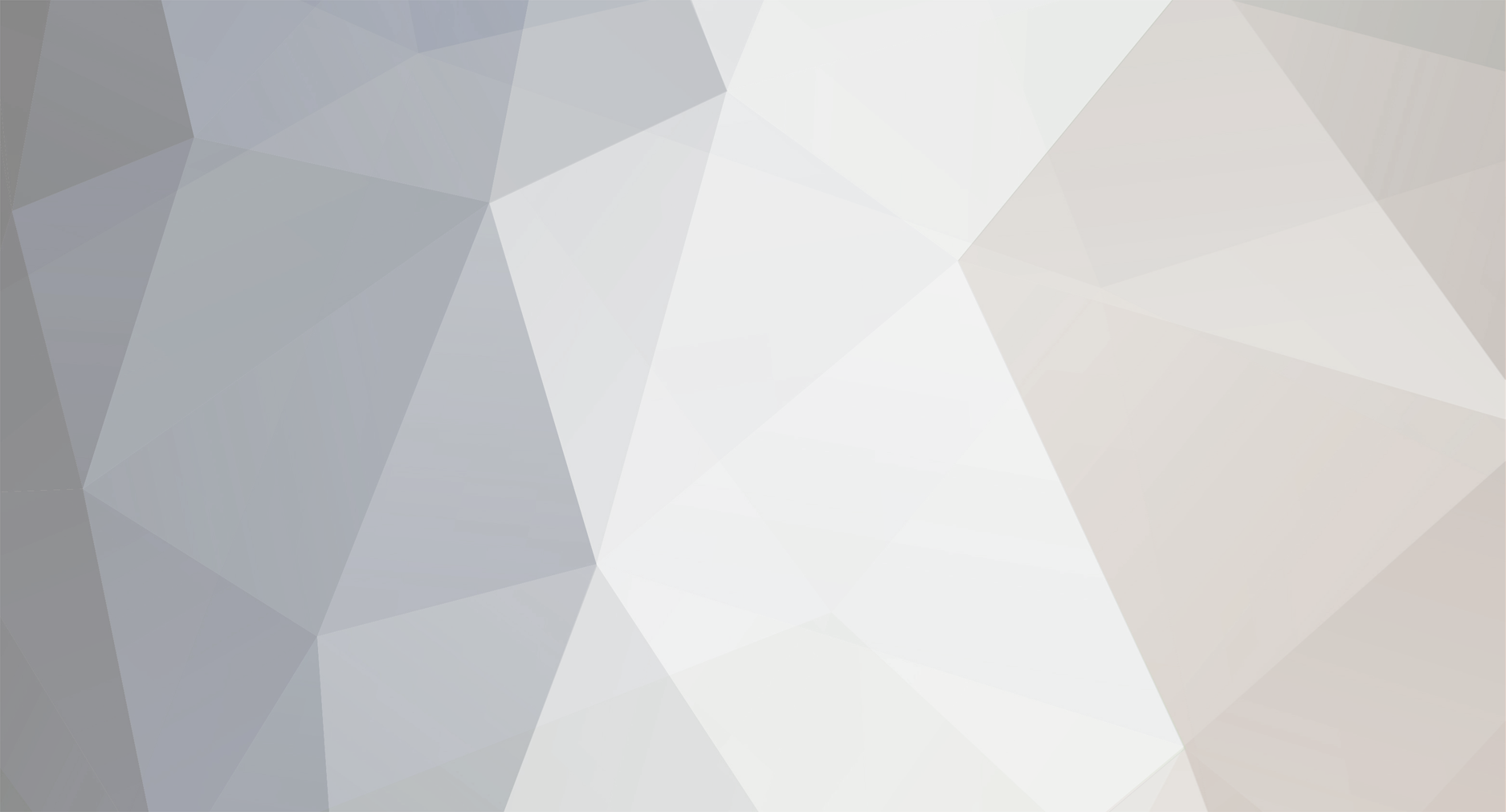
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
I fixed a few errors in that that should have been obvious, maybe you should be displaying errors and showing us them
-
login <?php error_reporting(E_ALL | E_NOTICE); require 'connect.php'; session_start(); if (isset($_POST['submit'])) { $username = trim($_POST['username']); $password = trim($_POST['password']); if (empty($username)) { echo "You did not enter a username, Redirecting..."; echo "<meta http-equiv='refresh' content='2' URL='login.php'>"; exit(); } if (empty($password)) { echo "You did not enter a password, Redirecting..."; echo "<meta http-equiv='refresh' content='2' URL='login.php'>"; exit(); } //Prevent hackers from using SQL Injection to hack into Database $username = mysql_real_escape_string($username); $md5_password = md5($password); $password = mysql_real_escape_string($md5_password); $sql = "SELECT * FROM $tbl_name WHERE username='$username' AND password='$password'"; $result = mysql_query($sql); $count = mysql_num_rows($result); $row = mysql_fetch_assoc($result); $user_level = $row['user_level']; if ($count == 1) { $_SESSION['loggedIn'] = true; $_SESSION['username'] = $_POST['username']; } } ?> register <?php error_reporting(E_ALL | E_NOTICE); require 'connect.php'; echo "<title> Register </title>"; if (isset($_POST['submit'])) { if (isset($_POST['username']) && isset($_POST['password'])) { $username = trim($_POST['username']); $password = trim($_POST['password']); $email = $_POST['email']; $username = mysql_real_escape_string($username); $email = mysql_real_escape_string($email); $md5_password = md5($password); $password = mysql_real_escape_string($md5_password); /* why checking for a username and also a password? isn't this registration? don't you want just no duplicate names? */ $usrsql = "SELECT * FROM $tbl_name WHERE username='$username' AND password='$password'"; $usrres = mysql_query($usrsql); if (!$usrres) { die("Query Failed."); } if (mysql_num_rows($usrres) > 0) { echo "<style>body {background-image: url('http://desktopwallpapers.biz/wp-content/uploads/2014/09/Website-Background-Cool-HD.jpg');} #uexist {position: fixed;top: 200px;left: 550px; font-size: 24px;}</style>"; die("<font color='yellow' face='Tahoma'> <b> <center> <p id='uexist'> The username you entered is already in use. </p> </center> </b> </font>"); } else { // DO NOTHING } if (empty($username)) { print("<font color='red'>You did not enter a username!</font> <br> <br>"); } else if (empty($password)) { die("<font color='red'>You did not enter a password!</font>"); } if (strlen($username) < 3) { die("<font color='red'>Your username must be over 3 characters!</font>"); } else if (strlen($username) > 20) { die("<font color='red'>Your username is over 20 characters and must be under! usernames are 3 - 20 characters!</font>"); } if (isset($_POST['email']) && filter_var(trim($_POST['email']), FILTER_VALIDATE_EMAIL)) { $email = trim($_POST['email']); } $query = "INSERT INTO `x_users` (username, password, email) VALUES ('$username', '$password', '$email')"; $result = mysql_query($query); if ($result == 1) { print("Thank you, your account has been created!"); } } } ?>
-
You don't need stripslashes, mysql_real_escape_string does that Be sure to trim on both registration and your login. grr were doing it 2x $username = $_POST['username']; $password = md5($_POST['password']); Just this $username = mysql_real_escape_string(trim($_POST['username'])); $md5_password = md5(trim($_POST['password'])); $password = mysql_real_escape_string($md5_password); If this don't work tom, post both your current register and login forms.
-
You mean the advice didn't work? trimming post values didn't work? You know that's going to be as secure as just checking if the password is bobby? Little overboard, but not that far off. $md5_password = md5(trim($_POST['password'])); $sql = "SELECT * FROM $tbl_name WHERE username='$username' AND password='$md5_password'";
-
I guess you skipped past my post, answers to your issue in there.
-
oops my bad, meant to add the submit or even !empty For the rest it depends if have multiple forms or optional fields within a form. I got into the habit of checking them while trim or filter.
-
The snippet repository section has been read only and nothing new for a long time. Most of the ones there are pretty old. The tutorials sections last post was from 2010 The old pagination tutorial needs a good mysqli and pdo version there. http://www.phpfreaks.com/tutorial/basic-pagination I noticed how slow the sites been a while now, maybe need something to lure more in.
-
Cockpit CMS Blog example not working
QuickOldCar replied to CrashyBang's topic in Third Party Scripts
Most likely a directory path issue, can try doing this before the require dirname(__FILE__) ensures that the require_once function uses an absolute rather than relative path Use the folder names $directory_path = dirname(__FILE__) . DIRECTORY_SEPARATOR; require_once($directory_path . "/folder_name/folder_name/partials/errorlogging.php"); echo these as well <?php=$post['content'];?> to <?php echo $post['content'];?>- 3 replies
-
- content-management
- syntax-error
-
(and 3 more)
Tagged with:
-
show the error you get here is it actually not seeing it in the $data array, or is it your logic echo $data['unprinted'] and see if has a value do a var_dump($data) and see what's going on with it
-
Welcome echo. Were probably better off waiting, php was really buggy then and has come a long way from what it once was.
- 3 replies
-
- introductions
- greetings
-
(and 3 more)
Tagged with:
-
What your code should do: check if POST is set if(isset($_POST){ //proceed with code } else { //show a message,any errors,redirects or a form } assign variables to POST values only if they exist trimming the unseen whitespace as well with trim() (if a password has whitespace at the ends would become a different hash) checking for empty or blank values if(isset($_POST['username']) && trim($_POST['username']) != ''){ $username = trim($_POST['username']); } checking what type of data the value is character type checking filter validation filter functions sanitize email example if(isset($_POST['email']) && filter_var(trim($_POST['email']), FILTER_VALIDATE_EMAIL)){ $email = trim($_POST['email']); } as Frank said you compare 2 encrypted passwords, one encrypted from the form value and the saved one in database don't use md5() but something like password_hash() don't use outdated mysql_* functions, use mysqli_* or pdo check/sanitize/filter then escape anything you are using in a mysql query mysqli_real_escape_string() or prepared statements before you try and do anything with the database, ensure that all your variables exist, not empty, data you expect and escaped it's nice to incorporate error handling to assist you and your users as to whats going on can create an error array or messages upon any errors throughout your script error reporting While you are creating code add this to the top of your php script error_reporting(E_ALL | E_NOTICE); ini_set('display_errors', '1'); later on set it to log errors or not show errors a production site error_reporting(0); ini_set("log_errors", 1); ini_set("error_log", "/tmp/php-error.log");//or can look default folder
-
I thought the above ones were a bit bloated, not to mention not all free. I managed to make my own version using the html5 contenteditable attribute Saw this tutorial, used my own ajax and secured the request form with sessions and a lot of checking http://w3lessons.info/2014/04/13/html5-inline-edit-with-php-mysql-jquery-ajax/ Works like a champ, I made a domain policy restrictions table with wildcard,allow/ban
-
I guess depends on the type of game, if sessions doesn't work then save data to the database instead. Something has to track the games played each time and then reset them each time the script is loaded, can't just do it with code.
-
Your best bet is to make a session to retain the data I would make a $_SESSION['already_played'] array Every time a map is played it can add it to $_SESSION['already_played'] For the game maps list you would show all but the ones that are in the $_SESSION['already_played'] When the number of maps left is 1...you can clear the $_SESSION['already_played'] so they all show again Guessing this may work session_start(); //top of the script //define it if doesn't exist if (!$_SESSION['already_played']) { $_SESSION['already_played'] = array(); } $new_maps = array(); if ($message->getUserTeam() == "CT") { $team = ($this->side['team_a'] == "ct") ? $this->teamAName : $this->teamBName; $maps = \eBot\Config\Config::getInstance()->getMaps(); if (in_array($preg['mapname'], $maps) && !in_array($preg['mapname'], $_SESSION['already_played'])) { $this->playMap['ct'] = $preg['mapname']; $this->say($team . " (CT) \003Removed \004" . $preg['mapname']); //adding map into session $_SESSION['already_played'][] = $preg['mapname']; } else { $this->say($preg['mapname'] . " was not found! Available maps are:"); $new_maps = array_diff($maps, $_SESSION['already_played']); //maps in session removed from maps array //reset already played session when list is 1 or less if (count($new_maps) <= 1) { $_SESSION['already_played'] = array(); } foreach ($new_maps as $map) { $mapmessage .= "$map, "; } } $this->say(substr($mapmessage, 0, -2));
-
Modify video frames with Facebook data
QuickOldCar replied to Krishnaveni's topic in PHP Coding Help
It seems like you are taking the correct approach to do something like this. Of course it's tedious!!, look what you are trying to do there. Only way to get around flash is to use html5 video. Will need to save multiple video types depending which browser the person is using. If a person is using an old browser will not work (yes people still use old ones then complain) Mozilla explains this nicely https://developer.mozilla.org/en-US/docs/Web/HTML/Supported_media_formats Basically you will want something like this which means 3 files needed. So now you can see why forcing people to install flash is still being commonly used. Nice idea, but unless all browsers get on board with a same file type all can use...is a huge waste of resources.. <video id="html5Movie" width="640" height="360" preload controls> <source src="HTML5_H264.mov" type='video/mp4; codecs="avc1.42E01E, mp4a.40.2"' /> <source src="HTML5_Ogg.ogv" type='video/ogg; codecs="theora, vorbis"' /> <source src="HTML5_WebM.webm" type='video/webm; codecs="vp8, vorbis"' /> </video> -
I agree with Jacques1 100% He gave the perfect response. If someone doesn't want to follow good advice and get offended....is their loss.
-
Need help with database query (I think?) WORDPRESS
QuickOldCar replied to drewhew's topic in Applications
I have to admit for the cheap price they give a lot, one of nicest directories with a theme have seen to date for wordpress. Reminds me of airbnb.com Is going to be harder to get help because not many would be willing to go buy it just to help you out. Most likely doing it in a running environment as well. Hard enough to get free help, but redesigning, changing the functions that theme and plugin is probably more than think it is. You never know what custom functions they did or how hooked into wordpress until looked at it all. The creators have a huge advantage over anyone else, they know how they made it work. I assumed was just a plugin using any theme, what you have there will be more complicated to modify, not impossible, but more work. -
Use a database and retrieve only what you need from it. Large arrays will never be good to work with.
-
Trying to use variables from another file out of memory
QuickOldCar replied to Andrew789123's topic in PHP Coding Help
The script does not have enough memory determined by php settings It's exceeding 128mb of memory. The random and hashing process using too much? If you added this to the top of the script can set the memory per script ini_set('memory_limit','256M');//set amount to what need For testing purposes you can set it to unlimited. ini_set('memory_limit', '-1'); I feel if you set the memory higher and still runs out or is taking long to execute the script then you need to redesign your code to be more efficient. To increase the timeout is this. set_time_limit(100);//amount in seconds You can also make changes to your php.ini file, but you don't really want to allow too much per script or will for sure run into issues entire server. -
Not able to open Network shared folder in Chrome
QuickOldCar replied to jessentha's topic in PHP Coding Help
You can try this http://omnitechsupport-reviews.com/using-passive-ftp-in-google-chrome-browser/ Maybe this is an assignment, but I'll say this... I had to change something in firefox as well to see ftp:// links I don't mess around with ftp through browsers much. If I want to give a user access I do it through sftp and user accounts. If I want to share files can make them downloadable in other ways. glob() is one way. Merely by making a folder with no index file can show the files within. To connect to ftp/sftp I always use filezilla, I suppose you can get a plugin for chrome -
Because you need to have everything in your while loop or make them an array and do later on. $stmt=$pdo->query("select datediff(due, paid) as diff where name =:name"); $stmt->execute() $total_points = 0; while ($row = $stmt->fetch (PDO::FETCH_ASSOC)) { $diff=$row['diff'] ; switch ($diff) { case 0: case 1: case 2: case 3: case 4: case 5: $point = 6; break; case 6: case 7: case 8: case 9: case 10: $point = 4; break; default: $point = 1; } $total_points += $point; } echo $total_points;
-
Not able to open Network shared folder in Chrome
QuickOldCar replied to jessentha's topic in PHP Coding Help
I don't believe anything you do in the php script can change that, that is browser limitations and settings. -
Supplying some code would make this easier. If you have the map data in an array... doing by it's array key with unset() unset($array[0]); array_splice() array_splice($array, 0, 1);//array,key position, amount to remove from key position deleting an element in an array if only know it's value $key = array_search($value,$array); if($key!==false){ unset($array[$key]); } To reset the array keys array_values() $array = array_values($array);
-
is my php correct? cant find any syntax errors
QuickOldCar replied to Jayden_Blade's topic in PHP Coding Help
Try this for error reporting error_reporting(E_ALL | E_NOTICE); ini_set('display_errors', '1'); With mysqli you have to pass the database variable for the connection along with the query Look in dbcon.php for whatever you named it. $result = mysqli_query($database_connection,$sql); did you mean to use curly braces? $sql = "SELECT * FROM `user` WHERE `username`='($_POST['username'])' LIMIT 1"; Escape anything used in a query to your database mysqli_real_escape_string() So try this (with your proper database connection variable) $sql = "SELECT * FROM `user` WHERE `username`='".mysqli_real_escape_string($database_connection ,$_POST['username'])."' LIMIT 1"; -
Need help with database query (I think?) WORDPRESS
QuickOldCar replied to drewhew's topic in Applications
So you are using javo directory, did you contact the creators and ask for such a feature? Sometimes they are willing to add them to improve their product.