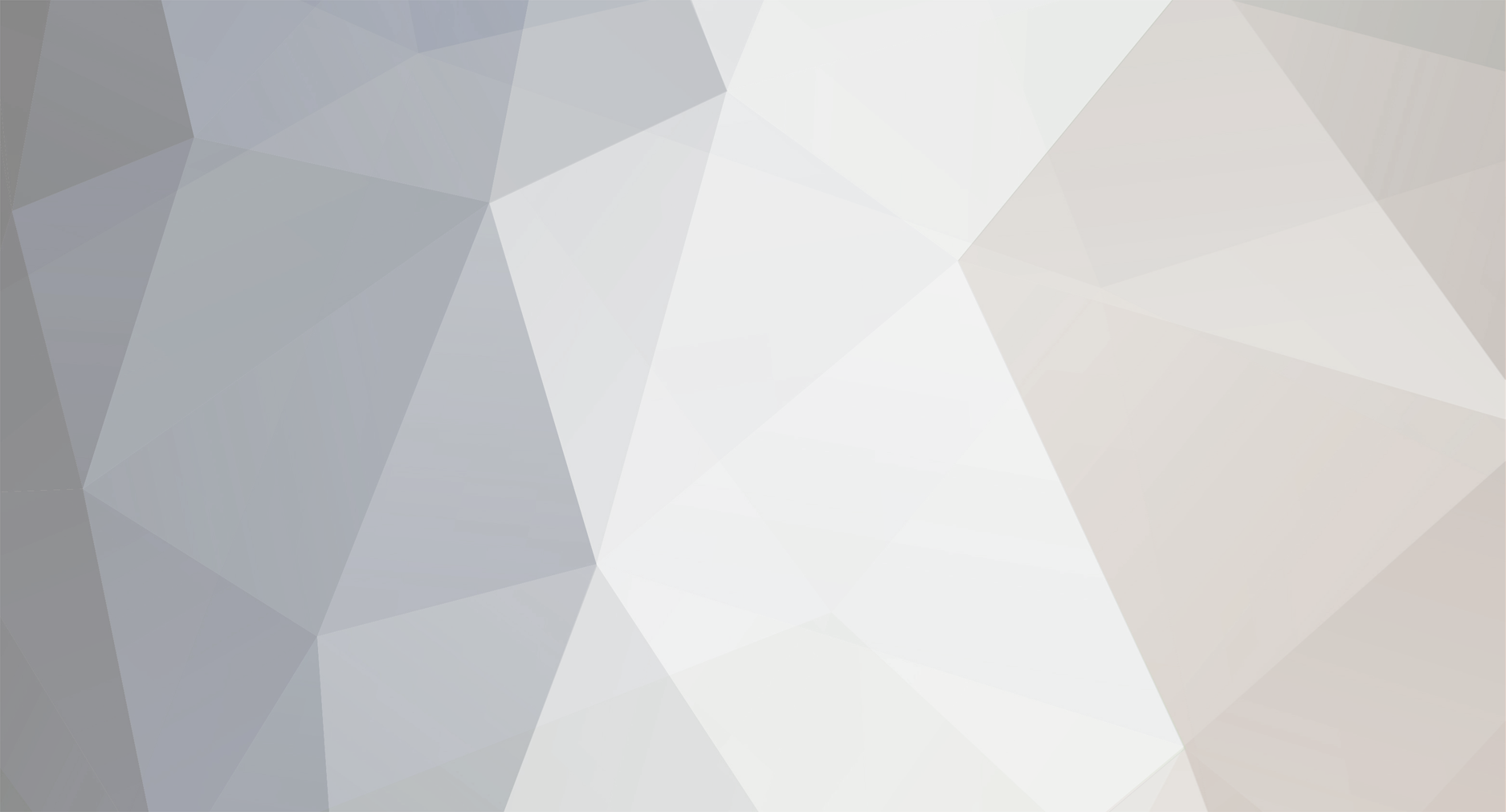
jazzman1
Staff Alumni-
Posts
2,713 -
Joined
-
Last visited
-
Days Won
12
Everything posted by jazzman1
-
Just for test, get rid addslashes() off of your query string. Run this: <?php $db_server = mysql_connect('localhost','userName', 'userPass') or die(mysql_error()); mysql_select_db('cms') or die("Unable to select database: " . mysql_error()); $query = "UPDATE `pagina` SET `title` = '".$title."', `korting`='".$korting."', `volg`='".$volg."', `product` = '".$product."',`product_id`='".$product_id."', `visible` = '".$visible."', `metadescription` = '".$metadescription."', `url` = '".$url."', `content` = '".$content."', `headerpicture`='".$headerpicture."',`picture`='".$picture."', `form_id` = '".$form_id."',`related`='".$related_string."', `datum` = NOW() WHERE `id`=$id"; $result = mysql_query($query) or die(mysql_error()); $output = array(); while ($row = mysql_fetch_assoc($result)) { $output[] = $row; } echo '<pre>'.print_r($output, true).'</pre>';
-
Can I see how you connect to the database and set the table name?
-
Does the name of the database is the same in your first post?
-
Hm....it looks just fine. Now, try this: $query = "SELECT LENGTH(COLUMN_NAME) FROM INFORMATION_SCHEMA.COLUMNS WHERE TABLE_SCHEMA='DB_NAME' AND TABLE_NAME='pagina' AND column_name ='title'";
-
PHP Parse error: syntax error, unexpected T_VARIABLE
jazzman1 replied to phpnewbieca's topic in PHP Coding Help
I think the double quotes are not corrrect. Try, $handle = fopen($myfile, 'a+') or die("Couldn't open $myfile"); OR $handle = fopen($myfile, "a+") or die("Couldn't open $myfile"); -
Of course, you can made the tests on the local machine. If the version of your apache server is not the same like remote hosting maybe some part of the script and you need to check the manual of apache web site. So, to be more spesific why your own test doesn't work, you should provide us all scripts and the structure of the directories on apache server as well.
-
Try, $query = "SELECT LENGTH(COLUMN_NAME) FROM INFORMATION_SCHEMA.COLUMNS WHERE TABLE_SCHEMA='DB_NAME' and TABLE_NAME='pagina'"; $result = mysql_query($query) or die(mysql_error()); $output = array(); while ($row = mysql_fetch_assoc($result)) { $output[] = $row; } echo '<pre>'.print_r($output, true).'</pre>';
-
Well, there are no columns inside the table pagina Also, you should output the result of the query in php, I think.
-
Are you sure that your database and table's name is correct? Try: SHOW COLUMNS FROM `db_table` FROM `db_name`;
-
Try to run this to get a length of all columns, in case you have empty spaces somewhere. SELECT LENGTH(COLUMN_NAME) FROM INFORMATION_SCHEMA.COLUMNS WHERE TABLE_SCHEMA='DB_NAME' and TABLE_NAME='pagina'; Substitute the name of your database.
-
Hm..... can you give me the output of: locate mysql_secure_installation Does your database server is up?
-
Try to send this exampe from php.net: <?php $to = 'nobody@example.com'; $subject = 'the subject'; $message = 'hello'; $headers = 'From: webmaster@example.com' . "\r\n" . 'Reply-To: webmaster@example.com' . "\r\n" . 'X-Mailer: PHP/' . phpversion(); mail($to, $subject, $message, $headers);
-
Console or terminal is just a program that gives you access to different "shells" on the same or not machine. That's my understanding about that Yea, open up a terminal and try to run this program-http://dev.mysql.com/doc/refman/5.0/en/mysql-secure-installation.html
-
Open up the mysql console and run mysql_secure_installation and change the root password from there.
-
Don't change the utf8_general_ci collation in your database to another. It works just fine. Tested few times. Few things you need to check. 1. Make sure that your database collation and the tables which contain accented characters in their name are under utf8_general_ci. 2. Check that everything is speaking utf-8. HTML forms, pages or http headers in apache (if you're using it). 3. When you established the connection to the database and set a database table(s), before send the actual searching query string to DB, make sure that PHP is using a utf-8 connection to MySQL. You could use a mysql_set_charset('utf8', $link_identifier) (personally i prefer) or a SET NAMES utf8 mysql function. In this way, you will be sure that all data you really send is utf-8! In your first post, I don't see wher you've been set up a character encoding in php to DB.
-
You are doing something wrong. The regular expression is just a pattern that describes a set of strings, so you can compensate that
-
The link of your home page 'http://www.labtec.0fees.net/Gardenable/?page=home' is equivalent "http://www.labtec.0fees.net/Gardenable/index.php?page=home" So if you want this link to be much more pretty something like - 'http://www.labtec.0fees.net/Gardenable/Home/' go to .htaccess and rewrite a rule. Assuming, this file is located inside Gardenable directory. RewriteEngine on RewriteBase /Gardenable/ RewriteRule ^/?([a-zA-Z_]+)$ index.php?page=$1 [L PS: That link contains an invalid URL - 'http://localhost/Gardenable/home?page=home'
-
ZipArchive () problem with special characters in filenames
jazzman1 replied to gdfhghjdfghgfhf's topic in PHP Coding Help
Wow....I saw your urgent post onto the php freelancing section. That one could be very simple with 2-3 lines of code, no more. Just run a unzip linux command through bash with shell_exec in php and display the results on the page. That's all. PS: what happened here? -> http://forums.phpfreaks.com/topic/280579-regexiterator-recursive-iterator-cant-search-file-with-accents/ -
MySQL Select x FROM y WHERE z="a", b="c", d="e";
jazzman1 replied to SteelyDan's topic in PHP Coding Help
Hey Nick, few things I have to mention before to post my solution on that. 1. Your code looks a little bit messy. You need to separate the logic from the presentation, as you can see later. 2. Stop using all mysql_* functions, rather than start to learn the mysqli_* or pdo library. 3. Using too much AND....and....AND...and...AND after the "WHERE" clause points me out that there is something wrong in your database design. I highly recommend, you to watch these 9 videos. 4. I don't know how you validate the values of the html form fields, but you should spend a time to learn more of that too. So, try the code below and come back later on it, if you have any problems. Regards, jazz. <?php include_once 'header.php'; require_once 'login_builds.php'; include_once 'functions.php'; $db_server = mysql_connect($db_hostname, $db_username, $db_password); mysql_select_db($db_database) or die("Unable to select database: " . mysql_error()); if (isset($_POST['buildname']) || isset($_POST['weapon']) || isset($_POST['category']) || isset($_POST['id'])) { if ($_POST['buildname'] == "") { $buildname = "*"; } if ($_POST['weapon'] == "") { $weapon = "*"; } if ($_POST['category'] == "") { $category = "*"; } if ($_POST['id'] == "") { $id = "*"; } } $buildname = sanitizeString($_POST['buildname']); $searchstring = "SELECT buildname,weapon,category,id,author FROM weapons WHERE buildname='$buildname' AND weapon='$weapon' AND category='$category' AND id=$id"; $result = mysql_query($searchstring); if (!$result) die("Database access failed: " . mysql_error()); // end the logic of your script here and start to display your presentation ?> <table class="fixed" border="1"> <tr> <td class="newsbody">ID</td> <td class="newsbody">Build Name</td> <td class="newsbody">Weapon</td> <td class="newsbody">Category</td> <td class="newsbody">Author</td> </tr> <?php if (mysql_num_rows($result) == 0) { // check if there are no records found in the database echo '<tr><td colspan="5">No records found</td></tr>'; } else { while ($row = mysql_fetch_assoc($result)): // start table data layout ?> <tr> <td class="newsbody"><?php echo $row['id']; ?></td> <td class="newsbody"><a href="./builds/<?php echo $row['buildname'] ?>.php"><?php echo $row['buildname']; ?></a></td> <td class="newsbody"><?php echo $row['weapon'] ?></td> <td class="newsbody"><?php echo $row['category']; ?></td> <td class="newsbody"><?php echo $row['author']; ?></td> </tr> <?php endwhile; // end while loop and data layout } // end the else statement echo "</table>\n"; // end the table ?> <html xmlns="http://www.w3.org/1999/xhtml"> </body> -
What result you get if you try to echo the $thumbnail variable. $thumbnail = $_SERVER['DOCUMENT_ROOT'] . '/'. $arr[1] . '/' . $arr[2] . '/' . $url_id. '/original/8.jpg'; echo $thumbnail;
-
MySQL Select x FROM y WHERE z="a", b="c", d="e";
jazzman1 replied to SteelyDan's topic in PHP Coding Help
Good for you. I like people who listen jazz and rock. So, try that: $searchstring = "SELECT buildname,weapon,category,id,author FROM weapons WHERE buildname='$buildname' AND weapon='$weapon' AND category='$category' AND id=$id"; -
@psycho, I have to disagree with my reply too, but that was the first working thing that was jumped on the top of my head reading that post -easy and fast Sorry, if I disappoint you
-
MySQL Select x FROM y WHERE z="a", b="c", d="e";
jazzman1 replied to SteelyDan's topic in PHP Coding Help
Hey SteelyDan, there is a beautiful fussion jazz band with the same name. I like them very much Well, so far as I know that's not correct SQL statement especially after the "WHERE" clause. $searchstring = "SELECT buildname,weapon,category,id,author FROM weapons " . "WHERE buildname="$buildname", weapon="$weapon", category="$category", id="$id""; Also, it contains a php syntax error. Go to mysql' documentation and check how to use the sql where clause. -
Use () to separate the regEx class. Try, $regex = "/\[(.*?)\]\s?/"; OR, $regex = "~\[(.*?)\]\s?~";
- 3 replies
-
- array
- preg_split
-
(and 1 more)
Tagged with: