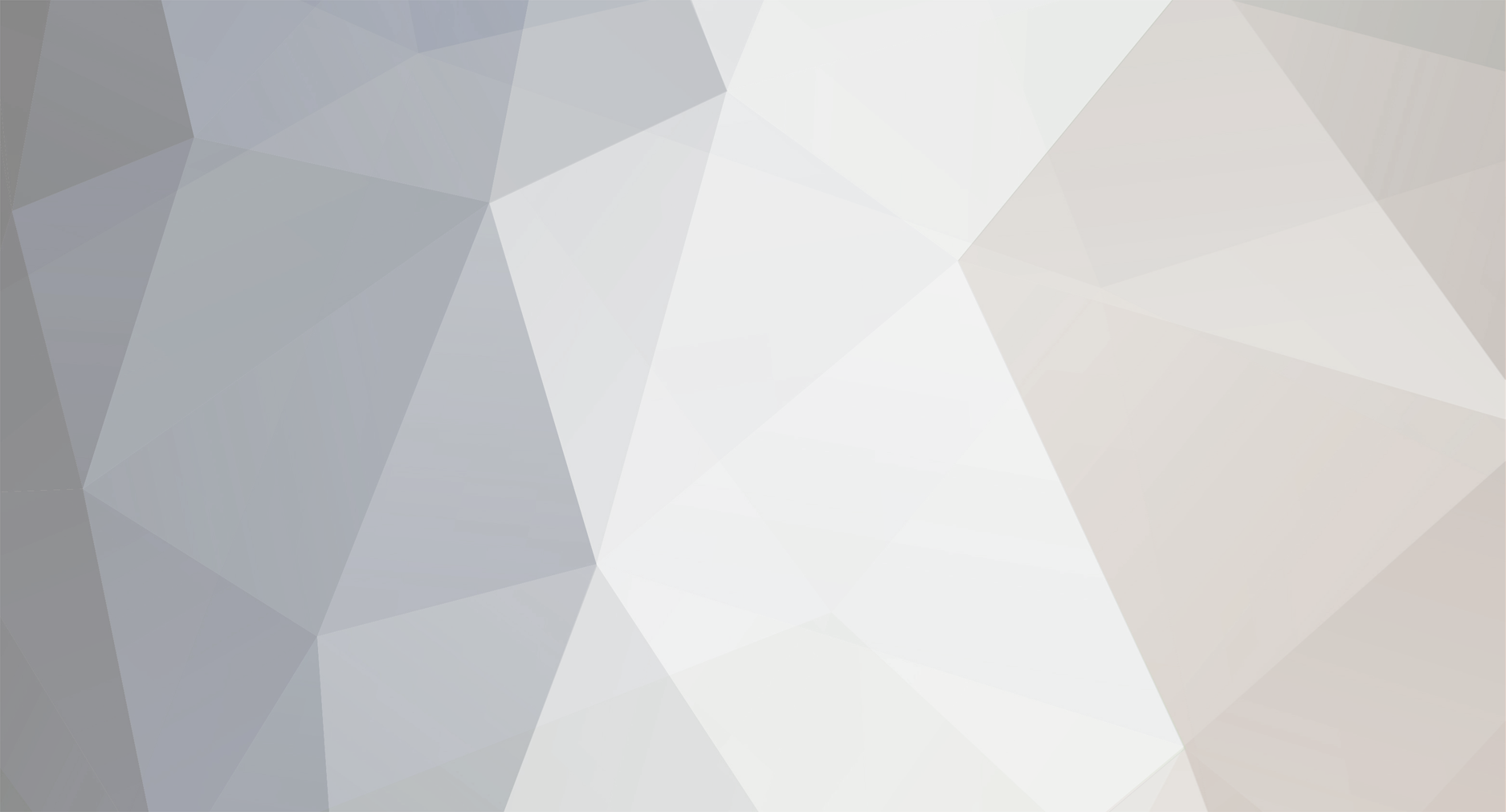
scootstah
-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Posts posted by scootstah
-
-
Interesting, but I don't see much of a use case for that.
-
This site is susceptible as well. What does that have to do with your site?
-
Thank you so much for the help and suggestion, guys. I will make sure to take application on what you have taught me !
The flow of this program is that i want the user to input how many questions he/she would like to create and the system would automatically generate the inputted questions along with the answers. So, i ended up using this loop:
for ($x = 1; $x <= $MC; $x++) { echo "<form metod = 'POST' action = 'createquestions.php'>"; echo "Question Number $items:"; echo "<input type = 'text' name = 'questions' style='width: 500px'><br><br>"; echo "A. "; echo "<input type = 'text' name = 'ans1'>"; echo "B. "; echo "<input type = 'text' name = 'ans2'><br>"; echo "C. "; echo "<input type = 'text' name = 'ans3'>"; echo "D. "; echo "<input type = 'text' name = 'ans4'><br>"; echo "Correct Answer: "; echo "<input type = 'text' name ='cans'><br><br>"; $items++; echo "</form>"; }
I know i have a lot of things to work with, but i'm planning to get this function first before designing the page.What this is going to do is create a <form></form> block for every item. Since your submit button is in yet another, totally separate form, none of this data is ever going to make it to your PHP script. Only the form inputs that are inside of the <form></form> block that is submitted is going to get sent to the server.
-
Why would each client have their own credentials to the database?
Typically your application should have a single set of database credentials.
-
1
-
-
This forum is more for answering specific coding problems you may be having with an application, such as Magento. For example if you began the process of creating your own video gallery plugin and ran into specific difficulties, then we would be able to help.
I'm sure that Magento has multiple existing plugins that will help you achieve your goal. For example this is the very first Google result.
If you want to create it yourself you're going to need to do some research on creating custom Magento plugins. You'll then have to do some research on how to embed videos from Youtube, Vimeo and Dailymotion.
-
And it's “method”, not “metod”.
It took me a second to figure out where you were seeing that, which made me realize that OP has three separate forms.
Your $_POST indices are empty because the form that you actually submitted only has the submit button. Your other form elements need to be inside of the form that gets submitted for their data to be sent.
-
Please copy/paste code to your reply using the forum's code tags instead of posting a screenshot...a screenshot is very hard for us to work with.
actions ='createquestions.php'
This should be "action" not "actions".
-
If someone can tell me how to do what I asked for I will be really thankful.
Sure. What you want to do is not possible, sorry. The system you're trying to build is fundamentally flawed and easily circumvented. Why are you adamant in security for this one particular, unnecessary thing when you have blatantly ignored security in many other areas?
Hmm, why you guys all are complaining about stuff that I am the one who should worry about!
Because we are passionate about what we do and we see someone doing it horribly wrong. We also see someone making the web an unsafe place. Do you know how many big time sites have been compromised recently that have published huge recovered password lists, due to similar security problems? It's insane, ridiculous, and completely preventable.
-
1
-
-
You said you wanted to connect to a websocket, and the WebSocket object is how you do that.
-
Can you post the function definition?
-
$number = get_additional($tcgname,'Trades');
-
Why not use the WebSocket class?
-
We cannot see your screen. You're going to have to be more specific when you say things like "didn't work".
-
echo get_additional($tcgname,'Trades');
Can we see what that function does?
-
Why are you using output buffering and then echo'ing it 4 lines later? Completely unnecessary.
What are you trying to achieve with your code? What problem are you trying to solve?
-
Those errors are pretty descriptive.
You're trying to use an array index that doesn't exist. It doesn't exist yet if you haven't submitted your form. You should either do isset($_POST['submit']) or just check for a non-empty $_POST array, !empty($_POST)Notice: Undefined index: submit in C:\xampp\htdocs\xenxarra\_php\graphicArt\testmail.php on line 2
You have not defined $errors in this scope. You should initialize $errors at the top of your script so that it's available everywhere. As an aside, you don't need to be doing unset($errors) - it cannot persist between page reloads.Notice: Undefined variable: errors in C:\xampp\htdocs\xenxarra\_php\graphicArt\testmail.php on line 34
Same as above.Notice: Undefined variable: errors in C:\xampp\htdocs\xenxarra\_php\graphicArt\testmail.php on line 36
Since $errors isn't defined, it therefore is not an array or iterator, so it can't be used with foreach(). In addition, you're checking that the array is empty and not that it is not empty. if (count($errors)==0) { should be if (count($errors) > 0) { or, simply, if (!empty($errors)) {Warning: Invalid argument supplied for foreach() in C:\xampp\htdocs\xenxarra\_php\graphicArt\testmail.php on line 36
-
What are your errors?
-
I don't believe that is possible with PHP. You're going to want to find some kind of command line library that can process and encode MP3's. You can then call that from PHP.
-
The parameter was added in 5.3.6. But still, very outdated. You should be on 5.6.
-
You're using a very old version of PHP then. The parameter was added in PHP5.3. You should look into updating to 5.6 because you're not getting any more security updates to the version you're running.
-
Javascript uses prototypal inheritance. It doesn't even have classes, so you can't really compare the two.
Using anonymous functions like you are makes unit testing very difficult, makes your code tightly coupled, and doesn't really allow for reusability.
-
And what would that error be?
-
This is what I came up with:
<?php $PSN_url = 'https://status.playstation.com/en-us/'; $PSNhtml = file_get_contents($PSN_url); $PSNdom = new DOMDocument(); @$PSNdom->loadHTML($PSNhtml); $PSNxpath = new DOMXPath($PSNdom); //PSN Query $PSN_query = "//div/ul[@class='main-content']//li"; $PSN_rows = $PSNxpath->query($PSN_query); $statuses = array(); //PSN Status foreach ($PSN_rows as $PSN_object){ // get the raw HTML of this node so that we can // examine the status image $html = $PSN_object->ownerDocument->saveHTML($PSN_object); // determine if this service is up // the image "./images/green.png" means the service is up $isUp = (preg_match('/img src="\.\/images\/green\.png"/', $html) === 1); // add this service to the statuses list $statuses[trim($PSN_object->nodeValue)] = $isUp; } echo '<pre>' . print_r($statuses, true) . '</pre>';
It will look at each of the services on that page and determine if there is a "green.png" image, which would presumably mean that service is up. It will create an array of each service with a true/false status. This is the output:Array ( [Account Management] => 1 [Sign In] => 1 [Create Account] => 1 [Gaming and Social] => 1 [PlayStation™Now] => 1 [PlayStation™Video] => 1 [PlayStation™Vue] => 1 [PlayStation®Store] => 1 [Purchase] => 1 [Download] => 1 [Browse] => 1 [Search] => 1 [Redeem Voucher] => 1 )
EDIT: Here's the same thing but with strpos instead of preg_match (regex wasn't really necessary)
<?php $PSN_url = 'https://status.playstation.com/en-us/'; $PSNhtml = file_get_contents($PSN_url); $PSNdom = new DOMDocument(); @$PSNdom->loadHTML($PSNhtml); $PSNxpath = new DOMXPath($PSNdom); //PSN Query $PSN_query = "//div/ul[@class='main-content']//li"; $PSN_rows = $PSNxpath->query($PSN_query); $statuses = array(); //PSN Status foreach ($PSN_rows as $PSN_object){ // get the raw HTML of this node so that we can // examine the status image $html = $PSN_object->ownerDocument->saveHTML($PSN_object); // determine if this service is up // the image "./images/green.png" means the service is up $isUp = (strpos($html, 'src="./images/green.png"') !== false); // add this service to the statuses list $statuses[trim($PSN_object->nodeValue)] = $isUp; } echo '<pre>' . print_r($statuses, true) . '</pre>';
-
Put the id as the option value.
$dropdown .= "\r\n<option value='{$row['id']}'>{$row['type']}</option>";
Then on "report.php" you can get the id from the $_POST variable.$id = $_POST['items']
PHP bootstrap contact form modul help please
in PHP Coding Help
Posted
You haven't posted the Javascript code responsible for handling your contact form submission.