a href='{$_SERVER['PHP_SELF']}?$qs'><</a> "; } // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($x == $currentpage) { // 'highlight' it but don't make a link echo " [<b>$x</b>] "; // if not current page... } else { // make it a link $_GET['currentpage'] = $x; $qs = http_build_query($_GET, '', '&'); echo " <a href='{$_SERVER['PHP_SELF']}?$qs'>$x</a> "; } // end else } // end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $currentpage + 1; // echo forward link for next page $_GET['currentpage'] = $nextpage; $qs = http_build_query($_GET, '', '&'); echo " <a href='{$_SERVER['PHP_SELF']}?$qs'>></a> "; // echo forward link for lastpage $_GET['currentpage'] = $totalpages; $qs = http_build_query($_GET, '', '&'); echo " <a href='{$_SERVER['PHP_SELF']}?$qs'>>></a> "; } // end if /****** end build pagination links ******/ ?> Maybe I'll figure out a way to handle the case for mysql and mysqli better a day and also add pdo to this. Didn't have time to fuss with it.
-
I happened to come across an article leading me to Mesosphere Datacenter Operating System the other day. The Mesosphere Datacenter Operating System (DCOS) is a new kind of operating system. It spans all the servers in your datacenter or cloud. Looks promising to me and the video is impressive. https://www.youtube.com/watch?v=UgJMlHdZEx4 Some perks Datacenter services include Apache Spark, Apache Cassandra, Apache Kafka, Apache Hadoop, Apache YARN, Apache HDFS, Google’s Kubernetes, and more. The Mesosphere DCOS supports all modern versions of Linux including: CoreOS, CentOS, Ubuntu and RedHat. It runs on-premise on bare metal or in a virtualized private cloud, such as with VMware or OpenStack. It also runs on all the major public cloud services, including Amazon Web Services, Google Compute Engine, Digital Ocean, Microsoft Azure, Rackspace and VMware vCloud Air.
-
You can also save the json and cache them with expire times If cache file doesn't exist or exists and past the expire time...do the api request and create one for next time some examples from stackoverflow I'm sure that's easier than what are trying to do with inserts.
-
if(isset($_GET['modsToBeChecked'])) { $modsToBeChecked = preg_replace(array("/\r\n+/", "/\r+/", "/\n+/"), "/n",trim($_GET['modsToBeChecked'])); $modsToBeChecked = explode("/n", $modsToBeChecked); var_dump($modsToBeChecked); } You can probably improve it more depending on spaces in mod names, wasn't sure what your data was
-
Maybe the email provider has to scour through a huge list first, not sure but is most likely their end and not yours. Did you read any the urls i posted with having more than a link in an email, have other content as well They all have their own systems for checking emails, is hard but have to work out which methods send more reliable.