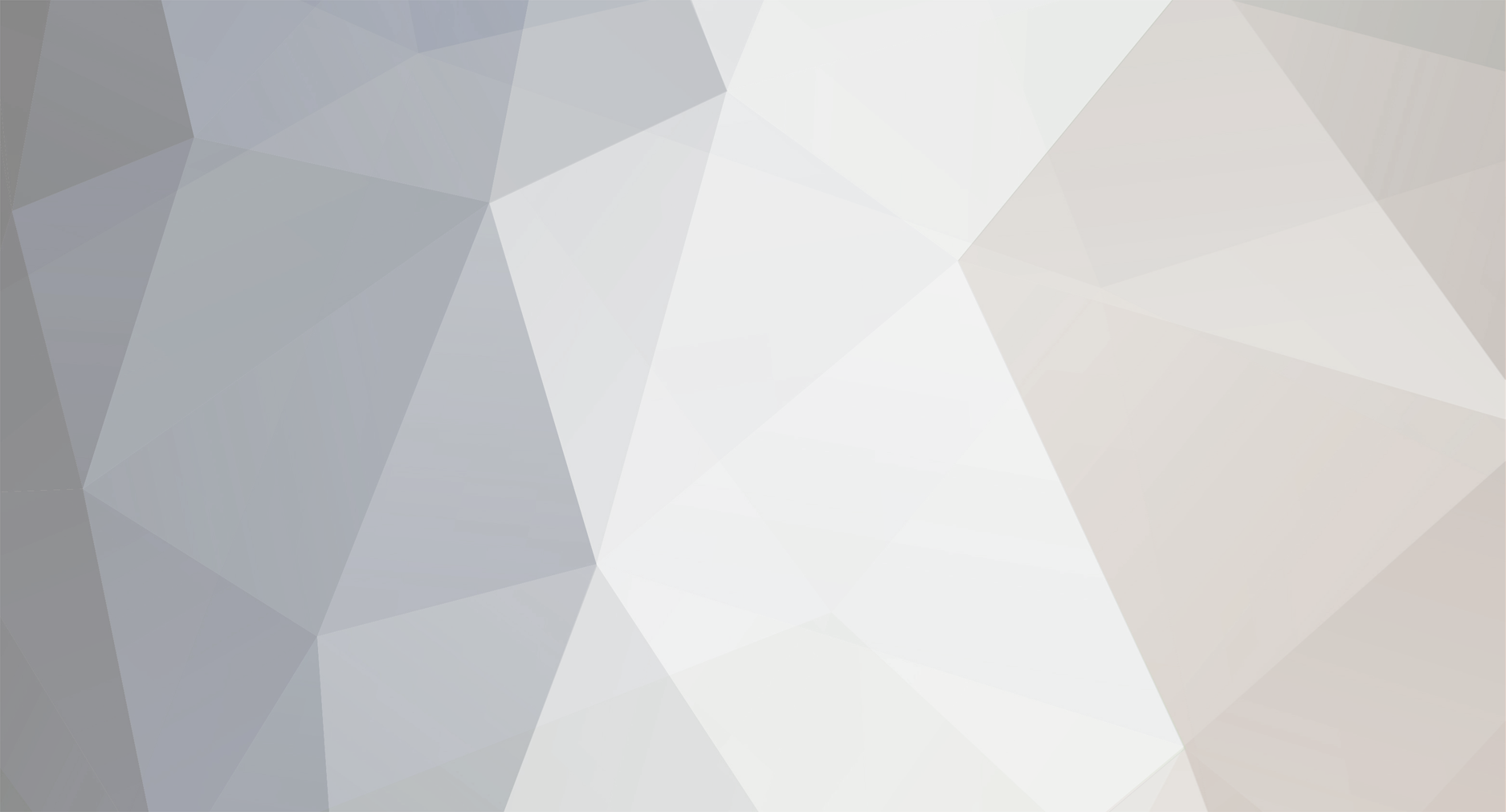
scootstah
-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Posts posted by scootstah
-
-
You would use a querystring parameter to pass the ID of a product to your "details" page. Something like: productDetails.php?productId=123
Then, on the productDetails.php page, you just look for $_GET['productId'] and select that product from the database.
Make sure you properly escape the $_GET value before you use it in the database.
-
It's not a 401 to Apache. You're just outputting a 401 to the browser. You'll need to include your error.php from your PHP script as well.
-
You are the only person in this thread that has been rude. The rest have given helpful advice and suggestions, and even wrote code samples doing what you asked for - even though you provided nothing on your end.
If you would like help in the future I recommend you actually put forth some effort on your part - instead of throwing a temper-tantrum when you aren't spoonfed the solution to your problems.
-
1
-
-
I have two methods, bar1() and bar2() which do different things. They both use method other() and use it 99% the same way and the only difference will be a conditional IF statement.
Instead of using an anonymous function, I "could" pass some value to other() and put the logic to use one of the two conditional checks there.
Would you recommend doing it that way, with an anonymous function, or some other way all together?
<?php class foo { public function bar1() { $a=123; $callback=function($b) use ($a){ return ($a==$b); }; $this->other($callback); } public function bar2() { $a=246; $callback=function($b) use ($a){ return ($a==2*$b); }; $this->other($callback); } private function other($cb) { $B=123; if($cb($B) ) { echo('$a == $b'); } } }
I mean, why not just do the logic in the method itself?
Anonymous functions are used when you need to pass a callable, but don't want to create a function or class just for that one thing. They're like one-time use functions. They can also be used to isolate scope.
What you're doing here though doesn't really make sense. You have two separate methods that both define an anonymous function - why not just put the logic from the anonymous function into the class method?
It's hard to really see where you're trying to go or what you're trying to do with your code, so it's hard to suggest something different. What problem are you trying to solve? How is this code actually going to be used in a real-world scenario; what is it doing?
-
I figure I should go crazy with them for a while, and then go back to reality!
I would argue that you should instead learn the proper application of callbacks and anonymous functions. I don't mean that in a mean way, but you are using them in a weird and non-typical way. Frankly, what you're trying to do doesn't make sense.
-
you all will yell at newbies for "asking for FREE help."
That's exactly what this is - free help. We volunteer our free time to help people. The least you could do is show some appreciation. Also, nobody is yelling, except for you.
And usually the answers suck, because you'll say something like "go to www.php.net/complicated_arrays" and tell me to "study up."
Yeah, that's how programming works. You have to be willing to look at a technical document and learn the material. You can't just write what people tell you to write - that's not how it works. By directing you to the manual, we are giving you the materials and resources to actually learn how certain things work, and become a better developer.
The point of this forum is to help people through problems with their code. The key phrase here is "their code". You didn't post any code. You said you spent 3 days working on it, so, what do you have to show us? Where are you stuck specifically? This forum is not, "hey I have this problem, can somebody write the solution for me?" If that's what you want, post in the Job Offerings section and pay for the work. But don't come here demanding free answers and then berate the people that give you the answer you don't want to hear.
-
2
-
-
You can log output like so:
/usr/local/bin/php -q /home/username/directory/directory/file-name.php >> /log/file/path.log
Make the path something that the CRON owner has write permissions to. Make sure that error reporting is turned on in the script. -
I don't think that still counts as a one-liner, lol.
-
Try just adding this to the top of your PHP script:
error_reporting(-1); ini_set('display_errors', 'On');
-
Did you restart Apache and/or PHP services afterwards? Are you sure that ini is being used?
-
I totally understand that the house is on fire, I am perfectly aware of all the problems, and that is exactly what I want to build, why?, because of the nature of the application of the page it needs that!
There is no way to tie a user to a specific device. There's no way to uniquely identify a device. The best you can do is use the user's IP address, but there's lots of problems with that. what if the user's IP changes frequently? What if the user regularly uses free hotspots? What if the user is part of a large network with other users that use your service, where they all have the same IP? What if the user uses a VPN or proxy service?
So, you simply can't do what you want.
-
Add these:
error_reporting = -1 display_errors = On
-
As i see, it's very hard to decrypt
It's not. You cannot secure PHP source code, and you'll just slow it down considerably by trying.
If you need to prevent people from seeing raw source code, don't use PHP - plain and simple.
-
What do you mean "the white page that PHP makes"? If you're getting just a white page then you're likely encountering a fatal error. Make sure error_reporting and display_errors are turned on in your php.ini
Alternately, check the Apache log for PHP errors.
-
You need to be more specific than "doesn't work".
-
$extension = $extensions[$check['mime']];
-
Use proper licensing, not code obfuscation.
-
It's cleaner to have a list of allowed MIME types and compare the uploaded file's MIME type to that list, as I have demonstrated in my earlier post.
or just remove it because I've already checked it before?
You checked that it's a valid image as determined by getimagesize(), but you have not checked that it matches the file types that you want to allow.
Another problem that I see is that you're assuming a specific naming convention for a file.
A file can have many dots in the name and still be valid. Instead, you should use the extension that is associated with the MIME type of the file.$resizedFile = $target_dir . "resized_" . $id . "_" . $time . "." . $kaboom[2];
$extensions = array( 'image/jpeg' => 'jpg', 'image/png' => 'png', 'image/gif' => 'gif', ); $extension = $extensions($check['mime']);
You can also use that array to check if the image is an allowed MIME type. You can either just do a simple isset($extensions[$check['mime']]), or use in_array:if (!in_array($check['mime'], array_keys($extensions))) { // not a valid image }
-
But not here:
if($imageFileType != "jpg" && $imageFileType != "png" && $imageFileType != "jpeg"
&& $imageFileType != "gif" ) { -
Anthony Ferrara - https://twitter.com/ircmaxell
Phil Sturgeon - https://twitter.com/philsturgeon
-
You can't "include" PHP from Javascript - the PHP has already finished executing by the time the Javascript is even sent to the browser. You will have to use AJAX requests to fetch content from PHP scripts.
-
1
-
-
You should actually get rid of this silly try-catch stuff altogether.
The whole point of making PDO throw exceptions is that you do not have to manually check every single query for errors. With exceptions, query errors are automatically detected and trigger a fatal error with all relevant information.
If you prefer to copy-and-paste the same error handling procedure over and over again, you don't need exceptions (but I'd rather do the opposite: keep the exceptions, get rid of the error code).
I agree. You can use set_exception_handler to capture any unhandled PDO exceptions to do what you're doing in one single location. If you actually need to handle an exception within your code then go ahead and use a try/catch block. But if you're simply displaying the exception message, that is totally unnecessary.
-
I have no idea where I'm going wrong with this code?
You're still only checking the file extension to determine if it is a valid jpg, png, or gif. The file extension is completely made up. You need to check the MIME type.
-
You will need to use an AJAX request to get the response from PHP.
First you should change the event to an "onSubmit" event instead of an "onClick" event.
$('.send-button').on('submit', function(){
Next we want to tell the form to not execute its default action when it is submitted. The default action would be to send a POST request to the URL specified in the action attribute. Since we'll be using AJAX to make that request ourselves, we don't want that functionality. We can do that with this:$('.send-button').on('submit', function(e){ e.preventDefault();
Next, we need to craft a data object to send to the server, using the values from the form.var data = { email: $('#senderEmail').val(), message: $('#message').val() };
Now we can send an AJAX request using the data from the form.$.ajax({ url: 'php/contact.php', type: 'POST', dataType: 'json', data: data });
The request will be sent, but nothing will happen on the client yet at this point. So we need to put in some handlers that will capture the server response. There is a success handler which will be called when the server request was successful. This is where you will show the "thank you" modal.$.ajax({ url: 'php/contact.php', type: 'POST', dataType: 'json', data: data, success: function(response){ if (response.success === true) { $('.th-popup').addClass('act'); setTimeout(function(){ $('.th-popup').removeClass('act'); }, 3000); } } });
Now the client will display the "thank you" modal if the physical request was successful and if the PHP script thinks it was successful. To determine if PHP thinks it is successful, we are checking for response.success inside of the success handler. This is something you'll want to add to your PHP script.So in your PHP script you'll want to return a JSON string that looks like this:
{ "success": true, "message": "Thanks for sending your message! We'll get back to you soon." }
We only want to send that kind of response if the request is from AJAX. You're sort of doing that check already, but not in the correct way. Instead of looking for an "ajax" querystring parameter, we can simply check for a specific header that is sent with AJAX requests.if (isset($_SERVER['HTTP_X_REQUESTED_WITH']) && strtolower($_SERVER['HTTP_X_REQUESTED_WITH']) == 'xmlhttprequest') {
That's kind of a long conditional so let's define a function instead.function isAjaxRequest() { return (isset($_SERVER['HTTP_X_REQUESTED_WITH']) && strtolower($_SERVER['HTTP_X_REQUESTED_WITH']) == 'xmlhttprequest'); }
Now then, let's make the response JSON. This will be replacing this code:// Return an appropriate response to the browser if ( isset($_GET["ajax"]) ) { echo $success ? "success" : "error"; } else {
// Return an appropriate response to the browser if (isAjaxRequest() === true) { if ($success === true) { $message = "Thanks for sending your message! We'll get back to you soon."; } else { $message = "There was a problem sending your message. Please try again."; } // send JSON response echo json_encode(array( 'success' => $success, 'message' => $message, )); // exit to make sure no other output is sent exit; } else {
Pay close attention to this part:echo json_encode(array( 'success' => $success, 'message' => $message, ));
See those keys, success and message? Well, those are what we use over in the AJAX success handler, on the response object. if (response.success === true) {The last thing to do would be to put the message received from the server into the "thank you" box, but I don't know how that looks. You'd simply need to select the element with jQuery and use the .text() method to apply the response.message data.
You may also want to add a error handler in case the request failed.
Here is what everything looks like all together:
Javascript
$('.send-button').on('submit', function(e){ e.preventDefault(); var data = { email: $('#senderEmail').val(), message: $('#message').val() }; $.ajax({ url: 'php/contact.php', type: 'POST', dataType: 'json', data: data, success: function(response){ if (response.success === true) { $('.th-popup').addClass('act'); setTimeout(function(){ $('.th-popup').removeClass('act'); }, 3000); } } }); });
PHP altered a little bit to cleanup/reduce duplication:<?php function isAjaxRequest() { return (isset($_SERVER['HTTP_X_REQUESTED_WITH']) && strtolower($_SERVER['HTTP_X_REQUESTED_WITH']) == 'xmlhttprequest'); } // Define some constants define( "RECIPIENT_NAME", "YOURNAME" ); define( "RECIPIENT_EMAIL", "YOUR@EMAIL.net" ); define( "EMAIL_SUBJECT", "Visitor Message" ); // Read the form values $success = false; $senderEmail = isset( $_POST['senderEmail'] ) ? preg_replace( "/[^\.\-\_\@a-zA-Z0-9]/", "", $_POST['senderEmail'] ) : ""; $message = isset( $_POST['message'] ) ? preg_replace( "/(From:|To:|BCC:|CC:|Subject:|Content-Type:)/", "", $_POST['message'] ) : ""; // If all values exist, send the email if ( $senderEmail && $message ) { $recipient = RECIPIENT_NAME . " <" . RECIPIENT_EMAIL . ">"; $headers = "From: " . $senderEmail . " <" . $senderEmail . ">"; $success = mail( $recipient, EMAIL_SUBJECT, $message, $headers ); if ($success === true) { $responseMessage = "Thanks for sending your message! We'll get back to you soon."; } else { $responseMessage = "There was a problem sending your message. Please try again."; } // Return an appropriate response to the browser if (isAjaxRequest() === true) { // send JSON response echo json_encode(array( 'success' => $success, 'message' => $responseMessage, )); // exit to make sure no other output is sent exit; } else { ?> <html> <head> <title>Thanks!</title> </head> <body> <p><?php echo $responseMessage; ?></p> <p>Click your browser's Back button to return to the page.</p> </body> </html> <?php } } else { // you'll want to handle this state - where $senderEmail or $message // are empty strings. }
Prevent default behaviour jQuery
in Javascript Help
Posted
Just an FYI, that is not limited to jQuery - that is part of Javascript itself. https://developer.mozilla.org/en-US/docs/Web/API/Event/stopPropagation