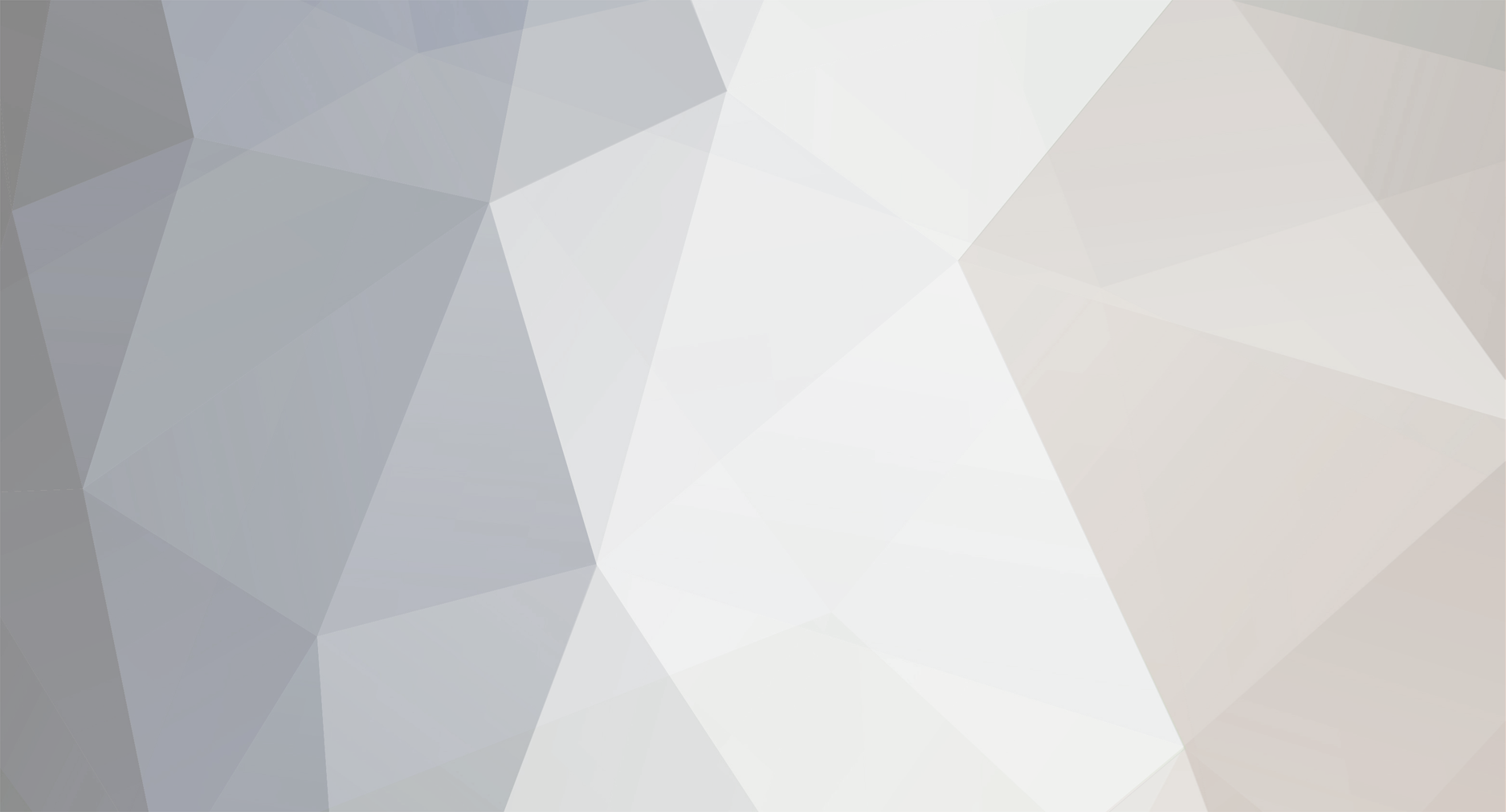
zq29
-
Posts
2,752 -
Joined
-
Last visited
-
Days Won
1
Posts posted by zq29
-
-
[a href=\"http://uk2.php.net/manual/en/language.types.string.php#language\" target=\"_blank\"]HEREDOC syntax[/a]
-
You could use explode(), here's how it works...
[code]<?php
$ip = "192.168.1.2";
$bits = explode(".",$ip);
echo $bits[0]; //Prints 192
echo $bits[1]; //Prints 168
echo $bits[2]; //Prints 1
echo $bits[3]; //Prints 2
?>[/code] -
Moved to our 3rd party scripts forum - The PHP Newbie Help forum is for requesting help with custom scripts that you are developing yourself - Not for requesting help with 3rd party scripts.
-
Yeah, that should be fine, you could probably even do it with a foreach() for less typing...
[code]<?php
$str = "";
foreach($_POST['category'] as $x) {
$str = $str.$x.",";
}
$str = substr($str,0,-1);
?>[/code] -
Just ran a few tests, and finaly managed to get it to [i]not[/i] work for me. Think I made a silly mistake in my code... Try replacing this line:[code]$newname = $random.substr($filename,$pos);[/code]
-
Try this: [code]<input name='category[]' type='checkbox' dir='ltr' value='9,' /.>
<input name='category[]' type='checkbox' dir='ltr' value='10,' />
<input name='category[]' type='checkbox' dir='ltr' value='11,' />
<input name='category[]' type='checkbox' dir='ltr' value='12,' />
<input name='category[]' type='checkbox' dir='ltr' value='13,' />[/code]
You can then try this on your processing page to see how its structured:
[code]<?php
echo "<pre>";
print_r($_POST['category']);
echo "</pre>";
?>[/code] -
[!--quoteo(post=375563:date=May 20 2006, 09:23 PM:name=semtex)--][div class=\'quotetop\']QUOTE(semtex @ May 20 2006, 09:23 PM) [snapback]375563[/snapback][/div][div class=\'quotemain\'][!--quotec--]What's the best way to solve this? I mean I can't use php's htmlentities() function on every echoed text, can I?[/quote]
You can, although what I do is just type the entities instead of the actual character - Just a matter of habit now...
[code]<?php
echo "I really fancy some tea & crumpets!";
?>[/code] -
[!--quoteo(post=375527:date=May 20 2006, 05:28 PM:name=toplay)--][div class=\'quotetop\']QUOTE(toplay @ May 20 2006, 05:28 PM) [snapback]375527[/snapback][/div][div class=\'quotemain\'][!--quotec--]Your code shows: $random=rand(0000,9999);
Putting four zeros is pointless. If you want to always have a four digit number, then you have to set the minimum random value to start with one thousand:
$random = rand(1000, 9999);[/quote]
An option could be to use str_pad(rand(0,9999),4,"0",STR_PAD_LEFT); should you wish to have four digits starting from 0000 to 9999.
[!--quoteo(post=375527:date=May 20 2006, 05:28 PM:name=toplay)--][div class=\'quotetop\']QUOTE(toplay @ May 20 2006, 05:28 PM) [snapback]375527[/snapback][/div][div class=\'quotemain\'][!--quotec--]SemiApocalyptic, just multiply by -1 to turn a positive number to a negative (and vice versa) instead of concatenating a dash (minus sign).
[/quote] Thanks! Learn something new every day!
With regards to the main question, I can't see why my original code doesn't work, along with Toplay's revision. I'll post it up again with comments to show whats going on:
[code]<?php
$filename = $_FILES['ulfile']['name']; //Lets call it test.txt
$random = str_pad(rand(0,9999),4,"0",STR_PAD_LEFT); //Pick a number between 0000 and 9999
$pos = strrpos($filename,"."); //Get the location of the last period we can extract the extension
$newname = $random.substr($filename,$pos * -1,$pos); //Add the random number to the extension .txt
?>[/code] -
You can just pull the required information from your database, loop through it and create the entereies in the select box. Here's an example:
[code]<?php
$result = mysql_query("SELECT `name`,`id` FROM `table`") or die(mysql_error());
echo "<select name='names'>";
while($row = mysql_fetch_assoc($result)) {
echo "<option value='$row[id]'>$row[name]</option>";
}
echo "</select>";
?>[/code] -
There are a number of topics already regarding prefered editors, a quick look turned up the following two that you may wish to read and/or participate in:
[a href=\"http://www.phpfreaks.com/forums/index.php?showtopic=54859\" target=\"_blank\"]Which PHP-Editor do you think is the best?[/a]
[a href=\"http://www.phpfreaks.com/forums/index.php?showtopic=93740\" target=\"_blank\"]PHP Coding software[/a]
-
I see no reason as to why this topic should be closed, so I have opened it so others can feel free to have their input on the mentioned software, or recommend alternatives. Without creating new topics on the same, or similar theme.
-
Check the manual for the following functions:
imagesettile()
imagefill()
Those should get you on the way. -
This [i]should[/i] work...
[code]<?php
$filename = $_FILES['ulfile']['name'];
$random=rand(0000,9999);
$pos = strrpos($filename,".");
$mpos = "-".$pos; //Not sure if substr would work with -$pos as a parameter, so created this var.
$newname=$random.substr($filename,$mpos,$pos);
?>[/code] -
As far as I was aware, GMT and UTC are the same thing.
To add 5 hours, you could do it like this. You can add 18000 to the seconds if you prefer though output would be the same.
[code]<?php
echo date("DdSF,Y g:i:sa",mktime(date("g")+5,date("i"),date("s"),date("m"),date("d"),date("Y")));
?>[/code]
As for making it DST correct, I guess you could use a switch() to match the current month, and if DST applies add/remove an hour. -
Nice, looks Mozilla like, kinda.
-
[!--quoteo(post=375242:date=May 19 2006, 04:06 PM:name=steelmanronald06)--][div class=\'quotetop\']QUOTE(steelmanronald06 @ May 19 2006, 04:06 PM) [snapback]375242[/snapback][/div][div class=\'quotemain\'][!--quotec--]
Alright. Fixed a few things while I had a bit of spare time before the meeting. The logo is clickable. I took out the login box and sized up the nav. There is now /login.php . However, what do I do with the login page? I stared at it for a while, just kinda looking at it, trying to think of something, but nothing came to mind. Any ideas on how to spice up that oh so empty page? Any ideas where I should move the login box instead?
[/quote]
Qui? -
The open source version of Maguma Studio - I use it and appears to have many of the features you have described. The cool thing is, when you type a function, a tooltip pops up explaining its use and acceptable parameters.
One annoying thing is, its not 100% stable, sometimes it decides to throw a tonne of error messages out at once, reload it and all is ok again. Doesn't happen ALL the time, just every so often. But as its open source, I would image it will get fixed soon. -
There is a class called SS_ZIP that I use for creating zip archives without any problems. You can find it [a href=\"http://www.smiledsoft.com/demos/phpzip/\" target=\"_blank\"]here[/a].
-
Ok, I came up with a workaround - I just used some CSS to recolour the scrollbar to the same as the background.
-
I'm opening a new window and require it to fill the entire screen, currently I have this:
[code]window.open('file.php','','fullscreen=yes,scrollbars=no');[/code]
I am getting a fullscreen window, but I still have an inactive vertical scrollbar - I just can't get rid of the thing! Does anyone have any suggestions?
NOTE: This is not for a live public application, I'm not trying to be annoying for the users - There are none! I'm wanting to display a project on a plasma screen without all the browser bits. -
There is a function reference over at php.net.
As for iterating through a string, you have the right idea - Just to expand on it though...
[code]<?php
$str = "The quick brown fox jumped over the lazy dog";
for($i=0; $i<strlen($str); $i++) {
echo $str[$i]."<br/>";
}
?>[/code] The above will go through the whole of $str and print each character on a new line...
[!--quoteo--][div class=\'quotetop\']QUOTE[/div][div class=\'quotemain\'][!--quotec--]I also need to construct a Select Case:[/quote] I don't understand what you mean, care to elaborate?
[b]EDIT:[/b] I just done a search on Google for Select Case, and it appears that its equivilent in PHP is the switch statement, used like this:
[code]<?php
$number = 1;
switch($number) {
case 0:
echo "Number is zero";
break;
case 1:
echo "Number is one";
break;
default :
echo "Number is not zero or one";
}
?>[/code] -
The command would be something like:
0 3 * * * /usr/local/bin/php /usr/home/*username*/public_html/script.php
The second part of the command "/usr/local/bin/php" points to the PHP install on the server, the script is run through the command line rather than through Apache. -
Is XMB open source, or do you have the source? If so, why not take a look at it and see how they have done it for some ideas...
-
[!--quoteo(post=374584:date=May 17 2006, 08:01 AM:name=samshel)--][div class=\'quotetop\']QUOTE(samshel @ May 17 2006, 08:01 AM) [snapback]374584[/snapback][/div][div class=\'quotemain\'][!--quotec--]
is it a bad idea to use function called
str_pad()
just was curious if it is what u need....
hth
[/quote]
Yes, that would be an easier solution - I knew I saw that function in the manual a while back, couldn't remember what it was called - After searching for it, and not finding it, I thought I imagined it! Hah!
PHP form data to Fax machine
in PHP Coding Help
Posted