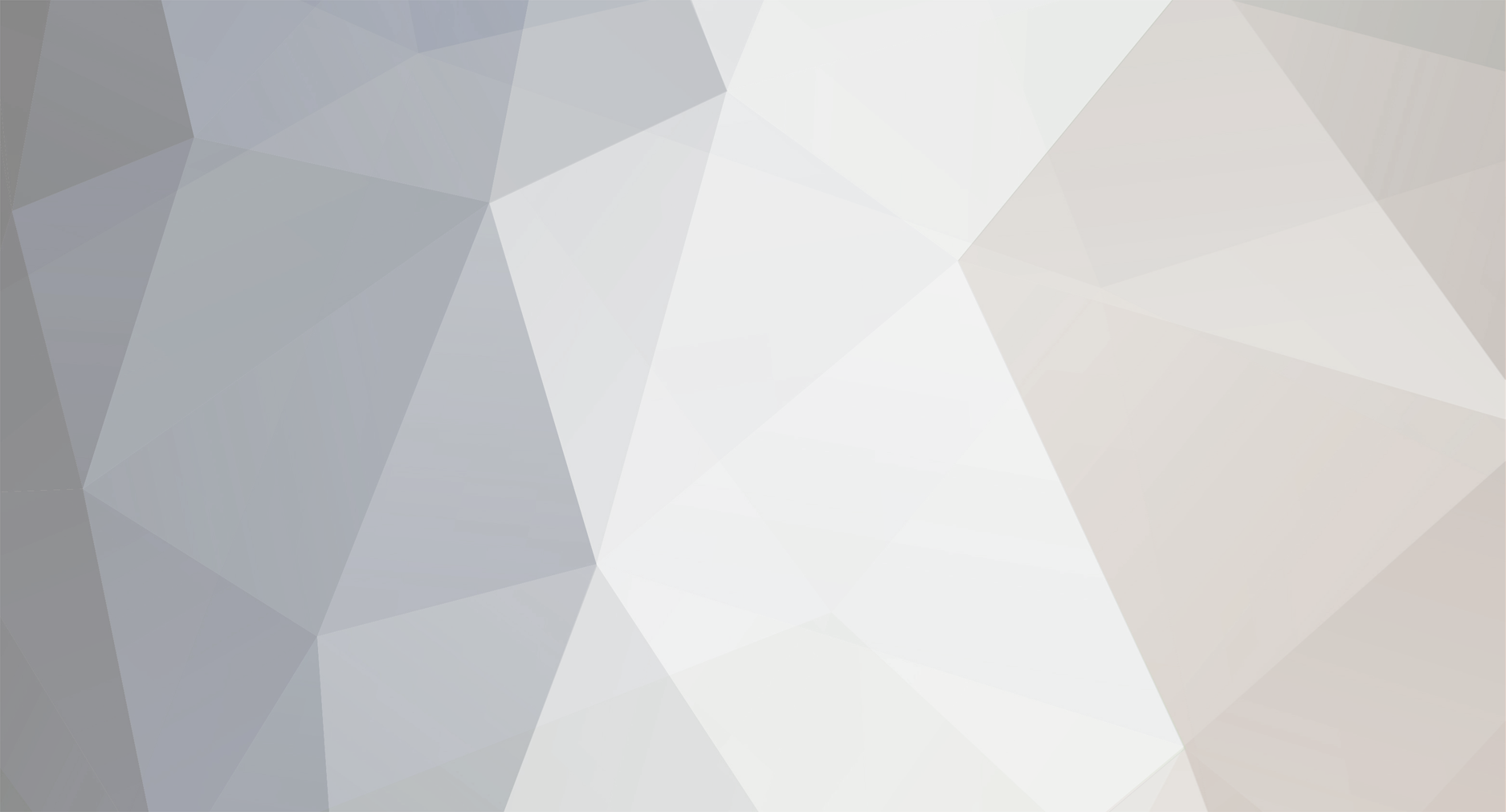
jazzman1
Staff Alumni-
Posts
2,713 -
Joined
-
Last visited
-
Days Won
12
Everything posted by jazzman1
-
A virtualization software and running some Linux distros, it would be great for you.
-
I see a lot of members here asking for solutions "How to upload multiple images to the fileserver and store some information into a database" So, I decided to provide mine, hope that helps someone. 1.First off, for uploading multiple images I'm using this API . You would download the free version created under GPLv2 licence. 2.Second, you need to create a database. To keep the things simple, I've been created a database with name - "images" and a table with name "img_tbl" with id and name columns. 3.Third, open up a file called - upload.php, find and add next lines of code: // Check if file has been uploaded if (!$chunks || $chunk == $chunks - 1) { // Strip the temp .part suffix off rename("{$filePath}.part", $filePath); // create your insert query statement $query = "INSERT INTO `images`.`img_tbl` VALUES(NULL,'$fileName')"; $result = mysql_query($query,$conn) or die(mysql_error()); $last_id = mysql_insert_id($conn); // if there is affected rows // proceed with next select statement if(mysql_affected_rows()) { // call select statement $query = "SELECT `name` FROM `images`.`img_tbl` WHERE id = ".$last_id; $result = mysql_query($query) or die(mysql_error()); // fetch a row by last inserted id $row = mysql_fetch_assoc($result); // call resize img photo resize_img($row['name']); } 4. Open up a new page and create your resize image function, save the file as resize_photo.php. This function will resized your original image to thumbnail. It's been created by the author of phpvideotutorials.com, few years ago. Check on the web and you will find much better solutions, I think. ** * The following four functions resizes the photos uploaded by a user * creates the thumbnail photo reproduction of the originally uploaded image * @param int $id * @return bool */ function resize_img($name) { // set original image location $img = './uploads/original/' . $name; // set our image canvas $canvas_width = 75; $canvas_height = 75; // create a blank canvas $canvas = imagecreatetruecolor($canvas_width, $canvas_height); // get width and height of original image list($img_width, $img_height) = getimagesize($img); $original = imagecreatefromjpeg($img); // copy original onto canvas imagecopyresampled($canvas, $original, 0, 0, (($img_width / 2 ) - ( ($canvas_width / 2) * 4 )), (($img_height / 2 ) - ( ($canvas_height / 2) * 4 )), $img_width / 4, $img_height / 4, $img_width, $img_height); if (imagejpeg($canvas, './uploads/thumbs/' . $name, 75)) { return true; } else { return false; } } 5. Create a new file where you will display your thumnails: display_thumbnails.php <?php include 'db_config.php'; $query = "SELECT `name` FROM `images`.`img_tbl` WHERE 1"; $result = mysql_query($query) or die(mysql_error()); $outputs = array(); while ($row = mysql_fetch_assoc($result)) { $outputs[] = $row; } foreach ($outputs as $output) { echo "<a href=./uploads/original/{$output['name']} style=margin-right:5px><img src=./uploads/thumbs/{$output['name']} /></a>"; } 6. Create a db_config.php file with your database credentials $conn = mysql_connect('db_host', 'db_name','db_pass') or die(mysql_error()); $db_name = mysql_select_db('images', $conn) or die(mysql_error()); 7. Inside the upload directory, create two new directories with names: original and thumbs 8. Include "db_config.php" and "resize_photo.php" to upload.php. Upload.php file should be similar like that: <?php include 'db_config.php'; include 'resize_photo.php'; /** * upload.php * * Copyright 2009, Moxiecode Systems AB * Released under GPL License. * * License: http://www.plupload.com/license * Contributing: http://www.plupload.com/contributing */ // HTTP headers for no cache etc header("Expires: Mon, 26 Jul 1997 05:00:00 GMT"); header("Last-Modified: " . gmdate("D, d M Y H:i:s") . " GMT"); header("Cache-Control: no-store, no-cache, must-revalidate"); header("Cache-Control: post-check=0, pre-check=0", false); header("Pragma: no-cache"); // Settings $targetDir = './uploads/original'; $cleanupTargetDir = true; // Remove old files $maxFileAge = 5 * 3600; // Temp file age in seconds // 5 minutes execution time set_time_limit(5 * 60); // Uncomment this one to fake upload time // usleep(5000); // Get parameters $chunk = isset($_REQUEST["chunk"]) ? intval($_REQUEST["chunk"]) : 0; $chunks = isset($_REQUEST["chunks"]) ? intval($_REQUEST["chunks"]) : 0; $fileName = isset($_REQUEST["name"]) ? $_REQUEST["name"] : ''; // Clean the fileName for security reasons $fileName = preg_replace('/[^\w\._]+/', '_', $fileName); // Make sure the fileName is unique but only if chunking is disabled if ($chunks < 2 && file_exists($targetDir . DIRECTORY_SEPARATOR . $fileName)) { $ext = strrpos($fileName, '.'); $fileName_a = substr($fileName, 0, $ext); $fileName_b = substr($fileName, $ext); $count = 1; while (file_exists($targetDir . DIRECTORY_SEPARATOR . $fileName_a . '_' . $count . $fileName_b)) $count++; $fileName = $fileName_a . '_' . $count . $fileName_b; } $filePath = $targetDir . DIRECTORY_SEPARATOR . $fileName; // Create target dir if (!file_exists($targetDir)) mkdir($targetDir); // Remove old temp files if ($cleanupTargetDir) { if (is_dir($targetDir) && ($dir = opendir($targetDir))) { while (($file = readdir($dir)) !== false) { $tmpfilePath = $targetDir . DIRECTORY_SEPARATOR . $file; // Remove temp file if it is older than the max age and is not the current file if (preg_match('/\.part$/', $file) && (filemtime($tmpfilePath) < time() - $maxFileAge) && ($tmpfilePath != "{$filePath}.part")) { unlink($tmpfilePath); } } closedir($dir); } else { die('{"jsonrpc" : "2.0", "error" : {"code": 100, "message": "Failed to open temp directory."}, "id" : "id"}'); } } // Look for the content type header if (isset($_SERVER["HTTP_CONTENT_TYPE"])) $contentType = $_SERVER["HTTP_CONTENT_TYPE"]; if (isset($_SERVER["CONTENT_TYPE"])) $contentType = $_SERVER["CONTENT_TYPE"]; // Handle non multipart uploads older WebKit versions didn't support multipart in HTML5 if (strpos($contentType, "multipart") !== false) { if (isset($_FILES['file']['tmp_name']) && is_uploaded_file($_FILES['file']['tmp_name'])) { // Open temp file $out = fopen("{$filePath}.part", $chunk == 0 ? "wb" : "ab"); if ($out) { // Read binary input stream and append it to temp file $in = fopen($_FILES['file']['tmp_name'], "rb"); if ($in) { while ($buff = fread($in, 4096)) fwrite($out, $buff); } else die('{"jsonrpc" : "2.0", "error" : {"code": 101, "message": "Failed to open input stream."}, "id" : "id"}'); fclose($in); fclose($out); unlink($_FILES['file']['tmp_name']); } else die('{"jsonrpc" : "2.0", "error" : {"code": 102, "message": "Failed to open output stream."}, "id" : "id"}'); } else die('{"jsonrpc" : "2.0", "error" : {"code": 103, "message": "Failed to move uploaded file."}, "id" : "id"}'); } else { // Open temp file $out = fopen("{$filePath}.part", $chunk == 0 ? "wb" : "ab"); if ($out) { // Read binary input stream and append it to temp file $in = fopen("php://input", "rb"); if ($in) { while ($buff = fread($in, 4096)) fwrite($out, $buff); } else die('{"jsonrpc" : "2.0", "error" : {"code": 101, "message": "Failed to open input stream."}, "id" : "id"}'); fclose($in); fclose($out); } else die('{"jsonrpc" : "2.0", "error" : {"code": 102, "message": "Failed to open output stream."}, "id" : "id"}'); } // Check if file has been uploaded if (!$chunks || $chunk == $chunks - 1) { // Strip the temp .part suffix off rename("{$filePath}.part", $filePath); // create your insert query statement $query = "INSERT INTO `images`.`img_tbl` VALUES(NULL,'$fileName')"; $result = mysql_query($query,$conn) or die(mysql_error()); $last_id = mysql_insert_id($conn); // if there is affected rows // proceed with next select statement if(mysql_affected_rows()) { // call select statement $query = "SELECT `name` FROM `images`.`img_tbl` WHERE id = ".$last_id; $result = mysql_query($query) or die(mysql_error()); // fetch a row by last inserted id $row = mysql_fetch_assoc($result); // call resize img photo resize_img($row['name']); } } In this example, I'm using the mysql library just for the test. You should avoid it, just create the same app using mysqli or PDO! You can also validate every sql insert value and make your app much more security, but just not a purpose here. Here it is!
- 1 reply
-
- upload images
- php
-
(and 3 more)
Tagged with:
-
In the future when you're posting your samples, please use code tags! So, fill and submit the form and give me the output string of $email_message. Add next line of code before to call a mail php function. ......................................................................................... // create email headers $headers = 'From: '.$email_from."\r\n". 'Reply-To: '.$email_from."\r\n" . 'X-Mailer: PHP/' . phpversion(); // add this echo '<pre>'.print_r($email_message, true).'</pre>'; exit; // use this instead yours if(mail($email_to, $email_subject, $email_message, $headers)) { echo 'Ok......message sent'; } else { echo 'Ok......message failed'; } } Also, turn on error_reporting on the top of the send_mail_form file.
-
PHP unzip compressed file and unzip tar.gz file
jazzman1 replied to rkrause54's topic in PHP Coding Help
Create a function that searches all archive files into this directory. Then make comparison. If the file extension is "tar.gz" use one method if a "zip" other. It's simple! -
In fact, there is a ton of tutorials on the web. Google -"Ajax/jQuery/PHP"
-
Sometimes emails with attached mp3's play, sometimes they don't
jazzman1 replied to njdubois's topic in PHP Coding Help
For file attachment I'd recommend to use SwiftMailer. Never had problems with godaddy and swiftmailer. -
And....your HTML form is?
-
No! Your application uses links like: http://www.grabkart.com/productdetail.php?prodid=9449 If you try to rewrite that link to "http://www.grabkart.com/prodid/9449" , this link won't be real for your app. Re-read my reply #25.
- 45 replies
-
- apache
- mod_rewrite
-
(and 1 more)
Tagged with:
-
When they enabled a SSH connection for you try to run your php script. If the problem is still there, you would run as @kicken said above cURL by shell terminal. It would be someting like: curl --verbose --output 'remote_style.css' 'http://static.trainingdragon.co.uk/assets/css/style.css'
-
To check that mod_rewrite is enabled to your domain (I'm pretty sure it is) , create .htaccess and display.php files inside web root or your current working directory. .htaccess RewriteEngine On RewriteRule ^.*$ display.php display.php <?php print 'mod_rewrite works fine!'; ?> Every request like (domainName/index.php) should be redirected to display.php file!
- 45 replies
-
- apache
- mod_rewrite
-
(and 1 more)
Tagged with:
-
You cannot use a copy function to do that. Instead of it you should use move_uploaded_file(). Replace: $copy = copy($_FILES['Filedata']['tmp_name'], $targetpath); to $tmp_name = move_uploaded_file($_FILES['Filedata']['tmp_name'], $targetpath); Also, turn on error_reporting on the very top of the page! http://php.net/manual/en/function.error-reporting.php http://php.net/manual/en/function.move-uploaded-file.php
-
Hey samnester, before to provide a solution about your topic, I have few questions. 1. Why do you want to save a binary data (images) into the database instead in the filesystem? Or....I'm wrong? 2. Who will have permission to upload and later on to comment these photos? Every users or only those who are already registrated on the system? 3. Can you explain me in clear English, what do you expect to do the columns - initiator | file | gallery | ....and why do you have duplicated columns inside comment table. In general, try to describe the whole idea.
-
Not according nmap, port 22, 80 are opened from an external network. Also, I tried to run the script through bash shell and got a correct result. The problem is inside your php script, especially this line - curl_setopt($ch, CURLOPT_NOBODY, true); Never used "cURL" in php but try to run next script that I created for you: <?php $url = 'http://static.trainingdragon.co.uk/assets/css/style.css'; // Create a curl handle to a non-existing location $ch = curl_init($url); //curl_setopt($ch, CURLOPT_NOBODY, true); curl_setopt($ch, CURLOPT_FILETIME, true); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $out = curl_exec($ch); if($out === false) { echo 'Curl error: ' . curl_error($ch); } else { $fp = fopen('css/style.css', 'w'); fwrite($fp, $out); } // Close handle curl_close($ch);
-
Wow......now get to work! "Google" would be your friend next hours Good spotting scope mac!
-
@Lawlssbatmbl, are you a big microsoft fan? I've never seen such beautiful backslashes in my life Irrelevant reply!
-
How the server knows that this user is on the session?
-
No, you're using sessions. Go to php.net and check out how to..... Try next: main.php <?php session_start(); $query = mysql_query("SELECT * FROM sport") or die(mysql_error()); $outputs = array(); while($row = mysql_fetch_array($query)) { $outputs[] = array($row['sport_ime'],$row['sezona']); } $_SESSION['output']=$outputs; // and then redirect back to html where it should echo the stuff header('Location:../view/vnos.php',true,303); exit; vnos.php <?php session_start(); echo '<pre>'.print_r($_SESSION['output'], true).'</pre>'; You should be consider how to use a session_start() and how to destroy that session. Go to php.net and come back again! EDIT: session_start() should be on very top of the page! I've made a typo -> $outputs[] = array($row['sport_ime'],$row['sezona']);
-
How did you echo it? Can I see the code? Also show us the result of: // dump the data echo '<pre>'.print_r($_SESSION, true).'</pre>';
-
Fetch all rows and push it inside an associative array. Try, $query = mysql_query("SELECT * FROM sport"); $_SESSION = array(); while($row = mysql_fetch_array($query)) { $_SESSION[] = array($row['sport_ime'],$row['sezona']); } // dump the data echo '<pre>'.print_r($_SESSION, true).'</pre>'; Or....for best performance use mysql_fetch_assoc() function. while($row = mysql_fetch_assoc($query)) { $_SESSION[] = array($row['sport_ime'],$row['sezona']); }
-
unserialize within a foreach within a while loop
jazzman1 replied to showme48's topic in PHP Coding Help
Yes, I know Misread it -
Change the encoding to utf-8. EDIT: If you don't want to change the encoding (I like UTF8), try to set a html base tag inside the head tag. (not tested): <base href="http://www.shugo.nl"> <link rel="stylesheet" type="text/css" href="../look/guidestyle.css">
-
unserialize within a foreach within a while loop
jazzman1 replied to showme48's topic in PHP Coding Help
Change: if($gamenames[$j]==$offerid){echo(" CHECKED") TO ($gamenames[$j]==$offerid) ? ' CHECKED' : NULL;