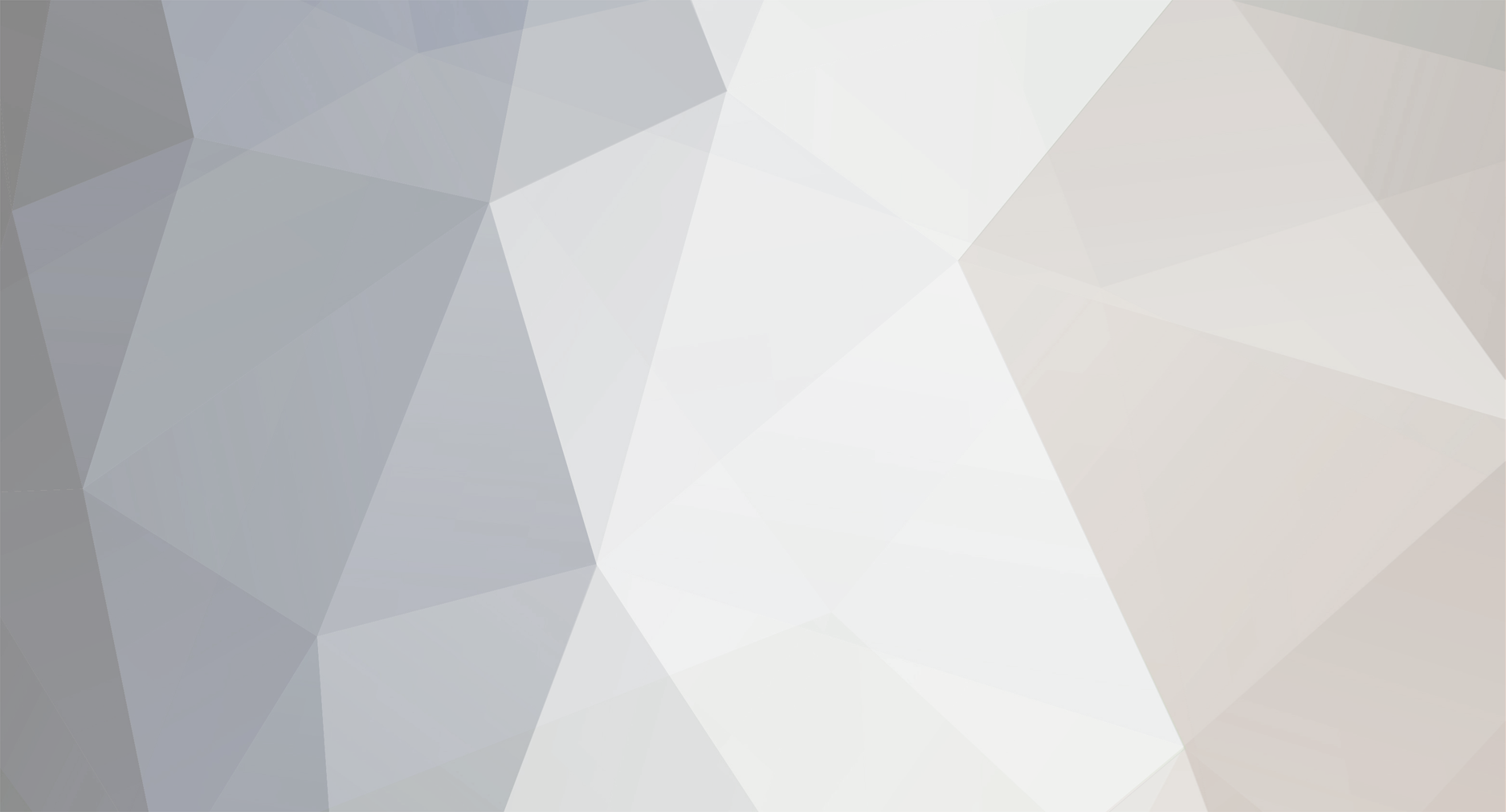
zq29
-
Posts
2,752 -
Joined
-
Last visited
-
Days Won
1
Posts posted by zq29
-
-
You could check that a user with authority is actually logged in before proceding to delete anything.
-
If you open the file with $handle = fopen("file.txt", "wb") it should place the file pointer at the beginning of the file ready for fwrite() to write to it at that pointer. I [i]think[/i]!
-
I haven't read through your code, but this is how you do it, shouldn't be too hard to understand and integrate into your code:
[code]<?php
$orderby = (isset($_GET['orderby'])) ? $_GET['orderby'] : "name";
$ascdesc = (isset($_GET['ascdesc'])) ? $_GET['ascdesc'] : "ASC";
$result = mysql_query("SELECT * FROM `table` ORDER BY $orderby $ascdesc") or die(mysql_error());
//Loop through results etc...
echo "<a href='?orderby=age&ascdesc=DESC'>ORDER BY DESCENDING AGE</a>";
echo "<a href='?orderby=name&ascdesc=DESC'>ORDER BY DESCENDING NAME</a>";
?>[/code] -
This method isn't properly recursive, but it will get what you want I think... [code]<?php
$handle = opendir("home/user1/cat1/");
while (false !== ($file = readdir($handle))) {
echo "$file<br/>";
if(!strpos($file,".")) {
echo "<ul>";
foreach(glob("home/user1/cat1/$file/*.*") as $content) {
echo "<li>$content</li>";
}
echo "</ul>";
}
}
closedir($handle);
?>[/code]
Untested, so you might need to tweak it a bit, but is that the kinda thing you wanted to do? -
You might be able to do it with money_format() - But other than that, I don't think there is one.
-
There is a function called money_format() that should be able to do what you want, although in the PHP manual it says that it is available in PHP versions >= 4.3.0. I'm running 4.3.11 on my development machine and it does not recognise the function, some others are having the same issue by reading the comments section on the manual page.
If you can get it to work, great, if not, heres a shorter way of doing it than you currently have... [code]<?php
function ten($number) {
$length = strlen($number);
if($length < 10) {
for($i=0; $i<(10-$length); $i++) {
$number = "0".$number;
}
}
return $number;
}
echo ten(54782); //Prints 0000054782
?>[/code] -
You can fetch all of a tables data and loop through it with something like: [code]<?php
$result = mysql_query("SELECT * FROM `table`");
while($row = mysql_fetch_assoc($result)) {
//Echo your columns here with $row['name'] to print the column 'name'
}
?>[/code]
You won't be able to do your second question as easily with PHP - Everything is processed on the server before it is passed to the users browser. You'll have to pass a count to and from your page to keep track of cycles and clicks. -
How is the category information stored in your database? (How is it structured?)
-
I don't understand why, or how, you want to use arrays to do this, but to create the rows of fields you can just use a simple for() like so: [code]<?php
$num = 4;
for($i=0; $i<$num; $i++) {
echo "<input type='text' name='field_a_$i'/>
echo "<input type='text' name='field_b_$i'/>
echo "<input type='text' name='field_c_$i'/>
echo "<input type='text' name='field_d_$i'/>
echo "<input type='text' name='field_e_$i'/>
echo "<input type='text' name='field_f_$i'/>";
}
?>[/code] At this point, you could pass $i as a hidden field to the next script so that you know how many rows you need to loop through. Then its just a case of looping through them: [code]<?php
$num = $_POST['num'];
for($i=0; $i<$num; $i++) {
mysql_query("INSERT INTO `table` (`field_a`,`field_b`....) VALUES ('$_POST[field_a_$i]','$_POST[field_b_$i]'....)") or die(mysql_error());
}
?>[/code] That should be enough of an idea to get you on your way. -
Are you experienced in another coding/scripting language then? Because OOP is [i]not[/i] a basic coding practice.
A good idea when troubleshooting is to print selected variables to the screen so that you can see what data is being passed around. -
[code]<?php
if (CCGetSession("username")) {
$shoppingcart->username->SetValue($username);
} else {
$stamp = strtotime("now");
$username = $stamp.$REMOTE_ADDR;
$username = str_replace(".", "", "$username");
$shoppingcart->username->SetValue($username);
CCSetSession("username", $username);
}
?>[/code] -
In your Apache config file - httpd.conf - Check the 'DirectoryIndex' is pointing to index.php as well as index.html
-
This should work...
[code]<?php
function return_date($day,$year=2006) {
$stamp = mktime(0,0,0,1,$day,$year);
$date = date("jS F Y",$stamp);
return $date;
}
echo return_date(134);
?>[/code] -
This should do it...
[code]$number = number_format($number,2);[/code] -
[!--quoteo(post=373148:date=May 11 2006, 02:14 AM:name=thorpe)--][div class=\'quotetop\']QUOTE(thorpe @ May 11 2006, 02:14 AM) [snapback]373148[/snapback][/div][div class=\'quotemain\'][!--quotec--]
Ah cool. So... we all have them, but can only see our own unless we are a mod etc. Cool. Bit paranoid.... never noticed it before. ( and I know I can get a little hot headed at times)
[/quote]
You've never noticed it before as that feature was only switched on the other day... -
I don't know of any legends for this, but whenever I get an error message that doesn't make much sense to me, I just drop it into Google, somewhere, someone has had the same error and has found out what it means - if it isn't obvious ofcourse.
-
Thats strange, str_replace("\"", "", $comment) should work fine, as long as the middle quotation mark is escaped...
-
Not sure if I have gone about this the long way, but this is the idea that first popped into my head...
[code]<?php
$needle = "fox";
$haystack = "The quick brown fox jumps over the lazy dog - What a great fox he is!";
$replace = "elephant";
if(strpos($haystack,$needle)) {
$pos = strpos($haystack,$needle);
$nlen = strlen($needle);
$tlen = strlen($haystack);
$newhaystack = substr($haystack,0,$pos).$replace.substr($haystack,($pos+$nlen),$tlen);
echo $newhaystack;
} else {
echo "$needle not found in $haystack.";
}
?>[/code] -
I'm quite sure there are registry enteries for those menu items - Can't remember exactly where they are though. Google should be able to help you out I would have thought.
-
You could write a PHP script to generate them, depends your PHP skill level and on their complexity though I guess, if you want them 3D looking and stuff - would be a pain in the arse getting the shadows and curvature of the lettering right. Flat 2D balls should be faurly simple though.
-
The last thing you tried: [code]$fixcomment = str_replace("\"", "", $comment);[/code] That should work...
-
I think it might be difficult to do this without having a file for each page in each language, because as far as the computer knows, they're all different files regardless if it is the same content be in different languages. You can either store all of your content in their own files, or in a database and pull the correct language out.
An alternate solution may be to have one copy of each bit of content in a database and dynamicaly submit it to an online translation service and pull the result back and display it. But as we all know, they don't do the best jobs on translation. -
You could use preg_match(). Check [a href=\"http://www.regularexpressions.info\" target=\"_blank\"]http://www.regularexpressions.info[/a] for help with regex.
-
You won't be doing that with just PHP, I can tell you that much. You'll need to use a client side language to do the closing of the window - JavaScript?
Upload an entire folder?
in PHP Coding Help
Posted
I'm no expert in JavaScript (I'm still picking it up as and when I need it), but does it have file functions? If so, maybe you could use them to select the directory and dynamically create a some pre-filled file input boxes in a hidden div or something?
Whatever method you choose, you must take maximum file size limits into consideration with PHP, as I think the default limit is set at either 2MiB or 8MiB, unless you have the power to alter this on your server.