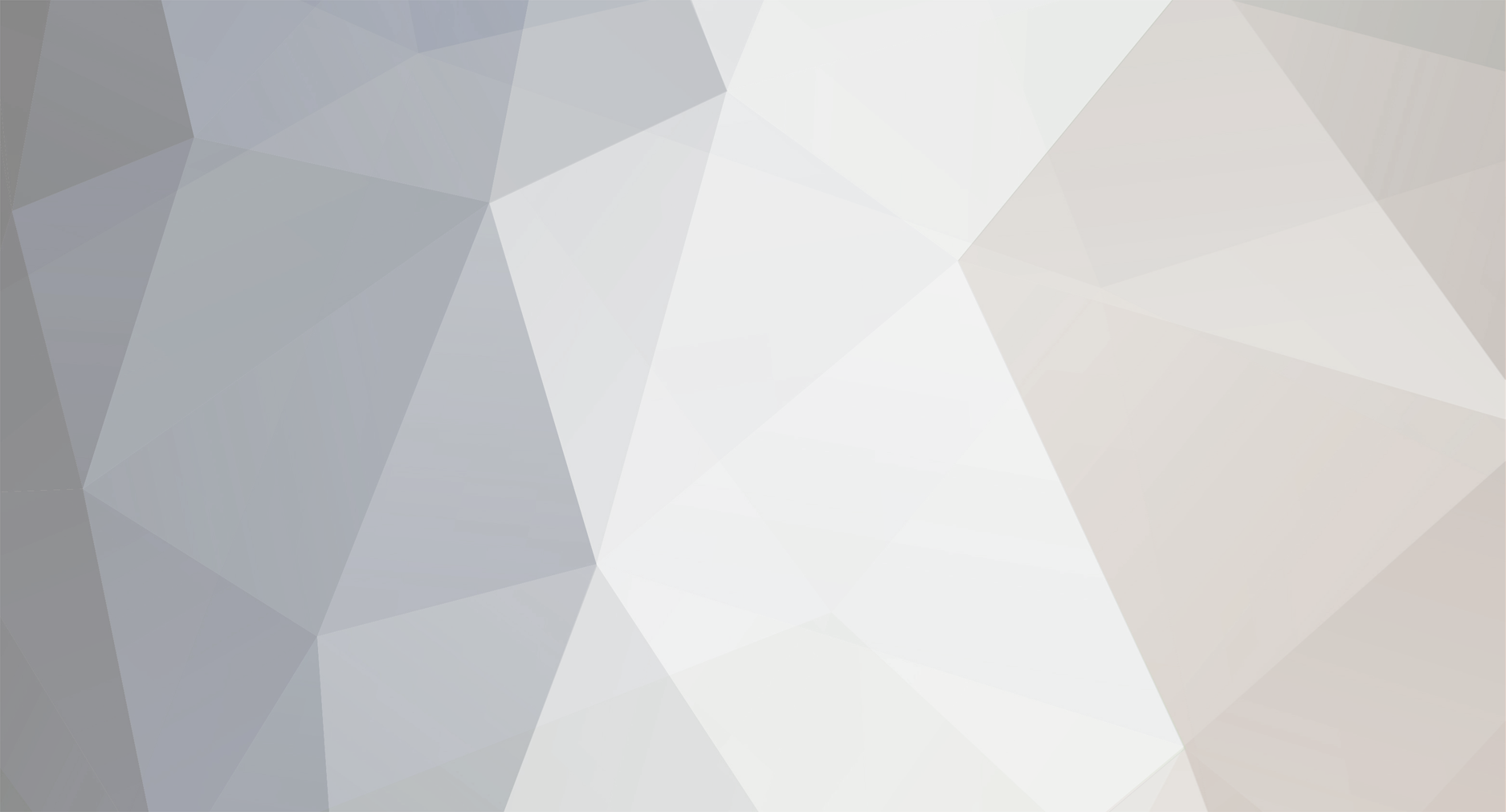
scootstah
-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Posts posted by scootstah
-
-
This works bro but its still not a countdown for me, it just says the time, it should say:
24:00
23:59
23:58
Yes, which is exactly what it does.
I have changed the interval time to one second, to simulate. View here: http://jsfiddle.net/tg9Lr8ym/
It counts down just as you describe it should.
-
The EXIF data should contain an "Orientation" if the image is rotated. You can read this data and apply the necessary corrections.
-
You don't want to connect directly to the database from your mobile app. You'd definitely want to go through some kind of service such as a REST or SOAP API, like you mentioned.
-
It does, assuming you give cover a width and height so it actually takes up space. http://jsfiddle.net/pznoh7o6/
-
Can you post your schema and what you're trying to search for with $keywords?
Kind of sounds like a not very efficient schema layout.
-
$password = md5($password); // Encrypt password
Do not use MD5 for hashing passwords. Use password_hash and password_verify.
-
You can start with the manual.
-
-
1
-
-
You could use implode.
$array = array(); while($row = mysqli_fetch_array($res)){ $array[] = implode('<br />', $row); }
-
Sorry, missed a dollar. for ($i = 1; $i <= $max; $i++) {
I did look for about 5 minutes. It gave me a headache. Hence my question: "How do I turn glob() values into an array?" .. it wasn't long after that I started to feel nauseous.. so I decided to sit back, have a think -- and write up some code that I understand. I can see you're unimpressed, but my solution was to the best of my ability. I am not a very good programmer; but I try hard.
You're going to have to put more effort into learning programming than spending 5 minutes on a problem before giving up because you "feel nauseous". The manual and user contributed notes pretty much tells you what you need to do to use glob.
<?php $dirs = array(); foreach(glob('[0-9]*', GLOB_ONLYDIR) as $dir) { if (!is_numeric($dir)) { continue; } $dirs[] = $dir; } echo max($dirs);
-
Did you even bother to read the manual for the functions I gave you?
If you want to hard code it, at the very least do this so it's not a maintenance nightmare:
$max = 20; for ($i = 1; $i <= $max; i++) { $dir = $path . '/' . $i; if (!file_exists($dir)) { echo 'Creating dir ' . $i; break; } }
-
You're submitting an empty form the second time, so if ($password_submitted == true) { fails.
What you're trying to do is inherently flawed though. In order to recreate the hash, you have to use the value that was just submitted as a password. But since you're not storing the hash, you'd have to generate a new hash when you try to compare it. Since bcrypt creates a unique salt, the new hash will be different than the first one.
You need to be storing the hash in some way (like a session, a database, a file, etc) that persists between page loads.
-
It returns an array.
Return Values
Returns an array containing the matched files/directories, an empty array if no file matched or FALSE on error.
-
-
The easiest way to handle stuff like this is to use a config file that has different values depending which environment it's in.
So something like:
config.php on development environment
<?php define('BASEURL', 'http://localhost/project_name/sub_project_name');
config.php on live environment<?php define('BASEURL', 'http://www.project_name.com/sub_project_name');
index.php<?php require 'config.php'; echo BASEURL;
-
You could manually build the offset yourself and send a request for each "page".
-
You need to put the script at the bottom of the body, after the rest of the DOM.
<body>
And yes, it does count down on JSFiddle - every 1 minute.
<div id="timer"></div>
<script>
function countdown(startDate, el)
{
var hour = startDate.getHours();
var min = startDate.getMinutes();
var interval;
var f = function(){
if (min == -1) {
min = 59;
hour--;
}
if (min < 10) {
min = "0" + min;
}
var time = hour + ':' + min;
el.innerHTML = time;
if (hour == 0 && min == 00) {
clearInterval(interval);
}
min--;
};
f();
interval = setInterval(f, 60 * 1000);
}
var date = new Date();
var offset = 2.00 * 60 + date.getTimezoneOffset();
var correctedDate = new Date(date.getTime() + offset * 60 * 1000);
countdown(correctedDate, document.getElementById('timer'));
</script>
</body> -
Hi @scootstah,Based on your post, I would like to ask how name="gender[<?php echo $row['id'] ?>]
work? Thanks
It creates an array of values in the corresponding $_POST variable. Normally you would just have a single value for each form element. So if you did <input name="gender" type="radio" value="male" />, then $_POST['gender'] would just be male. But if you did <input name="gender[1]" type="radio" value="male" />, then now $_POST['gender'] will be an array... like:
array(1 => 'male')
If we use the ID of the row inside the square brackets, name="gender[<?php echo $row['id']", then we can iterate through the $_POST array and determine which gender value matches which row.Since you're using that weird schema you probably want to put the ID of the person in the form name, and then do an INSERT ... ON DUPLICATE KEY UPDATE query.
-
I've already shown you how you can save the form results to the database. Why don't you take what I've given you and try to make something work? Post back with your own code and where you are having problems.
-
Okay, so check the network tab after adding public/ and see what URL it is now trying.
-
So if you point your browser to localhost/laravel/public/css/styles.css, it doesn't work?
Can you post your directory structure?
-
If your assets are in public/css, and it's only loading css, then it looks like you should change the asset path to include public.
<link rel="stylesheet" href="{!! asset('public/css/bootstrap.min.css') !!}"> <link rel="stylesheet" href="{!! asset('public/css/styles.css') !!}">
-
What "php confirmation" are we looking for in that massive wall of code?
-
You should always store datetimes in a DB as type DATETIME, format YYYY-MM-DD HH:MM:SS. Other formats are largely useless.
This is pretty much the answer you should be going with. Anything else is just a bandaid. It is pointless to store a date/time in a format that MySQL does not understand, because you're then removing a whole ton of modifiers that MySQL can use to retrieve/sort/order your data. And, it's much more usable in PHP without making hacky workarounds.
My suggestion would be to write a script that converts your weird format into a proper one, and then loop over each database result and update it to the correct MySQL datetime format.
-
1
-
JS Countdown script
in Javascript Help
Posted
So you just need to adjust this line to reset the hours to 24, instead of stopping the interval.