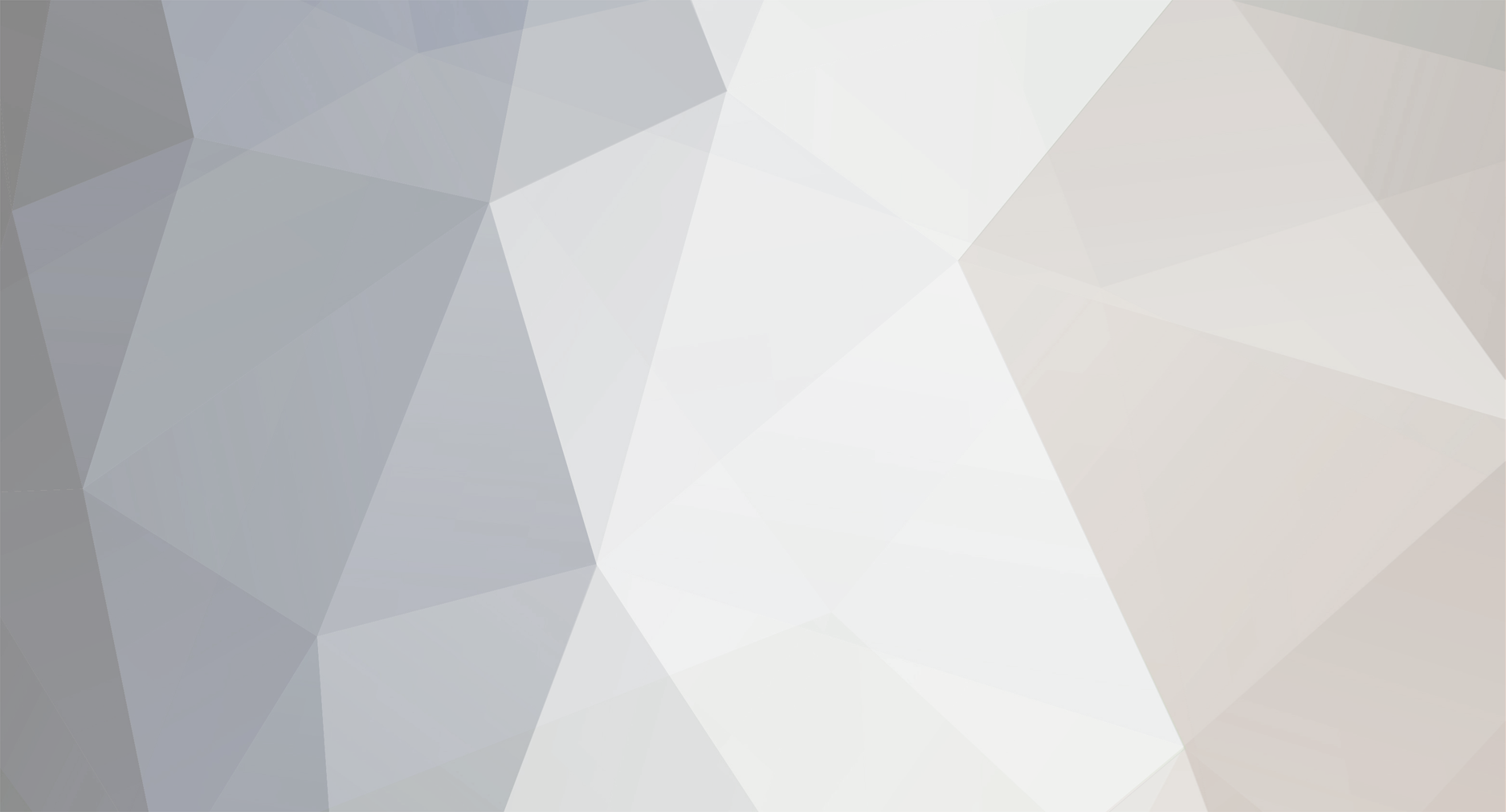
zq29
-
Posts
2,752 -
Joined
-
Last visited
-
Days Won
1
Posts posted by zq29
-
-
A possible alternative could use glob():
[code]<?php
$ext = array("txt","jpg");
$files = array();
foreach($ext as $e) {
foreach(glob("*.$e") as $filename) {
$files[] .= $filename;
}
}
?>[/code]
$files contains all of the .txt and .jpg filenames as an array. -
Post moved.
Please be advised that the PHP Help forum is for asking specific PHP scripting questions - Not for requesting 3rd party applications. -
[code]echo date("l jS \of F Y h:i:s A", $timestamp);[/code]Check the date() page in the manual for more information on how to format it.
-
[!--quoteo(post=368478:date=Apr 25 2006, 04:05 PM:name=ober)--][div class=\'quotetop\']QUOTE(ober @ Apr 25 2006, 04:05 PM) [snapback]368478[/snapback][/div][div class=\'quotemain\'][!--quotec--]
Comments, spacing, indents, and readable code. Variables should be descriptive and defined if they're meaning is not readily apparent. Classes should be well documented and snippets should explain complex loops and calls.
[/quote]
I'd pretty much agree with that - It really does annoy me when people don't follow those very simple concepts, especially the indents, without them it makes code a lot more difficult to read! -
I think you may be getting confused with JavaScript - Netscape originaly created JavaScript as far as I'm aware.
-
You [i]really[/i] need to slow down and actually [i]read[/i] peoples advice on here. You are still using the mail function incorrectly, heres an example of how it is used:
[code]<?php
$message = "This is the body of the Email";
mail("businessman332211@hotmail.com","The Subject", $message);
?>[/code] The above code will send an email to businessman332211@hotmail.com with the subject "The Subject", and the email will just contain "This is the body of the Email".
[b]EDIT: Turns out you got this bit working - Ignore the above then...[/b]
Form checkboxes require the name set as an array so that the user can select more than one checkbox, all of the checked boxes are put in the array, heres an example of using check boxes and displaying which ones were checked:
[code]<?php
if(isset($_POST['submit'])) {
echo "You selected the following colours:<br/>";
foreach($_POST['colours'] as $c) {
echo $c."<br/>";
}
}
?>
<h1>Select some colours</h1>
<form name="colour_select" action="" method="post">
<input type="checkbox" name="colours[]" value="Red"/> Red<br/>
<input type="checkbox" name="colours[]" value="Green"/> Green<br/>
<input type="checkbox" name="colours[]" value="Blue"/> Blue<br/>
<input type="submit" name="submit" value="Go!"/>
</form>[/code] -
You could try the GD function imagecopyresampled() - Check the manual, it gives examples on its use.
-
0xFE is hexadecimal for decimal 255 I think.
-
Please be aware that the PHP Help forum is for requesting help with specific PHP coding issues, not for requesting third party packages. Topic moved.
-
You haven't told us nothing about the module that you are using - Payment gateways usualy supply detailed documentation on how to use their systems and integrate them with your sites. Such documentation should be found on the companies website.
If you had your current site built for you, and you have no idea how it all works I think you are going to have a hard time integrating it all yourself, its probably going to be a lot easier to let your developers sort it out. -
Please don't post JavaScript questions in the PHP forums.
Moved. -
Thats a part of the IPB Forum software, looks like its done with JavaScript altering the styles on a div tag.
-
I believe its explained in the Classes and Objects section of the manual.
-
[!--quoteo(post=367321:date=Apr 21 2006, 10:14 PM:name=phrosty)--][div class=\'quotetop\']QUOTE(phrosty @ Apr 21 2006, 10:14 PM) [snapback]367321[/snapback][/div][div class=\'quotemain\'][!--quotec--]I have a question since I'm pretty new to PHP...does the above code check the URL address bar for the ?ABCDEFGH or does it check text in the code?
[/quote]
It checks everything after the first "?" in the URL. -
Generally, the page has to be reloaded in order to take input from a field and put it into a variable:
[code]<?php
if(isset($_POST['name'])) {
$name = $_POST['name'];
echo "Your name is $name!";
}
?>
<form name="name" action="" method="post">
<input type="text" name="name"/>
<input type="submit" name="submit" value="GO!"/>
</form>[/code]
With regards to session variables, I think they are destroyed when the browser is closed, although you could clear the session data with something like $_SESSION = array(); -
A mixture of Photoshop and HTML?
-
You shouldn't have to change any settings for this to work - Mine is set-up pretty much default. Maybe you are running an older version of PHP4? Searching for "Unable to fork" on Google turns up quite a few resources on the error - Maybe you could solve it better then?
Or maybe try out the system() command instead of exec() - You should just be able to swap the word exec for system, they both except the same paramiters. -
There are some limited scripts [a href=\"http://www.weather.com/services/oap.html?from=footer&ref=/\" target=\"_blank\"]here at weather.com[/a] that let you display a templated thing on your site with weather reports - Maybe you could dump it into a variable and extract what you need that way?
-
Just tested this on my WinXP Pro box and works ok - Bare in mind I have a processor with hyperthreading enabled so the output index might be different for the available memory compared to the machine you may run it on - If it is incorrect, run print_r($output) to get the correct index.
[code]<?php
exec("systeminfo",$output)
echo $output[26];
?>[/code]
Hope this helps.
NOTE: I have left the semi-colon off of the end of the exec() line as it appears I can't post with it there - Looks like a forum bug... -
Oh! Must have got my wires crossed somewhere then - My appologies.
-
I think you should be using mysql_close() when opening persistent connections with mysql_pconnect() as I don't [i]think[/i] they close themself...
-
Sounds like that should be sufficient, thanks for that!
-
Thats quite interesting, does that only happen on the site you are building, or any site that you happen to visit with the AOL browser?
As a fix, I guess you could run something this?:[code]<?php
foreach($_GET as $key => $val) {
$_GET[$key] = str_replace("?ABCDEFGH","",$val);
}
?>[/code] -
I have a script where I allow a user to add, edit or delete information from a database. I normally have three seperate sets of pages for each function, i.e. the form on one page, and the processing on another. In an attempt to make things cleaner I am integrating all of the scripts into one. My only problem is, if a user should decide to click refresh (for what ever reason) after perviously adding some info to the database, the info is added again, and again on any further refresh. Does anyone know of a method to prevent this, maybe by clearing the variables from the browser cache or something? - I don't know how this works technically at a browser level...
Heres a demo script to give you an idea of the kinda script I have:
[code]<?php
if(isset($_POST['submit'])) {
mysql_query("INSERT INTO `table` (`name`) VALUES ('$_POST[name]')");
}
?>
<form name="add" action="" method="post">
<input type="text" name="name"/>
<input type="submit" name="submit" value="Insert"/>
</form>[/code]
timestamp
in PHP Coding Help
Posted
[code]<?php
$str = "20060426152138";
$year = substr($str,0,4);
$month = substr($str,4,2);
$day = substr($str,6,2);
$hour = substr($str,8,2);
$minute = substr($str,10,2);
$second = substr($str,12,2);
?>[/code]