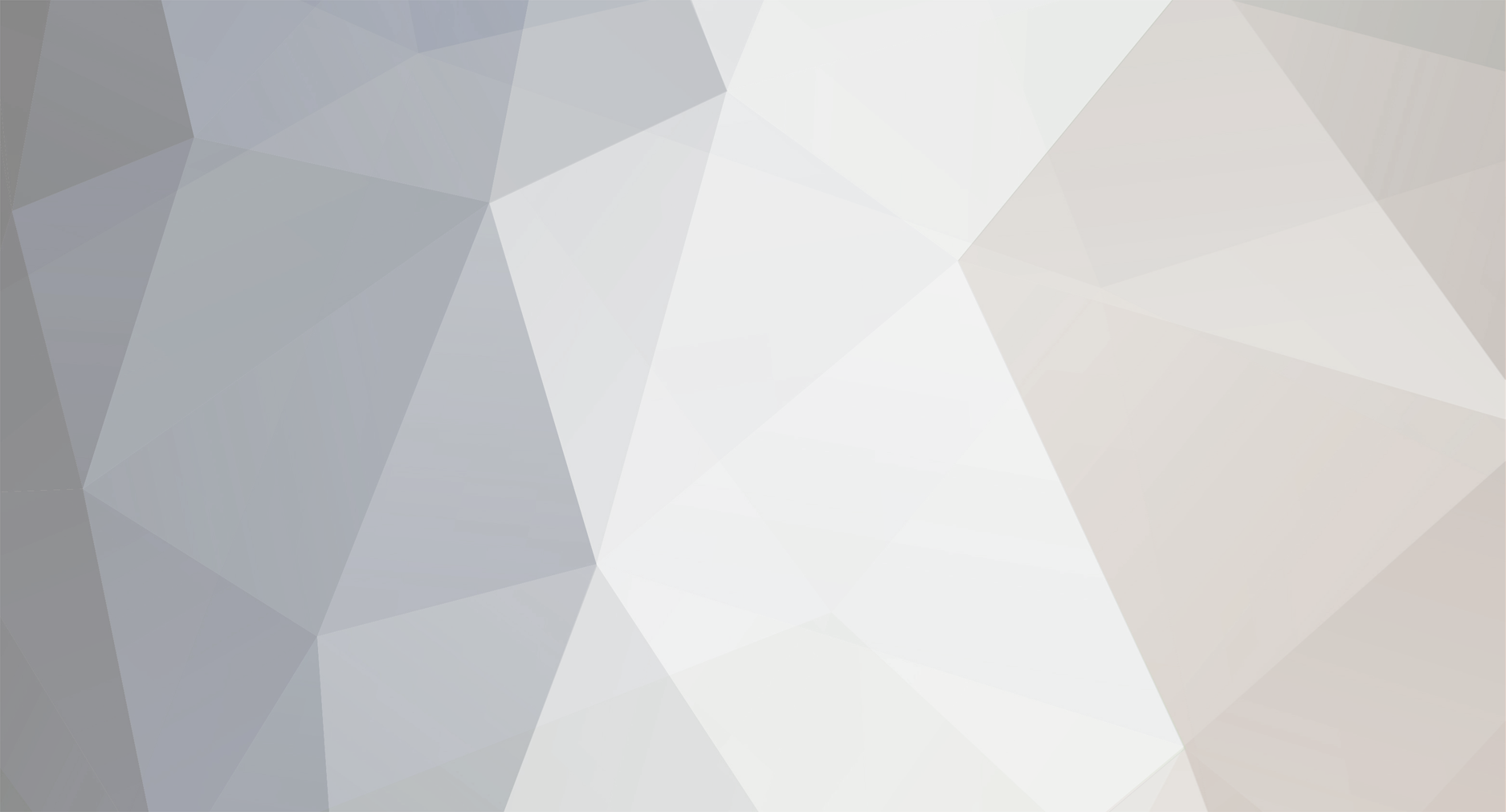
scootstah
-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Posts posted by scootstah
-
-
What exactly are you struggling with?
-
Are you connecting to the database server with a domain or an IP?
-
That is a back door which basically allows anyone to execute code on your server.
Sorry to say, but if the person that built your website did that, then you can not trust anything else he has done. Get rid of it, try to get your money back, and find a legit developer.
-
What are you trying to accomplish here? You're linking the same file in all cases. What is the point of all of those if statements?
-
Although it's worth mentioning that if you're willing to spend $15/month for Beanstalk, you could just buy better hosting. DigitalOcean has $5/mo VPS's that are excellent.
-
1
-
-
So if you have a repository of your own scripts/functions etc... Do you keep them outside of public_html?
Yes, typically. Only index.php needs to be in public_html. But that doesn't really matter in the context of your question.
-
1
-
-
__DIR__ returns the absolute path to the directory of the script it is executed from. If your site all runs through a single index.php file (like it should), then you can define a constant in index.php which would be your base app path. Like:
define('APPPATH', __DIR__);
Now when you include just doinclude APPPATH . '/somefile.php';
-
-
Where is this timestamp you are talking about?
-
The delay is part of the keyboard and operating system. Notice how if you hold down a letter in a text box, you get the same delay? That delay occurs on the keydown event as well.
One way to fix this is to assume that the key is held down until a keyup event occurs. So you press a key, it fires a keydown event and repeats that action until a keyup event occurs for the same key, which then stops the event. Does that make sense?
-
You need to include the namespace in the dynamic class name.
if(file_exists("{$this->_pathToIncludes}/{$class}.php")){ $class = "\test\\" . $class; $this->_inst = new $class(); }
-
Yikes. A lot to take in here. Let me attempt to pick out some of your problems and address them.
I don't know how to use this. I see that there's things like $query which I'm guessing is a variable because it has a $ in front of it, but does it need to be declared somewhere?
In that snippet, $query is being assigned to the value 'SELECT * FROM products WHERE categoryID = :category_id';
In the instructions it says that I need to Preparing, binding values and executing the query, but I have no clue what that means.
Prepared statements are a way to insert values into a query without the risk of SQL injection. SQL injection is a vulnerability which lets an attacker interrupt a query by entering specially crafted strings in user input. There is a ton of information readily available on SQL injection so I won't go into details. Basically, prepared statements mostly eliminate that vulnerability by binding the values internally in a safe manner.
The snippet you posted is an example of a prepared statement with bindings.
$query = 'SELECT * FROM products WHERE categoryID = :category_id'; $statement = $db->prepare($query); $statement->bindValue(':category_id', $category_id); $statement->execute();
Here, we are creating a named parameter in the query called :category_id which we will later use to bind the value to. The value gets bound in this line:$statement->bindValue(':category_id', $category_id);
So, internally, the :category_id parameter is replaced with the value of $category_id.Also we were told that part also needs to be in a try catch statement.
If configured to, PDO will throw exceptions when there are query errors, and errors in general. But, you did not tell it to do so. Right after you create a PDO connection you will want to add this line: $db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
Like this:
try { $db = new PDO($dsn, $username, $password); $db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); echo '<p>You are connected to the database!</p>'; } catch (PDOException $e) { $error_message = $e->getMessage(); echo "<p>An error occurred while connecting to the database: $error_message </p>"; exit(); }
Also, I noticed you are opening a PDO connection twice - you only need the one call, inside the try block.I know that foreach is a loop, but I don't know what the stuff in the parenthesis is.
The stuff in the parenthesis is the array to loop over, and the value of the current array index. Take this example:
$fruits = array('apples', 'bananas', 'oranges'); foreach ($fruits as $fruit) { }
foreach() will iterate over every item in $fruits. For each iteration, $fruit will be equal to the value of the current item. So on the first iteration $fruit is equal to apples, on the second iteration it is equal to bananas, and on the third and final iteration it is equal to oranges.And somehow I'm supposed to use POST to get the data. I vaguely understand POST.
POST is an HTTP method that is used to send data from the client (browser) to the server (PHP application). When you make an HTML form, most times you are doing so with the POST method. When the browser sends a POST request, like after submitting a form for example, there is a request body that is populated with the form data. Its structure differs depending on the encoding type used, but it might look something like this: city=Portland&state=Oregon
When PHP sees a POST request has been made, it does some internal stuff and populates the $_POST superglobal. Basically, it deconstructs that string and creates an array based on the key/value pair. So in my example it would create an array that looks like this:
array( 'city' => 'Portland', 'state' => 'Oregon', )
You can then access these values just like you would with any other array.$_POST['city']; // equal to Portland $_POST['state']; // equal to Oregon
You can then use these values to construct an SQL query (remember the named parameters earlier, with :category_id?) and select data from the database.Hopefully that clears up some things for you. If you like, or if none of that made sense, feel free to chat with me externally. You can find me here:
AOL Instant Messenger: scootstah@gmail.com
Yahoo Instant Messenger: scootstah@ymail.com
Google Hangouts: scott@scottbouchard.me
-
Where are you calling highlight_field()?
-
1
-
-
Can children also be parents? If so, you'll want a tree structure. I prefer the nested set model myself.
-
If you're using visiblity: hidden to make it "not visible", then the :visible selector will still select that element because it is still part of the DOM. You will either need to use display: none instead, or you'll have to filter your selection and check the visibility style.
-
Also you are creating a lot of extra work for yourself
At the moment you
- Select names from owners.
- User selects a name
- On submitting form
- select o_id from owners where the name matches
- use o_id in the insert
- Select o_id and name from from owners
- set the id as the option value so user selects id
(The same goes for the property)
You still need to do the query, to make sure that the user didn't pick an invalid value.
-
1
-
You probably have an empty result set now. You should always check for an empty result set instead of assuming there will be data returned.
-
$own and $pro are going to be mysqli_result objects. They do not have a __toString() method, so you can not use them as strings.
Typically you would use fetch_assoc() which will return an associative array containing your database column/value pairs.
-
You can use a service like Beanstalk to deploy to hosting that don't support Git. Basically, Beanstalk hosts your repository and then when you push, Beanstalk will use one of many deployment methods to send your code on to your server. You can tell it to automatically FTP your changes whenever you push.
It's not free, though. There may be some that are, I'm not sure.
-
1
-
-
Yes sorry, I was talking about X-Frame-Options.
-
It should be DENY, not NONE.
Source: https://developer.mozilla.org/en-US/docs/Web/HTTP/X-Frame-Options
-
X-XSS-Protection is supposed to be 1 if you want it enabled.
header("X-XSS-Protection: 1");
Also it's probably better to set these at the web server level to ensure they're always present. -
You have very little control over styling select boxes. You can change all of the option backgrounds, but you can't change the selected one or the hover color. You will have to use a Javascript replacement, such as Select2.
-
I don't really know what you're asking for. Right now, it starts at the current UTC+2 time, and it counts down to 0:00.
If you want it to start at a different time then you need to modify this portion here:
var date = new Date(); var offset = 2.00 * 60 + date.getTimezoneOffset(); var correctedDate = new Date(date.getTime() + offset * 60 * 1000);
button events
in HTML Help
Posted
Seems interesting. This is what I came up with: http://jsfiddle.net/ro79zjhh/
It's a little glitchy, sometimes it just flips with no transition.