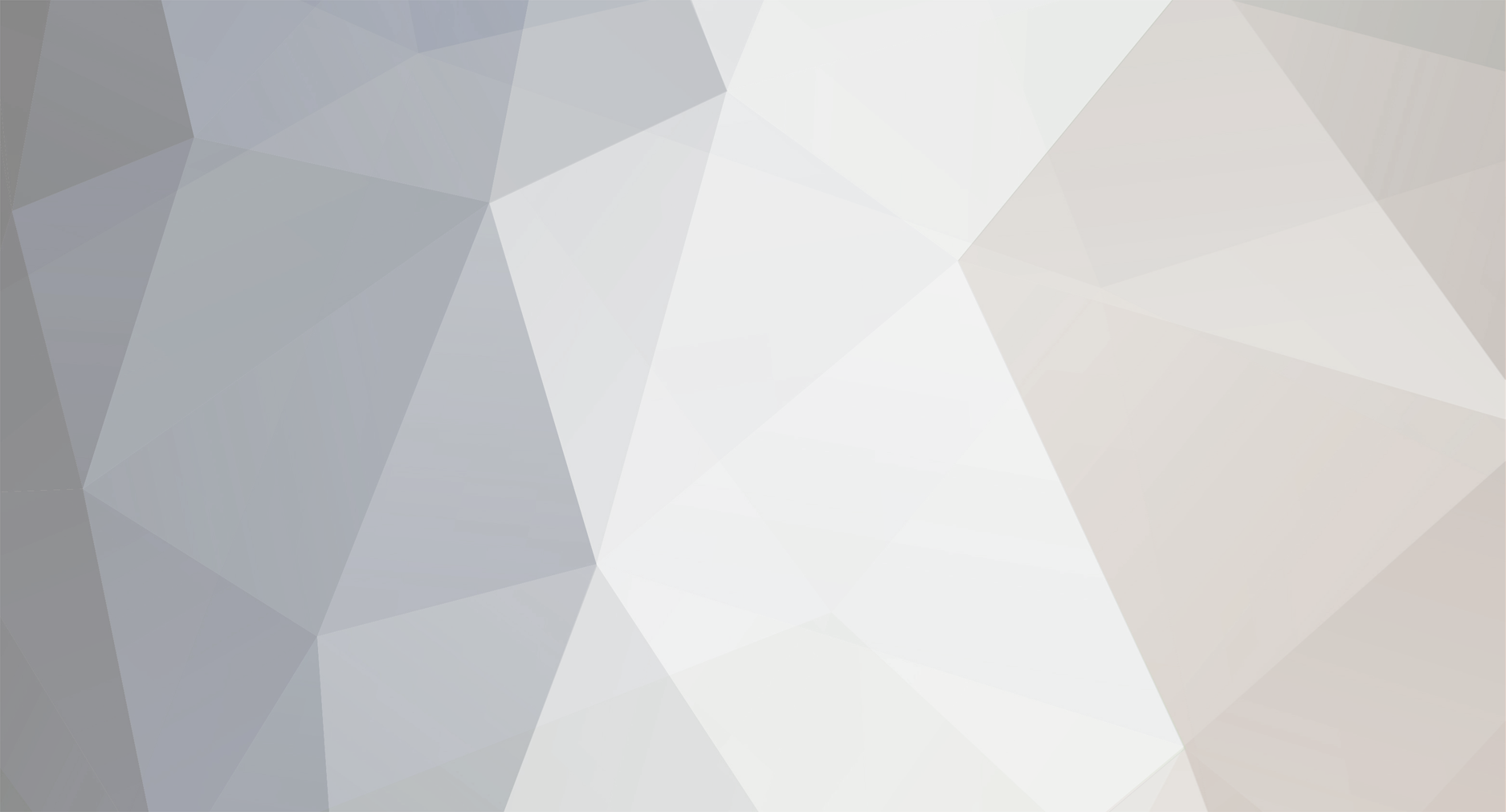
denno020
Members-
Posts
761 -
Joined
-
Last visited
-
Days Won
3
denno020 last won the day on May 3 2019
denno020 had the most liked content!
About denno020
- Birthday 02/22/1987
Profile Information
-
Gender
Male
-
Location
Australia
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
denno020's Achievements
-
The issue you're running in to is one of timing. The fact that you can see the toastr at all is very surprising, and likely because there aren't any other actions being performed on click.. As soon as there are some actions being performed within the click handler, then the toastr likely won't ever be seen! Unless of course, we fix it! Essentially we need to tell the browser to stop processing the click event so the page refresh doesn't occur Something like this will get you going: <script type="text/javascript"> $(document).ready(function () { $('#add').click(function (e) { e.preventDefault(); // Stop the browser from redirecting to click target (i.e. the href) // Perform actions // ... // ... toastr.success("Folder 2 has been clicked!", "Folder 2", ); window.setTimeout(function () { toastr.clear(); }, 3000); }); }); </script>
-
I completely agreed with @requinix, this is a situation where jQuery excels, and will save you a lot of time and headache. It's definitely possible to do this with vanilla JS, but it's a lot more code. One suggestion I will make is to use a `<template>` element, which can contain the HTML that you will clone. This way you don't have to clone an existing HTML DOM node, which will contain user changes and would require you to go through each field and clear the values of the inputs/textarea before injecting it into the page as a new node. Check out the MDN docs for `template`, they have great example code (which is actually in vanilla JS, so you can see how much is required!) Another thing I would suggest is to avoid using a `div` as a button. The New Line "button" has the `btn` class on it, but it's a div. This will be inaccessible to site visitors, so best to use a `button` element, with those same classes. P.s. Please excuse the use of back ticks, it appears the forum doesn't support markdown
-
PHP search function only searches one column
denno020 replied to Adamhumbug's topic in Javascript Help
The offending line that is only allowing you to search the first column is this one: td = tr[i].getElementsByTagName("td")[0]; The `[0]` portion of that statement means to literally get the first column only. An easy fix is to add a nested loop, that does essentially the same as the for loop for the `tr` element, but do it for `td`, provided `td` is assigned: td = tr[i].getElementsByTagName("td"); Simply move the if block inside that inner loop, and it should work (some variable names inside that second for loop will need to be updated) As for making this work with any table, I feel like the call to the function needs to pass a string. Without the quotes around customerTable, the code is going to assume that's a variable name, so maybe try updating to: onkeyup='searchTable("customerTableSearch", "customerTable")' These are all suggestions that require a minimal amount of refactoring of the current code, this doesn't mean I endorse that way it's written , there's lots of improvements that could be made for this to be much more maintainable in the future, but we'll leave that for another discussion -
I have never used Audio previously, but looking at the docs, audio.load() will reload the file, which means you should be able to omit it here. Also, the event to listen for is "loadeddata", rather than "load".. See how those updates go
-
Triggering The Same Function With Some Delay Between Each Run
denno020 replied to phdphd's topic in Javascript Help
You're going to want to make use of setTimeout. The syntax is as follows: setTimeout(function() { // Execute this code after the timeout of 1000ms }, 1000); Keep in mind that this function is asynchronous, which means $(document).ready(function(){ setTimeout(function() { intro('#hello', '#world'); }, 1000); setTimeout(function() { intro('#hi', '#all'); }, 1000); setTimeout(function() { intro('#hola', '#amigos'); }, 1000); }); Will execute all into functions after 1 second. This could be a solution: $(document).ready(function(){ setTimeout(function() { intro('#hello', '#world'); }, 1000); setTimeout(function() { intro('#hi', '#all'); }, 2000); setTimeout(function() { intro('#hola', '#amigos'); }, 3000); }); However that will quickly fail if the execution of the next function has to be 1 second after the previous function has finished (assuming there might be some delays/user input/processing inside the intro function) Hopefully that's enough information to get you on the right track to figuring it out -
Including content of JS file into gulpfile.js
denno020 posted a topic in Other Programming Languages
I'm having trouble figuring out how to import the contents of one JS file into another JS file - a gulpfile. I've got my gulp file set up and I would like to store an array of file names in a separate JS file, that the gulpfile will load in and pass as the parameter to the src command. The array in the separate JS file will be sort of like configuration. But it will be something that will change, and I don't like the idea of constantly editing an array inside my gulpfile To illustrate what I'm after, this is the folder structure I would have //directory root // - gulpfile.js // - arrayfile.js Then my files would look something like this //arrayfile.js var array = [ 'somefile.js', 'someotherfile.js' ]; //gulpfile.js import arrayfile.js //This is the part that I need to figure out properly gulp.task('javascript', () => { gulp.src(array) //where 'array' comes from arrayfile.js .pipe() //etc }); As you can see, I'm using ES2015, however I haven't been able to get import to work with gulp. What else could I do to be able to use the array defined in arrayfile.js as the src parameter in the gulpfile? The versions I'm using: Gulp cli: 1.2.1 Gulp local version: 3.9.1 Node: 6.2.1 Thanks, Denno -
dynamically loaded events are not working properly
denno020 replied to shan2batman's topic in Javascript Help
I think your best bet would be to add this to your CSS: .hidden_textarea, .hidden_edit_4_session, .hidden_edit_4_friend { display: none; } Then in your javascript, when you actually want to show something, you toggle the class on that element $('#element-i-want-to-toggle-visibility-of').toggleClass('hidden_textarea'); That will mean that any dynamically loaded data will be hidden by default, and only show once your code triggers it to be shown. However, if you want to keep your implementation, then all you would need to do is add $(".hidden_textarea").hide(); $(".hidden_edit_4_session").hide(); $(".hidden_edit_4_friend").hide(); to the end of your $.post(); callback function, like so $.post("st&com.php", {load:load},function(data){ $("#status_area").append(data); $(".hidden_textarea").hide(); $(".hidden_edit_4_session").hide(); $(".hidden_edit_4_friend").hide(); }); -
how to add a tinymce editor to a dynamically added textarea?
denno020 replied to shan2batman's topic in Javascript Help
You need to re-initialise TinyMCE once the AJAX (I assume) response has come back from the server and you're finished putting the data into the DOM. You will also want to fix up the ID, because you're going to have multiple <textarea> elements on the page with the same ID, which is invalid HTML, and could also mess with the initialisation of TinyMCE -
Integration of PHP Application to Credit Card and Paypall System
denno020 replied to DigiMartKe's topic in PHP Coding Help
Look for tutorials using Paypal IPN. It's a tricky concept at first, but if you can get your head around it, you'll be able to use it to handle payments from Paypal accounts, and payments from credit cards (using the Paypal site as a checkout)- 2 replies
-
- php
- creditcard
-
(and 1 more)
Tagged with:
-
Can 2 seperate forms write to the same csv file?
denno020 replied to sulee154151's topic in PHP Coding Help
Also, CSV's don't have "tabs", that's only a feature of spreadsheets. The fact that a CSV file opens up in your spreadsheet program is probably what's throwing you off here.. -
You could use CSS to get the same functionality. Add the mobile only content to a div, give it a class, and then in your stylesheet, set display: none; on that div, with a style directly after inside a media query, that will set display: inline; Example .mobileOnly { display: none; } @media (max-width: 300px) { .mobileOnly { display: inline; } } Set the max width to your desired "mobie only" size. Denno
-
PHP adding active to nav menu with dropdown
denno020 replied to alphamoment's topic in PHP Coding Help
A really crude but easy way is to do the exact same thing that you've done for determining which file to include: <?php $page = $_GET['page']; ?> <li<?php if($page == "home") { ?> class="active"<?php } ?>> <a href="?page=home">Home</a> </li> Note where the php tags open and close. You'll just do that for each link, with the appropriate checks for each Denno Edit: Actually I don't know if that would work the way I've written it.. It's been ages since I've done inline php like that.. You may have to do it like this: <li<?php if($page == "home") { echo ' class="active"'; } ?>> -
What error do you get? What is the response data from the server?
-
If you want to use flexbox: div { display: flex; align-items: center; } More info on flexbox: https://css-tricks.com/snippets/css/a-guide-to-flexbox/
-
I'm assuming that you meant to say "... then second magnific popup should not open till first is open closed." One option, when the document is loaded, as well as checking that #myModal exists, also check if the first popup is visible. If it is visible, then attach a click hander to the close button of that first popup, which will trigger the second popup to show. That will ensure the second popup will show directly after closing the first. Denno