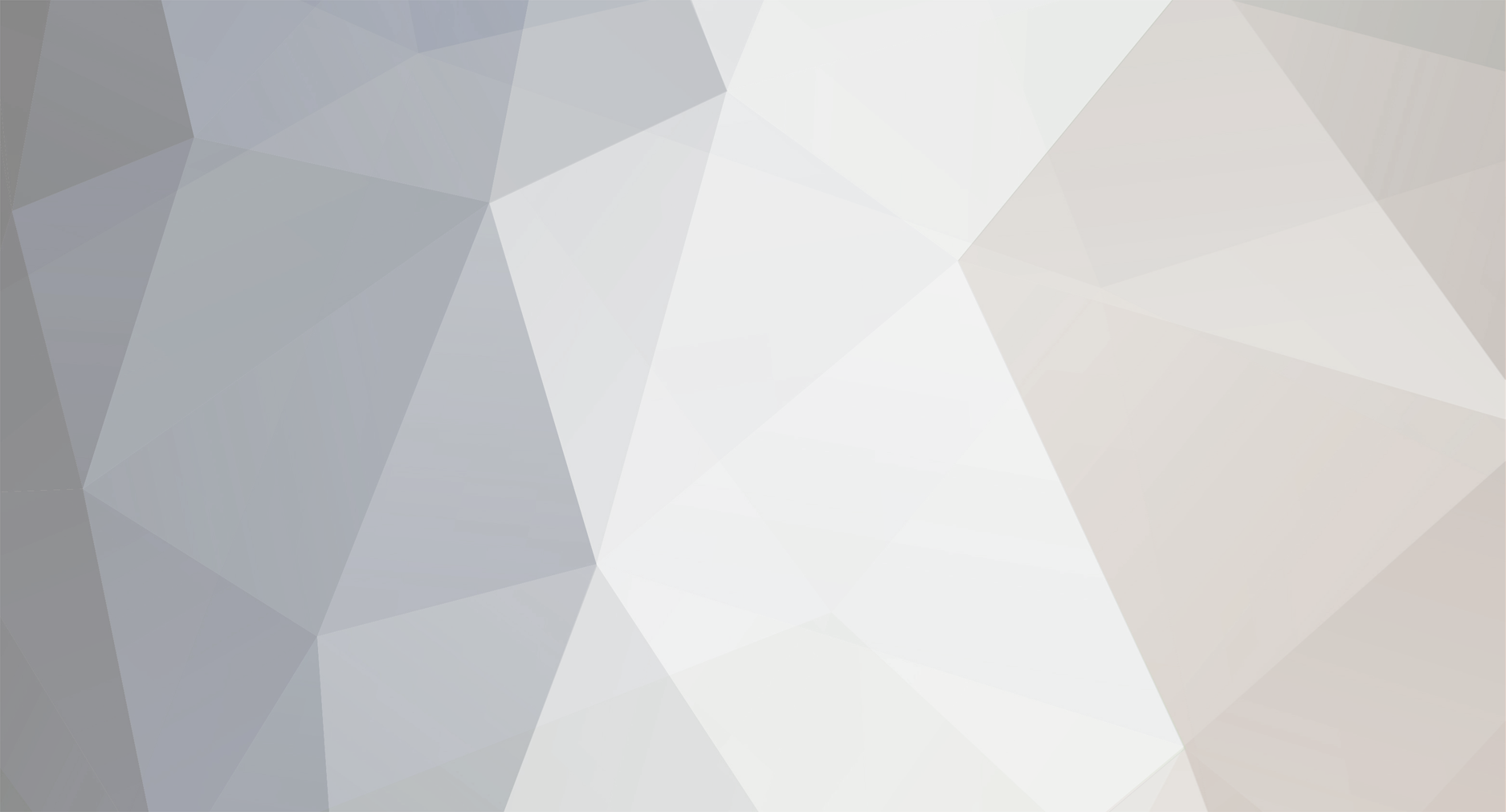
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Implementing a simple audit system
scootstah replied to NotionCommotion's topic in Application Design
I've been using Symfony2 with Doctrine for a long while now, so I will tell you how I accomplish this with that. Firstly, everything is an object in Symfony2. Using Doctrine ORM, all of the database tables are mapped to model classes/objects. Then, in a log table, it would store the primary key of the changed record, the primary key of the user who made the change, a timestamp, a serialized chunk of data changed, what operation was used (create, update, delete, etc), and the object class that the change was made on. So, you're on the right track. I would argue that it's good to store the actual change set, that way you know what actually changed. This can be important in some situations, and it also makes it possible to revert data back to the previous state. You don't necessarily need to maintain foreign key integrity in the audit table. Because if a record was deleted, the ID that it points to will be gone anyway. You don't want your audit log to be erased if an object is deleted. -
Is the "Execute failed" part showing?
-
Using PHP to log into another website and use their search.
scootstah replied to blmg2009's topic in PHP Coding Help
Yes it's possible. cURL can save and send cookies just like a browser can. -
That is specific to the implementation in that piece of software. If there is no resources or technical support for the platform then you'll probably have to dig through and figure out how they are generating the token.
-
I typically use a full height/full width fixed position element for this purpose. It being fixed position means that it will always be in your face no matter where you are on the page and no matter if you scroll.
-
Sounds like you just need to include the CSRF token in the request.
-
This is three blogs on one domain, but they are separate installations, with separate databases and environments. If that satisfies the requirements then it is the best way to go, in my opinion.
-
It is important to develop with error reporting turned on. You would have immediately seen an error for an undefined variable and figured out the problem without having to post here.
-
PHPMailer is a nice wrapper library for PHP's mail(), essentially. It supports any method of sending mail that PHP regularly would.
-
mail server or mail client to install to send email in php?
scootstah replied to colap's topic in PHP Coding Help
Any of the above. Ultimately you need a mail server to send email, but that doesn't mean you have to install one. You can connect to an external SMTP server (such as Gmail) and send mail with no problems. In fact if you don't know what you're doing, that is the approach that I recommend. -
It totally depends how you want the architecture. I'm not aware of anything off the top of my head that does it out of the box, but you can pretty easily set up multiple Wordpress or other CMS's to be on subdomains or under their own URI. For example, blog1.example.com, blog2.example.com, blog3.example.com - or - example.com/blog1, example.com/blog2, example.com/blog3. You can pretty easily configure both of these approaches to have their own virtual hosts and thus, their own docroots, configs, etc. Themes have nothing to do with PHP. You can either find themes that are built using plain HTML/CSS, or themes for a specific platform. But it has nothing to do with PHP.
-
I absolutely encourage this behavior. For me, the best way to learn how some system works is to build it myself and see all of what goes into it. But, if you were to need something in a production environment I would suggest something that already exists and is more polished. At the very least extend something that already exists - then it can be custom, built the way you want, but it's still solid. That's not really your burden. Remember that popular open source libraries are community-driven, and lots of people maintain, test, and fix the code. Depending on popularity, usually security concerns are found and addressed quickly. On the other hand, if you are the sole maintainer, how will you even know if you have a security problem? Attackers could be exploiting vulnerabilities for a long time before you stumble upon them.
-
Your database class should not be tightly coupled to any particular database schema. Doing so prevents your library from being portable, because now everyone's project needs to exactly match your database schema or it will not work. It is not necessary for you to build something like that. PHP already has a built in database driver called PDO which is already compatible with a whole bunch of database vendors using a single abstracted API. There is absolutely no reason you should attempt to build your own version of PDO. EDIT: But remember, that is another external dependency. Your classes should not make definitive decisions about dependencies if at all possible. For example instead of just depending on PDO throughout your library, create a new database interface that it depends on instead. Then you can implement many kinds of drivers that use that interface (PDO, MySQLI, etc). That way people are free to use whatever infrastructure they want to, and your library won't care. No. Bad. rand() is not meant to create cryptographically random and unique tokens, which is what you want for such a system. You want openssl_random_pseudo_bytes() instead. Also, you don't need to store the username in the cookie - just store the token.
-
I'm still not sure why you do not stick with an existing product, like Twig. All of the hassle is already done, and it's already been tested by the masses for security holes and such. Plus people don't have to learn yet another templating syntax.
-
At a glance, your classes have waaaay too much responsibility, and are way too tightly coupled. For example your User class is creating a new database connection, when it should be given an instantiated database object with dependency injection. Your User class construct is also doing way way too many things. A construct should be used to set up the class, not do a bunch of logic. Your User class should not be responsible for creating database tables and inserting initial records. Likewise, your database class should not be responsible for creating database tables either. Since your classes are very tightly coupled, this library is not very portable. It relies on the fact that a database connection is to be made, that specific tables must be created with specific columns, that data must be inserted in a specific way, that certain constants are required to exist, that things like $_SESSION are available, and that that is the way the session is accessed, etc. I recommend you do some more research on the principles of OOP. In particular, check out the Single Responsibility Principle, and Dependency Injection. Props for using password_hash() and password_verify() though.
-
['total_count'] ['%'] ['games'] ['name'];Where did you come up with this syntax? What do you think this is doing?
-
agree/disagree versus like
scootstah replied to QuickOldCar's topic in PHPFreaks.com Website Feedback
Agree. It's silly but some people might get offended if someone "disagree's" with their post, when they were trying to be helpful. I think it's fine to just quote them and correct them in a positive way. -
requinix is correct. See here: https://github.com/attdevsupport/ATT_APIPlatform_SampleApps/blob/master/RESTFul/Speech/PHP/app1/lib/Speech/NBest.php See all of those "getX()" functions? Those are called "getters", to retrieve the private/protected properties of a class, which is very common in object-oriented programming. EDIT: Oops, didn't see the file was uploaded here. Guess I google'd for nothing. Oh well.
-
Because you're continuously adding to an array on every iteration. $myArray[] = $row this pushes $row into the array on every iteration. At the end of the loop, $myArray contains the entire contents of your database result. That variable isn't even necessary. Just do: $message = json_encode(array($row));
-
I agree. A traditional forum posting style such as this does not lend itself very well to Q&A type threads. It's easy for multiple people to suggest something different, which makes the conversation flow difficult to follow. Honestly, something like the way Reddit threads work would be ideal, in my opinion. That way discussion can be forked and all replies to that sub-discussion are easy to follow and on-point. I have no idea if anything like that exists for IPB, or if it would have to be all custom. It would be a big change either way.
-
Thanks. I feel so sorry and sad for my daughter. She's the most precious little thing in the world and doesn't deserve this. She was born 6 weeks premature with complications and had to stay in the NICU for almost a month. It would be so much easier if there wasn't a kid involved.
-
I apologize if this is inappropriate or not suited to this board. I just need to vent. My head is spinning and I can't make sense of anything. Last night at midnight, my girlfriend of 5 years and mother of my beautiful 2 year old daughter confessed to me that she cheated on me, and wanted to end our relationship. She claims she was unhappy for a long time, even though that absolutely did not seem to be the case. From everyone else's perspective we were a perfect couple. Very very strong chemistry and bond. We never fought, rarely had arguments, and were just an all-around happy couple. Or so I thought, anyway. I did not see any signs of her unhappiness, nor did anyone else. Nor did she communicate anything. The person that I have grown with for 5 years is not the person that spoke to me last night. I do not know what happened, and she cannot tell me. She is completely unwilling to try to save our relationship, even for our child's sake. I do not understand how a person that I have been in the presence of every single day for 5 years can just up and leave everything behind in a split second, without even thinking twice. I am an emotional train wreck. I ball my eyes out at the slightest memory of our happiness. I cannot stand to think of waking up every morning without her by my side. I will never in my life love someone as much as I love her. My heart is absolutely destroyed. Sorry guys. I just need a vent. I don't know what to do.
-
I meant your php.ini
-
Can you post that file here please? Make sure to use the code tags by clicking the <> button.
-
Where did you turn on error reporting? It must be set in the php.ini to capture certain fatal errors, otherwise the error occurs before the code to turn on errors is executed. In your php.ini make sure display_errors is set to On and error_reporting is set to -1 And make sure to restart Apache after the changes.